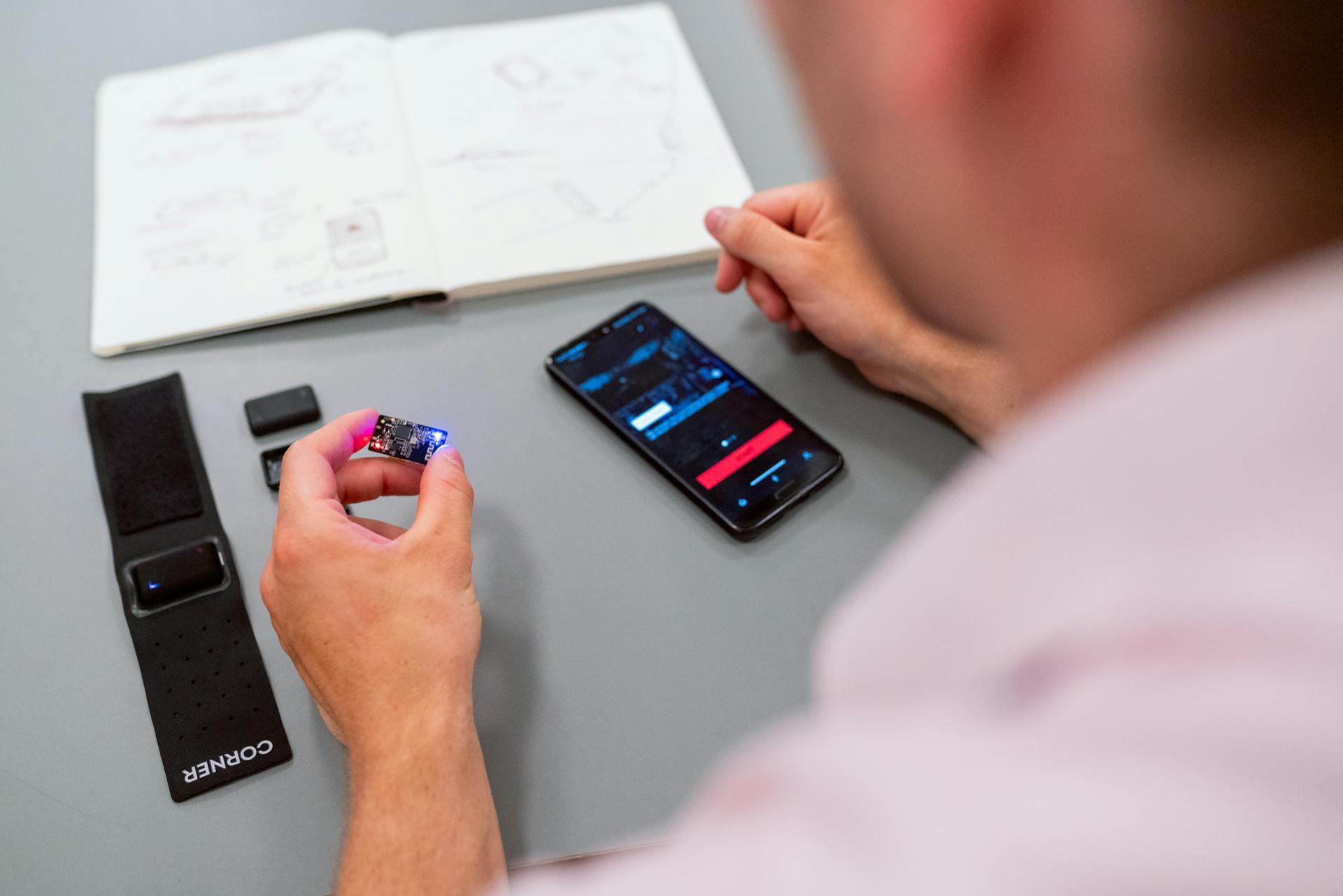
To get started with implementing a Next.js file upload API, you'll need to install the required packages, including `next` and `sharp`.
First, create a new Next.js project using the `create-next-app` command. This will set up a basic project structure and dependencies.
For file upload, you'll be using the `multer` middleware to handle multipart/form-data requests. This is a popular and well-maintained package for handling file uploads in Node.js.
In your `pages/api/upload` file, import the `multer` middleware and create a new instance of it. This will allow you to configure the upload settings, such as the upload directory and file type.
A fresh viewpoint: How to Upload File to Google Cloud Storage Using Reactjs
Setting Up
To set up your Next.js project for file uploads, you'll need to add the "use client;" directive at the top of your file, as the UploadButton component requires client-side execution.
This ensures that the UploadButton component functions as expected, which is crucial for a seamless file upload experience.
You'll also need to configure your API to handle file uploads, but that's a topic for the next section.
Readers also liked: Next Js Client Portal
Creating the Component
Creating the Component is a crucial step in building a Next.js file upload API. In Next.js, we'll create a React component to handle file uploads, which will be stored in a new file called index.tsx inside the src directory.
This file will contain the HTML and logic for the file upload component. Inside this file, we'll create the upload component that will be used throughout our application.
A different take: Nextjs Ui Library
Thing
To create the API endpoint for file uploads, we need to install the formidable library and run it.
You'll want to install formidable by running npm install formidable, or yarn add formidable if you're using yarn.
To handle multipart form data, we'll create a new API route in pages/api/fileUploadAPI.tsx. This route should import the necessary modules, including { bodyParser: false } from api. This tells Next.js not to use its default body parser, because formidable will handle parsing the incoming request data.
We can also use a plugin like UploadThing SSR to handle file uploads. This plugin can be imported in our layout file and configured to hide the loading state of the button, making it ready to take in file uploads faster.
Here are some key things to keep in mind when creating the API endpoint:
- Install formidable by running npm install formidable or yarn add formidable
- Create a new API route in pages/api/fileUploadAPI.tsx
- Import { bodyParser: false } from api to handle multipart form data
Creating the Component
Creating the Component is a crucial step in building your project.
To create a React component for file uploads, you'll need to create a new file inside the src directory.
This file will contain the HTML and logic for the file upload component, just like we're doing in this project.
The file will be called index.tsx, which is a standard naming convention for React components.
In this file, you'll write the code that will handle the file upload functionality, making it a key part of your project.
For example, we're creating a file upload component, which will allow users to select and upload files from their computer.
The component will be created inside the src directory, where all the project's source code lives.
This is where the magic happens, and your project starts to take shape.
Readers also liked: Nextjs Pathname Server Component
Handling File Upload
The file upload function in Next.js involves a bit of complexity, but it's manageable with the right approach.
To start, you'll need to use the s3.upload() method, which requires a filename, the name of your bucket, and the file itself from your form input.
The s3.upload() method returns an upload object that contains useful methods for monitoring the progress of your upload.
You can add an event handler to track the progress of the upload, which can be used to create a progress bar.
The upload object also provides a promise that you can await to track when the upload is finished.
It's a good idea to wrap the whole block in a try block to catch any potential upload errors.
To handle file uploads, you'll need to modify your index.tsx file to manage file data and send it to the Next.js API to save the files in a specified folder.
In this case, the folder is named "upload" and is located inside the public folder.
The updated code will allow you to manage file data and send it to the API for saving.
Discover more: Next Js Components Folder
Creating the Endpoint
To create the API endpoint for handling file uploads, you'll need to install the formidable library. This library will manage multipart form data, which is crucial when dealing with file uploads.
You can install formidable by running a command in your terminal. The library is necessary for parsing incoming request data, which is typically sent as multipart/form-data.
When creating the API route, you'll need to import the necessary modules. This includes the api object with bodyParser set to false. This tells Next.js not to use its default body parser, allowing formidable to handle the parsing.
Here's a key configuration to note: api: { bodyParser: false }. This is essential for file uploads, which are sent as multipart/form-data.
On a similar theme: Next Js Icons
Code and Implementation
To implement file uploads in a Next.js application, you can use the UploadButton component from UploadThing.
The UploadButton component connects to a specific endpoint, in this case imageUploader, and handles both successful uploads and errors with customizable callbacks.
It runs client-side, providing immediate feedback through alerts and console logs for completion or errors.
To display a preview of the uploaded image, you store the URL returned after a successful upload in the state variable imageUrl.
This URL is then used to display the uploaded image with the Image component.
Recommended read: Image as Background Nextjs
Error Handling and Routes
Error handling is crucial when it comes to file uploads, and we can achieve this by wrapping our server action calls in a try/catch block, as seen in the handleFileUpload function.
This approach also provides a place for validation and post-processing, ensuring that our file uploads are reliable and secure.
In Next.js, we can use API route handlers with the same logic as server actions, making it easy to handle errors and validate data in a consistent way.
Broaden your view: Next Js Server Side Api Call
Error Handling
Error handling is a crucial aspect of building robust and user-friendly applications. To handle file uploads, we can wrap the upload function in a try-catch block, as shown in Example 1.
This allows us to catch and handle any errors that may occur during the upload process. We can also use this block to add validation and post-processing to the uploaded file.
In Example 3, we see how to move the form to a client component to support validation and error handling. The handleFileUpload function wraps the calls to the server action in a try-catch block, providing a place for validation and post-processing.
The use of try-catch blocks is a simple yet effective way to handle errors in our application. By catching and handling errors, we can provide a better user experience and prevent crashes.
To handle errors in API routes, we can use the formidable library to parse the incoming request, as shown in Example 6. This allows us to get the files from the request and handle any errors that may occur during the upload process.
If an error occurs during the file upload or saving the file info to the database, we can set the status to 500 and return an error message, as shown in Example 6.
Here's a summary of the error handling techniques we've discussed:
- Wrap the upload function in a try-catch block to catch and handle errors.
- Use a try-catch block to add validation and post-processing to the uploaded file.
- Use the formidable library to parse the incoming request and handle errors in API routes.
- Set the status to 500 and return an error message if an error occurs during the file upload or saving the file info to the database.
Testing the Implementation
Testing the implementation of error handling and routes in your Next.js application is crucial. You can test your implementation by navigating to http://localhost:3000 in your browser.
To test the implementation of file uploads, you should see a success message and the files should be saved in the public/uploads directory. This is because the implementation is set up correctly.
To test the file upload implementation, use the file input to select multiple files and click the “Upload” button. This will trigger the file upload process.
If you're testing the file upload implementation, make sure to check that the files are saved in the correct directory. In this case, the files should be saved in the public/uploads directory.
Here's a step-by-step guide to testing the file upload implementation:
- Open your browser and navigate to http://localhost:3000.
- Use the file input to select multiple files and click the “Upload” button.
By following these steps, you can ensure that your file upload implementation is working correctly.
Frequently Asked Questions
How to send files to API in NextJS?
To send files to the API in NextJS, make a POST request to the /api/upload endpoint with the file included in the request payload as a form data field named "file". The API will handle the file processing and save it to the public/assets/ directory.
Can you upload a file in an API?
Yes, you can upload a file to an API, and there are three ways to do it: as the only content, as part of a multipart/form-data payload, or as part of a multipart/mixed payload. Learn more about the different methods and requirements for file uploads in our API documentation.
Sources
- https://upmostly.com/next-js/how-to-upload-a-file-to-s3-with-next-js
- https://currentior.com/implementing-multiple-file-uploads-in-next-js-a-step-by-step-guide/
- https://createappai.com/blog/file-upload
- https://blog.alexefimenko.com/posts/file-storage-nextjs-postgres-s3
- https://kodaschool.com/blog/next-js-file-uploads-made-easy-with-uploadthing-an-alternate-to-s3-bucket
Featured Images: pexels.com