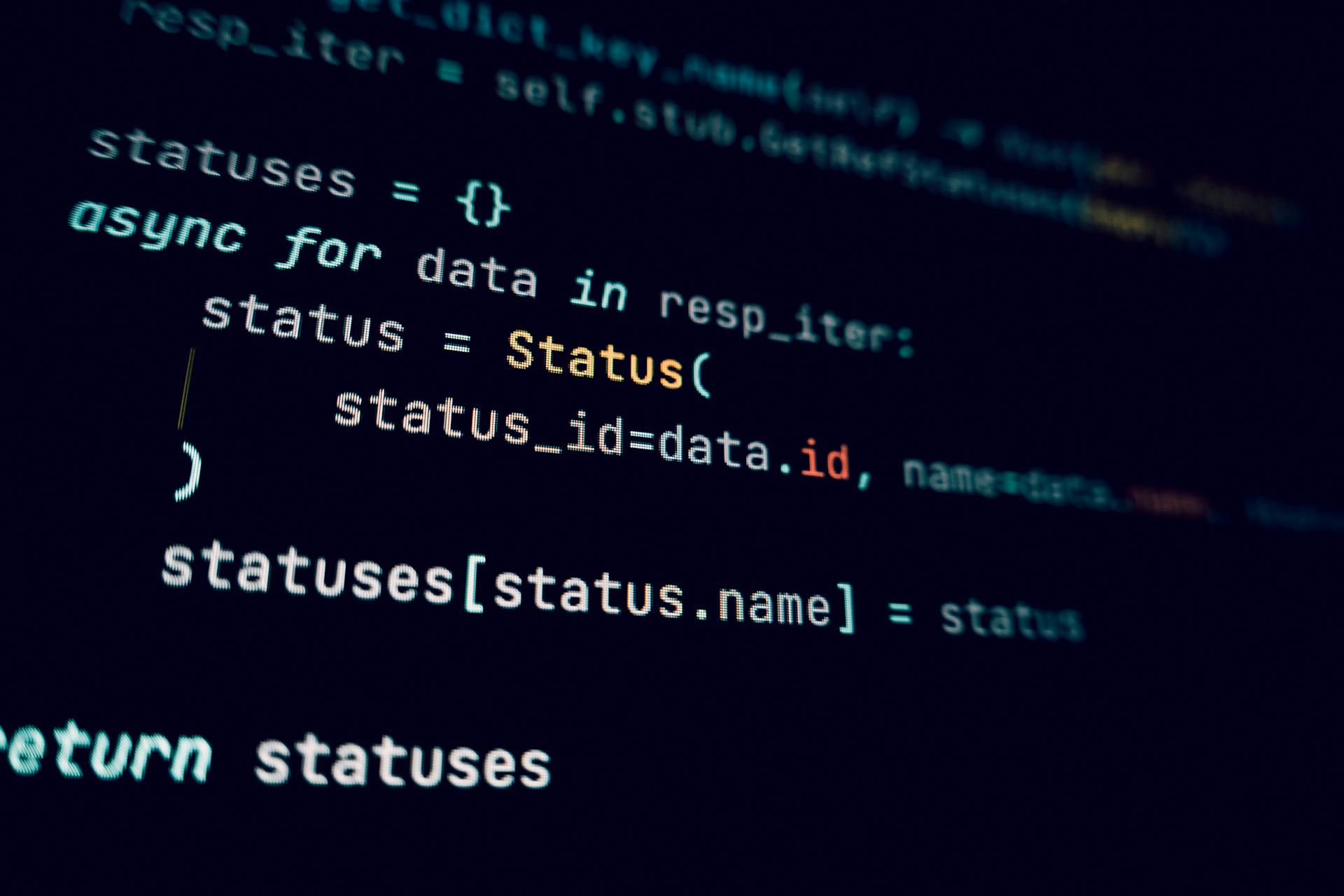
To call an API on the server in Next.js, you need to use the `fetch` API or a library like Axios.
You can create a new API route by creating a file in the `pages/api` directory. For example, you can create a file called `hello.js` with the following code: `export async function handler(req, res) { res.status(200).json({ message: 'Hello World' }); }`.
This code defines a simple API route that responds with a JSON object when accessed. The `handler` function is called when the API route is accessed, and it returns a response with a 200 status code and the JSON object.
Intriguing read: Next Js Fetch Data save in Context and Next Route
Server Actions
Server Actions are a powerful feature in Next.js that allow you to execute server-side code in response to user interactions. They've been a part of the framework since version 13, but became stable and incorporated by default in version 14.
Server Actions are perfect for tasks like fetching data from external APIs, performing business logic, and updating your database. You can use them for a variety of use cases, such as validating user input or processing payments.
Here are some common use cases for Server Actions:
- Feching data from external APIs
- Performing business logic
- Updating your database
These use cases are well-suited for Server Actions because they can be executed on the server without compromising performance or security.
For another approach, see: Next Js Custom Server
Understanding Server Actions
Server Actions are a powerful feature in Next.js that allow you to perform server-side logic without compromising performance or security. They've been a part of the framework since version 13, but it wasn't until version 14 that they became a stable feature.
Server Actions are well-suited for use cases like fetching data from external APIs, performing business logic, and updating your database.
You can use Server Actions to fetch data from external APIs without worrying about performance or security issues. This is especially useful when you need to retrieve data from third-party services.
Server Actions can also perform business logic that requires server execution, such as validating user input or processing payments.
Here are some common use cases for Server Actions:
- Fetching data from external APIs
- Performing business logic
- Updating your database
Server Actions are asynchronous JavaScript functions that run on the server in response to user interactions on the client. This means that when a user interacts with your application, Next.js will serialize the request parameters and send them to the server for execution.
Related reading: Nextjs Pathname Server Component
The server will then deserialize the request parameters, execute the Server Action, and serialize the response before sending it back to Next.js. From there, Next.js will deserialize the response and send it back to the front end.
To invoke a Server Action, you can use one of several methods, including the action prop, useFormState hook, or startTransition hook.
Video Tutorials
Learning about server actions can be a complex topic, but breaking it down into smaller chunks makes it more manageable.
One way to get started is by exploring video tutorials that cover the basics of server actions. Next.js API Routes Crash Course is a beginner-friendly video tutorial that covers the fundamentals of API routes in Next.js.
Building a REST API with Next.js API Routes is a step-by-step guide that helps you create a RESTful API using Next.js API routes. This is a great resource to follow along with as you learn about server actions.
Additional reading: Nextjs Video
Error handling and middleware functions are also crucial aspects of server actions. Next.js API Routes: Error Handling and Middleware is a resource that teaches you how to implement error handling and middleware functions in your API routes.
Here are some video tutorials to get you started:
Server-Side Calls
Next.js offers several methods to make API calls on the server side, improving performance and SEO by pre-fetching data before rendering the page.
You can use getServerSideProps to fetch data each time a user requests the page, making it perfect for applications that require the client to see the most up-to-date information.
getServerSideProps is executed on the server, making it ideal for dynamic pages that require up-to-date data.
This method is also great for performing secure operations or accessing databases without exposing sensitive data to the client.
Here are some benefits of using getServerSideProps:
- The data is refreshed each time a client loads the page, making it up to date as of when they visit the page.
- The data is rendered before it reaches the client, which is great for SEO.
Additionally, you can call internal API routes from both the client and the server side, making it easy to fetch data from internal API routes.
To optimize API calls in Next.js, consider using caching strategies such as HTTP headers or ISR to improve performance and reduce server load.
Sending Data
You can send data to an external API using Server Actions, which will trigger from a form submission.
Create a new Server Action, addComment, that expects one single parameter of the type FormData.
This new function will send data to a third-party API.
You can invoke this function when the user submits a form, but you'll notice that it looks unresponsive.
This is because there's no "loading" or "saving" activity shown to the user.
A unique perspective: Next Js Function Handlers to Filter Data
Handling Requests
Handling requests in Next.js API routes is a crucial aspect of building reliable and scalable applications. You can handle different request methods, such as GET and POST, using API routes.
To handle POST requests, you need to check the req.method property and respond accordingly. Here's an example: the handler function uses req.json() to parse the JSON data from the request body.
Error handling is a must in Next.js API routes. It ensures that your API endpoints can handle unexpected errors, provide clear error responses, and maintain a good user experience.
Recommended read: Can I Store Json in Nextjs
You can handle GET and POST requests using API routes. Here are some key points to keep in mind:
- Dynamic API routes enable you to create flexible routes that can handle different scenarios.
- By following these key takeaways, you'll be able to build fast, scalable, and secure API routes with Next.js.
API routes in Next.js allow you to create serverless API endpoints, making it easier to write business logic. You can handle different request methods, such as GET and POST, using API routes.
Optimizing Calls
You can significantly improve the speed and efficiency of your Next.js API routes by implementing performance optimization strategies.
Caching is a great way to reduce server load and improve performance. Next.js provides built-in support for caching API routes using the cache-control header.
For example, you can add the following lines to your now.json file to cache API routes for one hour.
Error handling is also crucial when making API calls. Always handle errors gracefully in your API calls using try/catch blocks or Promise.catch to manage issues with requests.
Authentication is another important aspect of API calls. Secure your API routes using authentication strategies such as JSON Web Tokens (JWT) or sessions.
Implementing rate limiting in your API routes can prevent abuse or performance degradation. This is also known as throttling.
Here are some tips to keep in mind when optimizing your API calls:
- Caching can improve performance by reducing the number of requests to your API.
- Error handling is essential to manage issues with requests.
- Authentication secures your API routes.
- Throttling prevents abuse or performance degradation.
Error Handling
Error handling is a crucial aspect of building reliable Next.js API routes. It ensures that your API endpoints can handle unexpected errors, provide clear error responses, and maintain a good user experience.
When building Next.js API routes, you should always anticipate that something can go wrong. Error handling is a crucial aspect of building reliable Next.js API routes.
To handle errors effectively, you need to use error handling mechanisms such as try-catch blocks. This will ensure that your API endpoints can handle unexpected errors.
Using try-catch blocks will also allow you to provide clear error responses to your users. This is essential for maintaining a good user experience.
Clear error responses will help your users understand what went wrong and how to fix it. This will reduce frustration and improve the overall user experience.
By incorporating error handling into your Next.js API routes, you can build more reliable and user-friendly applications.
HTTP Requests
In Next.js, you can handle different types of HTTP requests, such as GET and POST requests. Dynamic API routes enable you to create flexible routes that can handle different scenarios.
To handle POST requests, you need to access and process data from the request body. This is done by writing POST handlers.
You can build fast, scalable, and secure API routes with Next.js by following key takeaways. Here's a brief summary:
Remember to experiment and learn more about the various features and techniques available in Next.js API routes.
Setting Up
To set up API routes in Next.js, you need to navigate to the pages/api directory in your project. If it doesn't exist, create it.
Create a new file in the pages/api directory with a name that matches the desired API route, like users.js for a route handling user data.
In this file, export a default function that will handle the route, taking two parameters: req and res. This function will send a JSON response with a status code of 200 and a message.
Expand your knowledge: Cant Call Route after :id Next Js
Creating
To create API routes in Next.js, you'll need to define them in the pages/api directory. Each file in this directory corresponds to a specific route.
API routes are useful for building microservices or handling server-side logic in your Next.js application. They allow you to handle various HTTP methods, such as GET, POST, and perform authentication or database operations.
To create an API route, you can start by creating a new file in the pages/api directory. This file will correspond to a specific route, and you can define the logic for that route within it.
See what others are reading: Can Amazon S3 Take in Nextjs File
Accessing
Accessing the API route is straightforward. You can visit http://localhost:3000/api/[filename] in your browser to access the API route.
To access the API route for the users.js example, visit http://localhost:3000/api/users. Next.js automatically parses the request body and query parameters, making it easier to work with incoming data.
Return
API routes in Next.js allow you to create serverless API endpoints, making it easier to write business logic.
You can handle different request methods, such as GET and POST, using API routes. This makes it easy to handle various types of requests and responses.
API routes can be used to create endpoints that return data to the client, allowing you to build robust and scalable server-side APIs.
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/server-actions-and-mutations
- https://auth0.com/blog/using-nextjs-server-actions-to-call-external-apis/
- https://wallis.dev/blog/nextjs-serverside-data-fetching
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
- https://medium.com/@turingvang/nextjs-api-call-b4a8ac63bce9
Featured Images: pexels.com