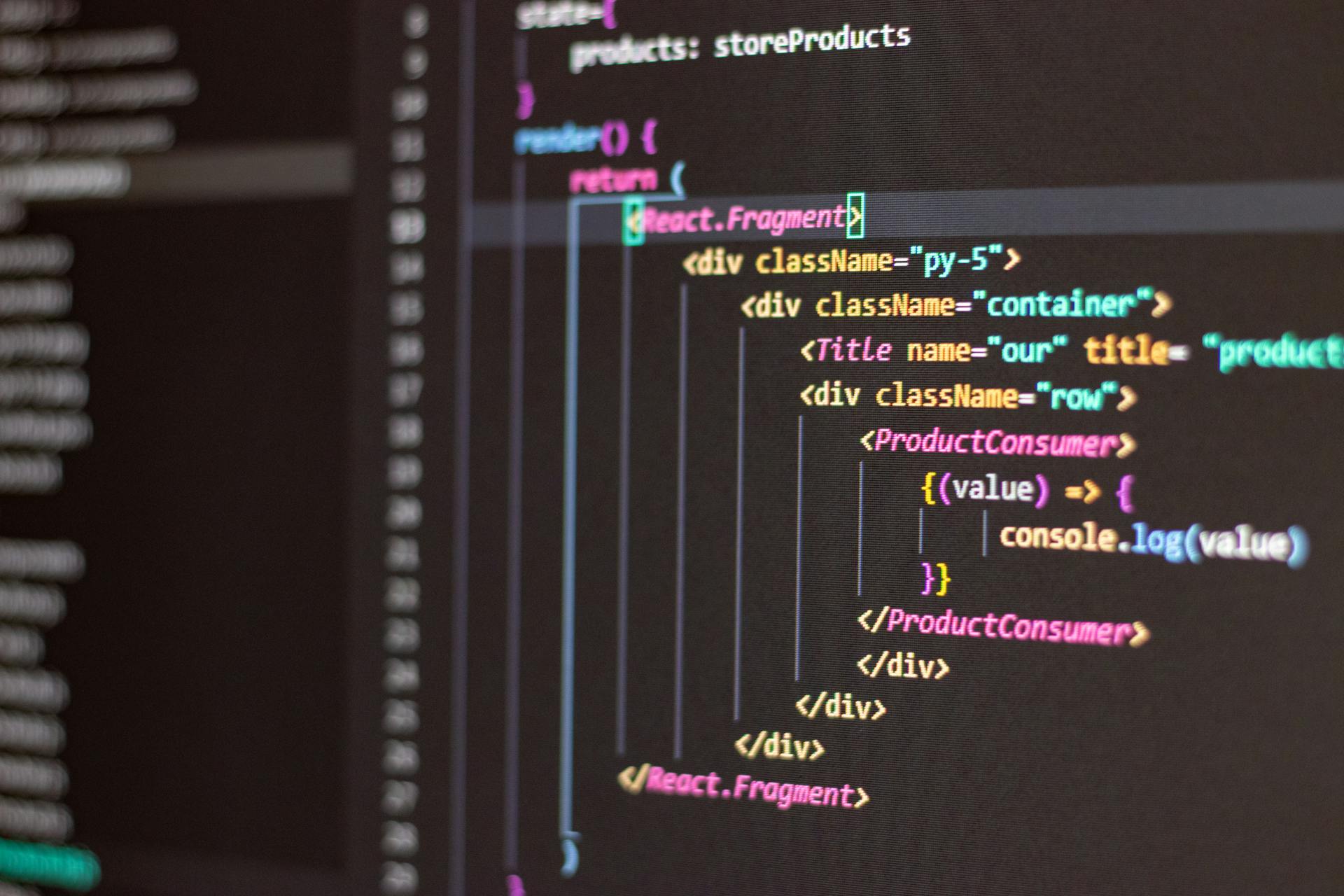
In Next.js, calling a route after an ID can be tricky, especially when it comes to Server-Side Rendering (SSR). The issue arises because Next.js uses the `getStaticProps` method to pre-render pages at build time.
This method doesn't work well with dynamic routes that include an ID, as it can't resolve the route until runtime. As a result, you can't call a route after an ID in Next.js, at least not in a way that works with SSR.
The solution is to use a different approach, such as using client-side rendering or a library like `next/router` to handle routing on the client-side.
What is the Issue?
In Next.js, the `getStaticPaths` function is used to pre-render pages at build time, but it has a limitation when it comes to dynamic routes. Specifically, it can't call `getStaticProps` after `:id` because it's a dynamic route.
This limitation is because `getStaticPaths` is used to generate static HTML files for pages, and dynamic routes like `:id` can't be determined until runtime. As a result, Next.js can't pre-render pages with dynamic routes like `:id` at build time.
Check this out: Next Js Getstaticpaths
Route not defined in getStaticPaths
The "Route not defined in getStaticPaths" error can be frustrating to encounter, especially when trying to optimize your Next.js application for static site generation.
This error occurs when the `getStaticPaths` function is called without defining the necessary routes for the application.
The `getStaticPaths` function is used to pre-render pages at build time, but it requires the `paths` property to be defined, which specifies the routes that need to be pre-rendered.
Without defining these routes, the `getStaticPaths` function will throw an error, preventing the application from being pre-rendered correctly.
This can cause issues with page loading, as the application will not be able to render the necessary pages at build time.
Curious to learn more? Check out: Nested Routes in Next Js
Route defined but not in getStaticPaths
You've defined a route, but it's not showing up in your `getStaticPaths` function. This can be frustrating, especially if you've double-checked your code.
The issue here is likely that your route is not being statically generated, which means it's not being rendered at build time. This can happen if your route is dynamically generated, such as when using parameters or a database query.
Expand your knowledge: Nextjs Router Not Mounted
Make sure you're using `getStaticPaths` correctly, as shown in the example where it's used to generate static paths for a route with parameters. The `paths` array should be populated with the possible values of the parameters.
For example, if your route is `/posts/:slug`, your `getStaticPaths` function might return an array like this: `[ { params: { slug: 'hello-world' } }, { params: { slug: 'another-post' } } ]`. This tells Next.js to generate static HTML for each of these paths.
For more insights, see: Nextjs Slug
Dynamic Routing
Dynamic Routing is a crucial aspect of modern networking. It allows for the efficient allocation of network resources, reducing congestion and increasing overall performance.
In a typical network, packets of data are routed through a series of switches and routers, each with its own rules and priorities. This can lead to bottlenecks and inefficiencies, particularly in large-scale networks.
The Internet Engineering Task Force (IETF) has developed the Multiprotocol Label Switching (MPLS) protocol to address these issues. MPLS enables routers to make more informed decisions about packet routing, reducing latency and increasing throughput.
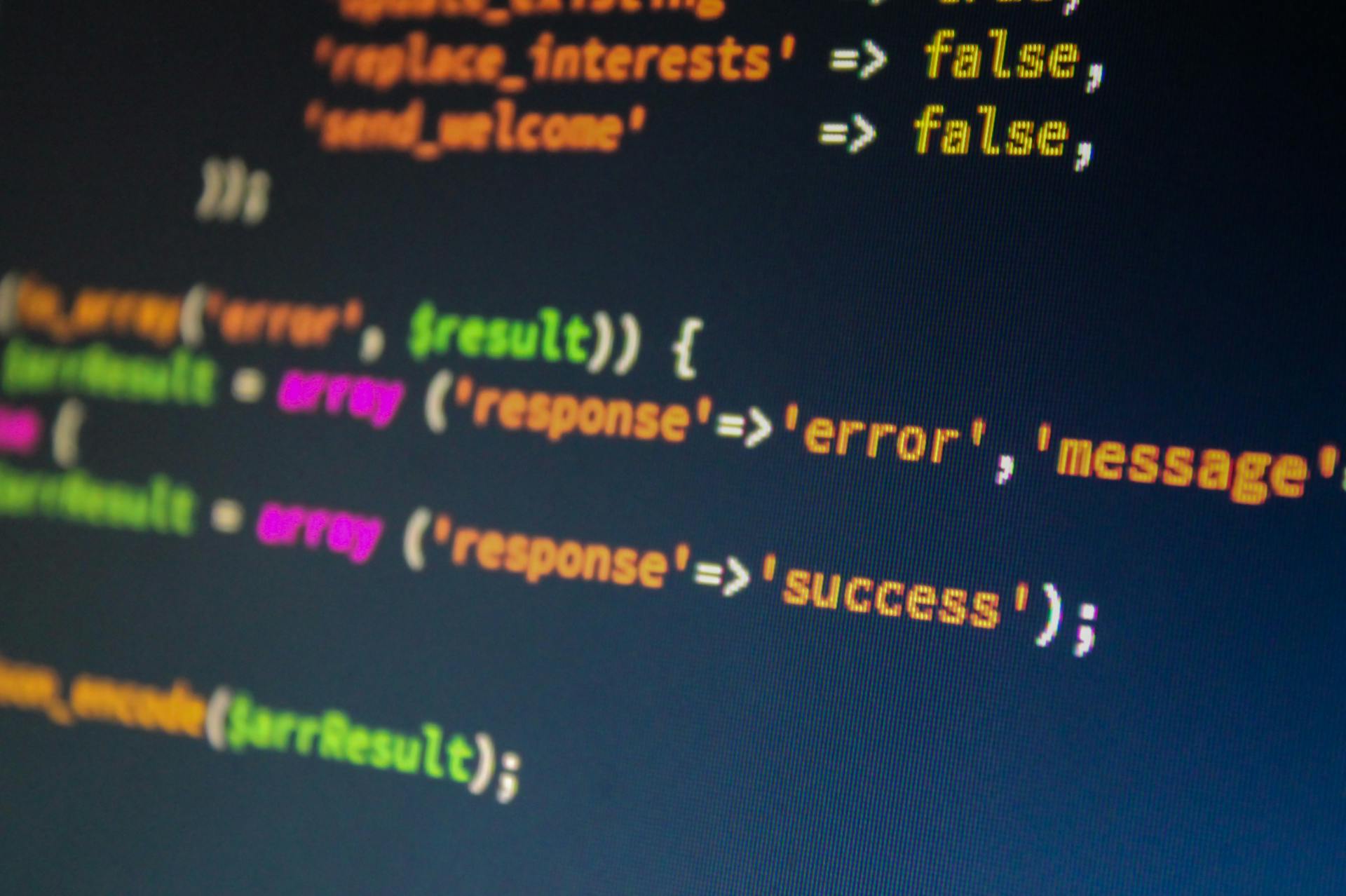
In practice, dynamic routing can be implemented using various techniques, such as link-state routing protocols and distance-vector routing protocols. These protocols allow routers to adapt to changing network conditions, ensuring optimal packet routing.
The benefits of dynamic routing are numerous, including improved network reliability, increased scalability, and enhanced security. By dynamically routing packets, networks can respond more effectively to changing conditions, reducing the risk of congestion and downtime.
Intriguing read: Next Js Dynamic
Server-Side Rendering
Server-Side Rendering is a technique that allows websites to generate HTML on the server, rather than the client's web browser. This approach can improve SEO by making it easier for search engines to crawl and index websites.
One of the key benefits of Server-Side Rendering is that it can improve page load times, as the server can generate the HTML in advance, reducing the amount of data that needs to be transferred to the client. This can lead to faster page loads and a better user experience.
Consider reading: Next Js App Router Page Transition Loader
In fact, studies have shown that Server-Side Rendering can improve page load times by up to 50% compared to traditional client-side rendering. This is because the server can generate the HTML in parallel with the database queries, reducing the overall latency of the page load.
Server-Side Rendering can also improve the accessibility of websites, as it can generate alternative text for images and other content that may not be accessible to users with disabilities. This is especially important for websites that need to comply with accessibility regulations, such as the Web Content Accessibility Guidelines (WCAG).
For your interest: Next Js 14 Redirect to Another Page Loading Indicator
Using getServerSideProps
Using getServerSideProps is a way to pre-render pages on the server. This helps to improve the performance of your Next.js application by reducing the number of requests made to the client.
getServerSideProps can be used to fetch data on the server and pass it to the page component as props. This allows you to handle data fetching and rendering on the server-side.
By using getServerSideProps, you can avoid making unnecessary API calls on the client-side, which can improve the user experience.
Additional reading: Nextjs Getserversideprops
Workarounds and Solutions
If you're encountering the "can't call route after :id" issue in Next.js, don't worry, there are workarounds and solutions to help you navigate this challenge.
One possible solution is to use a different route parameter, such as a query parameter, to pass the ID to the server. This can be achieved by modifying the API route to accept a query parameter instead of a path parameter.
You can also try using a dynamic page component to handle the route with the :id parameter. This approach allows you to define a separate page component for each ID, making it easier to manage and render the content.
Another approach is to use a catch-all route to handle the :id parameter, and then use a server-side function to determine which route to render based on the ID. This can be a more complex solution, but it provides a high degree of flexibility and control.
For another approach, see: Router Query Params Nextjs 14
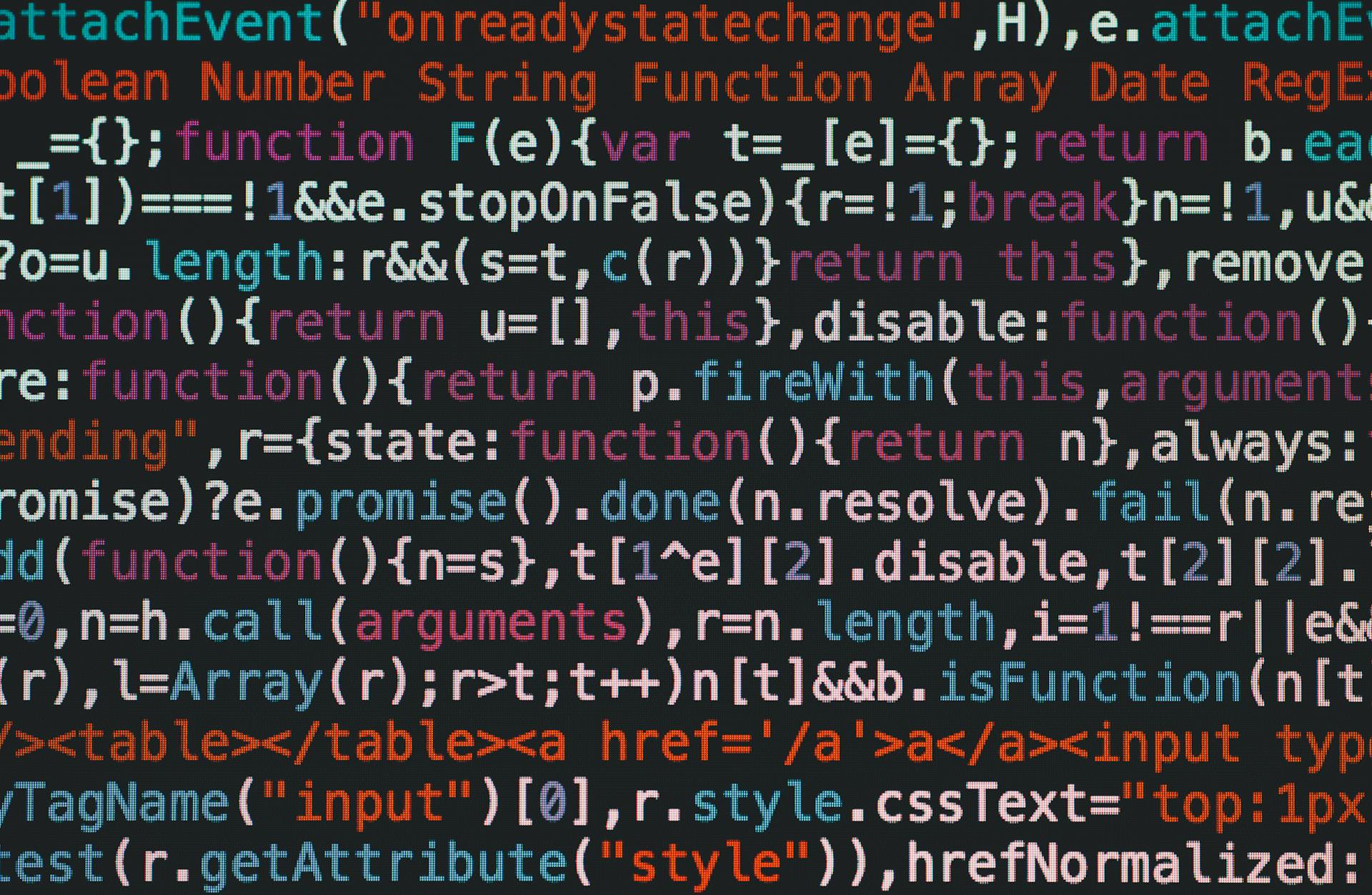
In some cases, the issue may be caused by a conflict between the client-side and server-side routing. To resolve this, you can try using a separate API route for the server-side rendering, and then use a client-side fetch or axios call to retrieve the data.
By implementing these workarounds and solutions, you should be able to overcome the "can't call route after :id" issue in Next.js and build a robust and scalable application.
Take a look at this: Next Js Server Side Api Call
Sources
Featured Images: pexels.com