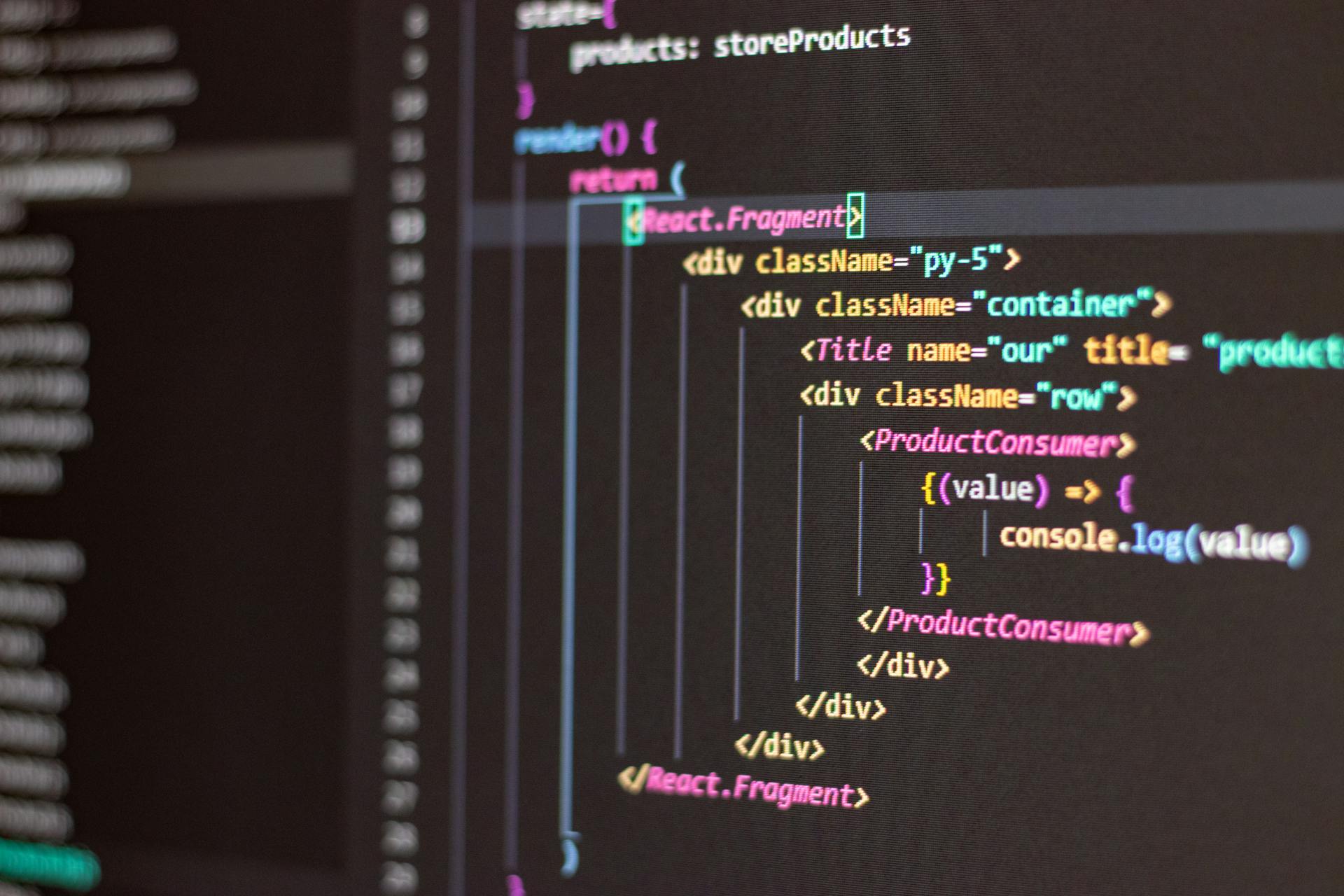
Server-side rendering (SSR) is a feature that allows Next.js to render pages on the server, improving performance and SEO. This is achieved through the use of getServerSideProps.
getServerSideProps is a function that returns an object with props, which is then passed to the page component. This function is called on the server, allowing Next.js to fetch data and render the page before sending it to the client.
This approach enables Next.js to handle dynamic data fetching, making it easier to build data-driven applications.
On a similar theme: Nextjs App Route Get Ssr Data
Understanding GetServerSideProps
GetServerSideProps is a powerful method in Next.js that allows you to fetch data from the server during a request.
It's used to retrieve data from the server, which is then passed as props to your page component. To define this function, you need to export it from your page component file.
This function runs on the server and allows you to fetch data, which is then passed as props to your page component. The structure of getServerSideProps is different from getInitialProps, and it returns an object with the props key containing the fetched data.
Intriguing read: Next Js Props
With getServerSideProps, the fetched data will be automatically passed as props to the page component. You can access this data using the props parameter of your page component.
Here's a quick rundown of the steps involved in migrating from getInitialProps to getServerSideProps:
- Remove getInitialProps: If your page components use getInitialProps, you need to remove it from those components
- Replace getInitialProps with getServerSideProps: Replace the getInitialProps method with the getServerSideProps method in your page components
- Pass props to the component: With getServerSideProps, the fetched data will be automatically passed as props to the page component
By using getServerSideProps, you can deliver the page with the necessary data pre-loaded, which ensures fast loading times and a better user experience.
From GetInitialProps to GetServerSideProps
Getting rid of getInitialProps is a great first step in migrating to getServerSideProps. You'll need to remove it from your page components.
Replacing getInitialProps with getServerSideProps is the next step. This involves changing the structure of your data fetching logic to return an object with the props key containing the fetched data. This is a key difference between getInitialProps and getServerSideProps.
With getServerSideProps, the fetched data will be automatically passed as props to the page component. You can access this data using the props parameter of your page component.
Explore further: Next Js Fetch Data save in Context and Next Route
Here's a simple example of how to migrate from getInitialProps to getServerSideProps:
- Remove getInitialProps from your page components
- Replace getInitialProps with getServerSideProps
- Pass props to the component
Here's a step-by-step guide to migrating to getServerSideProps:
1. Remove getInitialProps from your page components
2. Replace getInitialProps with getServerSideProps
3. Pass props to the component
By following these steps, you can migrate from getInitialProps to getServerSideProps and take advantage of improved performance and predictability in your Next.js application.
You might enjoy: Getinitialprops Next Js
GetServerSideProps Basics
To use GetServerSideProps, you need to export it as a function in your page component. This function should return an object with the data you want to pass as props to your component.
The GetServerSideProps function fetches data from an API and returns it as props to the component. You can access the context object to tailor your data fetching to the specifics of the request.
The context object provides information about the incoming request and includes properties such as params, req, res, query, preview, previewData, locale, and defaultLocale.
Here are the key properties of the context object:
- params: Contains the route parameters for dynamic routes.
- req: The HTTP request object.
- res: The HTTP response object.
- query: The query string parameters.
- preview: Preview mode status.
- previewData: Data set in preview mode.
- locale: The active locale configured for internationalized routing.
- defaultLocale: The default locale configured for internationalized routing.
You can use these properties to dynamically fetch data based on the user’s request.
Data Fetching
Data Fetching is a crucial aspect of Next.js, and getServerSideProps is the key to unlocking it. This feature allows you to fetch data on the server, ensuring that your page is pre-rendered with the necessary data before it's sent to the client.
You can use various data fetching methods to retrieve data from internal or external sources. For instance, you can fetch data from your own APIs or databases, or call external APIs or services to retrieve data. This flexibility allows you to integrate various data sources into your application seamlessly.
Internal resources can be fetched within the getServerSideProps function, while external resources can be called to retrieve data. This approach ensures that the data is ready on the server side and minimizes client-side data fetching.
Here are the different types of resources you can fetch data from:
- Internal Resources: Fetch data from your own APIs or databases.
- External Resources: Call external APIs or services to retrieve data.
By leveraging both internal and external resources, you can build robust applications that gather data from multiple sources, ensuring that your page components always have the latest data available. This is particularly useful for dynamic data that needs to be up-to-date when the page loads.
Advanced Features and Best Practices
The getServerSideProps function is an async function that takes a context object as an input parameter. This object contains information about the current request.
To optimize performance, consider the trade-off between slower initial page loads and the need for dynamic content generation. This approach may be suitable for pages that require data fetching on every request.
In terms of execution flow, the server fetches the data at the requested time and passes it to the page component, allowing for dynamic content generation. This flow is crucial for dynamic pages that rely on external data.
Here are some key aspects to keep in mind when using getServerSideProps:
Advanced Features
You can use getServerSideProps to fetch data from the server and provide it as props to your page component, ensuring quick load times and enhanced SEO.
getServerSideProps is an async function that receives a context object containing information about the current request. It fetches data based on the dynamic parameter (slug) from an external API or database, and passes the fetched data to the page component as props.
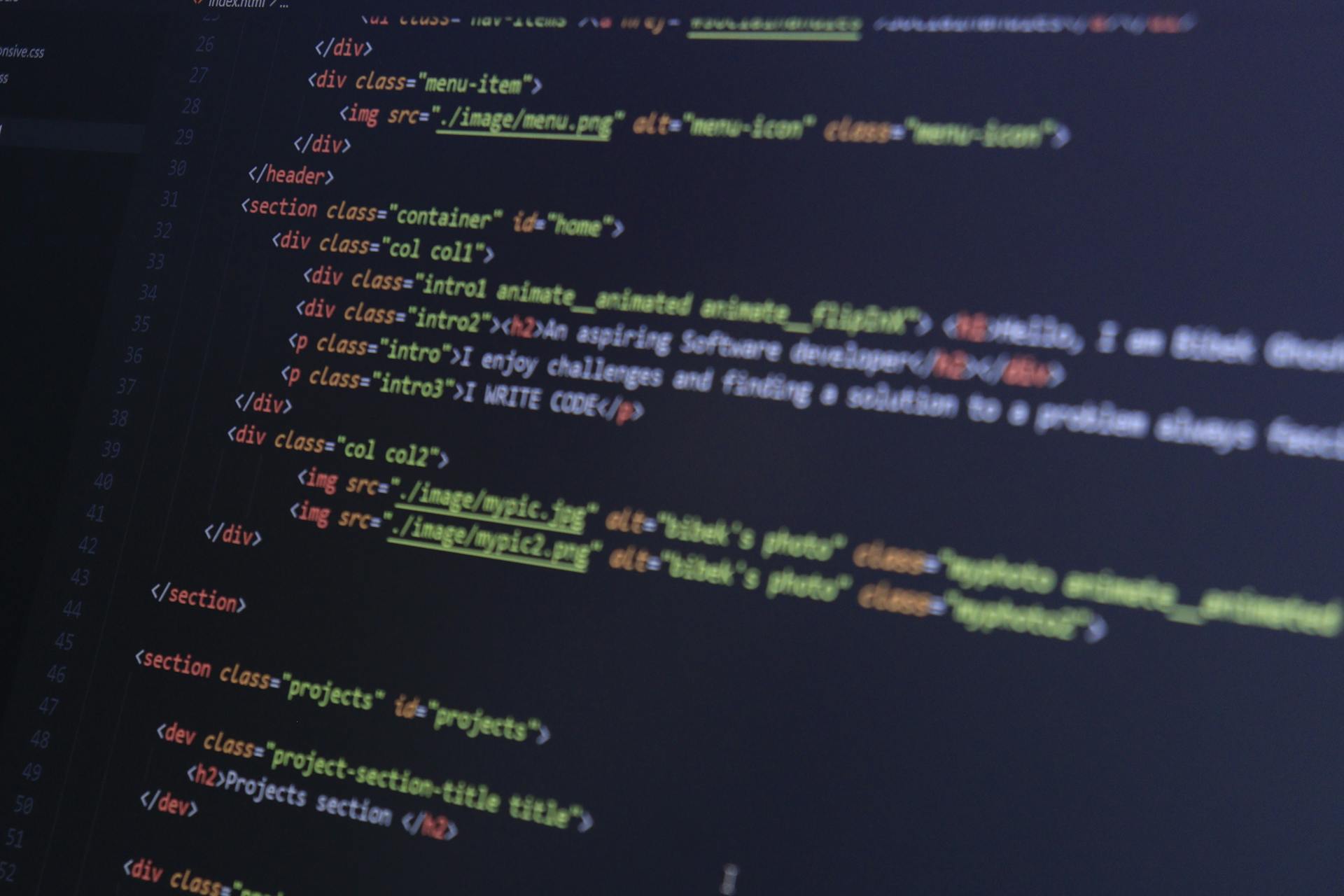
To optimize getServerSideProps for performance, make sure to return the required props object with the props property, which can help reduce the number of repetitive calls. This is because Next.js creates a server-side request to fetch the data, which can be quite expensive.
You can also take advantage of smarter caching, which works by caching the results of your request based on the content that was requested. This means that if a user requests a specific page, Next.js will only cache the data for that page.
Here's a simple table that breaks down the key aspects of the getServerSideProps function in Next.js dynamic content generation:
Migrating from getInitialProps to getServerSideProps or getStaticProps involves updating your data fetching logic to the new data fetching methods introduced in Next.js.
Redirection and Error Handling
Redirection and Error Handling is a crucial aspect of building robust and user-friendly applications with Next.js. Next.js allows you to redirect users to different pages from within getServerSideProps using the redirect object.
This is useful for handling situations where a page needs to redirect to another URL based on certain conditions. The redirect object specifies the destination and whether the redirect is permanent.
You can also handle cases where the data is not found by setting the notFound property to true in getServerSideProps. This will cause Next.js to display the built-in 404 page.
Redirecting users to a /not-found page is another way to handle situations where the data is not found. The destination is specified in the redirect object.
In some cases, you may want to render a custom 404 page instead of the built-in one. To do this, you can use the notfound boolean in getServerSideProps and set it to true when the data is not found.
NextJS Features and Benefits
NextJS offers improved performance and SEO through server-side rendering, which delivers fully rendered HTML to the client, reducing load times and enhancing the user experience.
Server-side rendering in NextJS is ideal for pages that require dynamic data, and it's perfect for handling complex data fetching methods, ensuring the latest data is available when the page is loaded.
The getServerSideProps function receives a context object containing information about the request, including dynamic route parameters and query string values, allowing for customized data fetching.
Here are some key aspects of the getServerSideProps function:
Server-side rendering in NextJS also supports internal and external resources, making it versatile for various use cases, such as querying a database or calling an external API.
NextJS combines the best of both worlds by providing server-side rendering for the initial load and client-side navigation for subsequent transitions, resulting in an enhanced user experience.
On a similar theme: Next Js Client Side Rendering
Implementation and Integration
To implement server-side rendering in Next.js, you need to create a getServerSideProps function in your page component. This function returns an object with a props property that contains the data fetched from the server.
The getServerSideProps function is called on the server, allowing you to fetch data and render the page dynamically. You can use this function to fetch data from APIs or databases.
For example, in the article section "Fetching Data with getServerSideProps", it's shown how to fetch data from a JSON file using the getServerSideProps function. This is done by returning a promise that resolves to the fetched data.
When you call getServerSideProps, Next.js will render the page with the fetched data. This approach is particularly useful for pages that require dynamic data, such as blog posts or product listings.
As seen in the example, you can also use the getServerSideProps function to pre-render pages at build time, reducing the need for client-side rendering. This can improve performance and SEO.
To integrate server-side rendering with your existing Next.js app, you'll need to update your page components to include the getServerSideProps function. This might require some refactoring, but the benefits are well worth the effort.
You might enjoy: Next Js Use Client
Example Using:
Using getServerSideProps in Next.js can be a game-changer for your application's performance and user experience. It allows you to fetch data on the server-side and pass it as props to your component, rendering it on the server before sending it to the client.
The MyPage component is a simple example of how to use getServerSideProps. It takes data as a prop and displays it. The getServerSideProps function is an asynchronous function that fetches data from an API.
Here are the key points to keep in mind when using getServerSideProps:
- The getServerSideProps function should return an object with a props key containing the data to be passed to the component.
- The MyPage component is then exported as the default export of the module.
- The getServerSideProps function is executed on the server-side, fetching the data and passing it as props to the MyPage component.
This approach provides a better user experience by rendering the page on the server-side before sending it to the client. It also allows you to handle errors gracefully by displaying an error message if the data-fetching process fails.
Frequently Asked Questions
Is get server-side props deprecated?
Yes, getServerSideProps is deprecated in Next.js, replaced by a newer approach to data fetching that leverages server components. Learn more about the updated data loading methods and how to migrate your code.
What are the differences between getStaticProps and getServerSideProps?
getStaticProps pre-renders pages on the server, while getServerSideProps runs on every page request, making them ideal for different use cases: static site generation for pre-rendered content and server-side rendering for dynamic data
Sources
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://blog.logrocket.com/data-fetching-next-js-getserversideprops-getstaticprops/
- https://www.dhiwise.com/post/mastering-nextjs-getserversideprops-a-comprehensive-guide
- https://blogs.purecode.ai/blogs/nextjs-getserversideprops/
- https://meje.dev/blog/nextjs-auth-with-cookies-and-getserversideprop
Featured Images: pexels.com