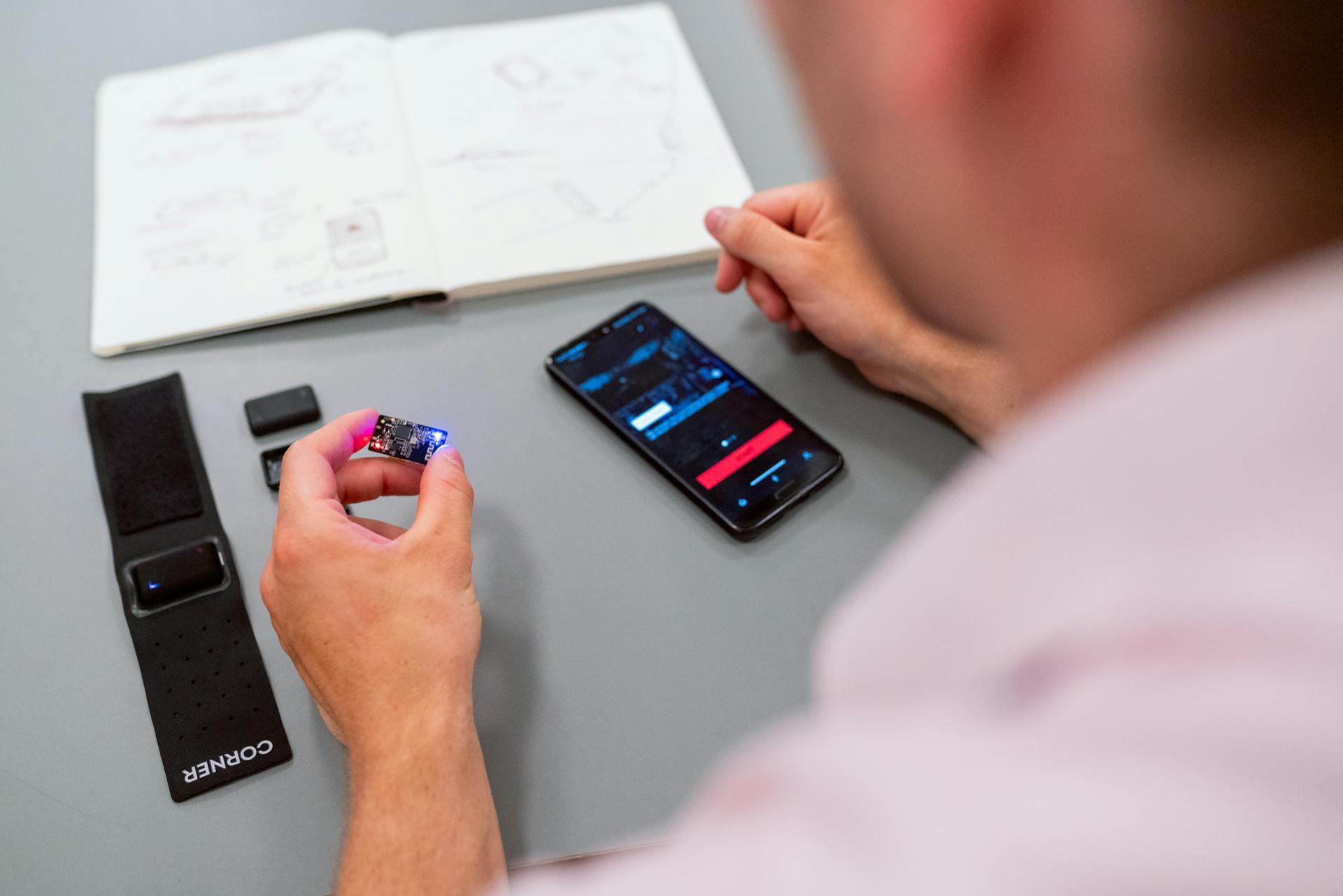
Using GetServerSideProps makes it easy to fetch data on the server for Next.js app routes. This approach ensures that data is only fetched when necessary, reducing unnecessary API calls.
GetServerSideProps is a function that allows you to pre-render pages at build time or on each request. It's a powerful tool for handling data fetching in Next.js.
By using GetServerSideProps, you can avoid unnecessary re-renders and improve page performance. This is especially important for pages that require data fetching, such as those with API calls or database queries.
In the context of Next.js app routes, GetServerSideProps provides a simple way to fetch data on the server, making it easier to manage data fetching and improve page performance.
Worth a look: Nextjs Intercepting Routes
What is SSR
Server-side rendering (SSR) is a technique where the server generates the HTML for a web page and sends it to the client.
This approach improves initial page load time and enables search engine optimization (SEO) since search engine crawlers can easily parse the fully rendered HTML.
SSR involves generating the HTML for a web page on the server and sending it to the client.
Next.js excels at server-side rendering, allowing you to pre-render pages dynamically or statically.
Here are some key benefits of using SSR:
- Improved initial page load time
- Enables search engine optimization (SEO)
Getting Started
To get started with Next.js app route get SSR data, you'll need to understand the basic setup.
You can create a new Next.js project by running `npx create-next-app my-app` in your terminal.
Next, navigate to the project directory and run `npm run dev` to start the development server.
The default Next.js project comes with a basic layout and a few pre-built pages.
For example, the `pages/index.js` file contains a simple React component that renders a "Hello World" message.
To enable Server-Side Rendering (SSR) for a page, you'll need to export a function from the page component.
For instance, in the `pages/index.js` file, you can export a function that returns a JSX element using the `getServerSideProps` method.
This method allows you to fetch data from an API or a database and pass it to the page component as props.
In the `getServerSideProps` method, you can use the `req` object to access the request object and the `res` object to access the response object.
Intriguing read: How to Run Nextjs to Build
For example, you can use the `req.query` property to access query parameters passed in the URL.
The `req.query` property returns an object with the query parameters as properties.
You can also use the `res.json` method to send a JSON response back to the client.
By following these steps, you'll be able to set up a basic Next.js project with SSR enabled and fetch data from an API or a database.
A fresh viewpoint: Nextjs App Router Query Params
Server-Side Rendering
Server-Side Rendering is a powerful feature in Next.js that allows you to pre-render pages on the server, improving initial page load time and enabling search engine optimization. This approach delivers fully rendered HTML to the client, reducing load times and improving the user experience.
Server-side rendering involves generating the HTML for a web page on the server and sending it to the client, which improves initial page load time and enables search engine optimization. Next.js excels at server-side rendering, allowing you to pre-render pages dynamically or statically.
Intriguing read: Next Js Client Side Rendering
You can choose between server-side rendering and client-side rendering based on your application's needs. Server-side rendering is ideal for pages that require up-to-date data, while client-side rendering provides interactivity and dynamic content updates.
Here are some benefits of server-side rendering in Next.js:
- Improved Performance and SEO
- Dynamic Data Handling
- Context Object for Data Fetching
- Seamless Integration with Page Components
- Support for Internal and External Resources
- Enhanced User Experience with Client-Side Navigation
SSR vs. CSR
Server-side rendering (SSR) and client-side rendering (CSR) are two approaches to rendering web pages. Server-side rendering involves generating the HTML for a web page on the server and sending it to the client, improving initial page load time and enabling search engine optimization (SEO).
This approach is particularly useful for search engine crawlers, which can easily parse the fully rendered HTML. Next.js excels at server-side rendering, allowing you to pre-render pages dynamically or statically.
Client-side rendering, on the other hand, relies on JavaScript to render web pages on the client's browser, providing interactivity and dynamic content updates but may result in slower initial page loads.
Next.js supports hybrid rendering, allowing you to select between server-side and client-side rendering based on the needs of your application.
Here are the key differences between SSR and CSR:
- SSR generates HTML on the server, while CSR relies on JavaScript on the client
- SSR improves initial page load time and enables SEO, while CSR provides interactivity and dynamic updates
- Next.js supports hybrid rendering, allowing you to choose between SSR and CSR
Server-Side Rendering Benefits
Improved Performance and SEO is a key benefit of Server-Side Rendering in Next.js, as it delivers fully rendered HTML to the client, reducing load times and improving the user experience.
By pre-rendering pages on the server, SSR can index your content more effectively, making it easier for search engines to crawl and understand your website.
Server-Side Rendering in Next.js is ideal for pages that need to display dynamic data, such as pages that fetch data from internal or external APIs.
With Server-Side Rendering, you can handle complex data fetching methods and ensure that the latest data is available when the page is loaded.
Here are some key advantages of Server-Side Rendering in Next.js:
- Improved Performance and SEO
- Dynamic Data Handling
- Context Object for Data Fetching
- Seamless Integration with Page Components
- Support for Internal and External Resources
- Enhanced User Experience with Client-Side Navigation
GetInitialProps
GetInitialProps is a method used in older versions of Next.js to fetch data on the server side before rendering a page. It was the primary data fetching method used in Next.js before newer data fetching methods like getServerSideProps and getStaticProps were introduced.
In older versions of Next.js, getInitialProps was the primary data fetching mechanism for both server-side rendering (SSR) and client-side rendering. It allowed developers to perform custom data fetching logic for each page and pass the fetched data as props to the page component.
Developers can use getInitialProps to fetch data on the server side before rendering a page, which helps with SEO, improves initial page load times, and ensures that the page has the necessary data before being served to the client.
To handle edge cases where getInitialProps might still be necessary, consider the following scenarios:
- Complex data fetching logic: If your data fetching logic is complex and can’t be easily achieved with getStaticProps or getServerSideProps, you might continue using getInitialProps.
- Custom SSR logic: If you need custom server-side rendering logic that can’t be achieved with the newer methods, you might choose to use getInitialProps.
- Legacy projects: If you’re working on a legacy project that was built using older versions of Next.js and heavily relies on getInitialProps, it might be more practical to continue using it rather than rewriting everything.
Optimizing and Handling
Optimizing getServerSideProps for performance in Next.js 13 can be achieved by returning the required props object with the props property, which reduces repetitive calls and memoizes the request, reusing the result for subsequent calls.
This can significantly improve performance by avoiding multiple requests to the server. I've seen this approach make a big difference in real-world applications.
Smarter caching in Next.js works by caching the results of your request based on the content that was requested, allowing for incremental updates and only updating the affected parts of the site.
Discover more: Next Js Props
Creating an API
Creating an API is a crucial step in building a Next.js application. To create an API route, you need to create a file inside the pages/api directory.
The file should be named based on the desired endpoint, for example, pages/api/users.js. Within this file, you can define your server action logic.
This allows you to control the timing of the server action invocation, as seen in the code snippet where startTransition wraps the invocation of the addTodo server action.
In the code above, the server action is executed when the button is clicked, and using startTransition allows you to control the timing of the server action invocation.
To return a response, you can use res.status(200).json(users), as shown in the example where a simple array of users is returned.
Worth a look: Nextjs App vs Pages
Optimizing for Performance
Server-side data fetching can happen on every request, which can slow down your site if you're doing a lot of data fetching or computations.
To optimize the use of getServerSideProps for performance in Next.js 13, make sure you're returning the required props object with the props property. This can help reduce the number of repetitive calls and improve performance.
Returning the required props object can also help Next.js memoize the request and reuse the result for subsequent calls, which can significantly improve performance.
Smarter caching is another way to optimize the use of getServerSideProps in your project. It works by caching the results of your request based on the content that was requested, which can save a lot of time.
Here are some benefits of smarter caching:
- It allows for incremental updates, so if you make a small change to your code, only the affected parts of the site will be updated in the cache.
- It stores only the necessary data in the cache, so it can load the site faster for users.
By using smarter caching and returning the required props object, you can improve the performance of your site and make it more efficient.
Handling Preview Mode
Handling preview mode is essential for developers who need to test changes before publishing them. Next.js offers a preview mode that allows you to bypass static site generation and server-side rendering to display draft content from a CMS or other data source.
Recommended read: Next Js Strict Mode
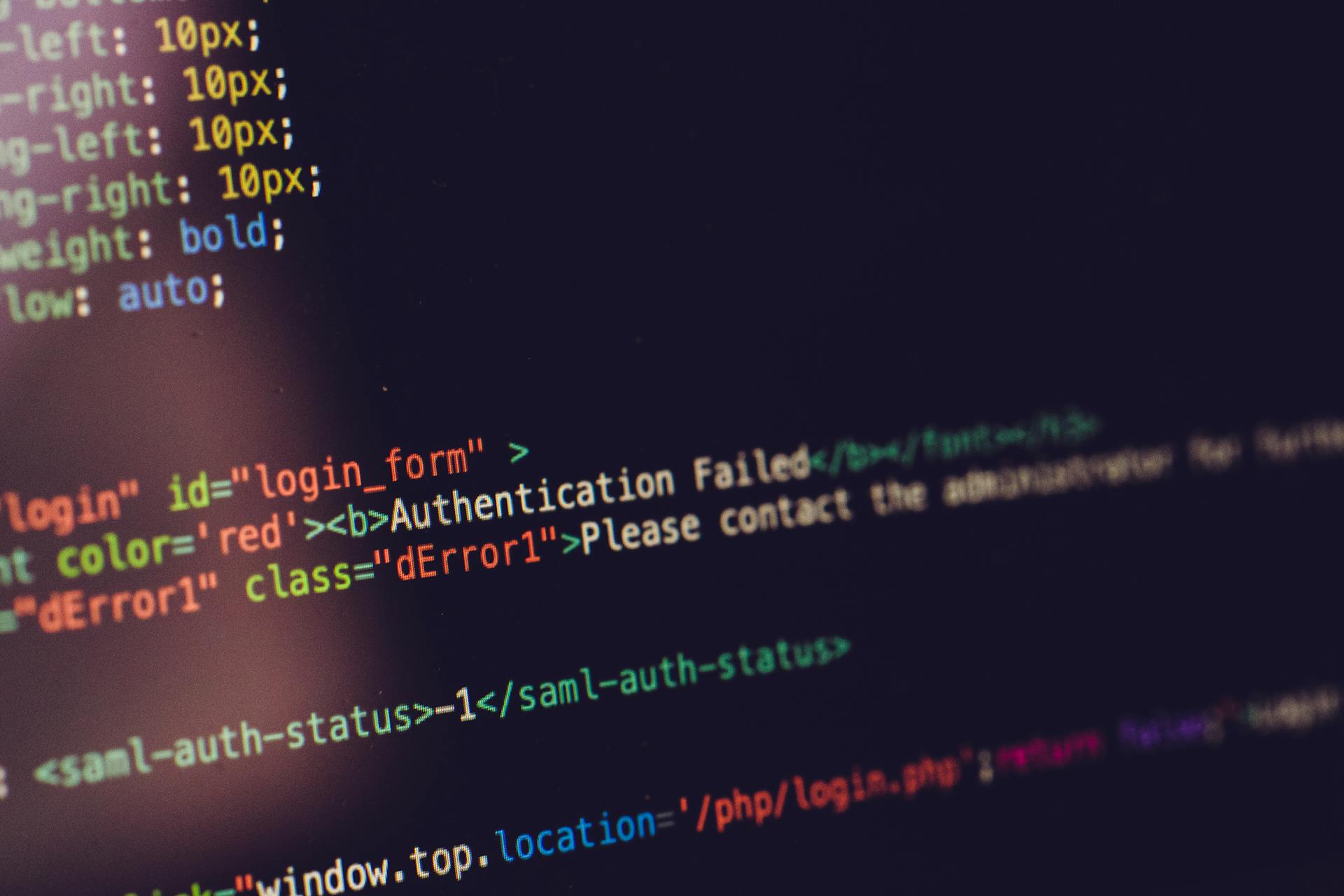
To enable preview mode, you can call `res.setPreviewData` inside an API route. This is particularly useful when you need to preview changes.
The `getServerSideProps` function will have access to preview data via the context object when preview mode is enabled. You can then use this data to fetch draft content.
In this approach, if preview mode is enabled, the function fetches draft data; otherwise, it fetches published data. This ensures that the correct version of the content is displayed based on whether preview mode is active.
Managing preview data sets involves ensuring that your `getServerSideProps` function correctly differentiates between preview and live content. This can be done by checking the context parameter.
By checking the context parameter, you can handle multiple data sets and display the correct version of the content based on whether preview mode is active.
Additional reading: Nextjs Getserversideprops
Redirection and Error Handling
Redirection and Error Handling is crucial for a seamless user experience. Next.js makes it easy to handle situations where a page needs to redirect to another URL based on certain conditions.
You can use the redirect object in getServerSideProps to send users to a different page. This is particularly useful when data is not found and you want to redirect to a /not-found page.
The redirect object specifies the destination and whether the redirect is permanent. In the example, if the fetched data is not found, the user is redirected to a /not-found page.
The notfound boolean in getServerSideProps is another way to handle cases where the data is not found. When set to true, it renders a 404 page automatically.
If the data is not found, the notFound property is set to true, causing Next.js to display the built-in 404 page. This saves you from having to create a custom 404 page from scratch.
Expand your knowledge: Nextjs App Loading Page Not Working
Advanced Features
With Next.js, you can leverage advanced features like getServerSideProps to fetch data on the server and render it on the client-side.
This allows you to pre-render pages at build time and fetch additional data on the server, reducing the amount of data transferred to the client.
Check this out: Next Js Debugging
getServerSideProps can be used to fetch data from APIs, databases, or even external services, making it a powerful tool for dynamic content rendering.
By using getServerSideProps, you can also cache the results of expensive API calls, reducing the load on your servers and improving performance.
You can also use getServerSideProps to handle authentication and authorization, ensuring that sensitive data is only accessible to authorized users.
This feature also enables you to use server-side rendering for static site generation, making it easier to create fast and secure websites.
Check this out: Nextjs Server Rendering Tailwind
Implementing SSR
Implementing SSR is a powerful way to fetch data on the server in Next.js. This approach ensures that your page is pre-rendered with the necessary data before it's sent to the client.
To implement SSR, you can use the getServerSideProps method, which is an asynchronous function that retrieves data from the server before producing a page. This method is ideal for pages that require dynamic data and frequent updates.
For more insights, see: Nextjs Loading Page Ssr
You can fetch data from both internal and external resources using getServerSideProps. This flexibility allows you to integrate various data sources into your application seamlessly. For instance, if you need to fetch data from an internal API, you can do so within the getServerSideProps function.
Here are some key benefits of using getServerSideProps for data fetching:
- Fetched data is available on the server side, ensuring up-to-date data for your page components.
- You can use various data fetching methods to retrieve data from internal or external sources.
- Dynamic route parameters can be accessed through the context object, allowing you to fetch data specific to a particular page.
Server Side Communication Using Server Actions
Server actions in Next.js are a powerful feature that enables you to handle server-side logic and perform operations such as data manipulation, authentication, and more.
To add server actions in your Next project, go into your next.config.js file and enable the experimental serverActions flag, allowing you to create custom server endpoints to handle server actions seamlessly.
Server actions are constructed upon React Actions and can initiate data alterations on the server-side, leading to decreased client-side JavaScript and enhanced forms that progress over time.
Server actions can be implemented using API routes in Next.js, which enables you to create custom server endpoints to handle server actions seamlessly.
A fresh viewpoint: Nextjs Custom Server
Here are the steps to implement a server action:
1. Define an asynchronous function with the "use server" directive at the top of the component function body.
2. The component takes a prop, which presumably represents the ID of the product being displayed on the card.
3. The addToCart function is an asynchronous function declared inside the component, triggered when the form is submitted.
4. Inside the addToCart function, there is a line "use server"; indicating that the following code should be executed only on the server-side.
5. The nextCookies().get('cartId')?.value code retrieves the value of the cartId cookie using the nextCookies function from the next/headers module.
6. The await saveToDatabase({ cartId, data }) code represents the asynchronous task of saving the data along with the cartId to the database.
By following these steps, you can implement server actions in your Next.js project, enabling you to handle server-side logic and perform operations such as data manipulation and authentication.
Intriguing read: Nextjs Code Block
Routing and Layouts
Routing and Layouts are crucial for a seamless user experience in Next.js. Each folder inside the app directory corresponds to a route segment, making the folder structure intuitive and easy to manage.
The structure is defined by page.js files, which create routes for the home page, about page, and dynamic blog post page. For example, a home page is defined by page.js directly inside the app directory.
Layouts in the app directory are defined using layout.js files, which provide a consistent structure across all pages within a specific directory or subdirectory. These layouts are hierarchical and can nest within each other, allowing for complex nested layouts.
Here's a breakdown of the layout structure:
- RootLayout provides a header and footer for the entire application.
- AboutLayout adds a sidebar for the "about" section.
- Nested Layouts are achieved by placing layout.js files within subdirectories.
- Dynamic Routing is implemented using square brackets in the folder names, such as [id] folder inside blog for dynamic routes for individual blog posts.
This structure supports dynamic routing and nested layouts, making it easy to create complex and dynamic applications with Next.js.
You might like: Nextjs Dynamic
Writing and Parsing
The getServerSideProps method in Next.js is an asynchronous function that retrieves data from the server before producing a page.
To define this function, you use the async keyword, allowing you to write asynchronous code using await.
The data is fetched from an API and passed to the page component as props, ensuring that the data fetching happens on the server side.
This approach provides up-to-date data to the page component.
The getServerSideProps function can access dynamic route parameters through the context object.
You can use dynamic route parameters to fetch specific data from an API, making the content tailored to the dynamic route.
The fetched data is then passed to the page component, providing a seamless user experience.
Here's an interesting read: Dynamic Route Nextjs 13
Context and Props
In Next.js, the context object is a crucial part of the getServerSideProps function, providing information about the incoming request.
The context object includes several properties, such as params, req, res, query, preview, previewData, locale, and defaultLocale, which can be used to tailor data fetching to the specifics of the request.
You can use these properties to ensure your page component receives the correct data. For example, you can access route parameters through the params property.
A different take: Next Js Fetch Data save in Context and Next Route
Here are the properties of the context object:
- params: Contains the route parameters for dynamic routes.
- req: The HTTP request object.
- res: The HTTP response object.
- query: The query string parameters.
- preview: Preview mode status.
- previewData: Data set in preview mode.
- locale: The active locale configured for internationalized routing.
- defaultLocale: The default locale configured for internationalized routing.
To fetch data on the server with getServerSideProps, you can use various data fetching methods to retrieve data from internal or external sources.
Sources
- https://refine.dev/blog/next-js-server-actions-and-data-fetching/
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://blog.logrocket.com/data-fetching-next-js-getserversideprops-getstaticprops/
- https://www.dhiwise.com/post/mastering-nextjs-getserversideprops-a-comprehensive-guide
- https://www.dhiwise.com/post/streamline-development-workflow-with-nextjs-app-directory
Featured Images: pexels.com