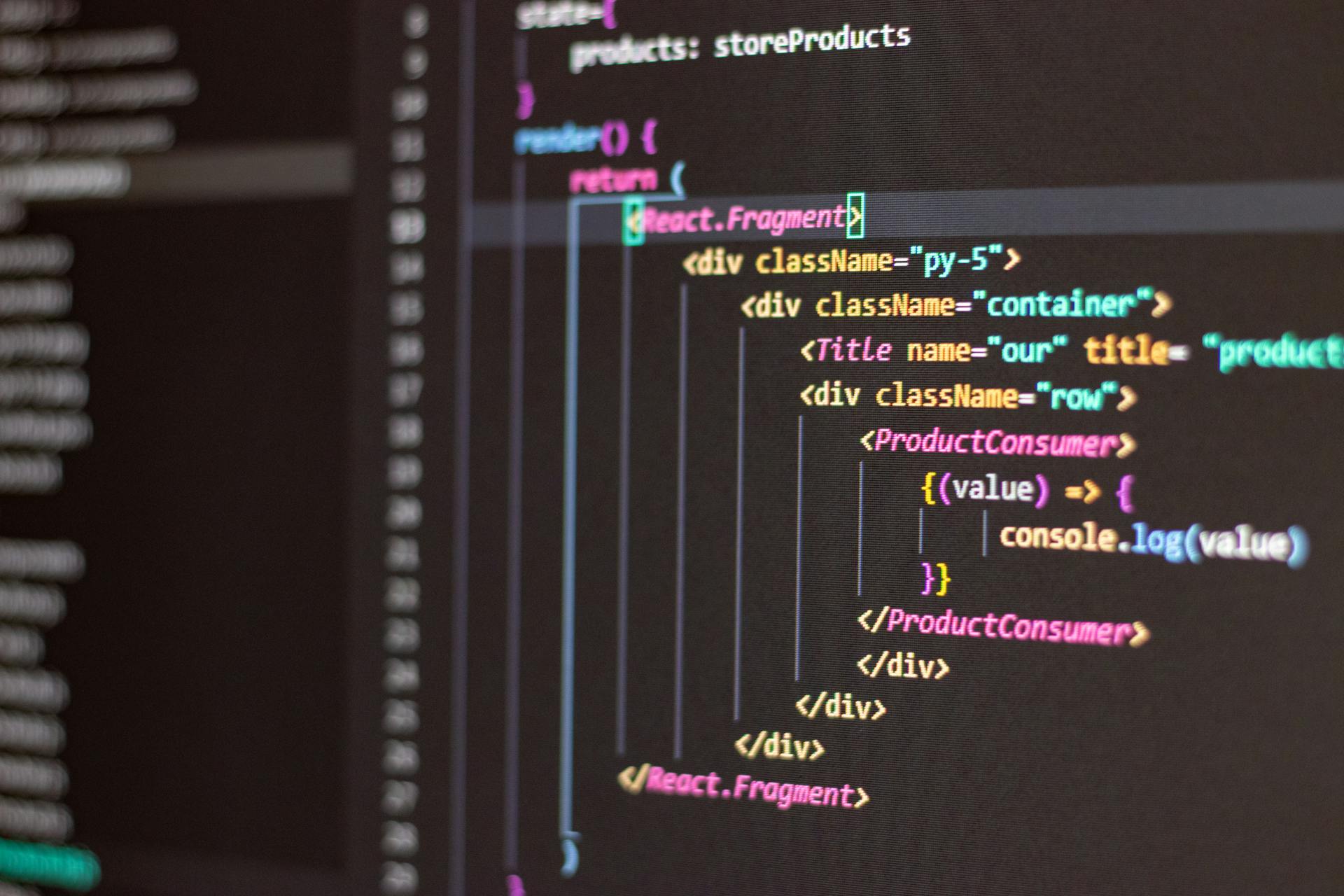
Next.js is a popular React-based framework for building server-rendered and statically generated websites and applications. This is made possible by its client-side rendering feature, which allows for faster page loads and improved SEO.
Client-side rendering in Next.js occurs on the client's web browser, where the application's UI components are rendered dynamically as the user interacts with the page. This is in contrast to server-side rendering, where the UI components are rendered on the server before being sent to the client.
One of the key benefits of client-side rendering in Next.js is that it allows for faster page loads, as the initial HTML is sent to the client quickly, and the UI components are loaded dynamically as the user interacts with the page. This results in a smoother user experience and improved performance.
By leveraging client-side rendering, Next.js developers can also improve SEO by allowing search engines to crawl and index the content of the page more efficiently.
What Is Client-Side Rendering
Client-side rendering is a key concept in Next.js, and it's essential to understand how it works. A Client Component is pre-rendered on the server and hydrated on the client.
This means that the initial HTML is generated on the server, but it's not interactive yet. The component becomes interactive only after JavaScript executes on the client-side. Client Components aren't entirely client-side, as Next.js tries to do as much work as possible in the pre-rendering step.
To turn a component into a Client Component, you need to add the use client directive. This is necessary if the component requires client-side interactivity, such as using useState or onClick events. Adding the use client directive is a straightforward way to make a component interactive.
Pre-rendering is when HTML is generated in advance, with minimal JavaScript. Hydration occurs once the page gets loaded into the browser, and JavaScript executes, making the component interactive. Next.js uses pre-rendering to alleviate the work required client-side, making it a powerful feature.
How It Works
Next JS uses a technique called hydration to bring interactivity to the DOM. This process involves sending the JS code to the browser after the DOM is displayed.
Client components are initially pre-rendered on the server, which means the user gets to see something before the JS code is even sent. The server sends the pre-rendered DOM to the client, giving the user a head start.
Server component trees are a way to organize server components for maximum efficiency. By structuring your components effectively, you can minimize the data transferred to the client for each request.
A Client Component is pre-rendered on the server and then hydrated on the client. This means the component is rendered partially on the server and then completed on the client.
Optimizing Client-Side Rendering
Optimizing client-side rendering is crucial for a seamless user experience. To do this, Next.js allows you to pre-render components on the server and hydrate them on the client.
To fully harness the power of client-side rendering, you should understand how to optimize the loading and rendering of client components. This includes using the process.browser check from Webpack to run client-only code.
By pre-rendering components on the server and hydrating them on the client, you can achieve partial pre-rendering (PPR). To enable PPR, add the following code to your next.config.js file.
Optimizing
Optimizing client-side rendering requires a deep understanding of how to load and render client components efficiently. This involves optimizing the loading of client components to ensure a smooth user experience.
To fully harness the power of client-side rendering, you need to understand how Next.js works. Next.js is a popular framework for building server-rendered and statically generated websites.
Optimizing the loading of client components is crucial for a seamless user experience. By minimizing the number of requests made to the server, you can improve page load times and reduce the risk of errors.
Client-side rendering can be optimized by using techniques such as lazy loading and code splitting. These techniques allow you to load only the necessary code and components, reducing the overall load time.
A good understanding of how to optimize client-side rendering can be gained by experimenting with different approaches and analyzing their impact on performance.
Enabling Partial Pre
Enabling Partial Pre is a crucial step in optimizing client-side rendering. To do this, you need to add a specific line of code to your next.config.js file.
After the project is initiated, go to next.config.js and add the following: To enable partial pre-rendering (PPR), you'll need to add this line of code.
This will allow for partial pre-rendering, which is a process where a Client Component is pre-rendered on the server and hydrated on the client. Client Components are rendered partially client-side, but not entirely.
Error Handling and Hydration
Error handling is crucial for a good user experience, especially when rendering components on the client side. You should anticipate potential issues such as network errors or missing data and provide appropriate fallbacks or error messages.
Proper error handling is not just about hiding errors, but about providing a good user experience. In some cases, suppressing errors might seem like an easy fix, but it's not a permanent solution.
The `suppressHydrationWarning` attribute can be used to hide errors, but it's not recommended as it only works one level deep and can impact site performance. If you do decide to use it, be aware of the potential issues.
Here are some things to consider when using `suppressHydrationWarning`:
- It only works one level deep
- This should be avoided if at all possible
- All the patching could impact site performance in some scenarios
Hydration and pre-rendering are related concepts in Next.js. Hydration occurs when JavaScript executes and your component becomes interactive, while pre-rendering generates HTML in advance with minimal JavaScript.
Pre-rendering is done in two forms: Static and Server-Side. The method used depends on how you fetch your data in Next.js.
Next.js Features for Client-Side Rendering
Next.js offers several features that make client-side rendering a breeze. The app router ensures subsequent navigations within the application are handled efficiently, reducing data transfer and improving performance.
Next.js uses the process.browser check to determine if code should run on the client-side, making it easy to implement client-only code. This check is inherited from Webpack, making the process surprisingly straightforward.
A Client Component is pre-rendered on the server and then hydrated on the client, allowing for interactive client-side rendering. This process makes client components feel fully rendered on the client-side.
Next.js Handles
Next.js Handles Client-Side Rendering with ease, delivering server-rendered HTML to the user's device initially. This approach allows for a fast initial page load.
The app router takes over after the initial load, managing subsequent navigations within the application by fetching and rendering only necessary client components.
Hydration is a crucial step where Next.js attaches event listeners and makes static HTML interactive, enabling client-side interactivity.
A Client Component is pre-rendered on the server, which means it's generated by server components and delivered to the client.
Static Site Generation
Static Site Generation is a process that generates static HTML files at build time, before the user even requests the page. This happens before deployment when the developer builds the application.
The server simply serves the pre-generated HTML file when the user requests a page, eliminating the need to render the content on the server. Modern frameworks still include JavaScript for interactivity and additional functionality if needed.
This method is great for websites that don't need to be updated frequently, such as blogs or portfolio websites. It's also easy to cache the HTML files for even faster load times.
Static Site Generation is good for SEO because search engines can easily index the content since it's already in the HTML files. However, if you need the content to be dynamic, this isn't the right approach.
The main problem with Static Site Generation is that the content is completely static, meaning the HTML files are generated before deployment and don't change until the next build.
Frequently Asked Questions
Does Next.js use client-side routing?
No, Next.js uses client-side navigation, but it doesn't require a separate routing library. Instead, it uses a built-in Link component to manage client-side routing.
Sources
- https://www.dhiwise.com/post/nextjs-client-side-rendering-what-you-need-to-know
- https://blog.hao.dev/render-client-side-only-component-in-next-js/
- https://javascript.plainenglish.io/next-js-rendering-patterns-a-comprehensive-guide-e20a092f0f21
- https://www.thetombomb.com/posts/use-client-nextjs
- https://tigerabrodi.blog/nextjs-client-side-vs-server-side-rendering-vs-static-site-generation
Featured Images: pexels.com