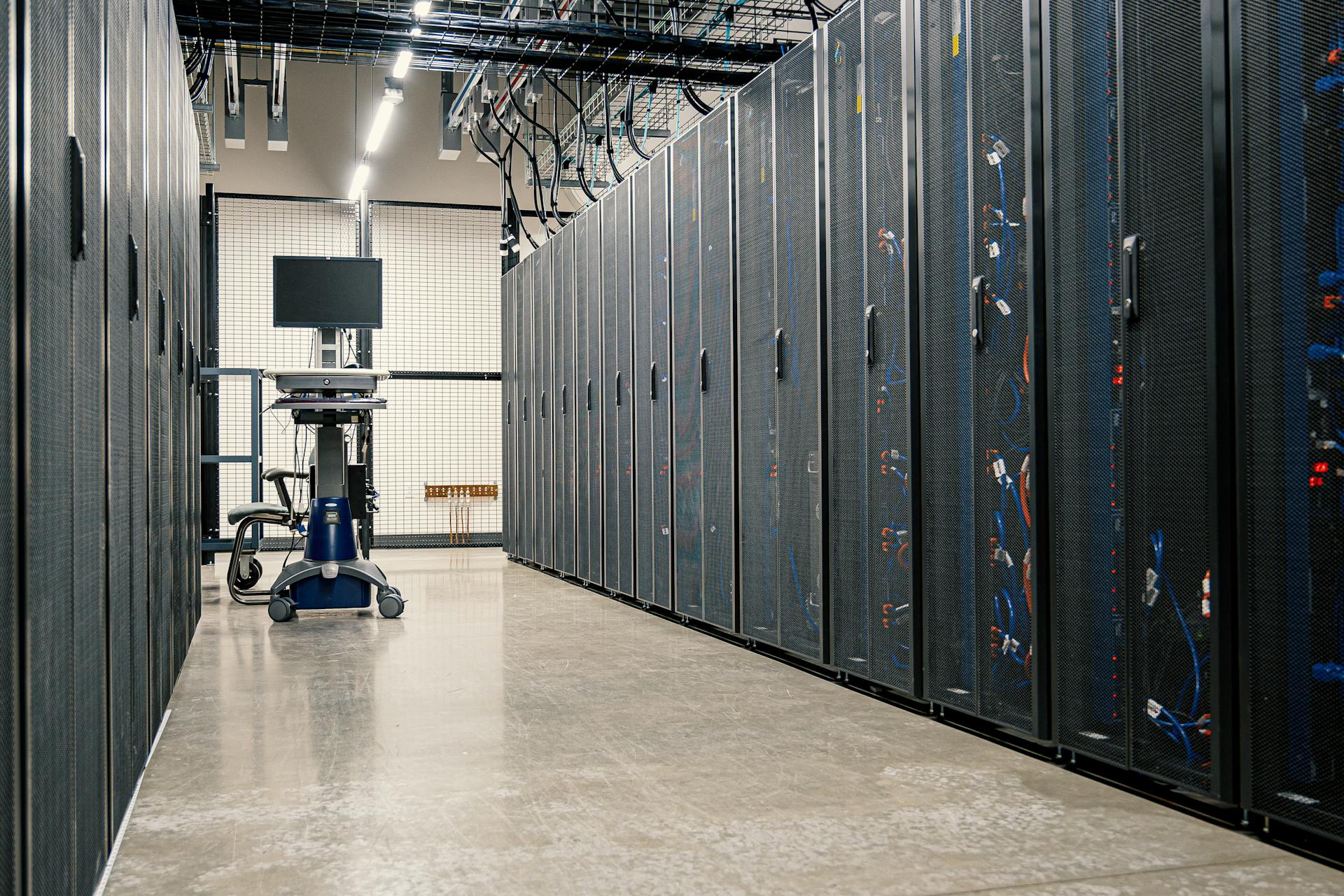
The Azure Container Client is a powerful tool that enables you to manage and interact with containers in Azure. It provides a simple and consistent way to work with containers across different environments.
To get started with the Azure Container Client, you'll need to install the Azure CLI on your machine. This can be done by running the command `az config` in your terminal.
The Azure Container Client supports various container orchestration systems, including Kubernetes and Docker Swarm. This makes it an ideal choice for developers who need to work with multiple container platforms.
With the Azure Container Client, you can create, list, and delete container instances, as well as manage container groups and orchestrations.
Constructors and Initialization
You can create an instance of the Azure Container Client using various constructors.
The BlobContainerClient class has several constructors, each with different parameters. For example, you can initialize a new instance of the class with a connection string, blob container name, and blob client options.
Here are some of the constructors you can use:
You can also create an instance of the ContainerClient class using various constructors. For example, you can create an instance with a URL pointing to a container, a storage shared key credential, and storage pipeline options.
To create a new container, you can use the BlobServiceClient.getContainerClient() method to get a container client instance, then create a new container resource.
The ContainerClient class has several constructors, each with different parameters. For example, you can create an instance with a URL string pointing to Azure Storage container, a storage shared key credential, and storage pipeline options.
Here are some of the constructors you can use:
Properties and Methods
The Azure Container Client has several properties and methods that make it easy to work with containers. The Properties property gives you access to the container's name, URI, and other useful information.
You can use the Name property to get the name of the container, which is a string value. The Uri property returns the container's primary URI endpoint, which is also a string value.
The get_container_properties method returns all user-defined metadata and system properties for the specified container, but it doesn't include the container's list of blobs.
Here's a summary of the Properties property:
You can also use the byPage() method to list the blobs in pages, which is an async iterable iterator. This is useful when you need to work with a large number of blobs.
The credential property is used to authenticate requests to the service, and you can provide an object that implements the TokenCredential interface. If not specified, AnonymousCredential is used.
Blob Operations
Blob operations are a crucial part of working with Azure Container Client. You can download a blob to a StorageStreamDownloader using the download_blob method, which requires a blob, an offset, and a length.
To download a blob in chunks, you can use the chunks() method, which returns an iterator that allows you to iterate over the content in chunks. This is useful for handling large blobs.
The upload_blob method creates a new blob from a data source with automatic chunking. It requires a name, data, blob type, length, and metadata. The blob type can be BlockBlob, PageBlob, or AppendBlob, with BlockBlob being the default.
Upload Blob
Uploading a blob is a straightforward process that can be done in a few easy steps. You can create a new blob from a data source with automatic chunking.
To do this, you'll need to specify the blob with which to interact, which is a required parameter. This can be a string, and it's the blob that you'll be working with.
The blob data to upload is also a required parameter, and it can be in the form of bytes, a string, an iterable of any string type, or an input/output stream of any string type. This means you can upload a variety of data types, from simple text to more complex binary data.
You'll also need to specify the type of blob you're creating, which can be either BlockBlob, PageBlob, or AppendBlob. The default value is BlockBlob, but you can choose the type that best suits your needs.
If you're uploading a large amount of data, it's a good idea to specify the length of the data you're uploading. This can help improve performance and ensure that your upload goes smoothly.
Finally, you can add metadata to your blob by specifying a dictionary of name-value pairs. This can be useful for storing additional information about your blob, such as its contents or any relevant context.
Here's a summary of the parameters you'll need to specify when uploading a blob:
Get Blob Client
To get started with blob operations, you'll need to get the Blob Client, which is a client library that allows you to interact with Azure Blob storage.
The Blob Client is available in multiple languages, including .NET, Java, and Python.
You can install the Blob Client using NuGet for .NET, Maven for Java, or pip for Python.
The Blob Client provides a simple and intuitive API for performing common blob operations, such as creating, updating, and deleting blobs.
You can use the Blob Client to interact with blob containers, including listing, creating, and deleting containers.
The Blob Client also provides features like caching and retry logic to help you handle errors and improve performance.
To use the Blob Client, you'll need to create a BlobServiceClient instance, which will give you access to the blob service.
Blob Retrieval and Management
To download a blob, you'll need to use the download_blob method, which takes a blob, an offset, and an optional length as parameters. This method returns a StorageStreamDownloader that you can use to read or download the blob content.
You can choose to read all the content using the readall() method or download it into a stream using readinto(). Alternatively, you can use chunks() to iterate over the content in chunks.
The download_blob method requires a blob, an offset, and an optional length. If you provide a length, you must also set the offset.
Here's a quick reference to the download_blob method parameters:
To list the names of blobs under a specified container, you can use the list_blob_names method, which returns a generator that lazily follows the continuation tokens returned by the service.
Download Blob
To download a blob, you'll want to use the download_blob method. This method allows you to interact with a blob and download its content.
The download_blob method requires a blob, which can be specified as a string. You can also provide an offset and length to download a specific section of the blob.
If you want to download the entire blob, you can use the readall() method. Alternatively, you can use readinto() to download the blob into a stream.
For optimal performance, it's a good idea to provide the length of the blob you want to download. This will help the method download the correct amount of data.
Here's a summary of the required parameters for the download_blob method:
Delete Blob
Deleting a blob can be a permanent action, so it's essential to double-check that you're deleting the correct blob. This is especially important when working with large datasets.
To delete a blob, you'll need to use the `Delete Blob` operation in Azure Storage. This operation will permanently remove the blob from the storage account.
Before deleting a blob, make sure you've downloaded any necessary backups or copies. This is a crucial step to prevent data loss.
The `Delete Blob` operation returns a 204 No Content status code, indicating that the blob has been successfully deleted. This is a confirmation that the operation was successful.
After deleting a blob, you can verify that it's no longer accessible by attempting to retrieve it. If the blob is deleted, you'll receive a 404 Not Found error.
Get Append Blob Client
To get an Append Blob client, you'll need to create a new instance of the AppendBlobClient class. This class is used to interact with append blobs, which are a type of blob that allows you to add new data to the end of the existing blob.
The AppendBlobClient class requires a BlobServiceClient instance to be created first. This BlobServiceClient instance is used to access and manage blobs in a storage account. You can create a BlobServiceClient instance by providing the URL of the storage account and an account key.
You can then use the BlobServiceClient instance to create an AppendBlobClient instance, which will allow you to interact with the append blob. This is a crucial step in retrieving and managing blobs, as it enables you to perform operations such as creating, updating, and deleting blobs.
Get Account Info
Getting account info is a crucial step in Blob Retrieval and Management.
To access your account information, you can log in to your account dashboard.
Your account dashboard will display your account name, which is a unique identifier for your account.
You can also view your account ID, which is a numerical identifier that is associated with your account.
To view your account's storage capacity, you'll need to access the storage settings section of your account dashboard.
Your account's storage capacity determines how much data you can store in your account.
Note that your account's storage capacity can be increased or decreased as needed.
To check your current storage usage, you can view the storage usage graph on your account dashboard.
Security and Authentication
To authenticate requests to the Azure Storage service, you can use various types of credentials, including AnonymousCredential, StorageSharedKeyCredential, or TokenCredential from the @azure/identity package.
You can also provide an object that implements the TokenCredential interface. If not specified, AnonymousCredential is used.
For Azure Active Directory (AAD) token credential, you can use an instance of DefaultAzureCredential from the azure-identity library, which requires some initial setup.
- Use the returned token credential to authenticate the client.
- Provide an instance of the desired credential type obtained from the azure-identity library.
Alternatively, you can use a shared access signature (SAS) token or a storage account shared key (also known as an account key or access key).
Storage Credentials and Options
Storage credentials are an essential part of authenticating requests to Azure Storage. You can provide a credential in various forms, including an instance of a credential type obtained from the azure-identity library, a shared access signature (SAS) token, or an account shared key.
To use an Azure Active Directory (AAD) token credential, you can use the DefaultAzureCredential class from the azure-identity library. This requires some initial setup, but it allows you to authenticate the client using a token credential.
A SAS token can be generated from the Azure Portal under "Shared access signature" or using one of the generate_sas() functions to create a sas token for the storage account, container, or blob. If your account URL includes the SAS token, you can omit the credential parameter.
You can also use an account shared key, which can be found in the Azure Portal under the "Access Keys" section or by running the Azure CLI command az storage account keys list. This can be used as the credential parameter to authenticate the client.
Here are the different types of credentials you can use:
Omitting the credential parameter is equivalent to using anonymous public read access, which allows anyone to read data from the container. This is useful for public-facing containers, but be aware that it can compromise security if not used carefully.
User Delegation Key
User Delegation Key is a way to authenticate with Azure Blob Storage by using a shared key credential. This allows you to sign Shared Access Signatures (SAS) with a user delegation key.
You can generate a string to sign for a SAS URI using the `generateUserDelegationSasStringToSign` method, which takes in `ContainerGenerateSasUrlOptions` and a `UserDelegationKey` as parameters.
The `generateUserDelegationSasUrl` method generates a SAS URI based on the client properties and parameters passed in, signed by the input user delegation key.
If you're using a ContainerClient constructed with a shared key credential, you can use the `generateSasStringToSign` method to generate a string to sign for a SAS URI.
Note that the `generateSasStringToSign` method has a warning about JavaScript Date losing precision when parsing startsOn and expiresOn strings.
Here's a summary of the methods related to user delegation key:
Encryption Configuration
Encryption is a crucial aspect of security, and configuring it properly is essential. To ensure encryption is enforced, you can set the require_encryption argument to True when instantiating a client.
The encryption version used can be specified with the encryption_version argument. It's recommended to use version 2.0, as version 1.0 is deprecated.
You can also use a user-provided key-encryption-key by passing it as the key_encryption_key argument. This key must implement specific methods.
Here are the details of the encryption configuration arguments:
- require_encryption (bool): If set to True, will enforce that objects are encrypted and decrypt them.
- encryption_version (str): Specifies the version of encryption to use. Current options are '2.0' or '1.0' and the default value is '1.0'. Version 1.0 is deprecated, and it is highly recommended to use version 2.0.
- key_encryption_key (object): The user-provided key-encryption-key. The instance must implement the following methods: key_resolver_function (callable).
Signed Identifier Array
A Signed Identifier Array is a crucial component in setting access policies for containers. It's an array of elements, each with a unique Id and details of the access policy.
Each element in the Signed Identifier Array represents a specific access policy, with its own unique Id and settings. This allows for fine-grained control over who can access the container and under what conditions.
The Signed Identifier Array is used in conjunction with the PublicAccessType to set access policies for containers. This combination gives you a high degree of flexibility in managing access to your container data.
The array can be empty, in which case the existing container ACL will be removed. This can be a deliberate choice, but it's also worth noting that if no access or containerAcl is provided, the existing container ACL will be removed.
Configuration and Settings
Configuration and settings for the Azure Container Client are crucial to ensure it works as expected. The client has several configuration options that can be specified when instantiating it.
You can configure the retry policy by passing in keyword arguments such as retry_total, retry_connect, retry_read, and retry_status. These arguments allow you to control the number of retries for different types of errors.
To configure other client or per-operation settings, you can use keyword arguments such as connection_timeout, read_timeout, and transport. You can also use per-operation keyword arguments like raw_response_hook, raw_request_hook, and client_request_id to customize the request.
Here is a summary of the retry policy configuration options:
Client/Config
You can configure the client to suit your needs by using various options. The connection timeout defaults to 20 seconds, but you can change it to a different value if needed.
If you're experiencing issues with reading data from the server, you can adjust the read timeout to a longer period, such as 60 seconds, to give it more time to respond.
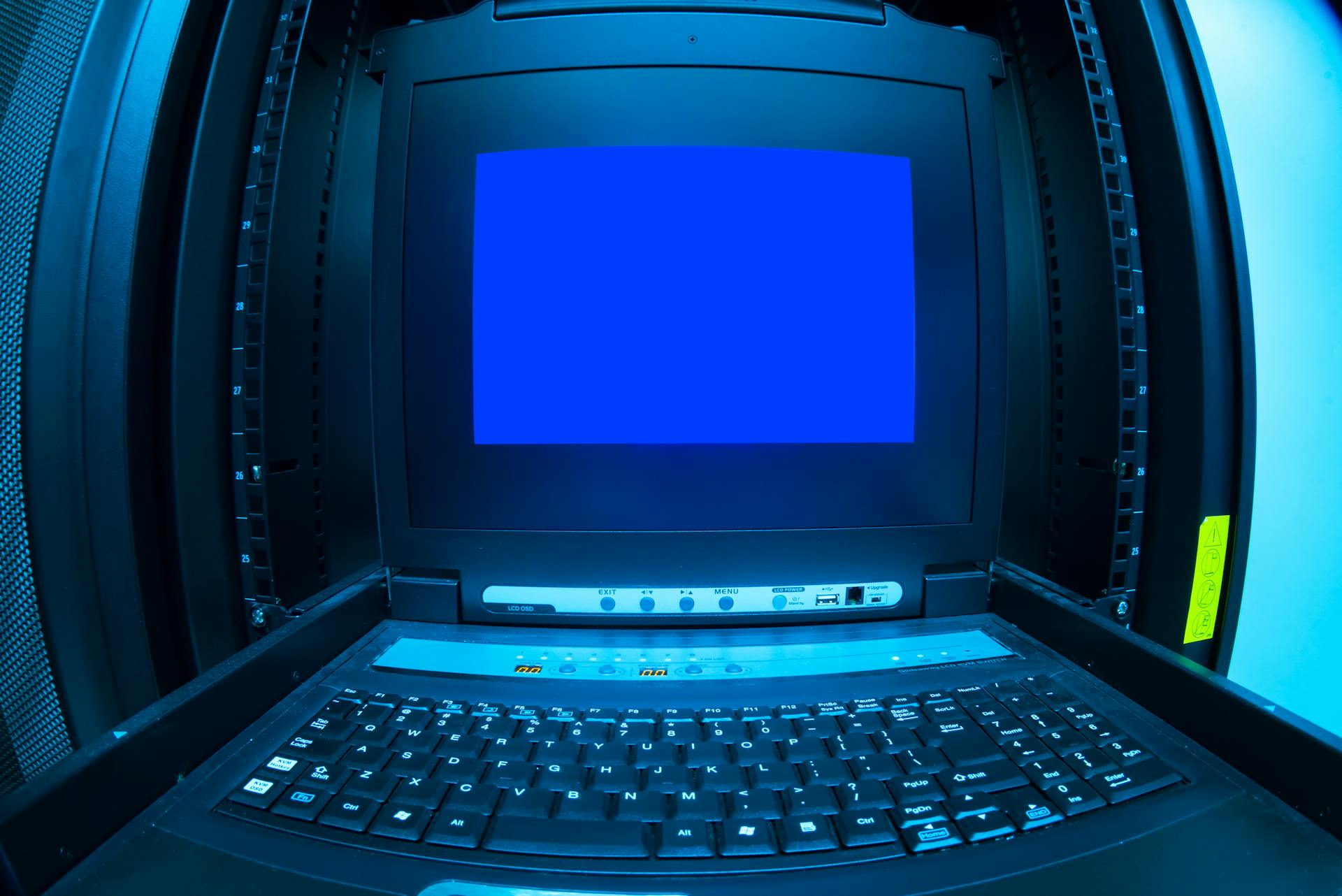
One option for authentication is to use a user-provided transport to send the HTTP request. This can be especially useful if you're working with a custom setup.
You can also pass in a custom callback function to use the response returned from the service. This is particularly useful for debugging purposes.
To add a custom identification to your requests, you can specify a client request ID. This can be helpful for tracking and debugging purposes.
You can also append a custom value to the user-agent header to be sent with the request. This is useful for identifying your application or service.
If you want to enable logging at the DEBUG level, you can pass in the logging_enable parameter set to True. This will log all requests and responses.
You can also log the request and response body by passing in the logging_body parameter set to True. This will provide a detailed view of the data being exchanged.
If you need to pass in custom headers, you can do so by passing in a dictionary of key-value pairs. This is useful for adding additional metadata to your requests.
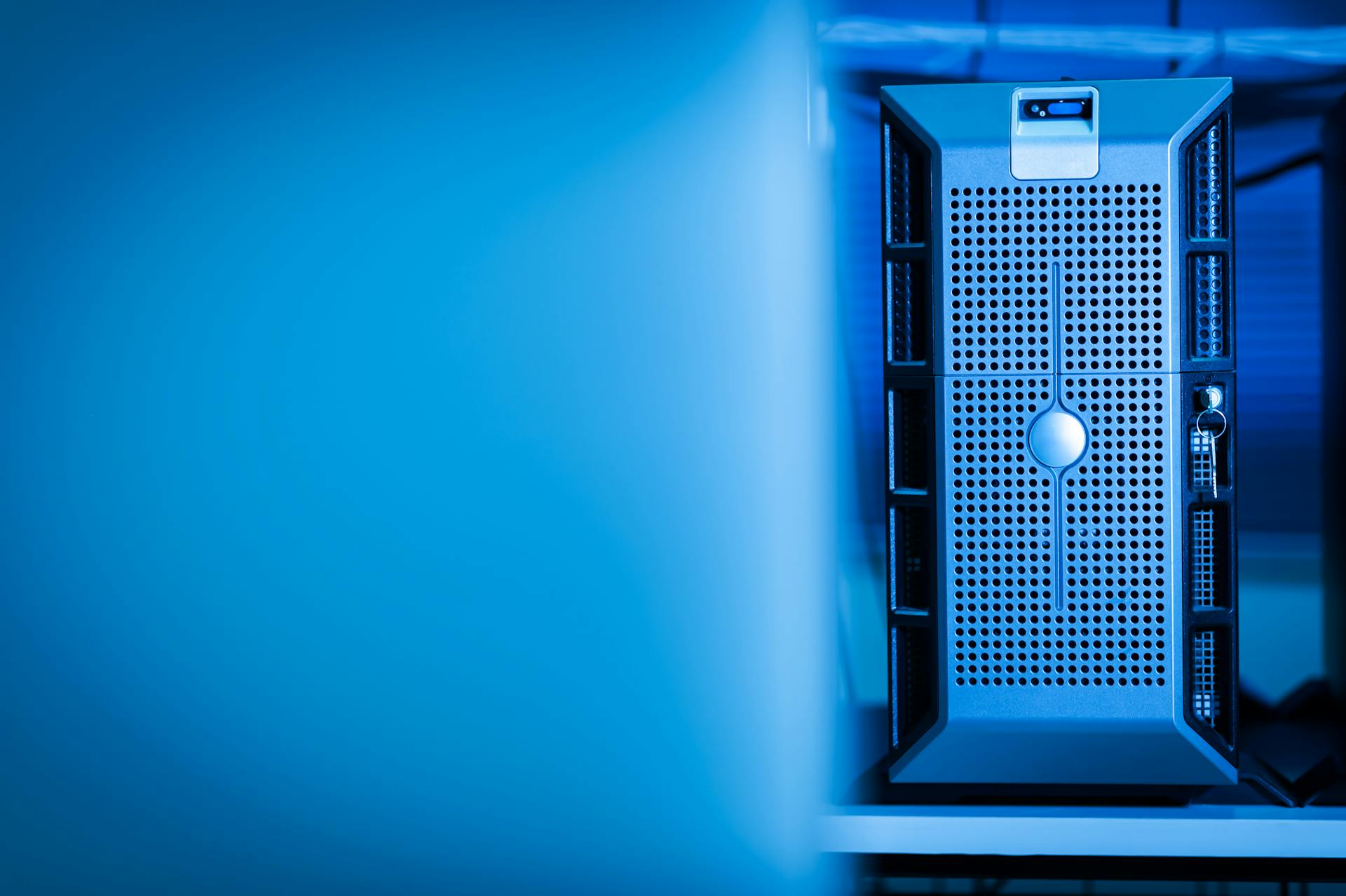
Here's a summary of the client configuration options:
Options
Options play a crucial role in configuring and setting up your Azure Storage account. You can provide an object that implements the TokenCredential interface to authenticate requests to the service.
Options to configure the HTTP pipeline are optional, but they can be specified to customize the behavior of the client.
You can use various credentials to authenticate requests, such as AnonymousCredential, StorageSharedKeyCredential, or any credential from the @azure/identity package.
Options to Container Create operation, such as ContainerCreateOptions, can be used to customize the creation of a new container.
Here are some common options used in Azure Storage client:
Options to Container Set Metadata operation, such as ContainerSetMetadataOptions, can be used to customize the setting of metadata for a container.
If no option is provided, or no metadata is defined in the parameter, the container metadata will be removed.
Retry Configuration
Retry Configuration is a crucial aspect of ensuring your client is resilient to errors. It's a good idea to configure retry policy when instantiating a client.
The total number of retries to allow can be specified with the retry_total argument, which takes precedence over other counts. By default, it's set to 10, but you can pass in retry_total=0 if you don't want to retry on requests.
You can also configure how many connection-related errors to retry on with the retry_connect argument, which defaults to 3. Similarly, you can specify how many times to retry on read errors with the retry_read argument, which also defaults to 3.
Another important aspect of retry configuration is how many times to retry on bad status codes, which can be specified with the retry_status argument and defaults to 3.
If you're using RA-GRS accounts and potentially stale data can be handled, you might want to consider enabling retry_to_secondary, which defaults to False.
Here's a quick rundown of the retry configuration options:
Frequently Asked Questions
What is blob container client?
The BlobContainerClient is a tool that enables you to manage and manipulate Azure Storage containers and their associated blobs. It's a powerful way to interact with your cloud storage resources.
Sources
- https://learn.microsoft.com/en-us/dotnet/api/azure.storage.blobs.blobcontainerclient
- https://learn.microsoft.com/en-us/python/api/azure-storage-blob/azure.storage.blob.containerclient
- https://learn.microsoft.com/en-us/javascript/api/@azure/storage-blob/containerclient
- https://pypi.org/project/azure-storage-blob/
- https://www.npmjs.com/package/@azure/storage-blob
Featured Images: pexels.com