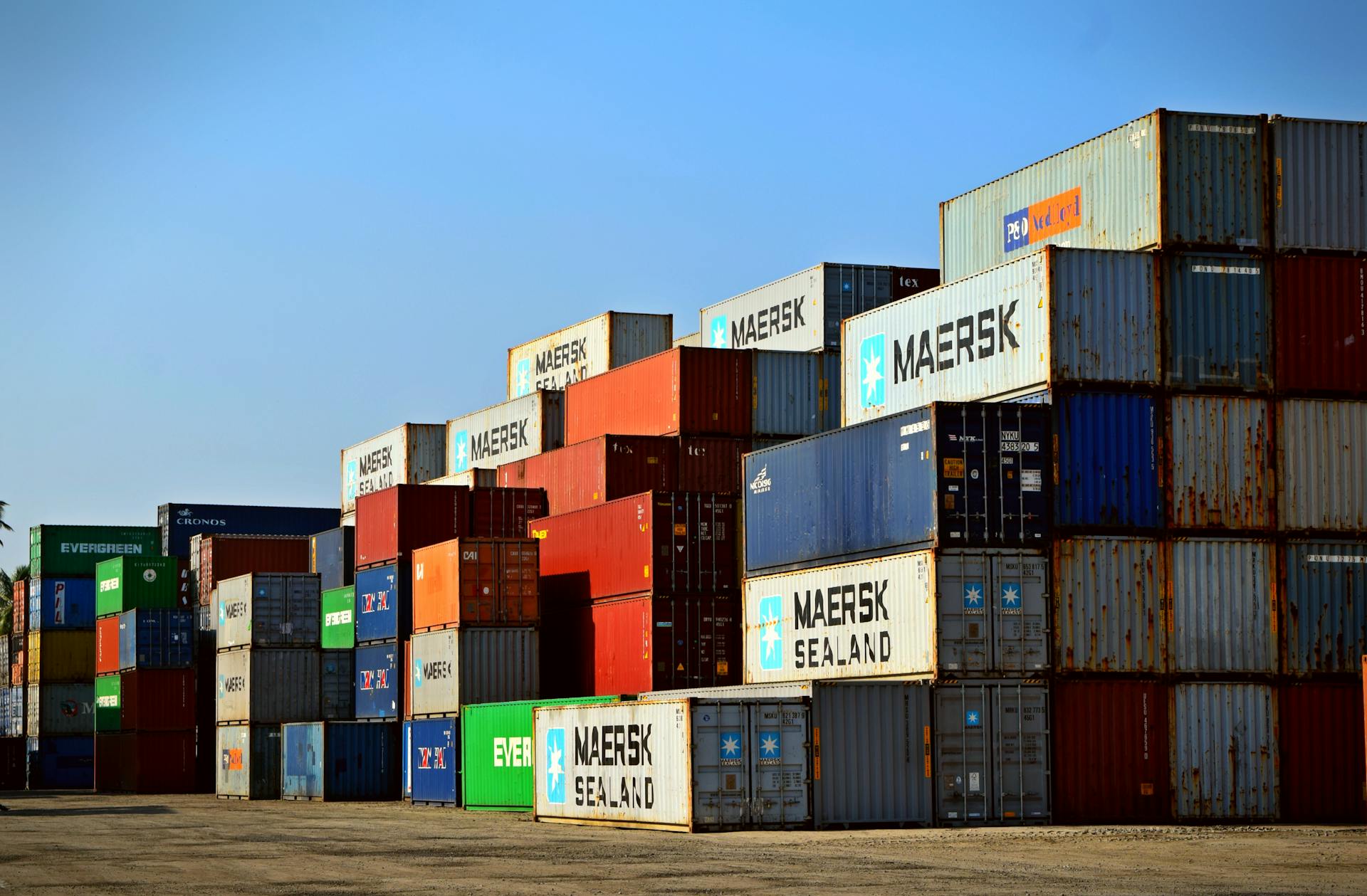
To move files between Azure Blob Storage containers in C#, you can use the Azure Blob Storage client library. This library provides a simple and efficient way to interact with Azure Blob Storage.
First, ensure you have the Azure Blob Storage client library installed in your project. You can install it via NuGet package manager.
To move a file from one container to another, you need to create a new instance of the BlobClient class, specifying the source and destination container names. The source container is where the file currently resides, and the destination container is where you want to move the file.
You can use the CopyFromUriAsync method to copy the file from the source container to the destination container. This method takes a URI of the source file as a parameter and returns a task that represents the asynchronous copy operation.
A different take: Azure Key Vault C#
Prerequisites
To get started with moving files between containers in Azure Blob Storage using C#, you'll need to meet a few prerequisites. You'll need an Azure subscription, which you can create for free if you don't already have one.
Before you begin, make sure you have two existing Azure Storage accounts, each with Blobs in one of them. This will allow you to work with the files you want to move between containers.
Here are the specific requirements:
- An Azure subscription (create a free account if you don't already have one)
- Two existing Azure Storage accounts with Blobs in one of them
Storage Account Credentials
To access your Azure Blob Storage container and upload files, you'll need to obtain your Storage Account Credentials. You can do this by going to Settings > Access Keys, where you'll find your Storage Account Name and Access Key, or Connection String.
You have two options to obtain these credentials: Storage Account Name and Access Key, or Connection String. Both options are available in the same place: Settings > Access Keys.
Here are the two options for accessing your Azure Blob Storage credentials:
These credentials are essential for accessing your Azure Blob Storage container and uploading files.
Installing Storage Blobs Client Library
To install the Azure Storage Blobs Client Library, you need to use the Azure.Storage.Blobs package from NuGet. This is the recommended way to access Azure Blob storage in .NET.
The version required for this task is 12.7.0. You can add this package using PowerShell with the command `dotnet add package Azure.Storage.Blobs`.
Inside your class, such as Azure.cs, you'll need to add a new using directive for the Azure.Storage.Blobs reference to avoid having to write the full namespace in front of your commands. This will save you time and make your code more readable.
For more insights, see: How to Connect to Azure Cosmos Db Using Connection String
Moving Files Between Containers
Moving files between containers in Azure Blob Storage can be a bit tricky, but it's doable. You can use Azure Storage Explorer to move Blobs between containers or between storage accounts.
Azure Storage Explorer is a tool that allows you to manage your Azure Storage resources, including moving Blobs between containers. It's a user-friendly interface that makes it easy to navigate and manage your storage resources.
To move a Blob, you'll need to create a storage container with folder hierarchies and store the Blob in it. Then, you can use Azure Storage Explorer to move the Blob to another container or storage account.
Readers also liked: Azure Blob Explorer
Here are the steps to move a Blob using Azure Storage Explorer:
- Create a storage container with folder hierarchies and store the Blob in it.
- Open Azure Storage Explorer and navigate to the container where the Blob is stored.
- Right-click on the Blob and select "Copy" to copy the Blob to the clipboard.
- Navigate to the destination container and right-click on the container to select "Paste" to paste the Blob into the new container.
Alternatively, you can use an Azure Function to move the Blob between containers. This involves using the Azure.Storage.Blobs NuGet package and writing a C# script to perform the necessary steps to copy the Blob.
Triggering and Customizing
There are a few methods to configure an Azure Function to get triggered on new blobs being created in an Azure Storage Account, such as Blob Trigger, Event Grid, etc.
Using the Blob Trigger is a simpler approach and a long tested and verified feature of Azure Functions that allows you to watch an Azure Storage Account Container for new blobs and automatically trigger execution of the Function App when new blobs are created within the Container.
On a similar theme: Azure Logic App Blob Storage Trigger
Triggering on New
Triggering an Azure Function on new blobs being created in an Azure Storage Account is a powerful feature.
There are a few methods to achieve this, but a simpler approach is to use the Blob Trigger for the Function App, which utilizes the long tested and verified feature of Azure Functions.
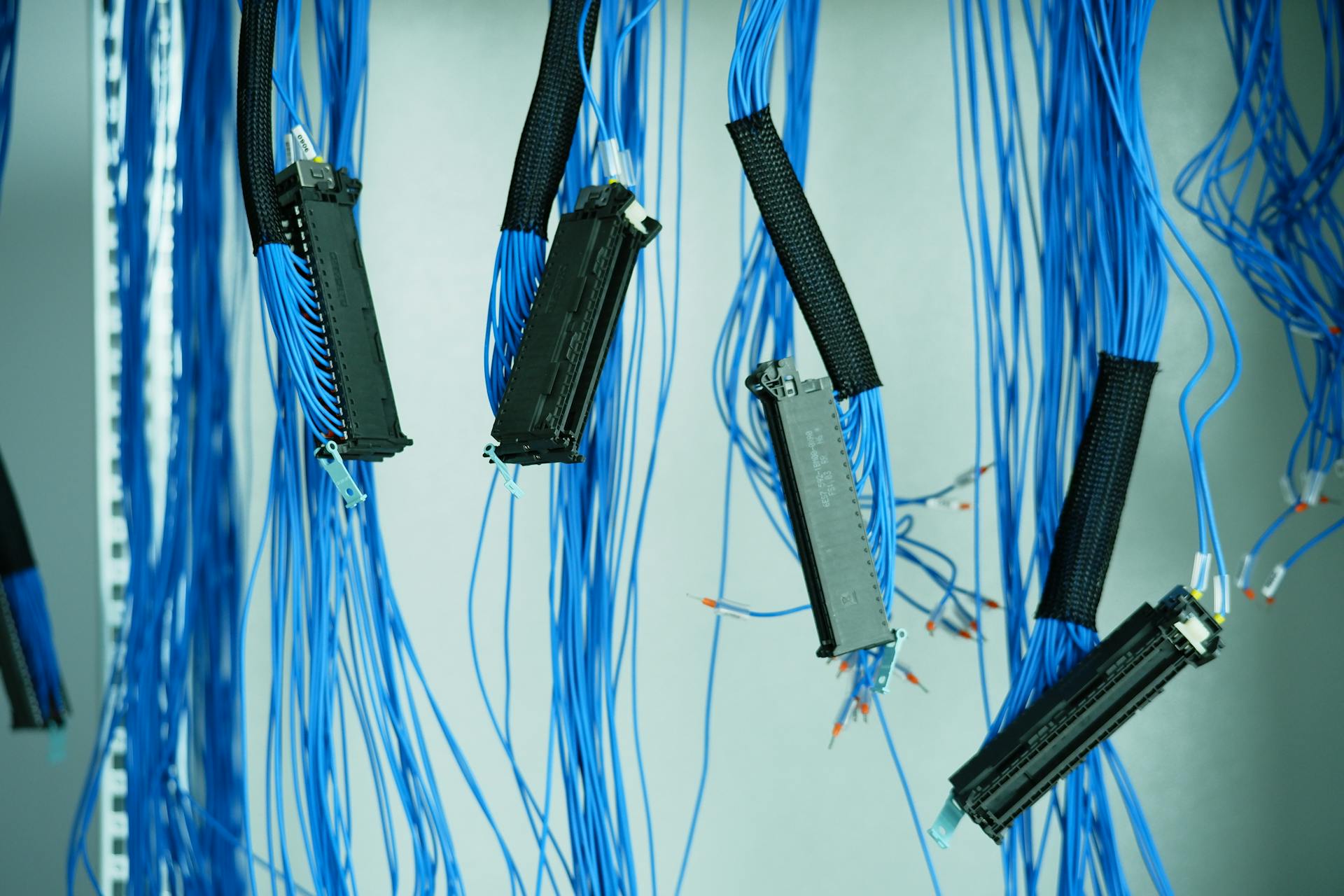
This method watches an Azure Storage Account Container for new blobs and automatically triggers execution of the Function App when new blobs are created within the Container.
The Azure Functions Binding definition within the function.json file sets up the Function to get triggered on new blobs being saved in the source Azure Storage Account.
The source_storage_connectionstring is the Application Setting on the Function App that contains the Azure Storage Account Connection String used to connect to and authenticate with the Azure Storage Account.
The path property defines the format for the Container (named containerName in this case) and the file name of the Blob (represented by {blobName}).
Custom Stream Provider
Creating a custom stream provider is a crucial step in triggering and customizing your Azure Storage experience. This involves extending the MultipartFormDataStreamProvider class.
You'll need to create your own AzureStorageMultipartFormDataStreamProvider, which will be responsible for writing streamed data directly to a blob in your container.
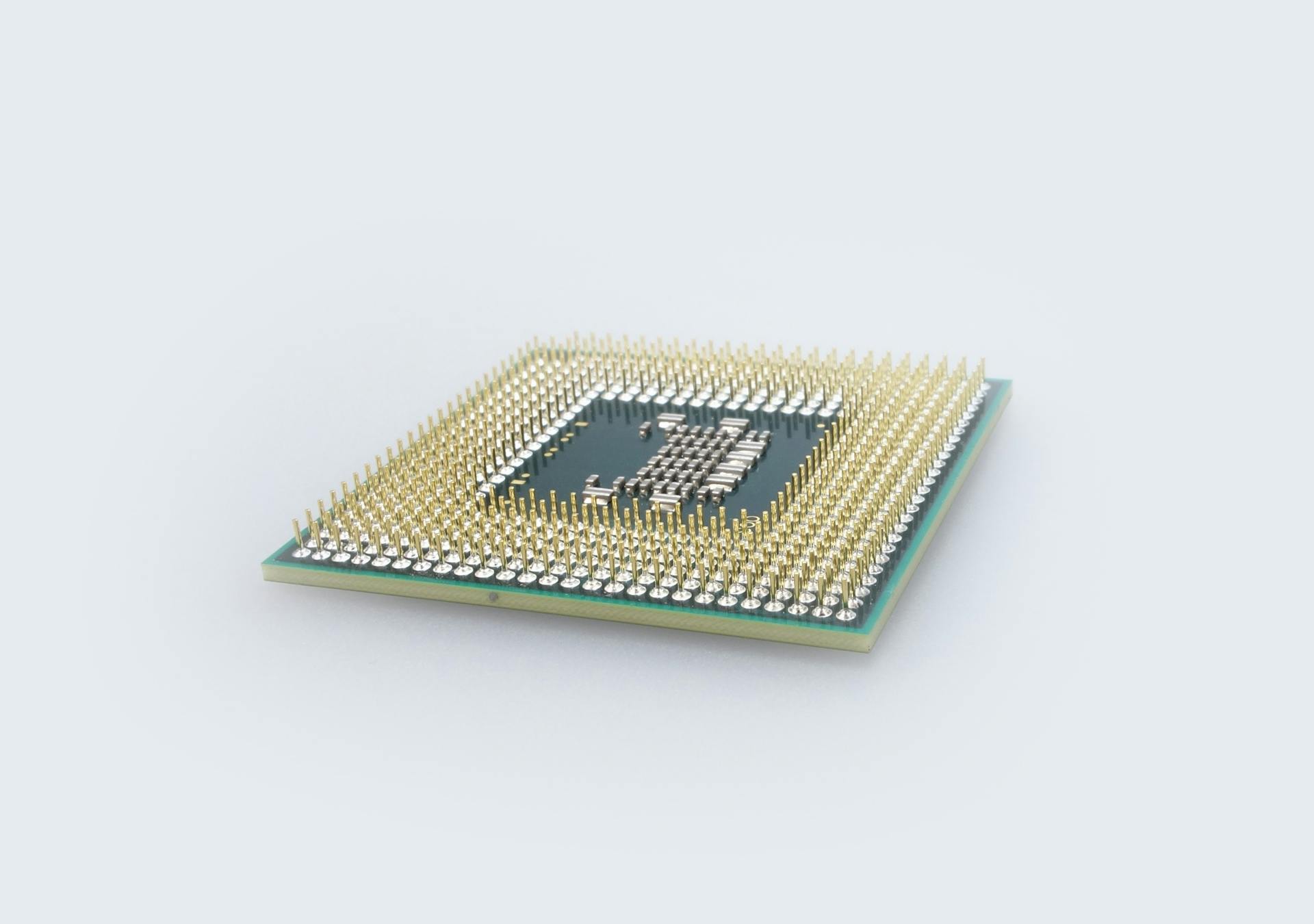
This new provider will handle the complexities of uploading data to Azure Storage, freeing you up to focus on other aspects of your application.
To write streamed data directly to a blob, you'll be using the AzureStorageMultipartFormDataStreamProvider class.
With this custom provider in place, you'll be able to take full control over how your data is stored and retrieved from Azure Storage.
Sources
- https://prashanth-kumar-ms.medium.com/azure-how-to-move-transfer-files-from-azure-files-shares-to-azure-blob-storage-4bdbc54df7fb
- https://build5nines.com/azure-functions-copy-blob-between-azure-storage-accounts-in-c/
- https://ppolyzos.com/2016/02/07/upload-a-file-to-azure-blob-storage-using-web-api/
- https://blog.matrixpost.net/accessing-azure-storage-account-blobs-from-c-applications/
- https://www.linkedin.com/pulse/how-move-azure-storage-blobs-between-containers-dhiraj-kumar
Featured Images: pexels.com