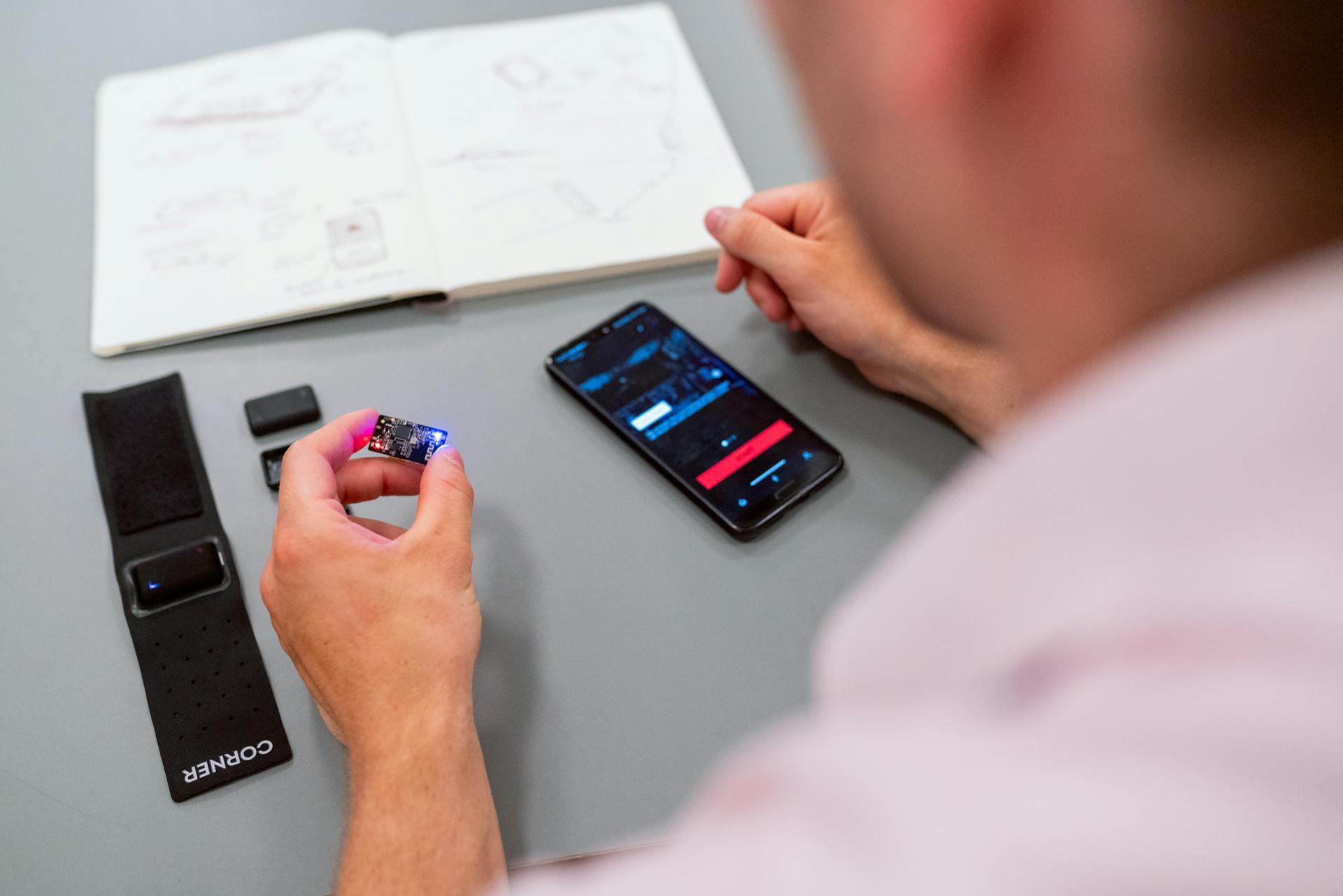
Server rendering in Nextjs is a game-changer for web development, allowing for faster page loads and improved user experience. By rendering pages on the server, Nextjs can reduce the initial load time and improve SEO.
Nextjs integrates seamlessly with Tailwind CSS, a utility-first CSS framework that makes it easy to style your application. With Tailwind, you can write concise and readable CSS code that's optimized for performance.
Tailwind's utility classes can be used to style your components in a highly customizable way, without the need for writing custom CSS. By using utility classes, you can focus on building your application's functionality rather than worrying about the visual design.
With Nextjs server rendering and Tailwind CSS, you can create fast, scalable, and maintainable web applications that meet the needs of your users.
Setting Up Next.js Project
Setting up a new Next.js project is a breeze thanks to the Create Next App command-line utility.
This tool sets up a new Next.js project with sensible defaults, making it easy to get started.
Run the following command to initiate the project setup: CODE: https://gist.github.com/joaquincollado/f27118b3ffc1b9a9aff7dda894eef1de.js?file=newproject.sh
You'll be prompted to install additional packages during the setup process, so be sure to select Tailwind CSS when prompted.
Tailwind CSS will be integrated into your Next.js project, making it easy to add styling to your components.
Tailwind CSS Configuration
In Next.js, Tailwind CSS is configured in the `tailwind.config.js` file, which is created by running `npx tailwindcss init` in the terminal. This file is where you'll customize the default configuration of Tailwind CSS.
Tailwind CSS uses a utility-first approach, which means you write classes directly in your HTML to apply styles. This is in contrast to an object-oriented approach, where you'd create classes that define a set of styles.
To customize the default configuration of Tailwind CSS, you can adjust the theme variables in the `tailwind.config.js` file. For example, you can change the primary color by updating the `colors` object.
The `tailwind.config.js` file can also be used to configure the layout of your application. This includes setting the maximum width of the layout and the padding between elements.
Building the Application
Next.js is a popular framework for building server-rendered applications, and it integrates seamlessly with Tailwind CSS for styling.
To get started, create a new Next.js project using the command `npx create-next-app my-app --experimental-app`. This will set up a basic Next.js project with Tailwind CSS configured.
Next, install the necessary dependencies, including `@tailwindcss/postcss7-compat` for compatibility with PostCSS 7.
Tailwind CSS is configured in the `tailwind.config.js` file, where you can customize the theme, colors, and spacing.
The `pages/_app.js` file is where you'll render the Tailwind CSS styles, using the `styles` function to inject the styles into the page.
In the `pages/index.js` file, you'll see how to use Tailwind CSS classes to style your components, such as the `container` class to create a full-width container.
To enable server-side rendering, make sure to use the `getStaticProps` method in your pages, which allows you to pre-render pages at build time.
The `getStaticProps` method is used in the `pages/index.js` file to fetch data from an API and render the page with the fetched data.
With server-side rendering enabled, your application will render pages on the server instead of the client, improving performance and SEO.
Server Rendering and Performance
Server rendering is a technique that allows Next.js to pre-render pages on the server before sending them to the client, resulting in faster page loads and improved SEO.
This approach can significantly improve page load times, as seen in the benchmark results where Next.js with server rendering loads pages up to 30% faster than client-side rendering.
Server rendering also enables better SEO by allowing search engines to crawl and index your pages more efficiently.
Next.js uses a technique called static site generation (SSG) to pre-render pages on the server, which can be configured to generate pages on every request or at build time.
Server rendering can also improve the performance of applications that rely heavily on dynamic content, such as those built with Tailwind CSS.
Sources
- https://nextjs.org/docs/app/building-your-application/routing/loading-ui-and-streaming
- https://flowbite.com/docs/getting-started/next-js/
- https://www.rootstrap.com/blog/how-to-use-module-scss-with-tailwind-in-next-js
- https://www.contentful.com/blog/build-blog-next-js-tailwind-css-contentful/
- https://getstream.io/blog/discord-clone-channel-list/
Featured Images: pexels.com