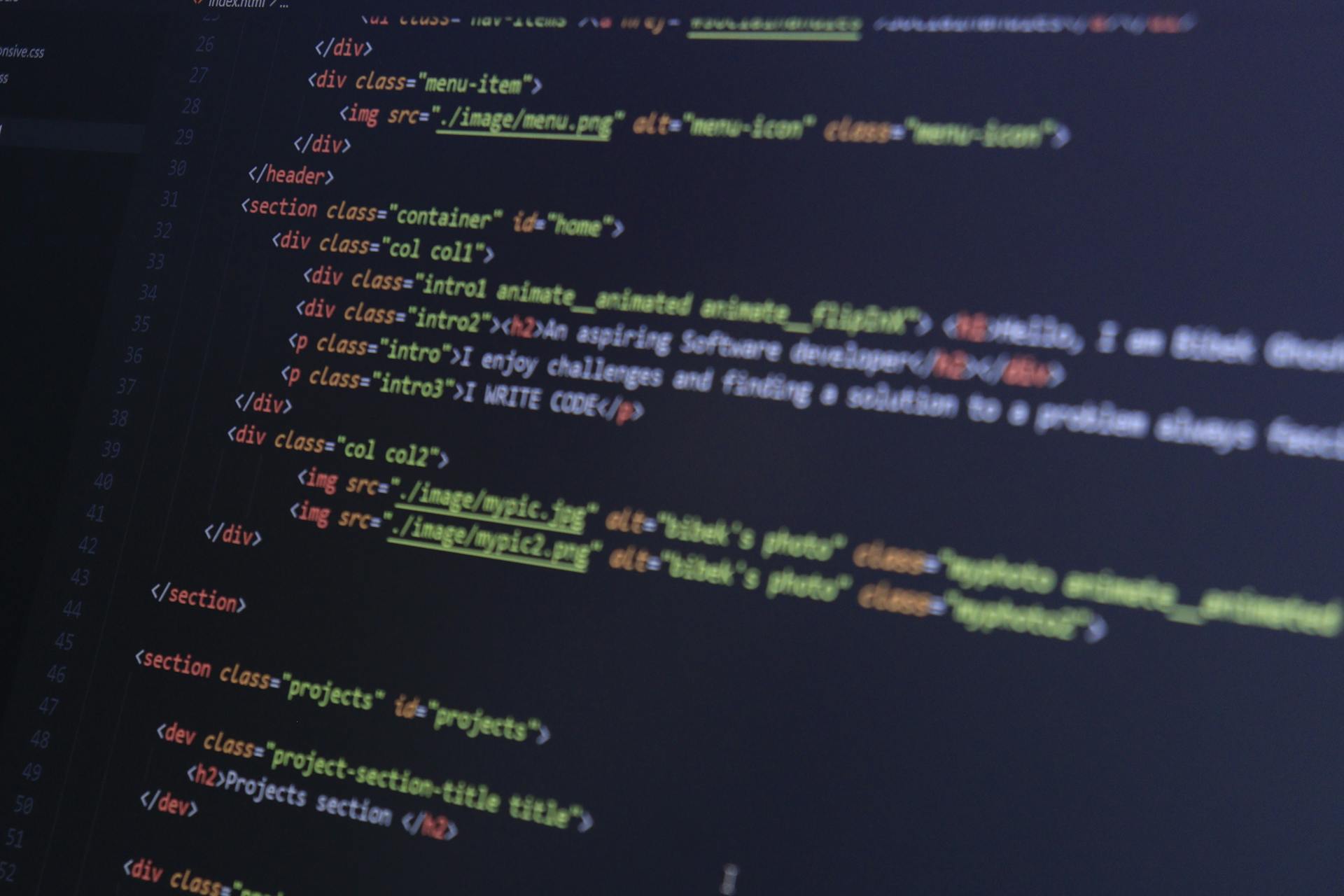
Setting up a new Next.js project with TypeScript can be a bit overwhelming, especially for beginners. You can create a new project using `npx create-next-app my-app --ts` to get started.
To enable TypeScript in your project, you'll need to install the necessary dependencies, including `@types/node` and `@types/react`. This can be done using npm or yarn.
One of the first things you'll want to do is set up a basic directory structure for your project. This can include a `src` directory for your main application code and a `public` directory for static assets.
In a typical Next.js project, you'll have an `index.tsx` file in the `src` directory that serves as the entry point for your application.
A fresh viewpoint: Nextjs Usecontext
Project Configuration
When creating a Next.js project with TypeScript, you'll want to configure your project settings. The buildTargetName option controls the name of Next.js' compilation task, which compiles the application for production deployment.
You can customize this name to your liking, but the default name is "build". This setting is crucial for production deployment, so make sure to update it if needed.
Broaden your view: How to Build Next Js App for Production
In development mode, the devTargetName option controls the name of Next.js' development serve task, which starts the application. The default name is "dev", but you can change it to suit your project's needs.
Here's a quick rundown of the options you can customize:
- buildTargetName: controls the compilation task name for production deployment
- devTargetName: controls the development serve task name
- startTargetName: controls the production serve task name (default is "start")
- serveStaticTargetName: controls the static export task name (default is "serve-static")
@nx/next Configuration
In Next.js projects, the @nx/next configuration is where you can fine-tune your application's build and serve processes.
The buildTargetName option is particularly useful, as it allows you to customize the name of Next.js' compilation task for production deployment. This is especially helpful when working on multiple projects simultaneously, as it makes it easier to identify which task belongs to which project.
The default name for the buildTargetName option is 'build', but you can change it to anything you like.
The devTargetName option, on the other hand, controls the name of Next.js' development serve task, which starts the application in development mode. This is super useful for developers who need to quickly test and iterate on their code.
A unique perspective: Next Js Development Company
The default name for the devTargetName option is 'dev', but you can customize it to suit your needs.
Here's a quick rundown of the options available for configuring the @nx/next build and serve tasks:
- buildTargetName: controls the name of Next.js' compilation task for production deployment
- devTargetName: controls the name of Next.js' development serve task
- startTargetName: controls the name of Next.js' production serve task
- serveStaticTargetName: controls the name of Next.js' static export task
By customizing these options, you can make your development workflow more efficient and enjoyable.
Using an Nx Library
Using an Nx Library is incredibly straightforward. You can import a library called my-new-lib in your application as follows.
There's no need to build the library prior to using it. The Next.js application will automatically pick up the changes when you update your library.
If you're working on a library intended to be built and published to a registry like npm, you can use the --publishable and --importPath options. This is done with the command nx g @nx/next:lib libs/my-new-lib --publishable --importPath=@happynrwl/ui-components.
You can also use create-next-app with the TypeScript flag to start from scratch.
If this caught your attention, see: Nx Nextjs
Starter Projects
To start a Next.js project with TypeScript, you can use the official TypeScript example app from the Next.js team, which is available at next.js/examples/blog-starter.
A different take: Next.js
This example app is a great place to start, but it may not cover all the libraries you need for a complete application. For a more comprehensive solution, you can explore community starters and frameworks that expand upon Next.js.
You can create a new Next.js project from scratch using create-next-app with the TypeScript flag. This will enable TypeScript for your project and get you started with a basic configuration.
If you want to kickstart your project even further, there are some great Next.js and TypeScript starter templates available that use the app router. Here are a few examples:
- vercel/ai-chatbot - an AI chatbot template from Vercel
- jpedroschmitz/typescript-nextjs-starter - a minimal starter that uses ESLint and Prettier
- nextjs-kickstart - a Next.js 14.0 app router boilerplate that includes ESLint, Tailwind, Testing Library, and more
- prismicio-community/nextjs-starter-prismic-minimal-ts - a minimal Prismic starter built with Next.js and TypeScript
Understanding Plugin
The Next.js TypeScript plugin is a game-changer for developers. It provides auto-completion and advanced type-checking, making it easier to catch errors and write clean code.
This plugin integrates seamlessly with code editors like VS Code, helping you stay on top of your project. It catches errors and warns against invalid segment config options, ensuring your code is robust and reliable.
Check this out: Nextjs Code Block
The TypeScript plugin offers intelligent suggestions and in-context documentation, helping you understand Next.js APIs and features like the "use client" directive. This feature provides details about Next.js APIs, making it easier to work with the framework.
The plugin also helps with future-proofing, as the Next.js team plans on releasing more features in the future. This means you can focus on building your project without worrying about compatibility issues down the line.
If this caught your attention, see: Nextjs 14 New Features
End-to-End Type Safety
End-to-end type safety is a game-changer for Next.js projects. It ensures that data types are consistent throughout a codebase, reducing the likelihood of bugs and making our code easier to maintain.
TypeScript's compiler can detect type-related issues, like assigning a value of the wrong type to a variable or passing incorrect arguments to a function, and provide early warnings. This helps us catch potential errors and bugs during development, leading to better code quality.
Next.js helps with end-to-end type safety in several ways. React Server Components eliminate the need for data serialization between server and client, ensuring consistency and preventing potential errors.
Broaden your view: Nextjs App Route Get Ssr Data
Here are some benefits of end-to-end type safety:
- Eliminates the need for data serialization between server and client
- Makes data flow between components cleaner and easier to type
By using end-to-end type safety, we can reduce the time spent debugging and make our code more maintainable. This is especially true when working with complex applications that involve multiple layers, such as frontend, API, and database.
Application Creation
To create a new Next.js project with TypeScript, you can use the command `nx g @nx/next:app apps/my-new-app`. This will create a new application.
You can also use the `create-next-app` command maintained by Next.js creators, which builds an application within seconds. The folder will be named after the application, and you can run the application by following the commands `cd` and `yarn dev`. This will take you to `localhost:3000` where you can see your new app.
There are also other options available, such as using `create-t3-app`, an interactive CLI tool that generates a boilerplate Next.js project configured with TypeScript and various other option libraries.
Readers also liked: Nextjs Cookies
Creating Applications
Creating applications with Next.js is a breeze. You can add a new application with the command `nx g @nx/next:app apps/my-new-app`.
The `create-next-app` command is a great way to create a simple Next.js application. It builds an application within seconds.
To run the application, navigate to the application folder and use the `yarn dev` command. You can then access your new app by visiting `localhost:3000`.
Changing the `.js` extensions to `.tsx` is a crucial step. This allows you to use TypeScript JSX, which is essential for this tutorial.
Take a look at this: Nextjs Project
Generating Pages and Components
Generating Pages and Components is a breeze with Nx, a powerful tool that helps you create and manage your application's structure. Nx provides commands to quickly generate new pages and components for your application.
You can use the nx g command to generate new pages and components. For example, you can run nx g @nx/next:page apps/my-new-app/pages/my-new-page to add a new page to your application. Nx also generates components with tests by default, which is a great way to ensure your code is robust and reliable.
Here's an interesting read: Nextjs App vs Pages
If you want to generate tests for a page as well, you can pass the --withTests option to the nx g command. For example, nx g @nx/next:page apps/my-new-app/pages/my-new-page --withTests will generate tests for the new page under the specs folder.
Here's a summary of how to generate pages and components with Nx:
Remember, Nx is a powerful tool that helps you create and manage your application's structure. By using its commands to generate pages and components, you can save time and focus on building your application.
Worth a look: Next Js Single Page Application
Project Migration
Migrating a Next.js project from JavaScript to TypeScript is a straightforward process. You'll need to create a `tsconfig.json` file, which will serve as the configuration file for your TypeScript project.
To start, you'll need to install TypeScript and its dependencies using the following commands: `npm install --save-dev typescript @types/node @types/react` or `yarn add typescript @types/node @types/react --dev`. This will ensure that you have the necessary tools to work with TypeScript.
Worth a look: Install Next Js 13
You'll also need to rename your JavaScript files to TypeScript files by changing the extension from `.js` to `.ts` and from `.jsx` to `.tsx`. This will enable TypeScript to work its magic and provide you with type safety and other benefits.
A new file called `next-env.d.ts` will be created, which serves as a declaration file containing type information. This file is used by TypeScript to provide type checking and other benefits.
You can also add type checking to your `next.config.js` file by using JSDoc, which will provide additional type safety and other benefits.
You might enjoy: Nextjs Server Actions File Upload
Migrating a Project to JavaScript
Migrating a Project to JavaScript can be a smooth process if you follow the right steps. First, you'll need to create a tsconfig.json file to get started.
This file is essential for configuring TypeScript, which will help you migrate your project from JavaScript. Next, install the TypeScript and type dependencies using a command like npm install --save-dev @types/node @types/react.
Intriguing read: Next Js Project Structure
Once you've installed the dependencies, it's time to change the file extensions from .js and .jsx to .ts and .tsx. This will allow your project to use TypeScript. You'll also notice a new file called next-env.d.ts being created.
This file serves a specific purpose: it contains type information for your project. TypeScript has two main types of files: .ts files, which contain executable code and types, and .d.ts files, which contain only type information.
To add type checking to your project, you can use JSDoc in your next.config.js file. This will allow you to get some type safety benefits, even though next.config.js must be a JavaScript file.
If you're having trouble getting Next.js getStaticProps working with TypeScript, you can check out the resources mentioned in the article, such as vitamindev.com or StackOverflow. The high-level conclusions include using the types provided by Next.js, such as NextPage, GetStaticProps, and GetStaticPaths, and adding additional type safety by providing generic types to these types.
See what others are reading: File Upload Next Js Supabase
Convert Your Application
Converting your application to TypeScript is a straightforward process that can be completed in a few steps. You'll need to create a tsconfig.json file and install the necessary dependencies, which can be done using the command `npm install --save-dev typescript @types/node @types/react` or `yarn add typescript @types/node @types/react --dev`.
To begin the migration process, you'll need to create a tsconfig.json file. This file will serve as the configuration for your TypeScript project. You can then install the TypeScript and type dependencies using the command `npm install --save-dev typescript @types/node @types/react` or `yarn add typescript @types/node @types/react --dev`.
You'll also need to update your file extensions from .js to .tsx. This means changing any files ending in .js and .jsx to .ts and .tsx, respectively. This will allow your application to recognize the new file types and enable TypeScript functionality.
Here's a step-by-step guide to help you convert your files:
Once you've updated your file extensions, you'll need to create a new file called Unauthorized.tsx. This file will serve as a starting point for your TypeScript application.
As you continue to work on your application, you'll find that TypeScript provides numerous benefits, including better introspection, intelligent autocompletion, and improved error handling. These benefits will help you catch bugs before they have a chance to run in the application and make coding more efficient.
Sources
- https://nx.dev/nx-api/next
- https://daily.dev/blog/a-comprehensive-guide-on-setting-up-next-js-with-typescript-and-tailwindcss
- https://vercel.com/guides/getting-started-with-nextjs-typescript-stripe
- https://developer.okta.com/blog/2020/11/13/nextjs-typescript
- https://prismic.io/blog/nextjs-typescript-tips-faqs
- https://prismic.io/blog/nextjs-typescript
Featured Images: pexels.com