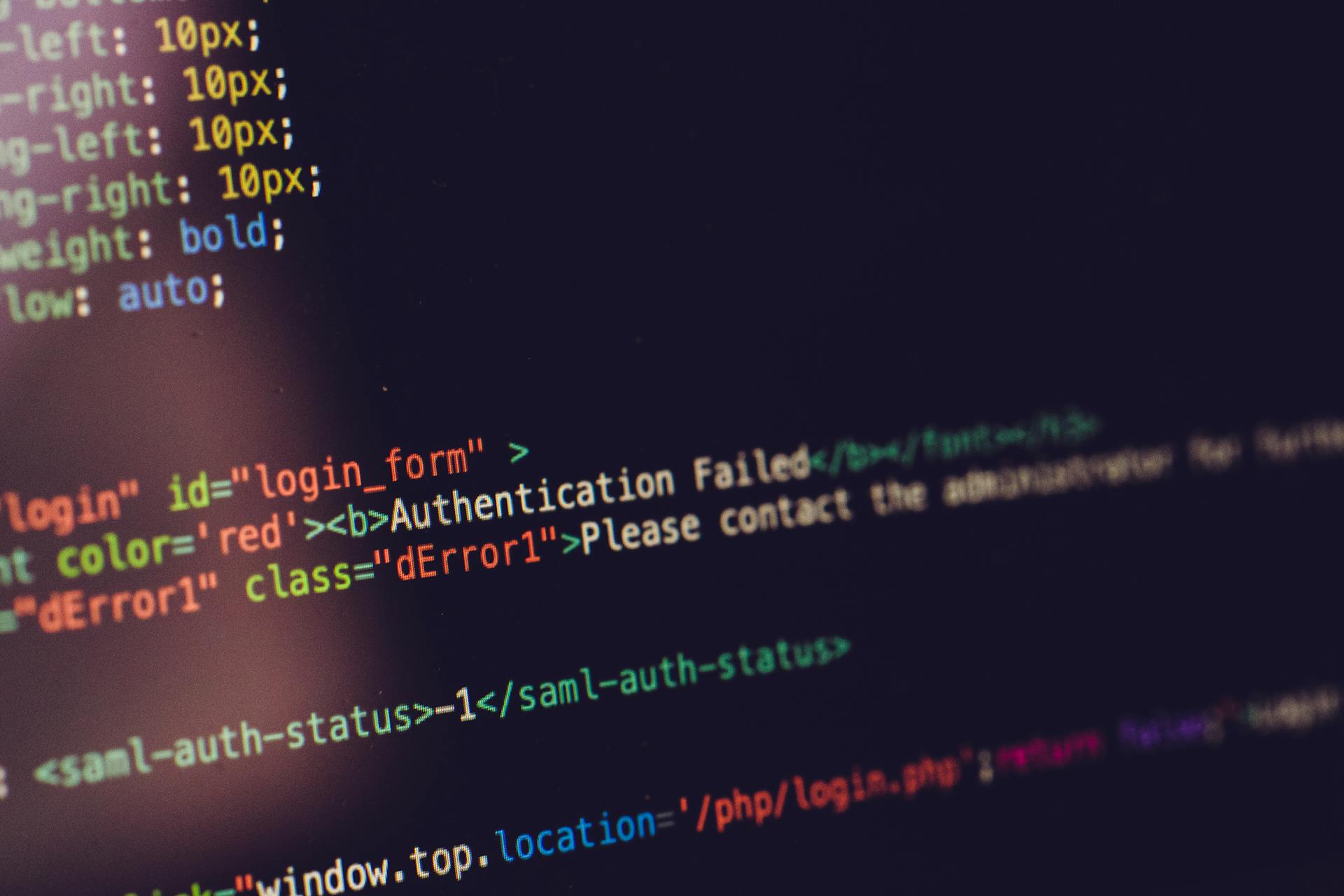
Developing a Next JS single page application requires careful planning and attention to detail.
To ensure a smooth development process, it's essential to set up a proper routing system, which can be achieved using the Next JS Link component. This allows for client-side routing, enabling a seamless user experience.
A well-structured routing system also helps with SEO, as it allows search engines to crawl and index individual pages. This is particularly important for large applications with many routes.
Next JS also provides built-in support for internationalization (i18n) and localization (L10n), making it easier to develop applications that cater to a global audience.
Setting Up an Application
To set up an application with Next.js, you can start by running `npx create-next-app my-app` in your terminal. This command will create a new Next.js app in a directory called my-app.
First, you need to install Next.js by running the following command: `npx create-next-app my-app`. This will create a new Next.js app in a directory called my-app.
You can start your development server with `npm run dev` or `yarn dev` after the installation is complete. Your Next.js application should now be up and running on http://localhost:3000.
Create a new file named index.js inside the pages directory. Create a new file named about.js in the pages directory.
Authentication and Authorization
You can add authentication and authorization to your Next.js app with the help of Ory Kratos. To get started, you can find a guide on GitHub that includes the source code for setting up authentication with Ory Kratos.
The guide uses the public playground deployment of Ory Kratos at https://playground.projects.oryapis.com by default. To use it, simply run a few commands to create a project and copy the SLUG to set the ORY_SDK_URL to the SDK URL of the project.
After setting up the project, you can see the app in action with login, registration, and user management at http://localhost:3000. The CLI displays the project details after it's created, which you can use to set up the Ory Kratos SDK in your Next.js app.
To add the Ory Kratos SDK to your Next.js app, you can use the package @ory/integrations, which takes care of all the heavy lifting for you. This package sets up the SDK so it connects with the Next.js Edge function you created earlier, making it easy to get started with authentication and authorization.
Routing and Navigation
Next.js routing is a built-in feature that allows you to create single-page applications that are fast, responsive, and easy to navigate.
You can manage page transitions, trigger animations or other effects, and create SEO-friendly URLs by leveraging Next.js routing.
Traditional routing is based on the file system, where each file inside the pages directory represents a route.
Dynamic routing allows you to create pages with variable parameters, enabling you to create pages that display different content based on the URL parameters.
To achieve dynamic routing, use square brackets in the file name, like [id].js, and then use the useRouter hook to access the dynamic parameters and fetch data based on them.
Nested routing enables you to create complex navigation structures by nesting routes inside each other.
You can create pages that are accessible through multiple URLs, and also have multiple pages inside a single parent page, using nested routing.
Custom routes allow you to define your own routing rules and patterns for your application.
You can create custom URLs that don’t follow the default routing rules in Next.js by defining a rewrites property in the next.config.js file.
To integrate React Router into your NEXT.js application, install the required packages and modify the _app.js file in the pages directory.
You can use the Link component from React Router to navigate between routes in your application.
To add client-side routing to your SPA, install the next/router package and use it to handle client-side navigation.
Single-page applications offer a fluid and immersive browsing experience, where users can access different parts of the application without the need for full page reloads.
Enhancing User Experience
Combining NEXT.js and React Router opens up a world of possibilities for building Single Page Applications (SPAs) with efficient routing and smooth transitions.
To build a user-friendly interface, keep user experience at the forefront of your design decisions. This will lead to a better overall experience for your users.
Adding smooth transitions between route changes can enhance the overall user experience.
Adding Transitions
Adding transitions is a great way to enhance the overall user experience. By installing the react-transition-group package, you can add smooth transitions between route changes.
This can be done by wrapping your route components in a CSSTransition component to apply transitions. You'll need to update the code in the index.js file to make this happen.
The key to smooth transitions is to use a package that can handle the animation for you. In this case, react-transition-group is a great choice.
Atomic Design
Atomic design is a powerful tool for building consistent and reusable design systems. It's especially useful in React, where componentization is the norm.
To get started with atomic design, create an index.js entry point for the components directory. This will help you dynamically export and import components, making it easier to move them around as needed.
The idea is to create a hierarchical structure of components, from simple atoms to more complex organisms. Don't worry too much about where to put each component at first – just create it and move it later if needed.
Here are some examples of simple components (atoms) you might create:
- IconButton
- AppBar
- Card
- CardActions
- CardContent
- List
- ListItem
- ListItemIcon
- ListItemText
- Loader
- MenuIcon
- SwipeableDrawer
- Toolbar
- Typography
- Grid
As you create more components, you can start to see how they fit together to form more complex organisms. This is where atomic design really starts to shine, making it easier to build consistent and maintainable design systems.
Integrate Material-UI
Integrating Material-UI is a crucial step in enhancing user experience.
Material-UI is an open source project that features React components implementing Google's Material Design.
To use Material-UI with the NextJS 6 framework, you need to install some additional packages.
You need to use the codebase from the official material-ui repo example with Next.js.
Material-UI is designed to provide a consistent and visually appealing design language for your application.
Deployment
Deployment is a breeze with Next.js.
You can deploy your app using platforms like Vercel, Netlify, or your preferred hosting service.
Vercel is a popular choice for Next.js apps, and it's easy to set up.
Just head to the Next.js deployment documentation for more details.
To deploy on Vercel, you'll need to configure your Ory Network Project SDK URL or the URL of your self-hosted Ory Kratos instance in your Vercel deployment.
This will allow your app to communicate with Ory Kratos.
To separate Ory Kratos deployments for staging, production, and development, use different SDK URLs for the different environments by un/selecting the checkboxes in the Vercel UI.
This is a great way to keep your environments organized.
If you want to call the Ory Network's Admin APIs from your Next.js Edge serverless functions, you can set up an Ory Personal Access Token.
This will give you the necessary credentials to make API calls.
Once you've set up your Vercel deployment, all you need to do is run the deploy command and connect it to the project you created.
This will deploy your app to Vercel and make it live.
Technology and Tools
For a Next.js single page application, we're using a technology stack that includes ReactJS for creating interactive UIs. This library is able to update and render components when data changes.
We're also utilizing GraphQL to work with APIs, which provides a comprehensive description of data in an API and makes it easier to get exactly the data we need in our requests. This is done with the help of Apollo-client, a tool that's easy to get started with and compatible with our JavaScript application.
Here are some of the other tools we're using: Babel for JavaScript compilation, ESLint for code quality checking, Jest + Enzyme for testing, and Yarn for package management. We're also using Storybook for UI component development and Material-UI for implementing Google Material Design.
Technology Stack
Our technology stack is a key part of what makes our application run smoothly. We use ReactJS to create interactive UIs, which can update and render components when data changes.
ReactJS is a declarative and component-based library that's perfect for building fast and efficient user interfaces. We also use GraphQL to work with APIs, which gives us a comprehensive description of the data and makes it easier to get exactly what we need.
Apollo-client is a great tool that we've adopted to make working with GraphQL even easier. It's incredibly compatible and flexible, and it's easy to get started with. NextJS 6 is a lightweight framework that helps us pre-render our ReactJS applications for better performance.
Babel is a JavaScript compiler that we need because of slow browser adoption of the latest JavaScript language features. It allows us to use all the newest JavaScript features even if they don't have browser support yet.
We use ESLint to check our code quality and find certain classes of bugs, such as those related to variable scope. Jest + Enzyme makes testing a much more pleasant process, with features like fast execution and helpful fail messages.
Yarn is our ultra-fast package manager, which we like for its speed, security, and reliability. We also use Storybook, a development environment for UI components, to browse component libraries and view the different states of each component.
Here's a list of our tech stack:
Configure Linters
Configuring linters is a crucial step in setting up your app for success. You'll want to integrate linters to avoid lots of refactoring in the future.
To start, add eslint as a development dependency. This will allow you to lint all valid Babel code. You'll also need to install a few dependencies, including Babel-eslint, which provides Airbnb's .eslintrc as an extensible shared config.
Choose the Airbnb eslint config, as it's a popular style guide that's widely accepted. You'll also want to choose the JSON format for your config file. Install the ESLint plugin with rules that help validate proper imports.
Select React as your framework, since you're working with JavaScript code. You can choose to install the dependencies now with npm, but it's not necessary. Now you should have an .eslintrc.json file with the configuration set up.
To fine-tune your config, you'll want to add an option to use .js extensions instead of .jsx. This is because JSX isn't standard JavaScript, and you want to ensure your code is compatible with all browsers.
Frequently Asked Questions
Can Next.js be used for spa?
Yes, Next.js can be used to build a Single-Page Application (SPA) with dynamic routing and client-side rendering. Next.js enables you to start with a static site and later upgrade to a SPA with server-side features.
Is Next.js spa or MPA?
Next.js is a hybrid framework that behaves as a Single-Page Application (SPA) after the initial server-rendered page load, but with server-side rendering for the first page load. This unique approach combines the benefits of both SPA and Multi-Page Application (MPA) architectures.
Sources
- https://www.ory.sh/login-spa-react-nextjs-authentication-example-api-open-source/
- https://dev.to/sarveshh/nextjs-routing-a-comprehensive-guide-to-building-single-page-applications-3l90
- https://clouddevs.com/next/building-a-single-page-application/
- https://techstaunch.com/blogs/building-spa-using-next-js
- https://rubygarage.org/blog/spa-tutorial-on-reactjs
Featured Images: pexels.com