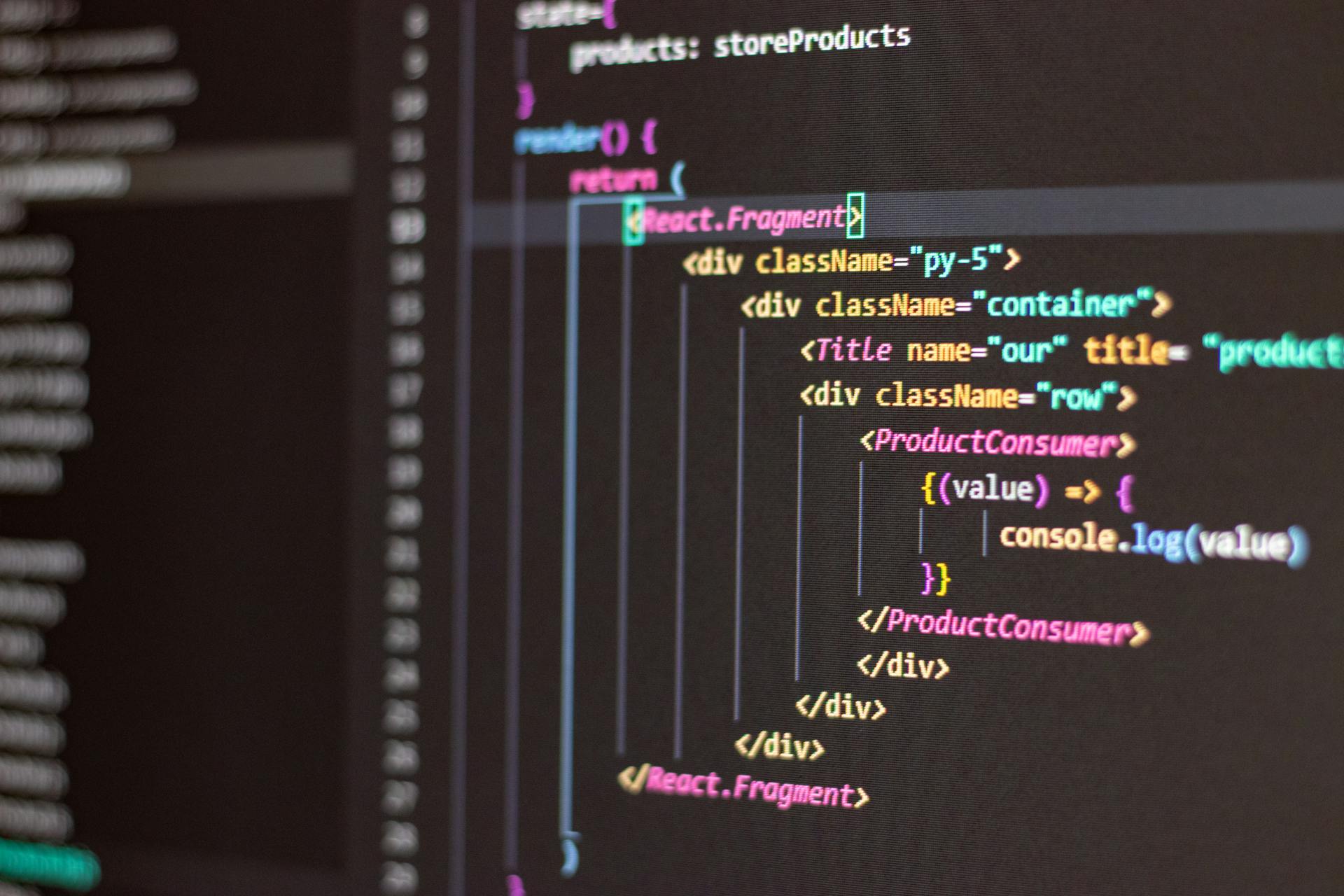
Creating a Next.js app from scratch is a straightforward process that requires a few key steps. You'll need to install Node.js and npm first.
Next, you'll need to create a new project using the `create-next-app` command, which will set up the basic directory structure for your app. This command will also install the necessary dependencies and create a `package.json` file.
With the basic setup complete, you can start building your app by creating a new page component. Next.js uses a file-based routing system, so you can simply create a new file in the `pages` directory to create a new page. For example, creating a new file called `[slug].js` will create a new page that can be accessed at the URL `/slug`.
Consider reading: Next Js Cookies
Getting Started
To create a Next.js app, you'll need to install the Next.js package using npm or yarn, which can be done by running the command "npx create-next-app my-app" in your terminal.
Next.js is built on top of React, so if you're already familiar with React, you'll feel right at home.
The project structure for a Next.js app is similar to a standard React app, with a few key differences, such as the presence of a pages directory for routing.
Take a look at this: Next Js React Fundamentals
Configure Your Environment
To configure your environment, you need your API token to be able to run models. Create a file called .env.local in the root of your project.
The npx create-next-app command you ran earlier created a .gitignore file that ignores .env.local files, which is a good thing because you don't want to accidentally commit your API token to your project's source code repository.
You can set your API token as an environment variable in your local development environment by adding it to the .env.local file. Next.js has built-in support for loading environment variables from this file into process.env.
Readers also liked: Next Js Project Structure
A New Application
First, let's talk about setting up a new application. This is where you'll create a new project in your preferred coding language, such as Python or JavaScript.
The next step is to choose a project structure, which will help you organize your code and make it easier to maintain in the long run. As we discussed earlier, this is crucial for large projects.
Additional reading: New Nextjs Project Typescript
For example, if you're building a web application, you'll want to set up a directory for your front-end and back-end code. This will keep your code organized and make it easier to find what you need.
Remember to also set up a virtual environment for your project, which will help you manage dependencies and keep your code isolated from other projects.
Curious to learn more? Check out: Nextjs Code Block
Building the App
You'll need to create the frontend code that renders a form, which will post data to the server-side endpoint you created in Step 4.
The frontend code should render a form that allows users to enter a prompt and submit it to the server-side endpoint.
You'll replace the existing content in the app/page.js file with new code, specifically the one mentioned in Step 5.
Explore further: Nextjs Form Component
Build the Backend
Now that we have our frontend up and running, it's time to build the backend. In Next.js, you can write your backend code in the same project as your frontend code.
On a similar theme: Is Nextjs Backend
Any code in a page.js file is treated as a frontend component, and any code in a route.js file is treated as a backend API endpoint. You'll create two server-side endpoints: one for running the model and one for polling the status of that request until it's complete.
Create a directory for these endpoints, and then create a file to handle prediction creation requests. Call it app/api/predictions/route.js and add the code that's provided. This file will handle requests to create a new prediction.
Create another file to handle requests to poll for the prediction's status. Call it app/api/predictions/[id]/route.js and add the code that's provided. Note the [id] in the directory structure, which is used for dynamic routing. This allows you to access the id part of the URL as a variable in your code.
Curious to learn more? Check out: How to Add Img to Nextjs
Build the Frontend
Building the Frontend is a crucial step in creating a seamless user experience.
You've already written the server-side code that talks to Replicate, so now it's time to focus on the frontend. The frontend code will render a form where users can enter a prompt and submit it to the server-side endpoint.
Suggestion: Next Js Client Side Rendering
Remove all the existing content in the app/page.js file and replace it with the new code. This file is used to render the default home route in Next.js.
The new code will post the form data to the server-side endpoint, which will run the model with Replicate and return a prediction.
Expand your knowledge: File Upload Next Js Supabase
Add Basic Styles
As you start building your app, it's essential to establish a solid foundation for your styles. Remove all the content in app/globals.css.
The Next.js starter app includes some CSS styles that are used on the default splash page, but they aren't really intended to be reused for a real app. This means you need to start from scratch.
To create a clean slate for your styles, replace the existing content in app/globals.css with the following basic styles:
These styles will provide a good starting point for your app's visual identity.
Discover more: Apply Css Nextjs
Bleeding-Edge React Frameworks
The Next.js team has agreed to collaborate with the React team in researching and developing framework-agnostic bleeding-edge React features.
For your interest: Create React App vs Next Js
Integrating React more closely with frameworks, specifically with routing, bundling, and server technologies, is a key opportunity to help React users build better apps.
These bleeding-edge React features are getting closer to being production-ready every day.
We're in talks with other bundler and framework developers about integrating these features, with the goal of having full support in all frameworks listed on the page within a year or two.
If you're a framework author interested in partnering with the React team to experiment with these features, please let them know!
You might like: React and Next Js
React
React is a powerful tool for building apps, and understanding its basics is crucial for success. Next.js is a popular framework built on top of React that makes it easy to create server-side rendered and statically generated websites and applications.
You can use Next.js to create a frontend that talks to a server-side endpoint, which runs a model with Replicate and returns a prediction. This is done by creating a new file, app/page.js, and adding code to it.
Next.js is maintained by Vercel, and you can deploy a Next.js app to any Node.js or serverless hosting, or to your own server. This means you have flexibility in choosing how to host your app.
The Next.js team is collaborating with the React team to research and develop new features, such as React Server Components. These features are getting closer to being production-ready and will likely be supported by many frameworks in the future.
Explore further: Nextjs Team
Multi-Tenant App
Building a multi-tenant app with custom domains is a great way to offer flexibility to your users.
You can use Next.js to build a multi-tenant app, which means you can create a single app that serves multiple clients, each with their own custom domain.
To achieve this, you'll need to configure Next.js to handle custom domains. This involves setting up a wildcard DNS record and configuring your Next.js app to use it.
This approach allows you to serve multiple clients from a single app instance, reducing costs and increasing efficiency.
By using Next.js, you can create a scalable and flexible multi-tenant app that meets the needs of your clients.
Explore further: Nextjs Multi Tenant App
Frequently Asked Questions
What is next JS app?
A Next.js app is a web application built using the Next.js framework, which combines React with server-side rendering and static website generation capabilities. It's a powerful tool for creating fast, scalable, and SEO-friendly web applications.
Is Next.js backend or frontend?
Next.js is a frontend framework that integrates with Node.js to provide a strong backend capability. It's a unique blend of frontend and backend features, making it a popular choice for full-stack development.
Is Next.js good for apps?
Yes, Next.js is ideal for building high-performance apps, thanks to its built-in optimization tools and streamlined development process. With Next.js, you can focus on core app functionality and enjoy a significant performance boost.
Sources
- https://replicate.com/docs/guides/nextjs
- https://react.dev/learn/start-a-new-react-project
- https://vercel.com/docs/frameworks/nextjs
- https://www.digitalocean.com/community/developer-center/deploying-a-next-js-application-on-a-digitalocean-droplet
- https://nextjs.org/docs/pages/getting-started/installation
Featured Images: pexels.com