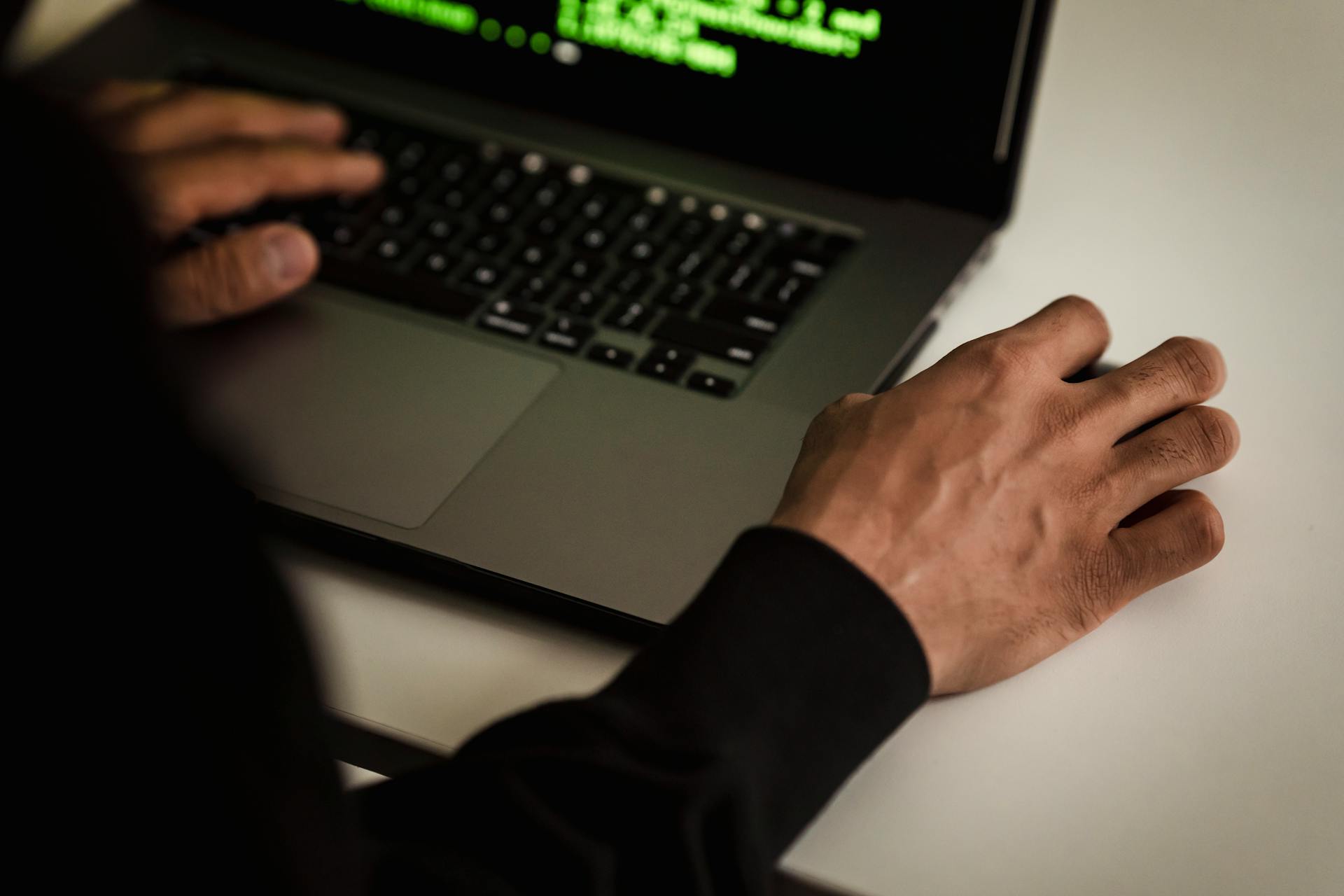
Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications. It's known for its flexibility and scalability.
The Next.js file structure is organized into several key directories, including pages, components, and public. The pages directory is where you'll find your route components, which are essentially functions that return JSX elements.
The components directory is where you'll find reusable UI components, such as headers, footers, and navigation menus. These components can be easily imported and used throughout your application.
Next.js projects are initialized using the create-next-app command, which sets up a basic file structure and configuration for you. This includes the creation of a pages directory, a public directory, and a few other essential files.
Readers also liked: Nextjs Pages
Project Structure
A well-structured Next.js project is essential for smooth development and long-term maintenance. A clear project structure helps developers work together smoothly and allows the application to expand without getting confusing.
Organizing files by what they do and putting them in the right folders makes everything easier to understand and maintain. For example, if you have components that you'll use in different parts of your app, keep them in the components folder.
Here are some common customizable folders and files that developers often add to their Next.js projects:
- components
- layouts
- lib
- services
- utils
- assets
- hooks
These folders help keep the project structure clean and well-maintained by separating the source files into different directories.
Enhanced
As you start building your Next.js project, it's essential to have a solid understanding of how to structure it for optimal performance and maintainability. A well-organized project structure can make a huge difference in the long run.
Here are some key takeaways to keep in mind: you can create a more manageable code structure by creating folders like components, layouts, lib, services, utils, assets, and hooks. This will help you keep your code organized and easy to navigate.
A unique perspective: Nextjs Code Block
To keep your structure scalable and maintainable, modularize your features into smaller, reusable modules, and avoid deep nesting by keeping the folder structure as flat as possible. Using index files within folders to re-export components can also simplify imports.
Here are some common customizable folders and files that developers often add to their Next.js projects, including the src directory, which keeps the project structure clean and well-maintained by separating source files into a different directory.
A clear project structure in Next.js is key for smooth development. Organize files by what they do and put them in the right folders, making it easier to understand and maintain. Using consistent names for files and folders makes it simpler to check if everything's there and working right.
Here are some common approaches to organizing your files and folders: grouping by feature and grouping by file type. Grouping by feature involves organizing files based on the feature they contribute to, while grouping by file type involves organizing files by their type, such as all React components in a components folder.
Expand your knowledge: Using State in Next Js
Environment Variables
You can store sensitive information like API keys or database passwords in environment variables, which are excluded from version control.
Environment variables are loaded at build time in Next.js, making them available in your app through process.env.
Create a .env.local file at the root of your project to define these variables.
You might enjoy: Nextjs Build Fails Environment Variables
File System and Routing
Next.js uses the file system to map files in the pages directory to the routing system, allowing you to create new routes with a straightforward and intuitive approach.
A file named about.js, for example, would automatically be accessible at the /about route. This convention provides a lot of flexibility in creating new routes.
The pages directory is special in Next.js, and each file in this directory corresponds to a route in your application. For instance, pages/index.js maps to the home page (/), and pages/about.js maps to /about.
Each .js or .tsx file within the pages directory corresponds to a route in your app, with the file name dictating the route's URL path.
Check this out: New Nextjs Project Typescript
Directory Naming Conventions
Directory naming conventions are crucial for readability and maintainability. Consistent naming conventions help developers quickly understand the purpose of a file or directory.
Use descriptive names that clearly describe the file or directory's purpose. This makes it easier for others to understand the code and reduces confusion.
Kebab-case is recommended for folder names, such as "user-profile". This is URL-friendly and commonly used in Next.js projects.
CamelCase is recommended for React component files, such as "UserProfile.js". This matches the component name and makes it easier to identify the file.
Lowercase is recommended for utility files and directories, such as "utils" or "fetch-data.js". This helps to keep the code organized and easy to find.
Here are some examples of directory naming conventions in a table:
Lib
In the context of a typical application, the lib folder is a crucial part of the file system, containing utility functions and helper classes used across the application.
These utilities can include custom hooks, data manipulation functions, or even third-party libraries that are used globally.
For another approach, see: Nextjs Spa
The lib folder is not just a collection of random functions and classes, but a carefully curated set of tools that help streamline the development process.
By keeping these utilities in a centralized location, developers can easily access and reuse them throughout the application.
This approach also promotes code reuse and reduces duplication, making maintenance and updates much more efficient.
Assets:
The assets folder is a crucial part of your application's file system, storing static assets like images, fonts, and icons that are referenced in the code and displayed to users.
These assets are typically stored in a separate folder to keep them organized and easily accessible.
Static assets can include anything from logo images to font files, and are used to enhance the visual appeal of your application's user interface.
In your code, you can reference these assets by their file path, allowing you to display them to users as needed.
For example, if you have an image file named "logo.png" stored in the assets folder, you can reference it in your code with a path like "/assets/logo.png".
For your interest: What Is .next Folder in Next Js
File System for Routing
Next.js uses the file system to map files in the pages directory to the routing system. This convention provides a straightforward and intuitive approach to creating new routes.
A file named about.js would automatically be accessible at the /about route, making it easy to create new routes without writing complex code.
The pages directory is special in Next.js, where each file corresponds to a route in your application. For instance, pages/index.js maps to the home page (/), and pages/about.js maps to /about.
This approach allows you to create new routes by simply creating new files in the pages directory, without having to write custom routing code.
The file name dictates the route's URL path, making it easy to understand and manage your routes. For example, a file named about.js would map to the /about route, while a file named index.js would map to the root URL (/).
If this caught your attention, see: Next Js Cookies
Custom Middleware Middleware
Custom middleware in Next.js allows you to execute code before a request is completed. You can organize your middleware functions in a middleware directory to run on the server side, client side, or both.
See what others are reading: Next Js Middlewares
This middleware function can be used to check authentication before a page or API route is served. By using custom middleware, you can add an extra layer of security to your application.
Custom middleware in Next.js is optional, but it can be incredibly useful for handling tasks such as authentication and authorization.
Worth a look: Next Js Custom Server
Frequently Asked Questions
Is Next.js app directory stable?
Yes, the app directory in Next.js is stable as of version 13.4, providing a recommended approach for structuring applications.
Should Next.js use src directory or not?
While not required, using a src directory in Next.js is highly recommended for a well-organized project structure that enhances readability, scalability, and maintainability. It's a best practice to consider for your Next.js project.
Where is the Next.js build folder?
By default, the Next.js build folder is located in the ".next" directory within your project folder. Learn more about customizing your build folder and app configuration in our article.
Sources
- https://medium.com/@mertenercan/nextjs-13-folder-structure-c3453d780366
- https://www.geeksforgeeks.org/nextjs-14-folder-structure/
- https://www.dhiwise.com/post/nextjs-folder-structure-simplified-a-comprehensive-overview
- https://www.getfishtank.com/insights/structuring-a-nextjs-project-in-sitecore-xm-cloud
- https://www.geeksforgeeks.org/next-js-src-directory/
Featured Images: pexels.com