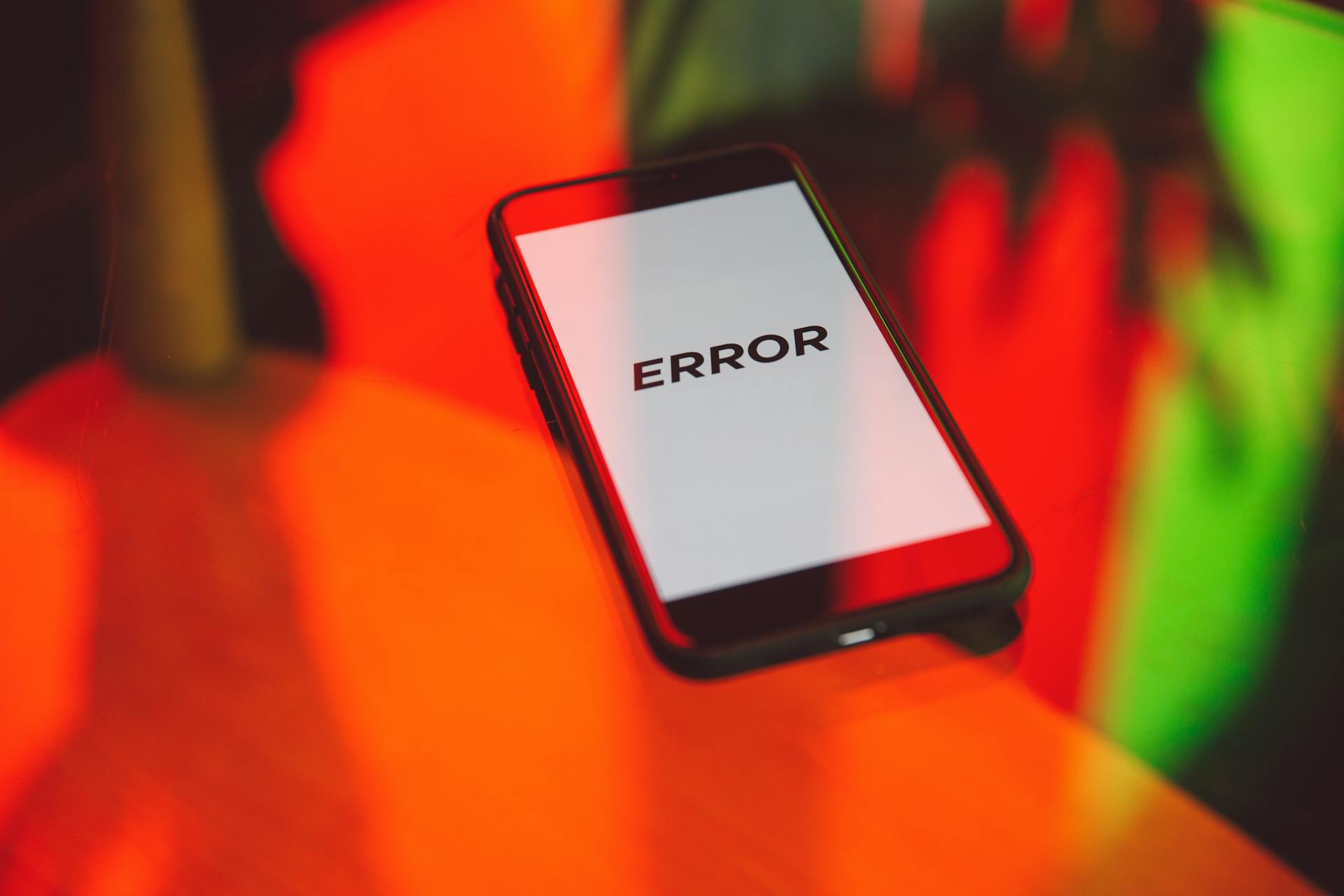
Next.js build fails can be frustrating, especially when environment variables are involved. Environment variables are used to store sensitive information, such as API keys or database credentials, that shouldn't be committed to your repository.
In Next.js, environment variables are typically stored in a .env file in the root of your project. This file contains key-value pairs, where the key is the variable name and the value is the variable value. For example, `API_KEY=1234567890`.
However, if your .env file is not properly configured, it can cause Next.js build fails. For instance, if you have a .env file with a variable named `NODE_ENV` set to `production`, but your Next.js project is trying to use a different value for `NODE_ENV`, it can lead to a build fail.
Take a look at this: Nextjs Server Actions File Upload
Understanding Next.js Build Fails
Next.js build fails can be frustrating, especially when they're caused by environment variables. Most often, this issue arises when you're trying to use environment variables in your Next.js project, but they're not properly configured.
One common cause of Next.js build fails due to environment variables is when you forget to add them to your .env file. This file is where you store sensitive information like API keys and database credentials. Forgetting to include these variables in the file can cause your build to fail.
To avoid this issue, make sure to add all your environment variables to the .env file, and don't forget to include the NEXT_PUBLIC_ prefix for variables that need to be exposed to the client-side.
Take a look at this: Next Js Project Structure
Handles
Handles are a crucial aspect of Next.js build fails. Next.js automatically loads environment variables from .env files into process.env. It supports multiple .env files for different environments, such as .env.local, .env.development, and .env.production.
This built-in support simplifies the process of managing environment variables across various environments. You can specify default configurations for application environments using .env files.
Next.js determines which environment variable values to use based on their definition order, known as the 'environment variable load order'. This sequence ensures that Next.js stops searching once the variable is found, impacting which value is ultimately used.
Here's a breakdown of the environment variable load order:
This load order is important to understand, as it can affect how your application behaves in different environments. For example, if you have a variable defined in .env.local, it will override any variable with the same name in .env.
Custom Server Configuration
Custom Server Configuration can be achieved through environment variables in Next.js applications. This allows teams to easily switch between different server environments without modifying the code.
Environment variables can be used to configure custom servers, making it possible to handle API requests in a specific way. By setting environment variables for the server configuration, teams can adapt to different requirements.
In a next.config.js file, environment variables can be set to configure a custom server. This is a straightforward process that enables flexibility in server setup.
Custom server configuration through environment variables promotes collaboration among team members. By using environment variables, team members can work on different aspects of the project without affecting the server configuration.
For your interest: Nextjs Team
Environment Variables in Next.js
Environment variables are a crucial part of Next.js development, and understanding how they work can save you a lot of headaches.
In Next.js, environment variables are used to store sensitive information like database passwords or secret keys, and are usually stored in files like .env.local or .env.development. Next.js follows a specific order when looking for environment variables, which is: process.env, env.${NODE_ENV}.local, env.local (only checked when NODE_ENV is not test), env.${NODE_ENV}, and finally .env.
Here are some key points to keep in mind when working with environment variables in Next.js:
- Always separate sensitive and non-sensitive variables.
- Avoid hardcoding sensitive information and store it as environment variables instead.
- Use environment files like .env.local or .env.development to store environment variables.
- Add environment files to .gitignore to prevent sensitive information from being committed to version control.
- Set default values for environment variables in case they are not defined.
- Never expose sensitive data to the client-side and use the NEXT_PUBLIC_ prefix for variables that are safe to be exposed.
Here are the steps to follow when accessing environment variables in Next.js:
- Use process.env to access environment variables on the server-side.
- Use the NEXT_PUBLIC_ prefix to expose environment variables to the client-side.
- Ensure that sensitive information is kept secure and only exposed to the browser when necessary.
- Use default values as a fallback in your code to handle missing or undefined environment variables.
By following these best practices and guidelines, you can ensure that your environment variables are used securely and efficiently in your Next.js application.
Here is a summary of the environment variable lookup order in Next.js:
- process.env
- env.${NODE_ENV}.local
- env.local (only checked when NODE_ENV is not test)
- env.${NODE_ENV}
- .env
Web Application Use Cases
In Next.js, environment variables are a powerful tool for managing different configurations across various environments. This is especially useful when it comes to configuring database connections.
You can specify different database URLs or credentials for your local development, test environment, and production environments using environment variables. This ensures that the right database is accessed depending on the runtime environment, which is critical for both functionality and security.
API keys should be stored as environment variables to keep them secure and out of your codebase. Storing API keys in this way is particularly important for services like Google Analytics or third-party APIs, where exposing these keys publicly could lead to unauthorized access or misuse.
Feature flags can be controlled dynamically using environment variables, allowing you to enable or disable features in different environments. For example, a feature flag can be enabled in a development environment for testing while being disabled in production until it's ready to be launched.
Here are some common use cases for environment variables in Next.js:
- Configuring Database Connections
- API Keys Management
- Feature Flags and Service Endpoints
Dynamic configuration is another powerful use case for environment variables in Next.js. You can adjust application behavior based on the environment without needing code changes, such as turning debugging on or off, modifying log levels, or changing the resource allocation.
Accessing in Your App
In Next.js, you can access environment variables in two main ways: server-side and client-side.
On the server-side, all environment variables declared in your .env files are available through process.env. This means you can use both public and private variables as needed for operations like fetching data, connecting to databases, or calling external APIs.
You can access environment variables on the client-side, but only variables prefixed with NEXT_PUBLIC_ are exposed to the browser. This ensures that sensitive information is kept secure and only information that needs to be publicly accessible is exposed.
Here's a key point to remember: environment variables are embedded during the build time, so changes to environment variables after the build won't affect the already statically generated pages.
Next.js also allows you to reference or compose other environment variables using the ${VARIABLE_NAME} syntax. This can help keep your environment variable files organized and avoid repetition.
To ensure consistency in your environment variables across all environments, use default values as a fallback in your code to handle missing or undefined environment variables gracefully.
In summary, understanding how to access environment variables in your Next.js app is crucial for secure and efficient use of environment variables in your application.
For your interest: Nextjs Loading Component
Naming Conventions
Naming Conventions for Environment Variables in Next.js are crucial to maintain security and functionality. Environment variables in Next.js must be prefixed with NEXT_PUBLIC_ to be accessed from the client-side code.
To ensure consistency, use UPPER_CASE and underscores to name your environment variables. This makes them easily identifiable in your code and separates them from regular variables. It's essential to follow a consistent naming convention to avoid confusion and make it easier to manage your variables.
Here are some best practices for naming environment variables in Next.js:
Consistent naming conventions also help increase code readability and maintainability. By following these best practices, you can ensure that your environment variables are easily identifiable and manageable in your Next.js application.
Setting Up Environment Variables
To set up environment variables in your Next.js application, follow these steps: Create environment files in your project's root directory. Next.js supports the following files: .env.local, .env.development, .env.test, and .env.production.
These files help you manage settings across multiple environments, crucial for maintaining the reliability and stability of your applications. Utilize .env.local for your local development overrides, .env.development for development settings, .env.test for test configurations, and .env.production for production settings.
Consider reading: How to Build Next Js App for Production
You can set default values for your environment variables in case they are not set in the environment files. This ensures that your application can still function even if the variables are not defined.
To set environment variables for your Next.js application, you'll need to create these files in your project's root directory, and then configure them according to your needs.
Here's a quick rundown of the supported files:
- .env.local: Used for local development settings
- .env.development: Specifically for development environment settings
- .env.test: For test configurations
- .env.production: Contains variables for the production environment
Managing Environment Variables
Next.js follows a specific order when looking for environment variables, which is: process.env, env.${NODE_ENV}.local, env.local, env.${NODE_ENV}, and .env. If the same variable is declared in multiple files, the one declared in the lowest file in this list wins.
To ensure secure management of environment variables, it's essential to separate sensitive and non-sensitive variables, avoid hardcoding sensitive information, and use environment files. You should also add these files to your .gitignore to prevent sensitive information from being committed to version control.
Here's a checklist for secure environment variable management:
- Never expose sensitive data, especially database passwords or secret keys, in environment variables accessible on the client-side. Use the NEXT_PUBLIC_ prefix for variables that are safe to be exposed.
- Do not commit your .env files to version control, especially if they contain sensitive information. Instead, add them to your .gitignore file and create a .env.example file with dummy values as a reference for other developers.
- Set default values for your environment variables in your code in case they are not set in the environment files.
- Use runtime configuration for variables that need to change at runtime (after the build phase).
- Avoid hardcoded values in your application that can be better managed through environment variables.
By following these guidelines, you can ensure that your environment variables are used securely and efficiently in your Next.js application.
Security and Best Practices
To avoid Next.js build fails caused by environment variables, it's essential to follow security and best practices. Prefix any environment variable that needs to be accessed from the client-side code with NEXT_PUBLIC_, ensuring only variables explicitly marked as public are exposed to the browser.
Use UPPER_CASE and underscores to name your environment variables, making them easily identifiable in your code and separate from regular variables. This consistent naming convention increases code readability and maintainability.
To keep sensitive information secure, store it as environment variables instead of hardcoding. This includes database passwords and secret keys. Never expose sensitive data by using the NEXT_PUBLIC_ prefix for variables that are safe to be exposed.
Here are some guidelines to keep in mind:
By following these guidelines, you can ensure the secure management of environment variables in your Next.js application.
Naming Best Practices
Naming your environment variables in a way that's easy to read and understand is crucial for security and maintainability.
To ensure your variables are easily identifiable, use UPPER_CASE and underscores to name them, just like you would with regular variables.
Prefixing public variables with NEXT_PUBLIC_ is a must, as it prevents sensitive data from being exposed to the client-side code.
Using descriptive names for your environment variables increases code readability and maintainability.
Here's a quick rundown of the best practices:
Consistency is key when it comes to naming environment variables.
Secure Practices
To maintain the security and integrity of your Next.js application, it's essential to follow best practices for managing environment variables.
Prefix public variables with NEXT_PUBLIC_ to prevent sensitive data from being exposed to the client-side. This ensures that only explicitly marked variables are accessible in the browser.
Use a consistent naming convention for environment variables, such as UPPER_CASE and underscores, to make them easily identifiable in your code.
Separate sensitive and non-sensitive variables to prevent unauthorized access to critical information.
Avoid hardcoding sensitive information, such as database passwords or secret keys, in your code. Instead, store them as environment variables.
Store environment variables in files like .env.local, .env.development, etc., and add them to your .gitignore file to prevent sensitive information from being committed to version control.
Set default values for environment variables in your code to ensure they are not left undefined.
Here are some key guidelines to follow:
By following these guidelines, you can ensure that your environment variables are used securely and efficiently in your Next.js application.
Sources
- https://www.dhiwise.com/post/how-to-manage-nextjs-environment-variables-for-better-security
- https://blog.logrocket.com/customizing-environment-variables-next-js-13/
- https://medium.com/@scalablecto/environment-variables-with-containerized-next-js-c13cc05ee099
- https://nextjsstarter.com/blog/nextjs-environment-variables-secure-management-guide/
- https://varenya.hashnode.dev/setting-environment-variables-for-runtime-and-build-time-in-nextjs
Featured Images: pexels.com