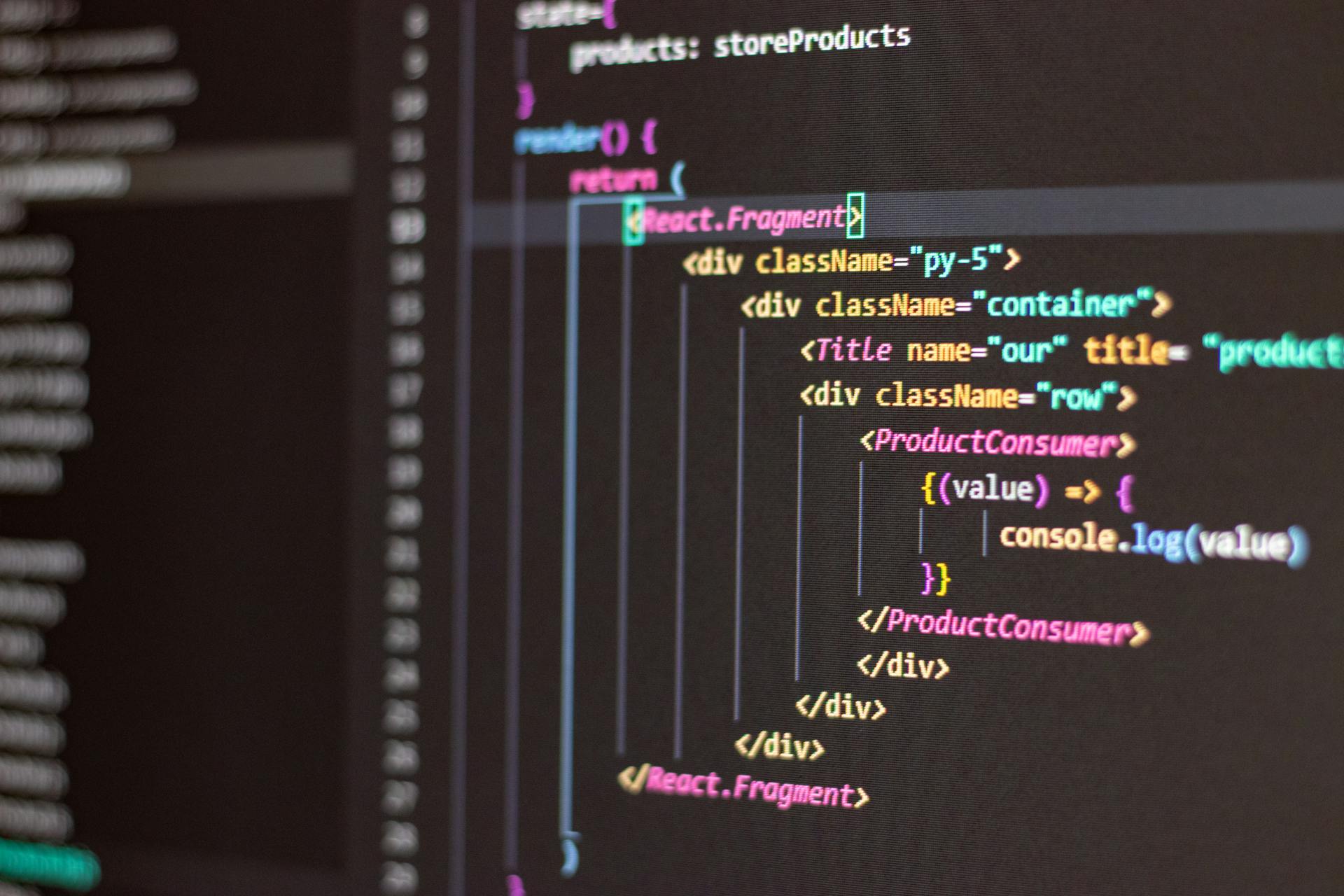
Building a Next.js app for production can be a daunting task, but breaking it down into smaller steps makes it more manageable.
To begin, you'll need to set up a new project using the command `npx create-next-app my-app`.
This command will create a basic Next.js project structure, including a `pages` directory where you'll build your application.
Next, you'll want to install the required dependencies, including `@babel/preset-env` and `@babel/preset-react`.
These dependencies will enable you to use modern JavaScript features and React in your application.
Now that you have your project set up, it's time to configure your environment variables.
This is crucial for a production-ready app, as it allows you to store sensitive information, such as API keys, securely.
To configure your environment variables, you'll need to create a `.env` file in the root of your project.
In this file, you can define your environment variables, such as `NEXT_PUBLIC_API_KEY`.
See what others are reading: Nextjs Build Fails Environment Variables
Project Setup
When structuring your Next.js project, it's essential to create a solid foundation. Start by creating your main pages as React components in the `pages` directory. This directory becomes the route for every file inside it.
You might enjoy: Src Directory Nextjs
In Next.js, every file inside the `pages` directory becomes a route, so make sure to create your main pages like Home, About, and Contact here. Create a `components` directory for reusable components like headers, footers, and buttons. This will help keep your code organized and make it easier to maintain.
Use the `styles` directory to hold your CSS files. Next.js supports CSS Modules out of the box, allowing you to import styles directly into your components.
Here's a quick rundown of the main directories you should create:
By setting up your project in this way, you'll be well on your way to creating a well-structured Next.js app.
Static Site Generation
Static site generation is a crucial step in building a Next.js app for production. You have two approaches to consider: build time secrets and deferring the static site generation until after deployment.
If you need to access deployment secrets and environment variables during the build process, deferring static site generation is the way to go. This approach involves replacing the entry point in your Dockerfile with a script that runs npm build before starting your server.
For your interest: Nextjs Static Site
To make this work, your deployment machines will need enough memory to run a build. You can check the required memory settings by looking into Fly's scale memory and swap_size_mb options. Additionally, you may need to adjust the grace period for any HTTP service checks.
Here are some key considerations to keep in mind:
- Your server will be inaccessible for a period of time while the site is being statically generated.
- If you run multiple machines, the static site generation will be run on each, increasing the total time before changes are fully deployed.
Static Site Generation
Static site generation can be done in two main ways: at build time or after deployment.
You can use build time secrets, but this approach has its limitations.
Deferring the static site generation until after deployment is an alternate approach that works with SQLite3 too.
This method involves running the build step just after deployment, before your web server is started. The upside is that your build has full access to all of your deployment secrets and environment variables.
You can replace the entry point in your Dockerfile with a script that runs npm build (or equivalent) prior to starting your server.
Intriguing read: Next Js Build Time Slow
This can be automated by letting the Dockerfile generator take care of these changes for you.
However, there are some things to keep in mind. Your deployment Machines will need enough memory to run a build.
You can check the memory requirements by looking at fly scale memory and swap_size_mb.
Additionally, you may need to adjust the grace_period for any HTTP service checks.
If you're running multiple Machines, the static site generation will be run on each, increasing the total time before any changes are fully deployed.
Here are some key considerations for deferring static site generation:
- Your deployment Machines will need enough memory to run a build.
- You may need to adjust the grace_period for any HTTP service checks.
- If you are only running one Machine there will be a period of time where your server is inaccessible while the site is being statically generated.
- If you run multiple Machines, the static site generation will be run on each increasing the total time before any changes are fully deployed.
Standalone Builds (Recommended)
Standalone builds are a recommended approach for Next.js apps, as they can reduce your total image size by ~400mb. This is achieved by using the standalone setting in your Next config, which allows your Dockerfile to only include files necessary for a production environment.
This approach is particularly useful when running fly launch or using the Dockerfile generator. By setting the standalone value, you can update your Dockerfile to exclude unnecessary files. After running the build script (npm run build), you'll need to replace the final COPY instruction with a new one.
Updating your Dockerfile in this way is a simple yet effective way to optimize your app's performance. By doing so, you can ensure that your app is running efficiently and effectively, even under heavy traffic.
Related reading: Docker Next Js
Optimizing Performance
Optimizing Performance is crucial for a smooth user experience. You can use the Next.js Image component to automatically optimize image loading.
Code Optimization is also essential. Review your code for any inefficiencies or unnecessary computations. Next.js's built-in performance optimizations like automatic code splitting and lazy loading can help.
Here are some key performance optimization techniques to keep in mind:
- Code Splitting: Next.js automatically splits your code into smaller bundles. Use dynamic imports (import()) for further optimization, especially for heavy components.
- Image Optimization: Use the Next.js Image component to automatically optimize image loading.
Static Generation and Server-Side Rendering can also help optimize load times. Ensure that your pages are using getStaticProps or getServerSideRendering appropriately.
Consider using a CDN like Cloudflare to cache your static assets close to the end users. This can reduce the load on your app and improve performance. Next.js generates filenames for static assets that can be cached for a long time, making it easy to implement.
A different take: Using State in Next Js
Security and Testing
Security and Testing are crucial aspects of building a Next.js app for production. You want to ensure your app is secure and stable, and that's where testing and security best practices come in.
To start with, unit testing is essential. This involves writing unit tests for your components using a testing framework like Jest. You can also use React Testing Library for this purpose.
End-to-end testing is another important aspect. This type of testing ensures that your entire application works seamlessly together. You can use tools like Cypress or Selenium for this purpose.
Here are some security best practices to keep in mind:
- Secure sensitive information like API keys using environment variables.
- Regularly update your dependencies to their latest versions to include security patches.
- Implement security measures for API routes, like rate limiting, to prevent abuse.
By following these security and testing best practices, you can ensure your Next.js app is secure, stable, and ready for production.
Select a Hosting Platform
Selecting a hosting platform is a crucial step in building a Next.js app for production. Vercel, the creators of Next.js, offers a seamless deployment experience with automatic deployments, serverless functions, and a global CDN.
You can also consider Netlify, another popular choice for static sites that offers continuous deployment from Git repositories. This feature allows you to deploy directly from your Git repository (GitHub, GitLab, or Bitbucket).
Suggestion: Deploy Next Js
For more control, you can use cloud services like AWS, Azure, or Google Cloud. These platforms require more configuration but offer greater flexibility.
Here are some key hosting options to consider:
Ultimately, the choice of hosting platform depends on your specific needs and preferences.
Continuous Integration and Deployment
Continuous Integration and Deployment is a crucial step in getting your Next.js app ready for production. Automate deployments by setting up your project for continuous deployment, which triggers a new deployment automatically whenever you push changes to your main branch.
To make your deployment process smoother, you can set up a CI/CD pipeline with GitHub Actions. This will automatically build and deploy your app whenever you push changes to your repository.
To define a workflow, create a file at `.github/workflows/deploy.yml` with the following contents. This file will guide GitHub Actions on how to build and deploy your app.
Here's a breakdown of the secrets you'll need to add to your GitHub Repo > Settings > Secrets:
- EXAMPLE_SECRET: a unique secret value, e.g. "supersecret-123"
- DOCKER_USERNAME: your Docker username
- DOCKER_PASSWORD: your Docker password
- SSH_PRIVATE_KEY: your private SSH key
Once you've added these secrets, update the `.kamal/secrets` file to read the secrets from the environment instead of being hardcoded. This will make it safer to commit this file to your git repo.
Curious to learn more? Check out: Next Js Folder Structure
Feature Development and Deployment
Feature Development and Deployment is a crucial step in building a Next.js app for production. Implement navigation using Next.js's Link component for client-side transitions between routes.
To ensure a consistent look, create layout components to wrap around your pages, including headers and footers. This will help maintain a cohesive design throughout your app.
If your app requires backend functionality, use API routes in the pages/api directory to handle server-side logic. This will allow you to keep your server-side logic separate from your client-side code.
Here are the key features to focus on during feature development and deployment:
- Navigation: Implement client-side transitions between routes using Next.js's Link component.
- Layouts: Create layout components for consistent headers and footers.
- API Routes: Use server-side logic in the pages/api directory for backend functionality.
Feature Development
Developing features for your application is a crucial step in bringing your idea to life. You can start by implementing navigation using Next.js's Link component for client-side transitions between routes.
To ensure a consistent look throughout your application, create layout components to wrap around your pages. This includes headers and footers that will be reused across your app.
See what others are reading: Next Js Spa
API routes in the pages/api directory can be used to handle server-side logic if your app requires backend functionality. This is a great way to keep your logic organized and separate from your frontend code.
Here are some key features to consider when developing your application:
- Navigation: Implement client-side transitions using Next.js's Link component.
- Layouts: Create reusable layout components for headers and footers.
- API Routes: Use the pages/api directory for server-side logic.
Fetching Data
Fetching data is a crucial aspect of feature development and deployment. You have two primary options to fetch data: static generation and server-side rendering.
Static generation uses getStaticProps, which runs at build time and fetches data that can be passed to your pages as props. This approach is ideal for pages that don't require real-time data.
Server-side rendering, on the other hand, uses getServerSideProps, which runs on each request and is suitable for pages that need real-time data.
Here's a summary of the two options:
- Static Generation: getStaticProps, runs at build time, suitable for pages that don't require real-time data.
- Server-Side Rendering: getServerSideProps, runs on each request, suitable for pages that need real-time data.
Build a Multi-Tenant
Building a multi-tenant app is a great way to increase scalability and flexibility. You can learn how to do it with Next.js, a popular framework for building server-rendered and statically generated websites and applications.
Developing a multi-tenant app requires careful planning and execution. It's essential to consider the custom domains feature, which allows each tenant to have their own unique domain.
With Next.js, you can build a multi-tenant app with custom domains using the framework's built-in features. This enables each tenant to have full control over their own branding and identity.
Building a multi-tenant app can be a complex task, but with the right tools and knowledge, it's achievable. By leveraging Next.js and its custom domains feature, you can create a scalable and flexible app that meets the needs of multiple tenants.
For more insights, see: Nextjs Multi Tenant App
Featured Images: pexels.com