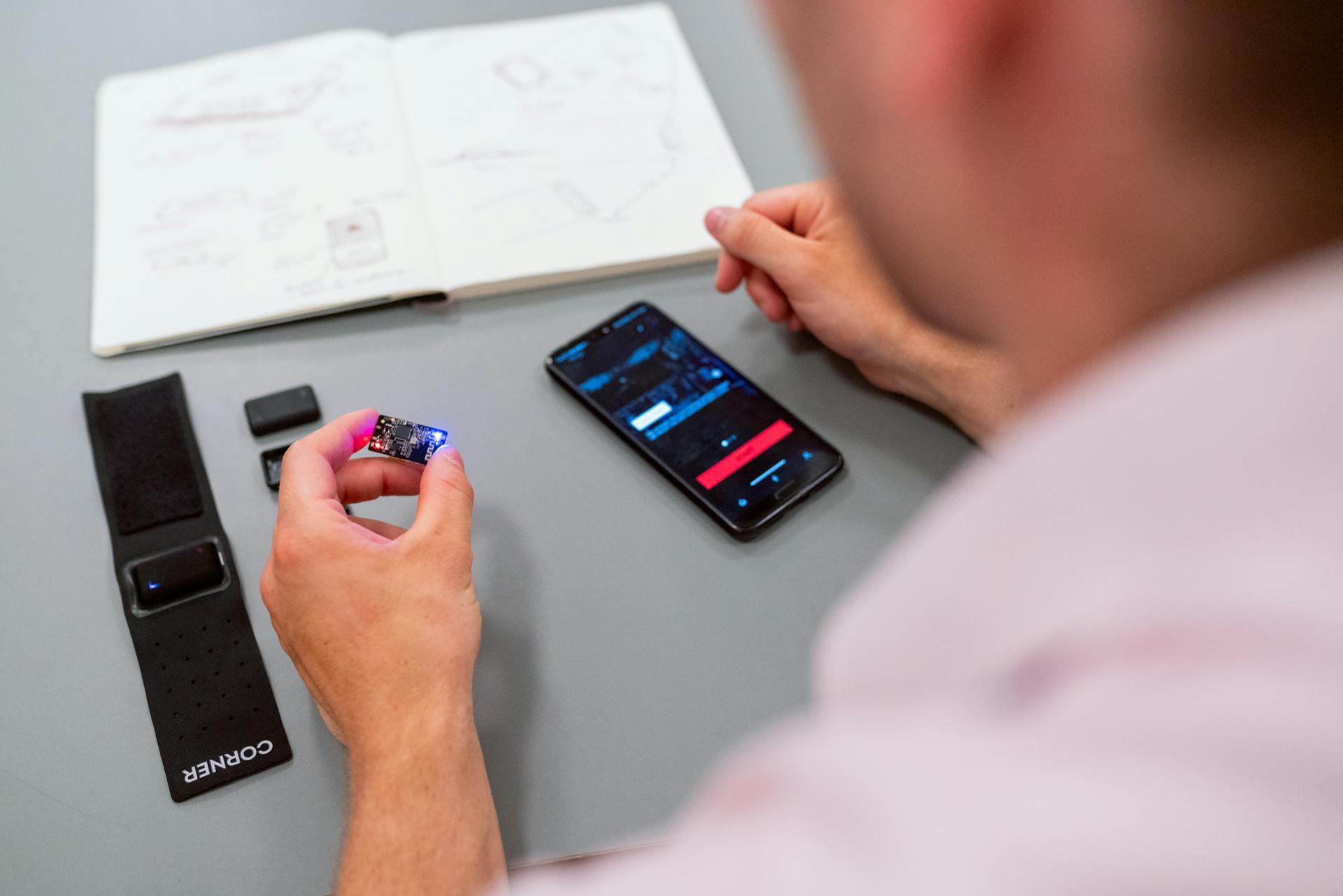
Static Nextjs site generation is a game-changer for improved performance. By pre-rendering pages at build time, Nextjs can serve static HTML files to users, reducing server latency and improving page load times.
This approach is especially effective for sites with a large number of pages or complex routes. Nextjs can handle this efficiently, generating static files for each page.
As a result, users enjoy faster page loads, and developers can focus on building a better user experience.
Related reading: Nextjs Pages
What Is Static Site Generation
Static site generation is a tool that generates a complete static HTML website based on raw data and templates. It delivers super-fast websites because it generates pages at build time.
Next.js is considered a great option for static site generation because it not only generates static pages but also provides benefits such as super-fast performance, up-to-date security, and improved user experience without compromising on SEO or the quality of the user interface.
Discover more: Responsive User Interface Design
Static site generation is the default page generation method in Next.js, offering improved performance and better SEO. With SSG, pages are generated at build time and served as static files.
To use static site generation in Next.js, you can use the getStaticProps function, which runs at build time and fetches the data needed to render the page. The fetched data is then passed as props to the page component.
Data is fetched once during the build process, making it a fast and efficient way to generate pages.
Related reading: Html Real Time Editor
Benefits of Using
Using a static Next.js site can significantly boost your website's performance. Static Site Generators are extremely fast, prefetching resources for other pages so navigating the site feels instantaneous.
With a static site, you'll publish static files, which means there's no direct connection to the database, dependencies, user's data, or other sensitive information. This approach is perfect for marketing sites, blogs, or manuals.
Static pages in Next.js offer improved performance, better SEO, and simpler deployment. Improved performance is achieved by pre-rendering pages throughout the development process, resulting in faster page load times and a smoother user experience.
Static pages are pre-rendered at build time and served as HTML files to the client, eliminating the need to generate the page on each request. This results in faster page load times and a smoother user experience.
By using static pages, you can improve your website's SEO, as search engines can easily crawl and index static HTML pages. Pre-rendered static pages also provide metadata that can be used by search engines to understand the content of your pages.
Deploying static pages is often simpler and more cost-effective compared to deploying server-side rendered or client-side rendered applications. You can deploy static pages to a variety of hosting platforms, including static site hosting services like Vercel and Netlify.
Using static pages in Next.js can also reduce server load, as static pages do not require server-side processing for each request. This is especially beneficial for high-traffic websites that need to handle a large number of concurrent requests.
Static pages can be cached by content delivery networks (CDNs) and served to users even when they are offline, providing a seamless browsing experience and ensuring that your content is always accessible.
Expand your knowledge: Next Js Client Side Rendering
App Creation and Configuration
To create a Next.js app for static site generation, start by running the command to create a new Next app. This will set up the basic structure of your project.
The project structure includes a pages directory where you'll store all your pages, and a pages/api directory where you can add API endpoints if needed. You can safely remove the pages/api directory if you don't need it.
Modify the next.config.mjs file to configure your project according to your needs. Change the project configuration to include the static HTML files and assets you want to host. For example, you can add the following code to next.config.mjs to change the out directory:
Discover more: Next Js Folder Structure
Introduction to Applications
Static Site Generators (SSGs) have been around for two decades, starting as niche tools for small, fast-loading sites.
Movable Type in 2001 and Jekyll in 2008 were early adopters of this approach, generating pre-rendered HTML that bypassed server-side rendering on every page load.
Broaden your view: Wix Website - Site Page to Different Webpage on Site
The use of SSGs surged around 2015 with the advent of the Jamstack architecture, which promotes a static-first approach to web development.
JavaScript frameworks like Gatsby and Next.js made SSGs accessible for modern React-based applications, contributing to their popularity.
Since Next.js introduced static site generation capabilities in version 9.3 (released in March 2020), it has become a leading choice for SSG.
Next.js combines the speed and SEO benefits of SSG with a powerful ecosystem, enabling developers to create fast, SEO-friendly websites without compromising user experience.
The Jamstack architecture has transformed the way we build web applications, making them faster, more secure, and scalable.
Next.js is a key player in this transformation, offering a powerful toolset for building high-performance web apps.
See what others are reading: Web Page Architecture
App Creation and Configuration
Creating a new Next.js app is as simple as running a command. We'll start by running `npx create-next-app my-app` to create a new Next app.
To get an idea of our project structure, let's take a look. The `pages` directory contains all our pages and defines routing for our app.
On a similar theme: Next Js Pages vs App
The `pages/api` directory is where we can add API endpoints, but in our case, we can safely remove it.
To configure our project, we'll modify `next.config.mjs` to suit our needs. We can change the `outDir` to a custom directory by adding `distDir` to `next.config.mjs`.
We can test our configuration by running the `build` command. Next should create a directory at the root of our project with all the static HTML files and assets ready to host.
If this caught your attention, see: Next Js Src Directory
Data Fetching
Data Fetching is a crucial aspect of building a static Next.js site. You can use the getStaticProps function to fetch data at build time and pre-render the pages as static files.
This method is suitable for data that doesn't change frequently and can be pre-rendered. For example, in a blog, you can use getStaticProps to fetch data from a content management system.
You can also use getStaticPaths to specify which paths should be pre-rendered. This function is executed at build time, giving you the flexibility to manage dynamic behavior.
Broaden your view: Next Js Fetch Data
In addition to getStaticProps, you can also use getServerSideProps to fetch data on the server for each request and render the page with the fetched data. However, this method is suitable for data that needs to be fetched at runtime and can't be pre-rendered.
Here are the different data fetching methods available in Next.js:
To fetch data in Next.js, you can use the getStaticProps, getServerSideProps, or client-side data fetching techniques. For example, in a blog, you can use getStaticProps to fetch data from a content management system.
Discover more: Nextjs App Route Get Ssr Data
Page Generation and Routing
Next.js provides a flexible and efficient way to generate pages with different content types. With static site generation, pages are generated at build time and served as static files.
Static site generation is the default page generation method in Next.js. This approach offers improved performance and better SEO. With SSG, pages are generated at build time and served as static files.
To use static site generation in Next.js, you can use the getStaticProps function. This function runs at build time and fetches the data needed to render the page. The fetched data is then passed as props to the page component, which can be used to render the content of the page.
Data is fetched once during the build process, making it ideal for static sites. This approach eliminates the need for server-side rendering or client-side rendering.
Next.js uses a file-based routing system, which means that each page in your application corresponds to a file in the pages directory. The file name determines the URL of the page.
For example, if you have a file called pages/about.js, the URL of the corresponding page will be /about. This makes it easy to organize your codebase and define the structure of your application.
File-based routing also supports nested routes by creating subdirectories within the pages directory. For example, if you have a file called pages/products/index.js, the URL of the corresponding page will be /products.
This approach simplifies the process of creating and organizing pages in your application. With file-based routing, you can easily create new pages and routes without manual configuration.
A unique perspective: Creating Restful Webservices in Java
Web Development
In the world of web development, there are many frameworks to choose from, but one that stands out is Next.js. Next.js is a popular framework for building static websites, and it's especially great for developers who are already familiar with React.
Next.js and React are closely related, in fact, Next.js is built on top of React. This means that if you're already using React, you can easily switch to Next.js and take advantage of its additional features.
One of the key benefits of using Next.js is its ability to pre-render pages at build time, which can improve performance and reduce load times. This is especially important for static websites, where every millisecond counts.
Next.js also has a built-in API routing system, which makes it easy to create and manage API routes for your website. This can be a huge time-saver, especially for developers who are new to web development.
Overall, Next.js is a powerful tool for building fast and scalable static websites.
For more insights, see: Webflow to React
How to Use Static Site Generators
Static site generators are a game-changer for Next.js sites. They generate complete static HTML websites based on raw data and templates, resulting in super-fast websites.
To use static site generators in Next.js, you can use the getStaticProps function, which runs at build time and fetches the data needed to render the page. This function is executed at build time, giving you the flexibility to specify which paths should be pre-rendered.
Static site generators offer several benefits, including improved performance, better SEO, and simpler deployment. Static pages are pre-rendered at build time and served as HTML files to the client, eliminating the need to generate the page on each request.
Additional reading: Designing Websites for Older Adults
What Are Site Generators?
Static Site Generators (SSGs) are tools that generate a complete static HTML website based on raw data and templates.
They deliver super-fast websites because they generate pages at build time, making them a great option for websites that need to load quickly.
Discover more: Designing Websites Free
Next.js is considered a great option for SSG because it not only generates static pages but also provides benefits such as super-fast performance.
SSGs are known for their ability to generate pages with or without data statically, which allows for a high level of customization.
Next.js allows you to generate pages with or without data statically, making it a versatile tool for building websites.
Curious to learn more? Check out: Data Text Html Charset Utf 8 Base64
How to Use SSG
To use Static Site Generators (SSG) in Next.js, you need to create a new page for the content you want to generate statically. This page will fetch data from a content management system, and you can use the getStaticProps function to fetch the data needed for the page.
You can use the getStaticProps function to fetch data at build time and pass it as props to the page's component. This function gets called at build time and allows you to pass the fetched data to the page's props.
On a similar theme: Next Js Getstaticprops
In Next.js, you can use the getStaticPaths function to specify which paths should be pre-rendered. This function is executed at build time and allows you to fetch external data to determine dynamic paths based on the parameter.
To generate a page using static site generation in Next.js, you need to create a new file in the pages directory for the page you want to generate statically. For example, let's create a file named blog.js. This page will fetch data from a content management system.
The getStaticProps function is used to fetch data at build time and pass it as props to the page's component. In the example, the getStaticProps function is used to fetch data for the Blog component.
Next.js allows you to export an asynchronous function named getStaticPaths from a dynamic page to specify which paths should be pre-rendered. This function is executed at build time and allows you to fetch external data to determine dynamic paths based on the parameter.
You can use the getStaticProps function to fetch data at build time and pass it as props to the page's component. This function gets called at build time and allows you to pass the fetched data to the page's props.
Additional reading: Next Js App
To manage dynamic behavior, Next.js allows you to export an asynchronous function named getStaticPaths from a dynamic page. This function is executed at build time and gives you the flexibility to specify which paths should be pre-rendered.
In Next.js, you can use the getStaticPaths function to specify which paths should be pre-rendered. This function is executed at build time and allows you to fetch external data to determine dynamic paths based on the parameter.
The getStaticProps function is used to fetch data at build time and pass it as props to the page's component. In the example, the getStaticProps function is used to fetch data for the Post component.
Consider reading: Next Js Dynamic
Frequently Asked Questions
Is Gatsby or Next.js better for static websites?
Gatsby is ideal for simple static websites, while Next.js is better suited for more complex web applications. Choose Gatsby for a fast and simple blog or site, but consider Next.js for dynamic content and back-end functionality.
Is Next.js static by default?
Next.js is not static by default, but it can be if a page has no blocking data requirements and doesn't use getServerSideProps or getInitialProps. This allows for prerendering of static pages.
Sources
- https://pagepro.co/blog/how-to-use-next-js-static-site-generator/
- https://developer.mozilla.org/en-US/blog/static-site-generation-with-nextjs/
- https://staticmania.com/blog/how-can-we-use-ssg-in-next-js
- https://nextjs.org/docs/pages/building-your-application/deploying
- https://www.squash.io/creating-dynamic-and-static-pages-with-next-js/
Featured Images: pexels.com