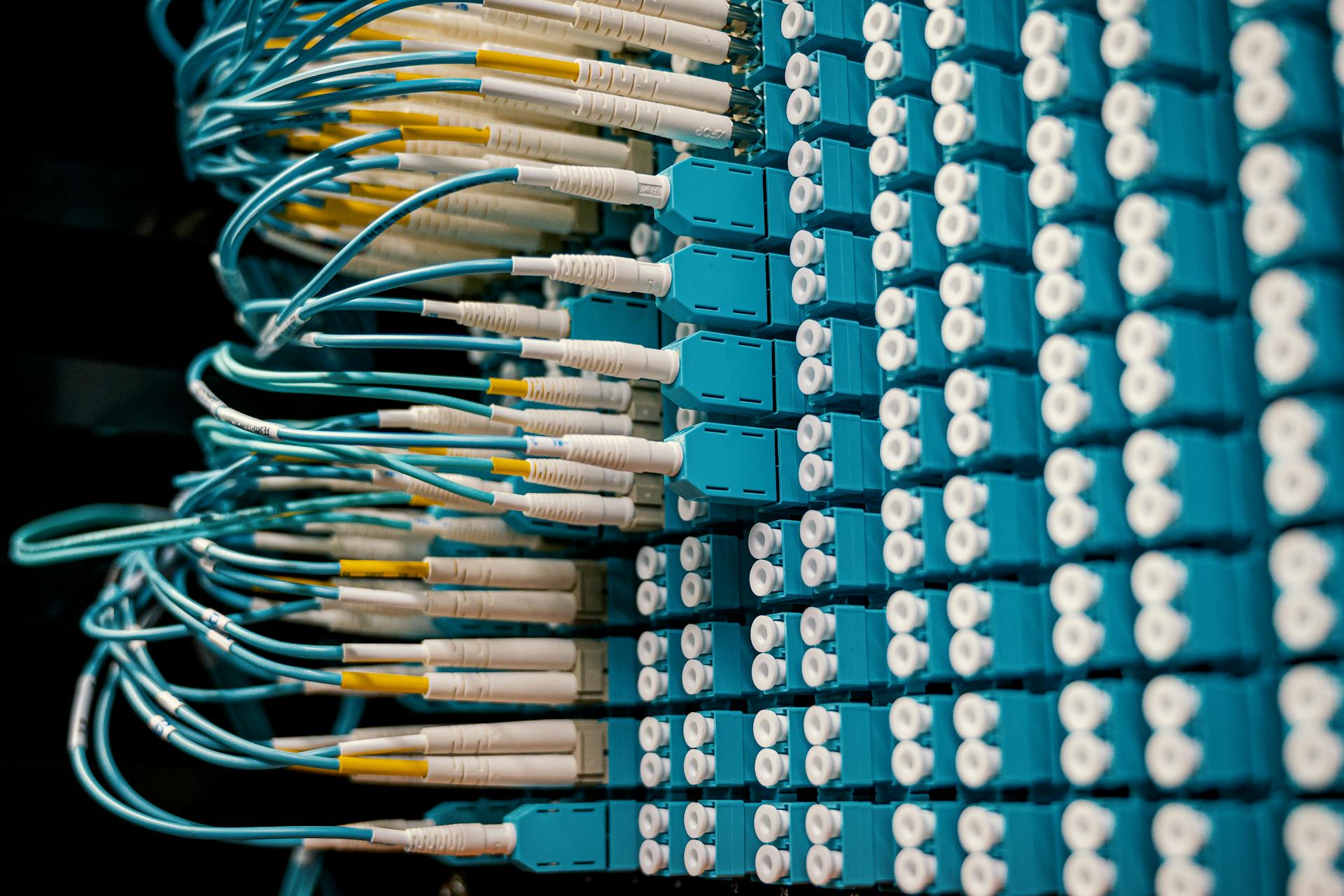
Creating a RESTful web service in Java involves defining the API endpoints and the data they will handle. This is typically done by creating a Java class annotated with @Path.
To start creating a RESTful web service, you need to create a Java class that will serve as the entry point for your web service. This class is annotated with @Path and defines the base URL for your web service.
The @Path annotation is used to specify the base URL for your web service. For example, if your web service is hosted at http://localhost:8080/mywebapp, you would use @Path("/mywebapp") to specify the base URL.
A simple example of a Java class that serves as the entry point for a web service is the Jersey framework's Application class. This class is annotated with @ApplicationPath and defines the base URL for the web service.
Additional reading: How to Create a Service Principal in Azure
Prerequisites
To create RESTful web services in Java, you'll need to meet some prerequisites.
We'll be using Eclipse Oxygen as our development environment.
The underlying JDK should be at version 1.8.
TomEE Plus will be our deployment target.
You can download TomEE Plus from the Apache project's home page.
We're choosing TomEE Plus over Tomcat or standard TomEE because it includes the necessary JAX-RS libraries.
Without these libraries, RESTful web services won't work with Tomcat or standard TomEE.
Setting Up the Environment
To start creating RESTful web services in Java, you'll need to set up the right environment. First, download and install the latest version of the Java Development Kit (JDK) from the official website.
You'll also need a good Integrated Development Environment (IDE) to write and manage your code. We recommend using Eclipse, IntelliJ IDEA, or NetBeans for Java web service development.
To deploy your web services, you'll need an application server like Apache Tomcat, GlassFish, or WildFly, which is part of the Java EE specification.
Here are the key tools and libraries you'll need to get started:
- Java Development Kit (JDK)
- Integrated Development Environment (IDE) such as Eclipse, IntelliJ IDEA, or NetBeans
- Application server like Apache Tomcat, GlassFish, or WildFly
- JAX-RS libraries
Creating the Project
To start creating RESTful web services in Java, you need to create a Java Web application project. This can be done by choosing File > New Project in Eclipse and selecting Java Web under Categories.
Select Web Application under Projects and click Next. The New Web Application wizard will open. Alternatively, you can create a Maven Web Application by choosing File > New Project and selecting Maven under Categories.
To create a project using the New Web Application wizard, enter a project name, such as "CustomerDB", and click Next. You will then select either Java EE 6 Web or Java EE 7 Web and choose a server, but note that Java EE projects require GlassFish server 3.x or 4.x.
Here are the steps to create a project using the New Web Application wizard:
- Choose File > New Project in Eclipse and select Java Web under Categories.
- Select Web Application under Projects and click Next.
- Enter a project name, such as "CustomerDB", and click Next.
- Select either Java EE 6 Web or Java EE 7 Web and choose a server.
For Maven projects, you will need to set the server before creating a persistence unit. To do this, open the project's Properties and set the server in the Run properties.
Discover more: How to Create a Website Hosting Server
Entity Classes
Entity classes are a crucial part of creating RESTful web services in Java. They represent the data that will be exchanged between the client and the server. Entity classes can be created using the Java Persistence API (JPA) or by using the NetBeans IDE.
The Java Persistence API defines entity classes as lightweight persistence domain objects that typically represent a table in a relational database. Each entity instance corresponds to a row in that table. To create entity classes, you can use the NetBeans IDE's RESTful Services from Database wizard, which automatically generates the persistence unit.
Entity classes are annotated with JAXB annotations to enable serialization and deserialization of the data. The @XmlRootElement annotation is used to indicate that the class can be converted to and from XML. You can also use the @Entity annotation to indicate that the class is an entity class. For example, the Customer class in Example 1 is annotated with @XmlRootElement to enable XML serialization and deserialization.
Broaden your view: Create Schema Azure Data Studio
The Customer Entity
The Customer entity is a simple class that contains id, name, and address fields. It represents the Customer entity you manage with the web service.
The Customer class is created by right-clicking the restdemo project and selecting New>Class. You then type com.myeclipseide.ws in the Package field and Customer in the Name field.
To create the Customer class, you replace the default contents of the generated class with the following code. This code includes the necessary fields and methods for the Customer entity.
Here is a breakdown of the Customer class:
- The class is annotated with @XmlRootElement, which allows JAXB to convert the entity from Java to XML and back.
- The class has three fields: id, name, and address.
- The class has getter and setter methods for each field, which allow you to access and modify the field values.
By following these steps and using the provided code, you can create a basic Customer entity class that represents the Customer entity in your web service.
Entity Classes
Entity classes are a crucial part of RESTful web services in Java, and they play a vital role in mapping objects to relational databases.
Entity classes are Java classes that represent objects in a relational database, typically mapping to a table in the database. According to The Java EE5 Tutorial, "An entity is a lightweight persistence domain object."
Broaden your view: Web Page Design Classes Online
To create entity classes, you can use NetBeans IDE, either by creating them in the same process as RESTful web services or by generating them from existing entity classes. The IDE can also be used to create RESTful web services from existing entity classes.
Entity classes are typically generated by the RESTful Services from Database wizard, which automatically generates the persistence unit. The wizard also generates JAXB annotations, which allow for the conversion of entity classes from Java to XML and back.
The Java Persistence API relies on entity classes to communicate with a database, and each entity instance corresponds to a row in the database table. The persistence unit consists of the set of entity classes, the data source, the persistence provider, and the persistence unit’s own name, as defined in a persistence.xml file.
Here is a summary of the steps to generate entity classes and RESTful web services:
- Right-click the CustomerDB node and choose New > Other > Web Services > RESTful Web Services from Database.
- Select the jdbc/sample data source from the Data Source drop-down field.
- Type entities for the Package name.
- Click Next, and then click Finish to generate the entity classes and RESTful web services.
By following these steps, you can create entity classes and RESTful web services in the same process, making it easier to manage your database and web services.
Recommended read: Webflow Development Services
Implementing the API
Implementing the API is where the magic happens. We'll start by creating a simple RESTful API that returns a list of employees, which we'll manage with Maven to handle dependencies and the build process.
To create this API, we'll use JAX-RS, a Java API for RESTful Web Services. We'll also use the ApplicationPath annotation to define the base URL of our web service.
The ScoreService class is the heart of our RESTful web service example. It contains three getter methods that allow RESTful web clients to query the number of wins, losses, or ties. These methods are invoked through an HTTP GET invocation and return the current win, loss, or tie count as plain text.
Each of these getter methods has a JAX-RS @GET annotation, a @Produces annotation indicating they return a text string, and a @Path annotation indicating the URL clients need to use to invoke the method.
The increase methods of the ScoreService class follow a similar pattern, but are triggered through an HTTP POST invocation.
To return JSON from our RESTful web service, we can use @Producer annotations and simply return JavaBeans. However, since we're using static variables in our Score class, this approach gets a bit messy.
We'll save this for a future tutorial on RESTful web services with Eclipse.
A different take: How to Use Inspect Element to Find Answers
Deploy the
Deploying a RESTful web service in Java is a crucial step in making it accessible to clients. You can deploy a web service by right-clicking on the project and selecting Run As > Run on server, which will deploy the web project and start the Apache TomEE Plus server that hosts the application.
There are several ways to test a deployed web service, including typing the URL of the RESTful web service into a web browser. You can also use curl commands in a Bash shell to test the web service.
To deploy a web service using MyEclipse, right-click the project and select Debug As (or Run As) > MyEclipse Server Application. This will package the web service project and deploy it in exploded format to the application server.
Once deployed, you can use the REST Web Services Explorer to test the web service. Right-click the project and select Web Service Tools > Test with REST Web Services Explorer. This will open the explorer, where you can select the method to test and enter information to run the test.
For another approach, see: Web Dev Services
Here are the steps to deploy a web service using MyEclipse:
- Right-click the project and select Debug As (or Run As) > MyEclipse Server Application.
- Select MyEclipse Tomcat and click Finish.
Alternatively, you can use a wizard in the IDE to generate the tests in a new project. Right-click the project and choose Test RESTful Web Services to generate the test client.
Here are the steps to test a deployed web service:
- Right-click the project and choose Test RESTful Web Services.
- Click the entities.customer node and select either GET (application/json) or GET (application/xml).
- Click Test to send a request and display the result in the Test Output section.
You can also test a deployed web service using a REST client like Postman or Insomnia. Deploy your web service to the application server and send a GET request to http://localhost:8080/your-app-name/api/employees to receive a JSON response with the list of employees.
Troubleshooting and Tools
If you're getting a 404 error, check if your API endpoint is correctly registered in your web.xml file, as we saw in the "Configuring the REST Service" section.
A good IDE like Eclipse or IntelliJ can help you catch common mistakes and errors in your code, such as missing import statements or incorrect syntax.
When testing your REST service, make sure to use a tool like Postman or cURL to send HTTP requests to your API endpoint, as we did in the "Testing the REST Service" section.
Java's built-in logging mechanism can be useful for debugging purposes, especially when using a framework like Log4j.
The Java API for RESTful Web Services (JAX-RS) provides a number of built-in features for handling errors and exceptions, such as the @Produces and @Consumes annotations.
For more insights, see: Webflow A/b Testing
Why and How to Use APIs
Using APIs in Java is a no-brainer, especially when it comes to creating RESTful web services. JAX-RS, a set of APIs designed to facilitate the development of RESTful web services using Java, makes it easy to define resources, methods, and response formats.
With JAX-RS, you can build scalable and high-performance web services that can handle a large number of concurrent users. It supports various data formats like XML and JSON, promoting interoperability between different systems.
Here are some key benefits of using JAX-RS for creating RESTful APIs:
- Ease of development: JAX-RS annotations make it easy to define resources, methods, and response formats.
- Standardization: JAX-RS is part of the Java EE specification, ensuring a consistent approach to developing RESTful web services across different platforms.
- Interoperability: JAX-RS supports various data formats (e.g., XML, JSON) for exchanging information between the client and the server, promoting interoperability between different systems.
- Scalability: JAX-RS can be used to build scalable and high-performance web services that can handle a large number of concurrent users.
What Is?
APIs are a crucial part of modern software development, and understanding what they are and how they work is essential for building successful applications.
JAX-RS is a set of APIs designed to facilitate the development of RESTful web services using the Java programming language.
JAX-RS is part of the Java EE specification, which means it can be used with any Java EE compliant application server.
RESTful web services are a type of API that uses HTTP requests to interact with a server, making it a popular choice for web development.
JAX-RS provides annotations and APIs to simplify the process of creating, consuming, and deploying RESTful web services.
Take a look at this: Frontend Development Company
Why Use APIs?
Using APIs can greatly simplify the development process, especially when it comes to creating RESTful APIs. JAX-RS annotations make it easy to define the resources, methods, and response formats.
APIs provide a consistent approach to developing web services across different platforms. JAX-RS is part of the Java EE specification, ensuring a standardized way of building RESTful web services.
APIs promote interoperability between different systems by supporting various data formats. For example, JAX-RS supports exchanging information in XML and JSON formats.
APIs are also scalable and can handle a large number of concurrent users. This makes them ideal for building high-performance web services that can handle a high volume of traffic.
For your interest: Building Progressive Web Apps
Frequently Asked Questions
How do I create a REST service?
To create a REST service, start by identifying the core resources and designing intuitive URIs, then determine the data format for each resource. This foundation will help you build a scalable and user-friendly API.
Which is the Java API for RESTful web services?
JAX-RS is the Java API for building RESTful web services. It simplifies web service development with its annotation-driven approach.
Sources
- https://www.theserverside.com/video/Step-by-step-RESTful-web-service-example-in-Java-using-Eclipse
- https://www.genuitec.com/docs/web-services/developing-rest-web-service/
- https://netbeans.apache.org/tutorial/main/kb/docs/websvc/rest/
- https://javarevisited.blogspot.com/2022/02/spring-boot-restful-web-service-example-tutorial.html
- https://reintech.io/blog/java-web-services-creating-restful-apis-with-jax-rs
Featured Images: pexels.com