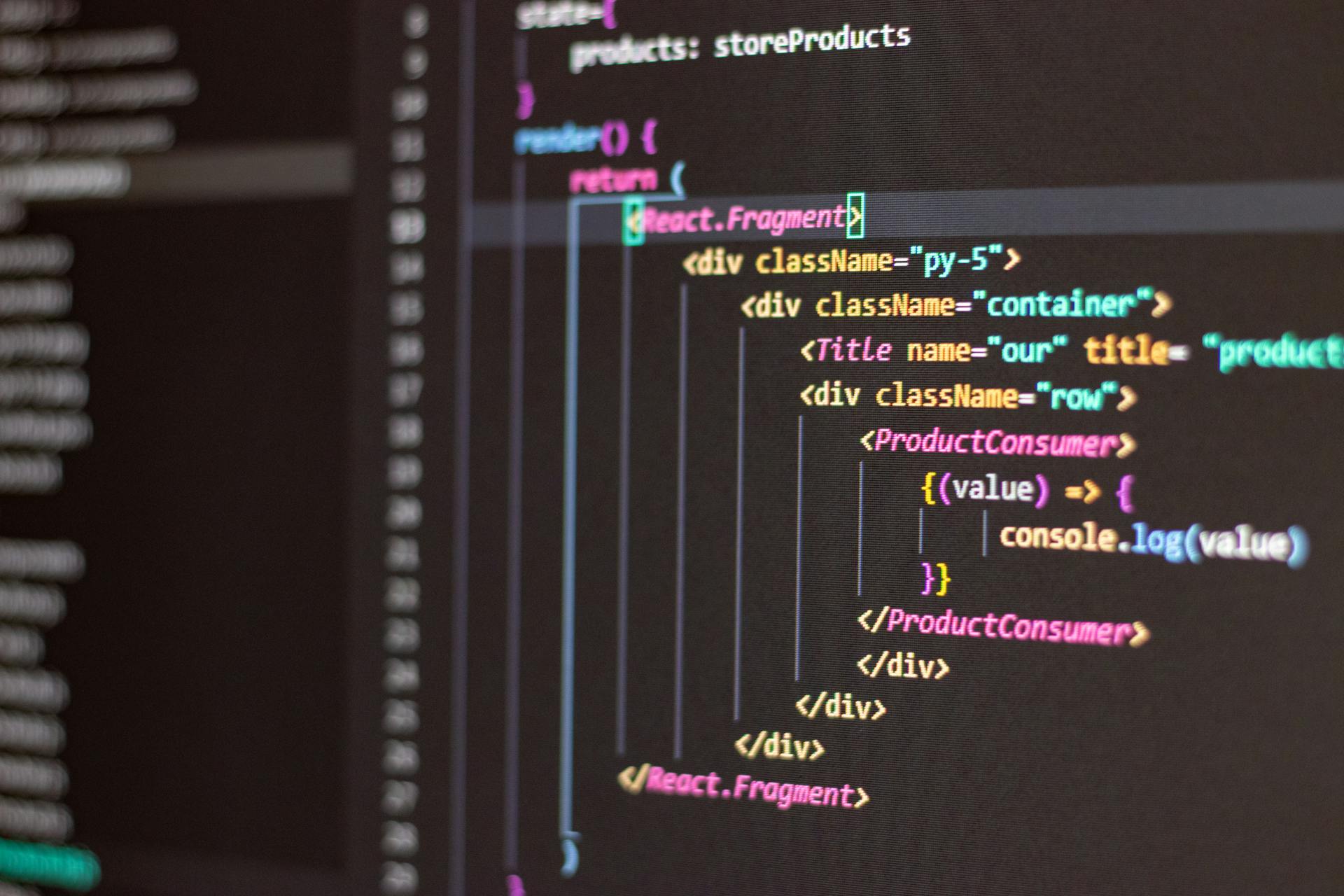
Next JS pages development is a game-changer for web development. With Next JS, you can create server-side rendered pages that are fast, scalable, and SEO-friendly.
By using Next JS, you can easily create pages that are optimized for search engines, thanks to its built-in support for server-side rendering. This means your pages will load faster and appear higher in search engine results.
One of the key benefits of Next JS pages is that they can be pre-rendered at build time, which reduces the load on your server and improves performance. This is especially useful for complex pages with a lot of dynamic content.
Next JS also makes it easy to handle dynamic routes and pages, allowing you to create a more flexible and scalable application.
Installation and Setup
To get started with Next.js, you'll first need to install it. You can do this by running npm init next-app in your terminal. This will create a basic structure for your project, including a place for your pages and static assets.
Next.js takes care of the complexity for you, hiding it in the dependencies. This includes a whopping 65MB of JavaScript code in the node_modules folder.
The initialization script will also give you the option to choose between a default starter app or a more sophisticated example. For this article, we'll be using the default option.
Dynamic Routes
Dynamic routes in Next.js are a powerful feature that allows you to create routes that are generated on the fly, rather than being hardcoded into your application. This is done by using a special syntax in the filename of the page, where brackets are used to define a dynamic parameter.
For example, if you want to create a dynamic route for user profiles, you can create a file called `pages/users/[name].js`. The `[name]` placeholder in the filename defines a new dynamic parameter, which can be accessed using the `useRouter` hook from the `next/router` module.
You can also use catch-all routes to handle URLs with multiple parameters. For instance, if you have a file called `pages/docs/[...params].js`, it will match URLs like `/docs/a`, `/docs/a/b`, or `/docs/a/b/c/d/a/b`.
To create a dynamic route, you need to create a new file in the `pages` directory with a filename that includes brackets to define the dynamic parameter. The file itself should be a standard Next.js page, exporting a React component as its default export.
Here are some examples of dynamic route filenames:
- `pages/users/[name].js`
- `pages/docs/[...params].js`
- `pages/notes/[id].js`
These filenames define the dynamic parameters that can be accessed using the `useRouter` hook.
Page Generation
Next.js provides various methods for generating pages, including static site generation (SSG), server-side rendering (SSR), and client-side rendering (CSR). The choice of page generation method depends on your specific requirements and the type of content you want to display.
Static site generation (SSG) is the default page generation method in Next.js, offering improved performance and better SEO. With SSG, pages are generated at build time and served as static files.
To use static site generation in Next.js, you can use the getStaticProps function, which runs at build time and fetches the data needed to render the page. The fetched data is then passed as props to the page component, allowing you to render the content of the page.
Creating New Page
Creating a new page in Next.js is a straightforward process. You can create a new page by adding a new JavaScript file to the pages directory that exports a React component.
The filename of the file will determine the new page's URL. For example, if you create a file called hello.js, the new route will be /hello. You can access this new page by opening http://localhost:3000/hello in your browser.
Creating multiple levels of subdirectories will result in a hierarchical URL structure. For instance, if you create the page /pages/products/shirts.js, its URL will be http://localhost:3000/products/shirts.
To create a path like /project/settings, you can use folders in your /pages directory. By adding an index page in a folder, you're telling Next.js that you want this component to be the index route for this path.
Next.js uses a file-based routing system, which means each page in your application corresponds to a file in the pages directory. The file name determines the URL of the page. For example, if you have a file called pages/about.js, the URL of the corresponding page will be /about.
Page Generation
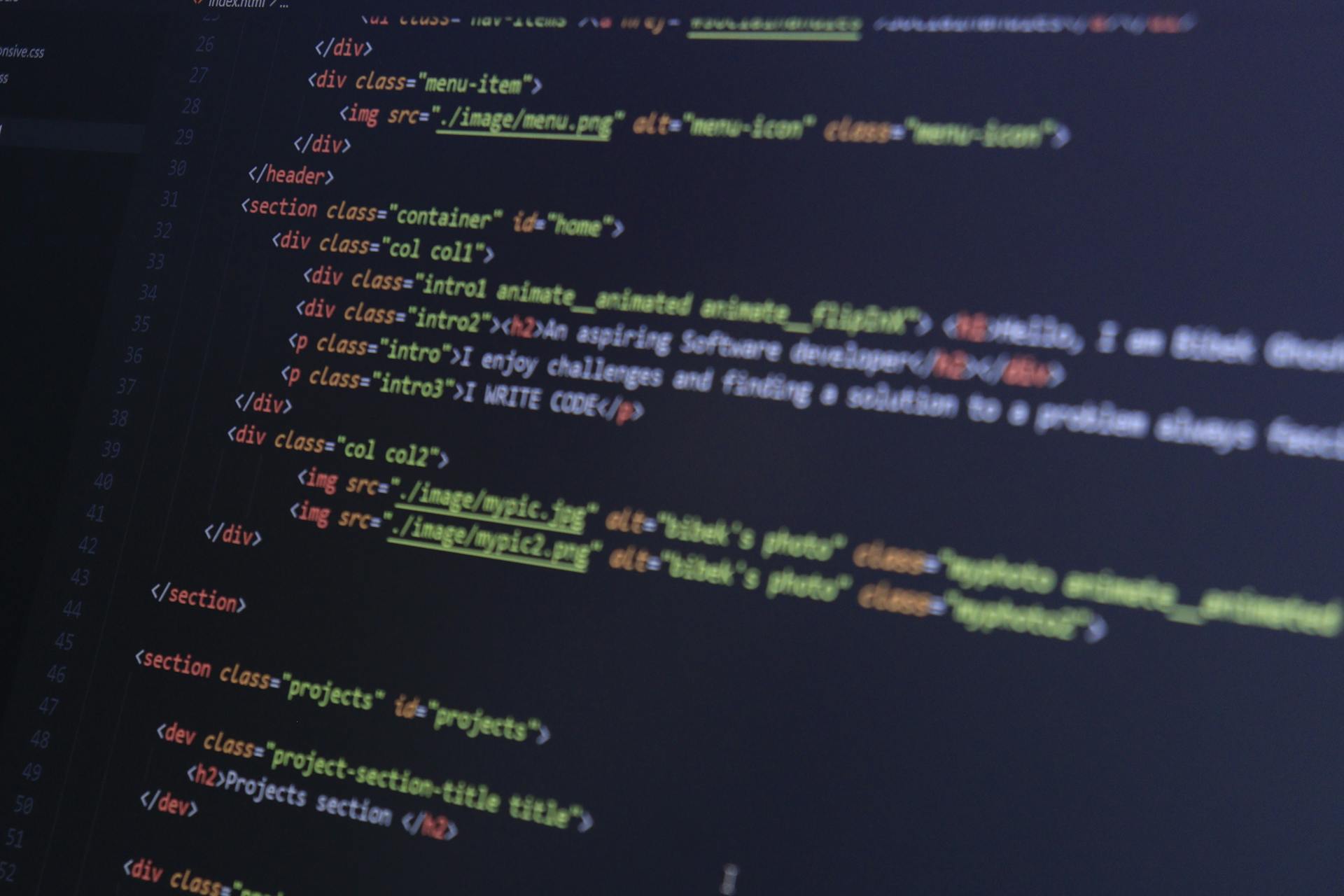
Next.js provides various methods for generating pages, including static site generation (SSG), server-side rendering (SSR), and client-side rendering (CSR). The default page generation method in Next.js is static site generation (SSG).
Static site generation (SSG) offers improved performance and better SEO, as pages are generated at build time and served as static files. To use static site generation in Next.js, you can use the getStaticProps function, which runs at build time and fetches the data needed to render the page.
The getStaticProps function is used for static site generation (SSG), where the HTML for each page is generated at build time and served as a static file. Inside the function, you can fetch data from an API or a database and return it as the props object.
Next.js also supports client-side rendering (CSR) and server-side rendering (SSR), but static site generation (SSG) is the default method. Client-side rendering (CSR) and server-side rendering (SSR) are used for different use cases, such as when the data needs to be fetched at runtime or changes frequently.
To use getStaticProps, you need to export it as an async function from your page component file. The fetched data will then be available as props in your page component, allowing you to render the content of the page.
Dynamic Routing
Dynamic routing allows you to create pages that are generated on the server or at build time, and the content can change based on user input or data from an external source.
To create dynamic pages in Next.js, you can use the dynamic routing feature, which allows you to define a page template and specify dynamic parameters in the URL.
You can create a dynamic route by creating a file in the pages directory with a name like pages/users/[name].js, where [name] is the dynamic parameter.
The [name] placeholder in the filename defines a new dynamic parameter, so that your code can identify the dynamic part of the URL as the parameter name.
The file itself should be built just like any other page: a JavaScript module that exports a React component as its default export.
To get the dynamic parameter of the route, you can use the useRouter hook from the next/router module to get a hold of the Router, which will provide you with the information you need.
Linking to a dynamic URL works like linking to any other page in Next.js, except that you have to provide two pieces of information: the URL you want to appear in the browser and the page's filename.
Here are some examples of dynamic routing:
You can access the dynamic parameters inside your page component using the useRouter hook, and they will be available as properties on the router.query object.
For example, if you have a dynamic route like pages/users/[name].js, you can access the name parameter using router.query.name.
Frequently Asked Questions
Can you use Pages router in Next 14?
Yes, you can still use the Page Router in Next.js 14, but it remains client-side, unlike the new App Router which is server-centric. The Page Router is an option for existing projects or those who prefer a client-side routing approach.
Does Next.js cache pages?
Yes, Next.js caches pages at build time to serve them faster on subsequent requests. This optimization enables faster page loads by reducing server-side rendering for repeated requests.
Sources
Featured Images: pexels.com