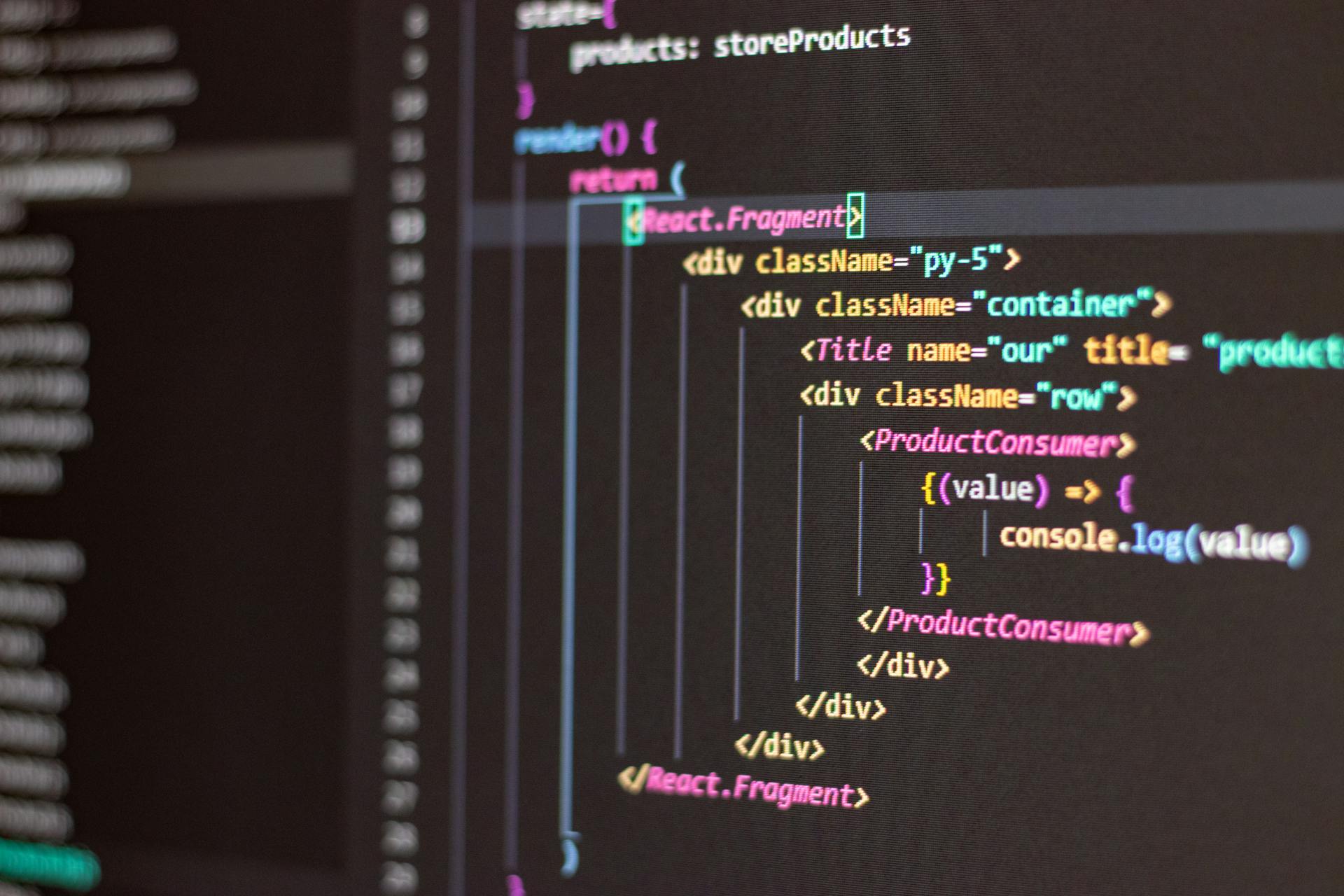
Next.js is a popular React-based framework for building server-rendered and statically generated websites and applications. In Next.js, state context allows you to share data between components without passing props down manually.
State context is a powerful tool for managing global state in Next.js applications. By using state context, you can easily share data between components without having to pass props down manually, making your code more organized and efficient.
To use state context in Next.js, you can create a context object using the createContext function from the react library. This context object can then be used to wrap your application or specific components to share state between them.
Middleware in Next.js allows you to execute code at different stages of the request-response cycle, making it a great tool for handling authentication, caching, and other tasks. By using middleware, you can keep your code clean and organized, and make it easier to manage complex logic.
Consider reading: Next Js Fetch Data save in Context and Next Route
Using State Context
You can use the Context API to access the state globally in your Next.js app. This is particularly useful when you have complex apps or need to pass down props multiple levels. The Context API lets you avoid prop drilling and access the state from anywhere in your app.
To create a context, you'll need to create a new file in a separate folder, for example, `context`. Inside, create a new file called `theme.js` and include the following code: We first created a new Context object, created a ThemeProvider function, and set the initial value for the Context to light.
You can access the state of the theme by importing the `useThemeContext` hook into your individual pages or components. This hook will allow you to access the theme state after you import it.
To access the state of the theme, navigate to `index.js` and include the following code: We first imported `useThemeContext`, then accessed the theme state and the `setTheme` function to update it when necessary.
See what others are reading: State Management in Next Js 14
You can wrap the `ThemeProvider` around the entire app by heading to the `_app.js` file and including the following code: This will let you access the state of the theme in the entire application.
The Context API is a great solution for managing global state in your Next.js app. You can create separate contexts for different states, such as authentication, user data, or theme settings.
Here are some scenarios where global state is preferable:
- User authentication status that needs to be accessed across various parts of the app.
- Theme settings or UI preferences that affect the entire application.
- Data that is fetched and should be cached and shared, like a user's profile information.
To structure your global state effectively, remember to modularize your state, ensure a single source of truth, and treat your state as immutable. This will help you maintain consistency and efficiency in your app.
You can also test your global state management logic using unit tests, integration tests, and mocking. This will ensure that your components interact with the global state correctly and that your app is reliable.
See what others are reading: Using State in Next Js
Middleware and Integration
Middleware integration in Next.js is a powerful feature for managing requests and responses throughout your application. It allows you to run code before a request is completed, giving you a flexible way to manipulate requests and responses and effectively manage global states.
Middleware shines in scenarios where you need to manage user authentication states globally, such as checking if a user is authenticated and redirecting unauthenticated users to a login page before rendering protected routes.
Middleware also enables you to manage theme settings based on user preferences or system settings, allowing you to intercept requests and modify response headers or cookies to reflect the user's preferred theme.
A different take: Nextjs Middleware Firebase Auth
Middleware Integration
Middleware integration is a powerful feature in Next.js that allows you to manage requests and responses throughout your application.
You can create a middleware.js file within the /pages or /app directory to define your middleware logic. This is where you'll run code before a request is completed.
Middleware shines in scenarios where you need to manage user authentication states globally. For instance, you can check if a user is authenticated and redirect unauthenticated users to a login page before rendering protected routes.
To do this, you can identify the route the user is trying to visit via request.nextUrl.pathname, and then use a hypothetical isUserAuthenticated() code to check if the user is authenticated.
A fresh viewpoint: Nextjs Code Block
If the user is not authenticated and attempts to access a route beginning with /protected, you can redirect them to a login page using NextResponse.redirect('/login').
Middleware also allows you to manage theme settings based on user preferences or system settings. You can intercept requests and modify response headers or cookies to reflect the user’s preferred theme.
For example, you can check for the user’s preferred theme in the cookies and fall back to 'light' if it is not found. Then, you can update the response to include the desired theme in the cookies by calling response.cookies.set('theme', preferredTheme).
Recommended read: Nextjs Cookies
Using Other Technologies
Using Other Technologies can be a game-changer for your app. You can use the React Context API alongside other state management technologies like Redux.
This hybrid approach ensures you're using the best tool for each part of your app that performs key roles. By doing so, you can create efficient and maintainable applications.
Curious to learn more? Check out: Nextjs save Data Pulled from Api Using State Context
In a Next.js application, you have the flexibility to choose from a variety of state management solutions. The native option provided by React is one of them.
The Context API is recommended for complex apps where props need to be passed down multiple levels or states need to be accessible globally.
Managing State
Managing state in a Next.js application can be a challenge, but there are solutions to help you tackle it. Ensuring that state remains synchronized throughout the application is crucial, as any changes in one component need to be reflected in other components that rely on that state.
Prop drilling, the process of passing state down through multiple layers of components, can lead to a tangled web of props and make components less reusable and harder to maintain. To avoid this, developers often use the React Context API or third-party state management libraries.
The React Context API is particularly useful for global state that needs to be accessed in many parts of your Next.js app. It's a more centralized and efficient way of managing global state, helping to avoid prop drilling and make state accessible to any component.
Broaden your view: Next Js Context
Global state should be used when multiple components need access to the same state. This includes user authentication status, theme settings, and data that is fetched and should be cached and shared. Local state, on the other hand, is suitable for data confined to a single component or a small group of closely related components.
To structure your global state effectively, modularize your state by breaking it into smaller, manageable pieces that correspond to different features or domains of your application. Ensure that any piece of data has a single place in your state tree to prevent duplication and inconsistencies.
Here are some tips for testing global state management logic:
- Unit tests should be written for actions, reducers, selectors, and any other functions that manipulate global state.
- Integration tests should be used to ensure that components interact with global state correctly.
- Mock global state when testing individual components to isolate them and test them in a controlled environment.
By following these best practices, you can ensure that your global state is easy to manage, performant, and reliable.
Testing and Debugging
Testing and Debugging is a crucial step in ensuring your Next.js app using State Context is reliable and performant. You should write unit tests for your actions, reducers, selectors, and any other functions that manipulate your global state.
Writing unit tests will help you catch bugs early on and ensure that each component works as expected. Unit tests are like a safety net that catches any errors before they become major issues.
For integration tests, use a combination of unit tests to ensure that components interact with the global state correctly. This will help you identify any issues that arise from the interactions between different components.
Mocking global state when testing individual components is a great way to isolate them and test them in a controlled environment. This will help you identify any issues that might arise from the interactions between different components.
By following these best practices, you can ensure that your global state is easy to manage, performant, and reliable.
Step-by-Step Guides
To set up context API in Next.js, you need to create a new context in a file where you can define its shape and initial value.
This new context is the foundation of your state management system, allowing you to share data across your application.
Discover more: New Nextjs Project Typescript
Create a provider component that will wrap your application and provide the context to all components within your app.
This provider component is crucial, as it enables the flow of data throughout your app.
To make the context available to all components, you need to wrap your application with the provider component in your _app.js file.
This is typically done by importing the provider component and wrapping your application with it.
To consume the context in your functional components, you can use the useContext hook provided by React.
This hook allows you to access the context and use its values in your components.
On a similar theme: Next Js Provider
Sources
- https://blog.logrocket.com/guide-state-management-next-js/
- https://www.makeuseof.com/react-context-nextjs-13-app-directory-state-management/
- https://egghead.io/lessons/next-js-make-user-state-globally-accessible-in-next-js-with-react-context-and-providers
- https://www.dhiwise.com/post/nextjs-global-state-creating-and-managing-state
- https://www.js-craft.io/blog/using-react-context-nextjs-13/
Featured Images: pexels.com