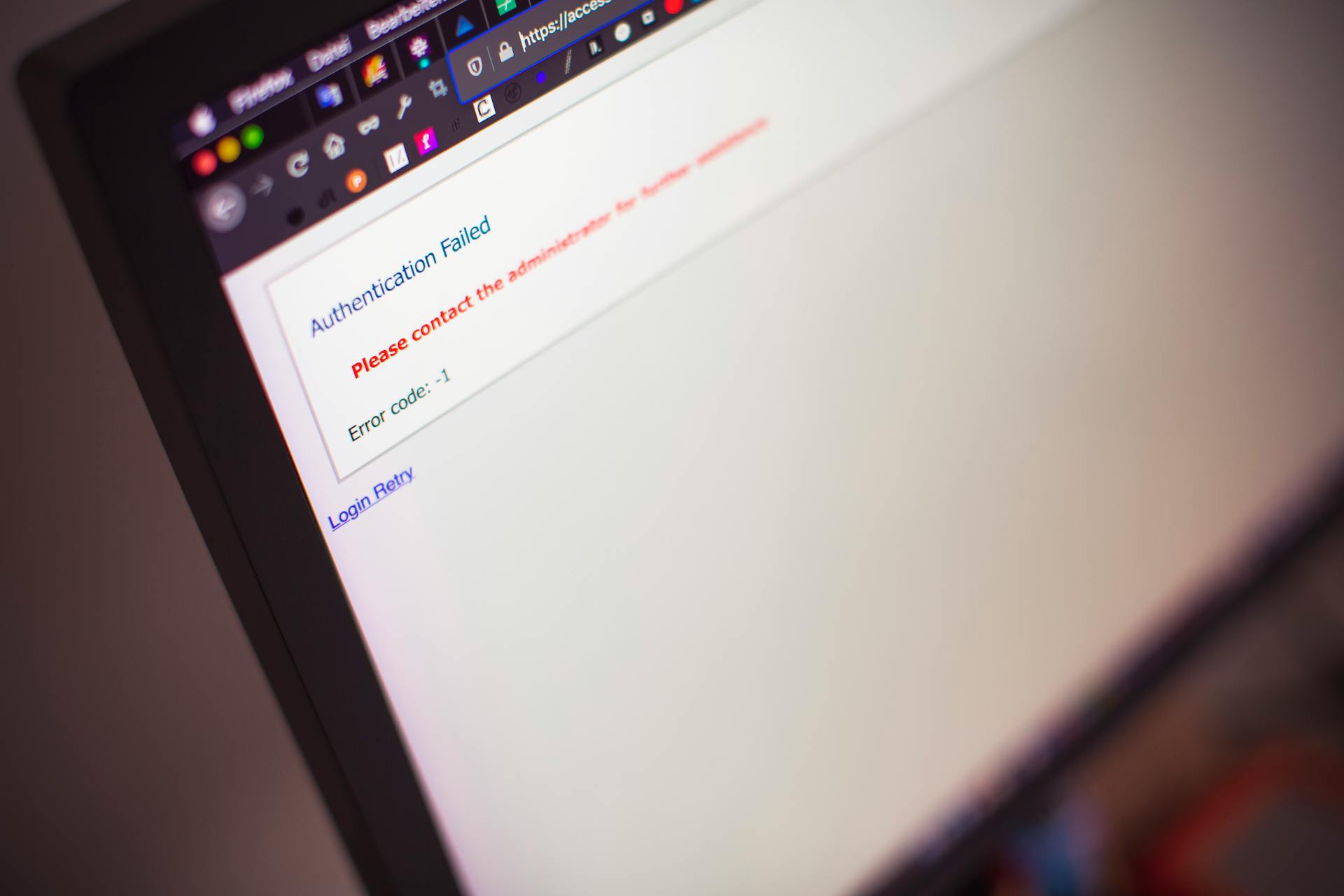
Using Next.js middleware with Firebase Auth allows for efficient server-side authorization. This approach enables developers to handle authentication and authorization in a centralized manner.
By leveraging Next.js middleware, you can create a robust authentication system that protects your serverless functions from unauthorized access. This is particularly useful for applications that require strict access control.
With Firebase Auth integrated into Next.js middleware, you can easily authenticate users and authorize access to specific routes and API endpoints. This is achieved through the use of authentication tokens and Firebase's built-in authorization mechanisms.
Next.js middleware provides a flexible and scalable solution for server-side authorization, making it an ideal choice for modern web applications.
You might enjoy: Firebase Firestore Nextjs
Setting Up Firebase Auth
Setting up Firebase Auth is a crucial step in implementing Next.js middleware Firebase Auth. You'll need to initialize Firebase Admin on the server-side, which involves sending an access token with each request from the client-side code.
To initialize Firebase Admin, you'll need to generate a private key JSON file, which contains fields like projectId, clientEmail, and privateKey. These three fields are enough to get it working.
Check this out: Auth Provider Nextjs
To integrate Firebase Authentication with your Next.js app, you can use the Express res.locals object, which will contain an authenticated Firebase App instance and the currently signed-in user. This can be accessed in getServerSideProps.
The web framework-aware Firebase deployment tooling will automatically keep client and server state in sync using cookies. This allows for seamless authentication and authorization across your app.
Here are the key steps to integrate Firebase Authentication:
- The client-side code sends an access token with each request.
- The server-side code verifies and decodes the access token using Firebase Admin.
- The server-side code extracts user information, such as the uid of the user.
Note that the server-side code will have privileged access to all Firebase resources and will ignore security rules, which are only relevant for client-side requests.
Explore further: Next Js Client Side Rendering
Implementing Auth Middleware
Implementing Auth Middleware is a crucial step in securing your Next.js application with Firebase Auth. To avoid code repetition, you can create a wrapper for your normal handler function, modifying the req and res objects as needed. This is known as middleware, and it's a powerful tool for handling page-level authentication and access control.
A fresh viewpoint: Spotify Auth Nextjs Template
Middleware in Next.js is a function that receives a NextRequest object and returns a NextResponse object or a Promise that resolves to a NextResponse object. You can use it to decide what to do next based on the request, and it can be defined inside a middleware.ts (or middleware.js) file stored on the src folder.
Here are some key benefits of using middleware for auth in Next.js:
- Restrict access to all pages unless authenticated users
- Filter middleware to only run on specific pages using the matcher
- Modify the request or response objects as needed
- Decide what to do next based on the request
By implementing auth middleware, you can create a seamless user experience and ensure that only authenticated users can access certain pages or resources. This is an essential step in building a secure and scalable Next.js application with Firebase Auth.
Expand your knowledge: Nextjs Firebase Auth
Middleware
Middleware is a powerful feature in Next.js that allows you to run server-side code before your actual page's functions.
In Next.js 12, middleware was introduced, enabling you to handle page-level authentication and access control before pages are rendered. It's a function that receives a NextRequest object and returns a NextResponse object or a Promise that resolves to a NextResponse object.
Middleware can be defined inside a middleware.ts (or middleware.js) file stored on the src folder, containing the logic for access to pages on your application. You can restrict access to all pages unless authenticated users are present or use the matcher to filter the Middleware to only run on specific pages.
One of the limitations of NextAuth.js and Middleware is that it only works with jwt tokens, not with database sessions. However, this can be revisited in the adapters section.
Next.js 13 allows you to define middleware functions inside a middleware.ts (or middleware.js) file, which is a convenient way to handle authentication and access control.
Middleware can be used to modify the request or response objects, or to decide what to do next based on the request. It's a great way to add an extra layer of security to your application.
By using middleware, you can ensure that only authenticated users can access certain pages or routes, which is especially useful for server-side resources.
To integrate middleware with Firebase Authentication, you can create a middleware.ts file in your project's root and configure it with the loginPath, logoutPath, apiKey, cookieName, and cookieSignatureKeys.
The matcher in the middleware configuration instructs the Next.js server to run the middleware against specific paths, such as /api/login, /api/logout, and /. This allows you to handle unauthenticated calls within API route handlers themselves.
On a similar theme: Nextjs Server Actions File Upload
Registration Page
Creating a registration page is a crucial step in implementing auth middleware. You'll need to define some variables and setters to hold your form state, just like we did in the registration page example.
Let's create a new page under ./app/register/page.tsx to handle user registration. The registration page should have form fields for email and password, and a confirmation field to ensure the password is entered correctly.
To validate the form submission, we'll check if the password and confirmation fields are equal. If not, we update the error state. This ensures that users enter the correct password and confirmation to proceed with registration.
The form submission logic also involves creating a user account with createUserWithEmailAndPassword from firebase/auth. If this step fails, we inform the user by updating the error state, as seen in the registration page example.
On a similar theme: Creating Shop Page Next Js
Login Page Creation
Creating a login page is a crucial step in implementing auth middleware. We create a login page under ./app/login/page.tsx, where all the magic happens.
The login page uses the signInEmailAndPassword function from firebase/auth to retrieve the user's idToken. This token is then used to call the /api/login endpoint exposed by the middleware, which updates the browser cookies with the user's credentials.
Finally, we redirect the user to the home page by calling router.push("/"). To sign out, we create a client component that uses the Firebase Client SDK to sign out from the user's account, and then calls the /api/logout endpoint exposed by the middleware.
Here are the key steps to handle redirects in Next.js apps:
- Authenticated users should be redirected to the home page when trying to access /register and /login pages.
- Unauthenticated users should be redirected to the login page when trying to access the home page.
To handle these redirects, we use the authMiddleware, which supports a number of options and helper functions. The handleValidToken function is called when a valid user token is attached to the request, and it should resolve with NextResponse.
Server Side Authorization
Server Side Authorization is a crucial aspect of securing your Next.js API routes. Authentication Middleware for Next.js API Routes makes it convenient to implement authentication as a middleware.
Suggestion: Auth Middleware Nextjs
You can create a wrapper for your normal handler function, modifying the req and res objects and returning early if errors occur. This is how I defined a withAuth middleware.
To verify and decode the access token and extract user information, you need an instance of firebase-admin on the server-side. The client-side code should send over an access token along with each request.
Here's a basic workflow for server-side authorization:
- The client-side code sends an access token with each request.
- The server-side code verifies and decodes the access token using firebase-admin.
- Based on the extracted user information, the server-side code applies logic to proceed or reject the request.
You can use the getServerSession function provided by the library to check if a user is authenticated. This function returns the session for authenticated users and null if the user is not authenticated.
Here are the steps to initialize firebase-admin:
- Generate a private key JSON file containing the projectId, clientEmail, and privateKey fields.
- Use these fields to initialize firebase-admin on the server-side.
Route Handlers and Security
Route handlers in NextAuth.js are created by importing NextAuth and the options object, and then initializing NextAuth with these options to obtain a route handler. This handler is then exported as both GET and POST to handle both types of requests.
Worth a look: Nextjs App Route Get Ssr Data
To handle server components or Route Handlers, we use the getServerSession function provided by the library, passing the same options object used to initialize NextAuth and set up the Route Handlers. This function returns the session for authenticated users or null if the user is not authenticated.
To ensure a page is only accessible to authenticated users, we use the notFound function from next/navigation, which makes sure the page is not found if the user is not logged in. This is useful for creating a secure home page that requires users to be authenticated before accessing it.
For more insights, see: Next Js Route Handlers
Role-Based Access Control
Role-Based Access Control is a method to determine if a user has the right to access a specific page or perform a particular task.
In many applications, authentication isn't enough, and we need to define roles based on authority and responsibility within the application.
We can use GitHub as an authentication provider, but since it doesn't support role management, we'll store the role for each user in the database as part of the user model.
To do this, we add a role column or field to the user model in our database, such as with Prisma, where we define that each user can only have one role.
We need to update the user profile by editing our options object to extend the profile and add a couple of callback functions to include the role in the token and session.
If we're using TypeScript, we'll have to extend the user, jwt, and session types to include the role to avoid type errors.
We can use the newly added role to define access rules on our pages, components, and middleware, and access the session on server and client components using getServerSession or useSession.
For controlling access to pages, we can use middleware, such as the example that only allows access to all pages under /admin/ to users with an "admin" role.
Recommended read: Nextjs Usecontext
Defining Route Handlers
To create route handlers for authentication in NextAuth.js, you need to create a route.ts file inside the /src/app/api/auth/[...nextauth]/ directory. This file will contain the necessary code to initialize NextAuth and obtain a route handler.
Here's an interesting read: Nextjs Project
The route handler is obtained by importing NextAuth and the options object, and then initializing NextAuth with these options. This ensures that your authentication routes are configured and ready to handle the required requests seamlessly.
You can export the handler as both GET and POST, as NextAuth will need to handle both types of requests to function properly. This is done by adding the export statements for GET and POST methods.
Here's a quick rundown of the steps to create a route handler:
- Create a route.ts file inside the /src/app/api/auth/[...nextauth]/ directory
- Import NextAuth and the options object
- Initialize NextAuth with the options object
- Export the handler as both GET and POST
By following these steps, you'll have a route handler set up to handle authentication requests in your NextAuth.js application.
Redirects, Rewrites, Headers
Redirects, Rewrites, and Headers are handled seamlessly in Next.js. The Firebase CLI respects redirects in next.config.js, converting them to their respective equivalent Firebase Hosting configuration at deploy time.
If a Next.js redirect can't be converted, it falls back and builds a function. This happens even if you're not using image optimization or SSR.
Next.js redirect, rewrite, or header configurations are respected by the Firebase CLI. They're converted to their equivalent Firebase Hosting configuration at deploy time.
The Firebase CLI can't always convert Next.js configurations to Firebase Hosting headers. In these cases, it falls back and builds a function instead.
Recommended read: Firebase Nextjs
Sources
- https://www.dingran.me/next-js-authentication/
- https://stackademic.com/blog/next-js-14-server-side-authentication-using-cookies-with-firebase-admin-sdk
- https://firebase.google.com/docs/hosting/frameworks/nextjs
- https://weknowinc.com/blog/technical-articles/authentication-and-role-based-access-control-nextjs/
- https://hackernoon.com/using-firebase-authentication-with-the-latest-nextjs-features
Featured Images: pexels.com