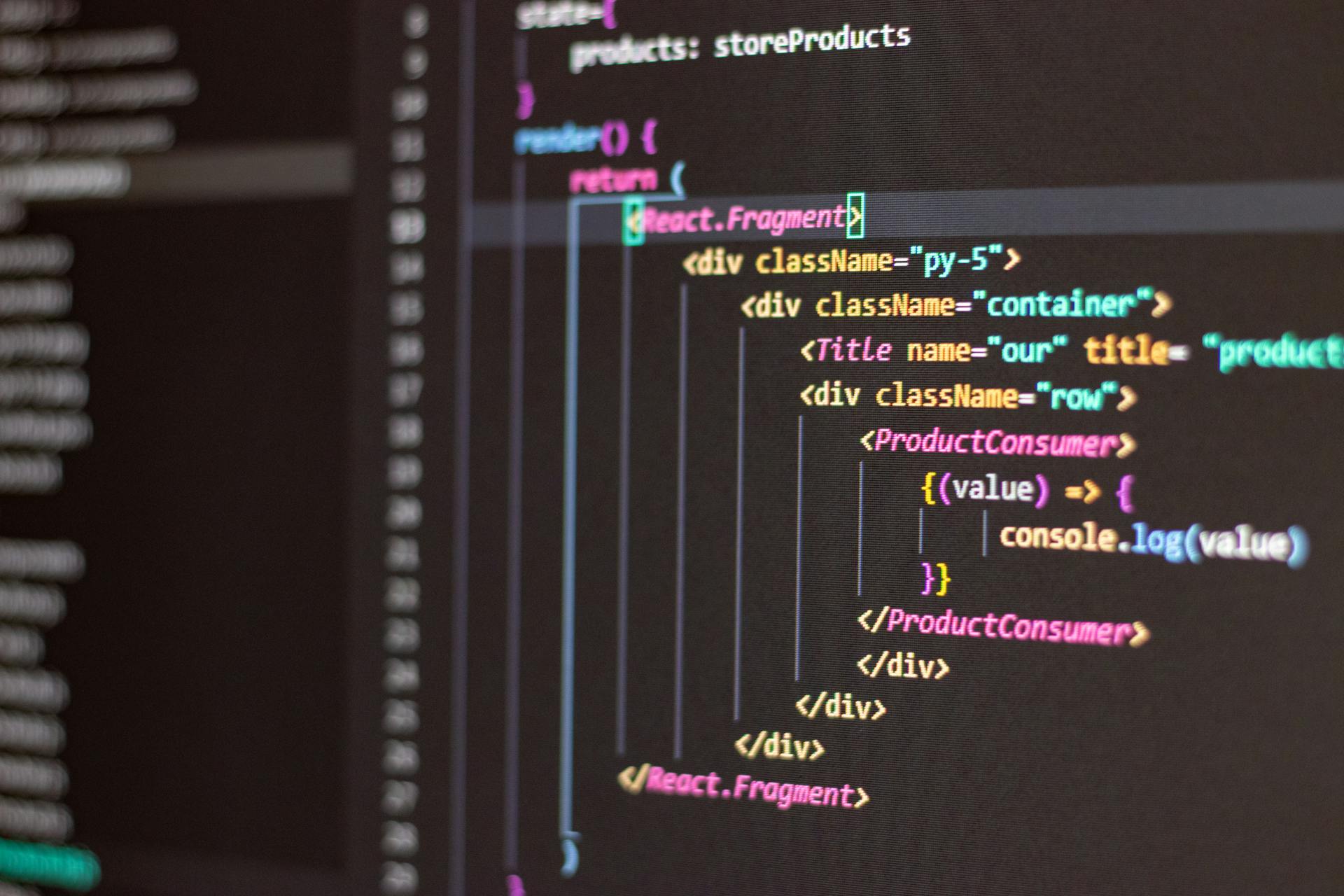
Creating a shop page with Next.js is a great way to boost your e-commerce success. With Next.js, you can create fast, scalable, and SEO-friendly shop pages that drive sales and revenue.
To start, you'll need to set up your Next.js project with the required dependencies, including the Next.js framework and a package manager like npm or yarn. As we discussed in the "Setting Up Your Next.js Project" section, this will give you a solid foundation for building your shop page.
Next, you'll want to design a visually appealing and user-friendly shop page that showcases your products in the best possible light. According to the "Designing Your Shop Page" section, a well-designed shop page can increase conversions by up to 20%.
Next.js Setup
To set up Next.js, start by opening a terminal and typing the command `npx create-next-app --typescript`. This will install all project dependencies and create files and folders.
The `--typescript` option sets up a Typescript project, which helps prevent runtime errors and allows for better refactoring.
Run `npm run dev` to serve your app at localhost:3000. Your server-side rendered Next.js e-commerce store should now be ready to go.
For more insights, see: How to Run Next Js App
Setting Up the Environment
To set up the development environment for Next.js, you'll want to start by opening a terminal and typing the command `npx create-next-app --typescript`. This will install all the project dependencies and create files and folders.
The `--typescript` option is a good choice, especially for beginners, as it sets up a Typescript project which can help prevent runtime errors and make refactoring easier in the long run.
Next, run `npm run dev` to serve your app at localhost:3000.
Explore further: Next Js New Project
Why Next.js?
Next.js has risen to prominence as an e-commerce platform, owing to its uncomplicated yet potent features. This framework, constructed upon the popular front-end library React, is equipped with a robust build instrument named “create-react-app,” making single-page applications (SPA) creation a breeze.
With Next.js, you can easily build a server-side or statically rendering app, which implements many implementation details for you. This abstracts other details such as app-bundling and transcompilation, giving you more time to focus on creating a great shopping UX.
Worth a look: React + Next Js
React’s virtual DOM provides a more efficient way of updating the view in a web application, and Next.js features like server-side rendering and static exporting push these benefits even further. This guarantees that your website/app will be SEO-friendly, which is vital to any e-commerce business.
The support for code splitting in Next.js facilitates the delivery of only essential content for the current page, making it an efficient choice for managing vast data volumes. This is especially useful when scaling and expanding your shopping cart integration.
Components and Layout
Creating a shop page in Next.js requires careful consideration of its components and layout.
The shop page will need a layout component that wraps around the main content. This component will likely be a container with a specific width and height.
The main content will be a list of products, which will be rendered using a map function. This function will iterate over an array of product objects, rendering each product as a separate item.
Each product item will have its own layout, including a title, description, price, and image.
The image will be a separate component that handles the image upload and display. This component will be reusable throughout the shop page.
The shop page will also need a header and footer component, which will be shared across all pages. These components will be imported and used in the shop page layout.
Customization and Styling
Customization and Styling is a crucial part of creating a visually appealing shop page. To keep things modular and maintainable, we'll create different components to display information about our store and products.
We'll use CSS modules to style our components, which can be especially handy if our CSS file gets large. To use CSS modules, we simply create a file respecting the [name].module.css convention, for example, Product.module.css or Product.module.scss.
By importing the CSS file as a styles object in the component's file, we can access the styles with it. This will help us style our website in a more organized and efficient way.
Worth a look: Next Js Cookies
Customizing Your
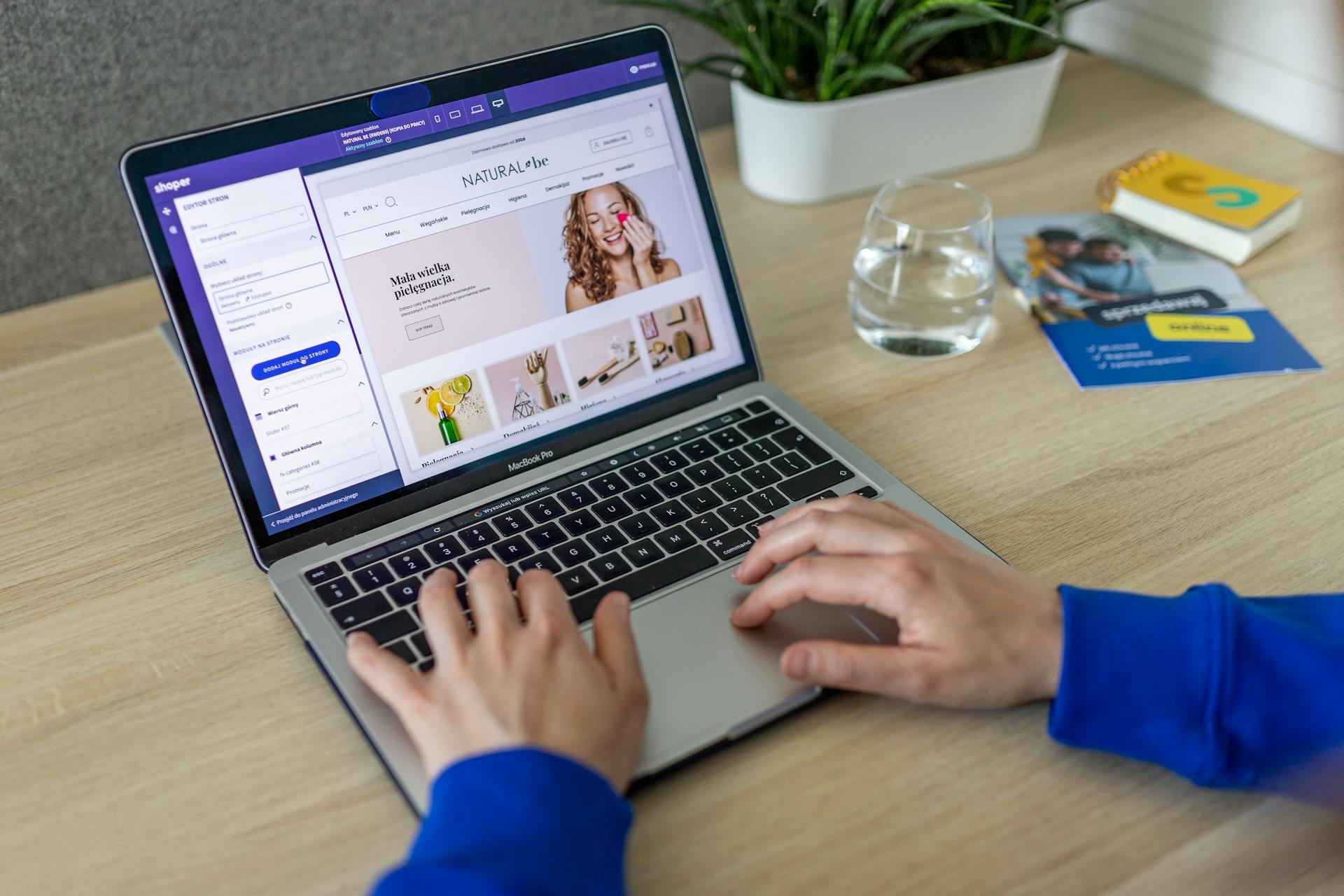
Customizing your website can make all the difference in how appealing it is to your customers. We'll create separate components to display store information and product details, keeping things modular and maintainable.
To assemble these components, we'll think about how to display them in a way that makes sense for our website. This will help us create a clear and organized layout.
Breaking down our website into smaller, manageable parts makes it easier to add or remove features as needed. This flexibility is key to creating a website that meets our evolving needs.
As we style our website, we'll consider the overall aesthetic and how each component contributes to the bigger picture. We want our website to be visually appealing and easy to navigate.
You might enjoy: Create React App vs Next Js
Setting Up CSS Modules
Setting up CSS modules for your Next.js project can be a game-changer, especially when your CSS file gets too large.
To use CSS modules, create a file following the [name].module.css convention, such as Product.module.css.
This convention is crucial, as it allows Next.js to recognize the file as a CSS module.
You can then import it as a styles object in the component's file and access the styles with it.
For example, you can import Product.module.css like this: import styles from './Product.module.css';
Expand your knowledge: File Upload Next Js Supabase
Data and API
To populate your shop page with products, you'll need to pre-render the data. Next.js offers two ways to do this: getStaticProps, which fetches data at build time, and getServerSideProps, which fetches data on each request.
You can use getStaticProps to decrease loading time by saving the user a request, especially if the product data doesn't change often. This method is suitable for our use case.
To import products from an e-commerce API, you can define an API with Cosmic JS, a Content Management System that facilitates the creation of tailor-made APIs. This allows you to import products directly into your Next.js application from the API.
A unique perspective: Next Js Build Collecting Page Data
Pre-Rendering Data and Components
Pre-rendering data and components is a crucial step in optimizing the performance of your application. This involves populating your products to the ProductList component, which can be achieved through various methods.
You can use React's useEffect lifecycle, but it won't get called on the server during static or server-side rendering. Thankfully, Next.js provides two alternatives: getStaticProps and getServerSideProps.
getStaticProps fetches data at build time, which is ideal for our use case since the product doesn't change often. This method decreases loading time by saving the user a request.
Intriguing read: Nextjs Use Server
Integrate Contentful and Fetch Data
To integrate Contentful and fetch data, you can use the Contentful GraphQL Content API, which can be accessed using the axios library.
The first step is to install axios by opening the terminal and running the command npm install axios.
Next, create a new folder called lib for some helper functions, and inside the folder, create an api.js file where you'll write the functions to fetch data via the Contentful GraphQL Content API.
You can use the following code as a reference to get an idea of what it's doing.
As you create your API, you'll need a Product component to show on your homepage, which will output whatever information you need to display about your product.
This component will require an IProduct interface that matches Snipcart's product definition and an IProductProps interface to define the types of your props.
Underneath the interface, add this component, which will be used to display your product information.
A fresh viewpoint: Next Js Fetch Data save in Context and Next Route
To pre-render your data and import components, you can use Next.js' getStaticProps method, which fetches data at build time, or getServerSideProps, which fetches data on each request.
In this case, since the product doesn't change often, you'll use getStaticProps to decrease loading time by saving the user a request.
You can do so by adding the following code inside the Index function return clause.
After establishing an e-commerce API through Cosmic JS, you can import products from this API into your Next.JS application using the GraphQL API endpoint.
To add a new product, simply select 'Create GraphQL API', copy the GraphQL API endpoint, and paste it into your Next.JS application, and then import the products using the GraphQL syntax.
Readers also liked: Using State in Next Js
Pages and Routing
To create dynamic route pages for products, we'll use the `@contentful/rich-text-react-renderer` package to parse the text content in the product description field, which is a rich text field in Contentful.
We'll create a page in `pages/products/[slug].js` with an add-to-cart form inside. This page will need to define a list of paths that have to be rendered to HTML at build time.
We'll export an async function called `getStaticPaths` from the page, which will statically pre-render all the paths specified by `getStaticPaths`. This is required for pages with dynamic routes that use `getStaticProps`.
On a similar theme: Next Js Dynamic
Functionality and Features
In Next.js, you can create a shop page with a wide range of functionality and features. The page can be built using various components, such as the `ShopPage` component, which can be created using the `getStaticProps` method.
The `ShopPage` component can be used to display a list of products, with each product having its own details, including price and description. This is achieved by using the `map` function to iterate over the products array and render each product as a separate component.
To handle product filtering, you can use the `useState` hook to store the filtered products in the component's state. This allows the user to filter products by category or price, making it easier to find the products they're looking for.
You might enjoy: Nextjs Usecontext
Add Review Functionality
Adding review functionality to your website is a great way to engage users and improve customer satisfaction. Next.JS provides several options for adding this feature.
You can use the "Comment" component to place a comment box underneath each product, allowing users to share their thoughts and opinions. This feature is particularly useful for encouraging user-generated content.
Explore further: Next Js Feature Flags
The "Vote" component is another option for creating user review features. It enables the creation of a user entry form with fields for user names and ratings, and Next.JS automatically retrieves data from users.
The "Like" component can also be used to allow users to rate products, making it easy to collect feedback and improve your offerings. By incorporating these features, you can create a more interactive and engaging experience for your users.
Add-to-Cart Form
The add-to-cart form is a crucial element on product pages. It allows customers to easily purchase items by passing product details to the cart.
To create a functional add-to-cart form, you need to add the action and method attributes to the form element. This is typically done using HTML.
The form should also include elements to pass product details to the cart, such as product ID, quantity, and price. This ensures that the customer's order is accurately reflected in their cart.
A well-designed add-to-cart form can significantly improve the user experience, making it easier for customers to make a purchase.
For your interest: Nextjs Form Component
Sources
- https://snipcart.com/blog/next-js-ecommerce-tutorial-example
- https://egghead.io/lessons/apollo-create-dynamic-next-js-shop-page-to-add-products-for-a-shop-with-apollo-usemutation
- https://www.gmihub.com/blog/ecommerce-store-with-next-js/
- https://developer.mozilla.org/en-US/blog/static-site-generation-with-nextjs/
- https://www.foxy.io/blog/build-a-jamstack-ecommerce-website-with-next-js-contentful-and-foxy/
Featured Images: pexels.com