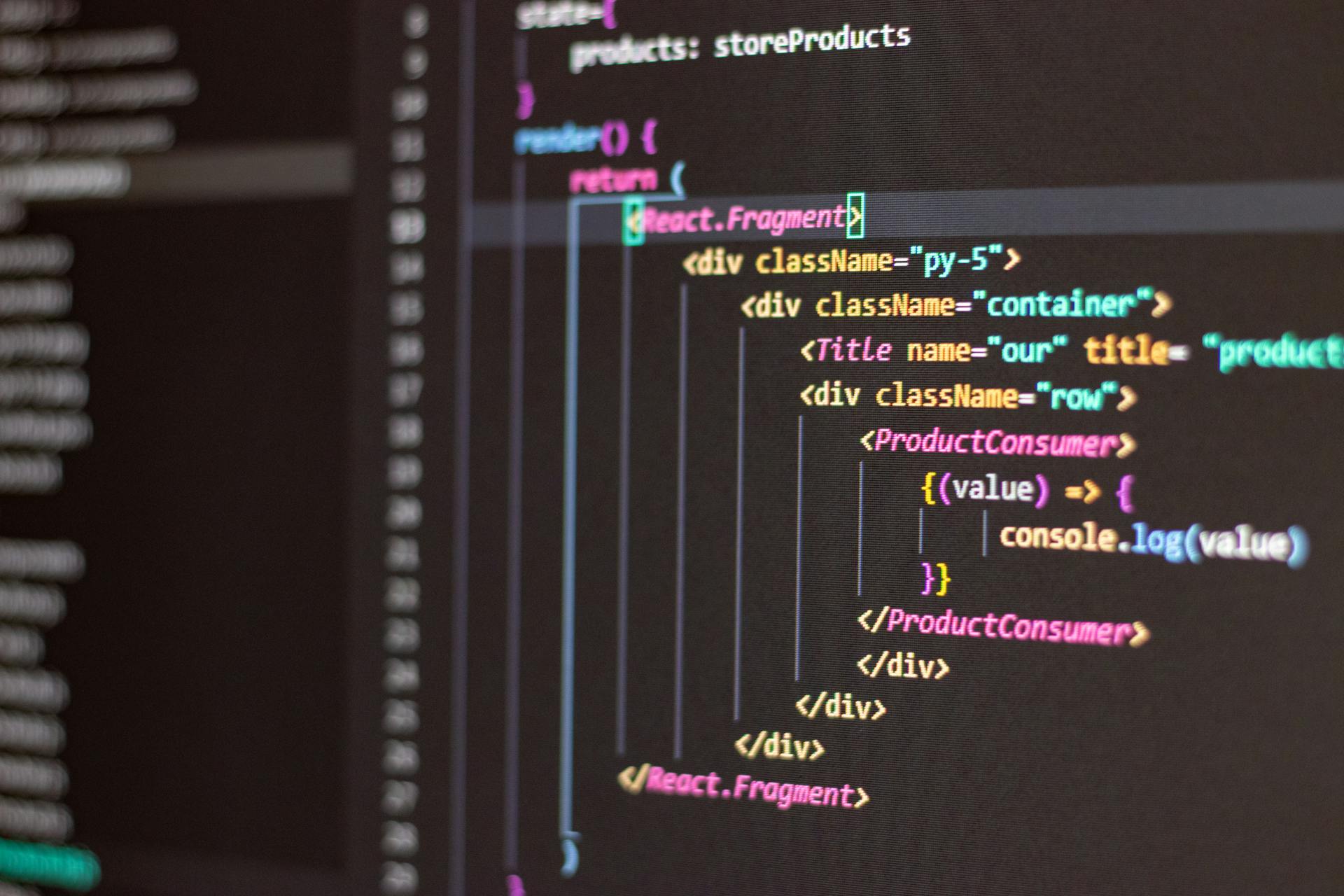
Configuring and managing Next JS feature flags can be a bit tricky, but with the right approach, it can be a game-changer for your development workflow.
To start, Next JS feature flags are enabled by default, but you can disable them by setting the `experimental.featureFlags` option to `false` in your `next.config.js` file. This will prevent feature flags from being used in your application.
Feature flags can be configured using the `next.config.js` file, where you can define different flags for different environments, such as development, staging, and production. For example, you can use the `env` option to specify a flag for a specific environment.
Feature flags can be toggled on and off using the `use` function, which is a built-in function in Next JS that allows you to conditionally render components based on feature flags.
For another approach, see: Next Config Js
Configuring Feature Flags
Configuring feature flags is a crucial step in implementing feature flags in your Next.js app. You can define feature flags across multiple environments or branches.
To configure your feature flags, you can use a tool like ConfigCat, which provides multiple ways to target your users with feature flags. You can target a certain percentage of your traffic allocation or through user segmentation.
You can also use a library like next-flag, which allows you to enable or disable features by ticking a checkbox in a GitHub issue. This library also supports React Server Side and Client Side Components, powered by the NextJS Cache.
To define custom conditions for your feature flags, you can use the requestToContext function, which takes a request object and returns a context object. This context object is then passed to the condition functions, which evaluate the conditions at runtime.
Here's an example of how you can define conditions for your feature flags:
You can also use the isFeatureEnabled method to check if a feature is enabled in your app. This method takes a feature string or array of strings and returns a promise that resolves to a boolean value.
Worth a look: Azure App Config Feature Flag
The isFeatureEnabled method has an options parameter that allows you to define extra in-line conditions that must be met for the feature to be enabled. This can be useful for complex feature flag logic.
By following these steps, you can configure your feature flags and start implementing feature flags in your Next.js app.
Static Site Generation (SSG)
Static Site Generation (SSG) is a powerful tool for Next.js developers.
With Next.js, you can resolve Unleash feature flags when building static pages, giving you more flexibility and control over your website's content.
This approach also works for ISR (Incremental Static Regeneration), allowing you to update specific parts of your static site without rebuilding the entire thing.
You can access the same features as in the client-side example, making it a seamless integration.
By leveraging SSG, you can optimize your website's performance and improve user experience.
Using SSG with Next.js and Unleash feature flags can be a game-changer for your website's development and maintenance.
Worth a look: Next Js Static
Server-Side Features
Server-Side Features are a game-changer for Next.js applications.
In short-lived serverless environments like Vercel, server-side metrics reporting can be challenging due to the lack of persistent in-memory cache across multiple requests.
To address this, the Unleash SDK provides a sendMetrics function that can be called wherever needed, but it should be executed after feature flag checks client.isEnabled() or variant checks client.getVariant(). This ensures that metrics are reported accurately.
Here are some key benefits of Server-Side Features in Next.js:
- Enable or disable features by ticking a checkbox in a GitHub issue.
- Define feature flags across multiple environments or branches.
- Supports React Server Side and Client Side Components.
- Define custom conditions that are evaluated at runtime to enable or disable features.
- Can be deployed as a stand-alone service to manage feature flags for multiple NextJS apps.
Middleware
Middleware is a powerful tool for managing feature flags in Next.js applications. It allows you to run the SDK in Next.js Edge Middleware, which is a great use case for A/B testing.
You can transparently redirect users to different pages based on a feature flag, and target pages can be statically generated, improving performance. This is a game-changer for large-scale applications.
To implement middleware, refer to the example in ./example/README.md#Middleware. Both getDefinitions() and getFrontendFlags() can take arguments overriding URL, token, and other request parameters.
Here's a quick rundown of the arguments you can pass to these functions:
By using middleware, you can take your feature flag management to the next level and create more engaging user experiences.
Server-Side Metrics
Serverless environments like Vercel, where Next.js is commonly hosted, can create challenges for server-side metrics reporting due to the lack of a persistent in-memory cache across multiple requests.
Typically, Unleash backend SDKs run in long-lived processes, allowing them to cache metrics locally and send them to the Unleash API or Edge API at scheduled intervals.
However, in short-lived serverless environments, metrics must be reported on each request.
To address this, the SDK provides a sendMetrics function that can be called wherever needed, but it should be executed after feature flag checks.
Setting up the Edge API in front of your Unleash API is also recommended to protect your Unleash API from excessive traffic caused by per-request metrics reporting.
For another approach, see: Next Js Serverless
Next.js Integration
Next.js integration is a breeze with feature flags. You can integrate feature flags into your Next.js project using a library like Flagsmith, which offers a user-friendly interface for managing feature flags, A/B testing, and segment overrides.
To integrate Flagsmith into your Next.js project, you can follow a simple guide that includes steps such as installing the Flagsmith SDK via NPM and importing it into your root index.js component. This will allow you to control feature behavior without modifying code.
Using feature flags in Next.js also allows you to perform phased rollouts, control feature visibility based on user segments, and dynamically change feature settings in real-time without redeploying the application. This is made possible by integrating feature flags into your Next.js project using a library like Flagsmith.
Here are some popular libraries for integrating feature flags into Next.js projects:
- Flagsmith
- ConfigCat
- NextFlag
These libraries provide a range of features, including feature flagging, A/B testing, and segment overrides. They can be integrated into your Next.js project using a simple SDK or API.
Vercel Integration
You can set up a Vercel account in just a few minutes. Vercel account setup is a straightforward process.
Consider reading: Nextjs by Vercel Meaning
To deploy a Next.js application, you'll need to create a basic Next.js application with Vercel Approuter. This will allow you to deploy your application with ease.
Here are the steps to deploy your application:
1. Set up Vercel and push the project to Vercel
2. Deploy the application
By following these steps, you'll be able to deploy your Next.js application in no time.
With Vercel and Next.js, you can build and deploy a config-controlled application from scratch in less than 15 minutes.
Here's an interesting read: Next Js Spa
Multiple Environments
Working with multiple environments in Next.js is a breeze thanks to the NextFlag package. By default, it will read process.env.NEXT_PUBLIC_VERCEL_ENV or other environment variables to determine the current environment.
You can customize this process by passing a getEnvironment function to the NextFlag constructor, giving you more control over how the environment is determined.
To associate a feature with a specific environment, simply add a subheading to the feature issue with the name of the environment (case-insensitive).
For more insights, see: Next Js Software Staging Environment Aws Amplify Gen 2
Here's a quick rundown of how environment-specific flags work:
Remember, the top-level feature flag will control whether the feature is enabled or disabled in all environments. If it's disabled, the feature will be disabled everywhere. If it's enabled, the environment-specific flags will determine whether the feature is enabled in each environment.
Intriguing read: Nextjs Build Fails Environment Variables
Next.js Integration
To integrate feature flags into your Next.js application, you can use a library like Flagsmith, which offers a user-friendly interface for managing feature flags, A/B testing, and segment overrides.
Flagsmith provides a powerful tool for managing complex projects with multiple feature variations, allowing you to control feature behavior without modifying code.
You can integrate Flagsmith into your Next.js project deployed on Vercel, gaining the ability to perform phased rollouts, control feature visibility based on user segments, and dynamically change feature settings in real-time without redeploying the application.
To integrate feature flags into your Next.js application, you can use a library like ConfigCat, which provides a JavaScript (SSR) SDK that you can install via NPM.
Check this out: 'use Server' Nextjs
Here are the steps to integrate ConfigCat into your Next.js application:
- Install the ConfigCat JavaScript (SSR) SDK via NPM
- Import the installed package into your root index.js component
- Refactor your getServerSideProps function to initialize the package using the SDK key copied from the dashboard
- Refactor the template to only render the feature if the feature flag is toggled on
Some popular libraries for feature flags in Next.js include Flagsmith, ConfigCat, and next-flag, which provides feature flags powered by GitHub issues and NextJS.
Here are some key functions and methods to keep in mind when working with feature flags in Next.js:
- useNextFlag: A hook that returns the loading state, features, error, and isFeatureEnabled function
- NextFlagProvider: A function that returns an element or null
- isFeatureEnabled: A method that returns a promise indicating whether a feature is enabled
- getFeatures: A method that returns the features
- POST: A method for updating feature flags
When working with feature flags, it's essential to consider the importance of feature flags, how to create a basic Next.js application with Vercel Approuter, and how to deploy the application.
Here are some key points to keep in mind:
- Feature flags provide a way to control feature behavior without modifying code
- Flagsmith and ConfigCat are popular libraries for feature flags in Next.js
- Next-flag provides feature flags powered by GitHub issues and NextJS
- useNextFlag and NextFlagProvider are key functions for working with feature flags in Next.js
Sources
- https://docs.getunleash.io/reference/sdks/next-js
- https://dev.to/kylessg/implementing-feature-flags-with-nextjs-and-app-router-1gl8
- https://vercel.com/blog/flags-as-code-in-next-js
- https://github.com/TimMikeladze/next-flag
- https://daveyhert.medium.com/implementing-feature-flags-in-a-next-js-application-ad3ed881aa83
Featured Images: pexels.com