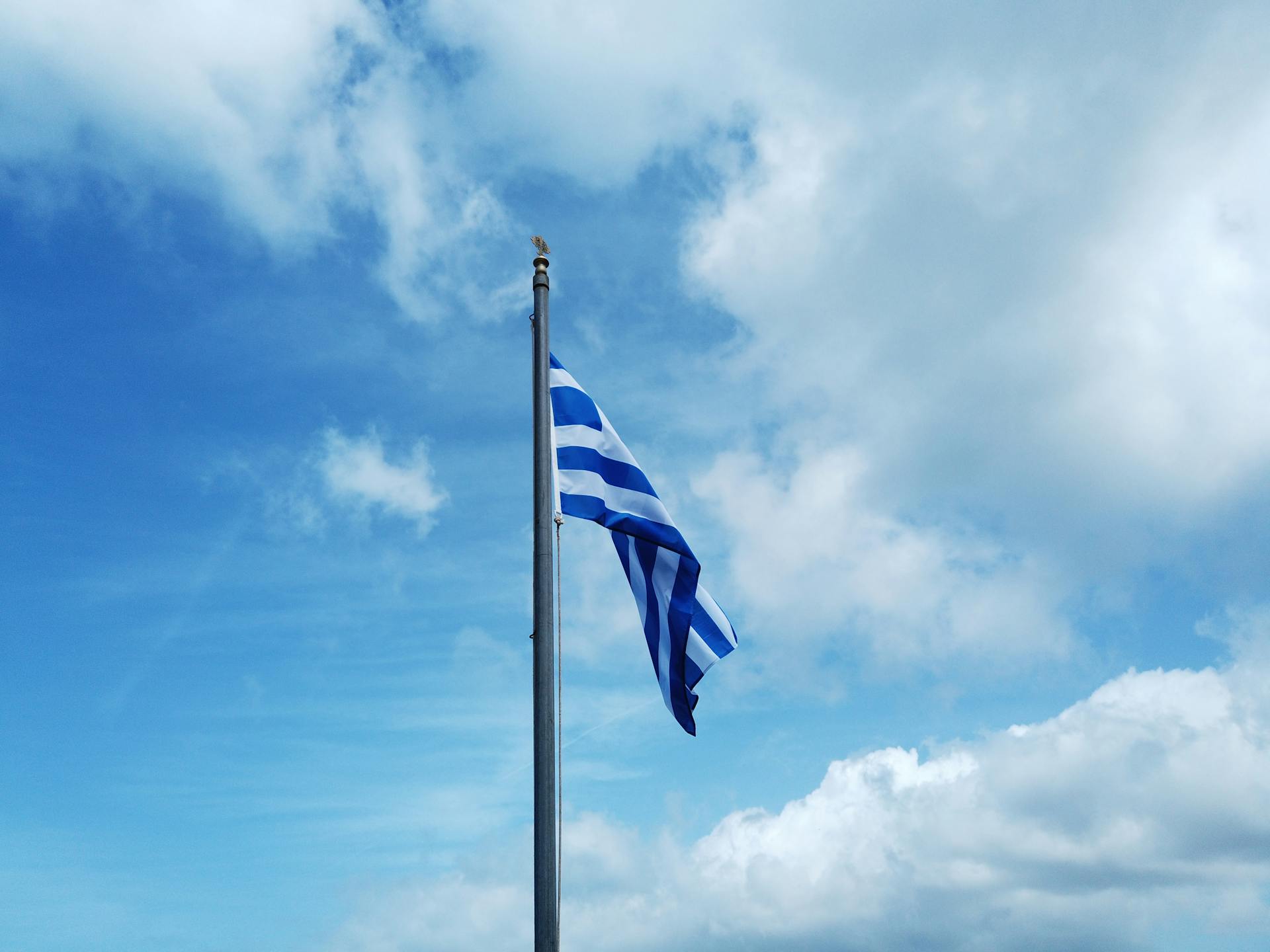
Azure feature flags are a powerful tool for managing and deploying features in your applications. They allow you to control which features are enabled or disabled, without changing the underlying code.
Feature flags can be used to roll out new features to a subset of users, helping to test and refine them before making them available to everyone. This approach reduces the risk of introducing bugs or breaking existing functionality.
Azure feature flags can be integrated with Azure DevOps, making it easy to manage and track changes to your feature flags. This integration also enables you to automate the deployment of your feature flags, streamlining your development process.
By using Azure feature flags, you can improve the efficiency and reliability of your application deployments.
Intriguing read: Azure Devops Epic Feature
Getting Started
To start using feature flags with Azure App Configuration, you'll want to head to the quickstarts specific to your application's language or platform.
If you're building an ASP.NET Core application, you can find the relevant quickstart there.
For a .NET background service, you'll need to follow a different set of steps, which can be found in the quickstarts as well.
Azure Kubernetes Service is another platform where you can find the necessary information to get started with feature flags.
Azure Functions is also supported, and you can find the corresponding quickstart to learn more.
Configuring Azure Feature Flags
To configure Azure Feature Flags, you'll need to start by enabling the feature flag upon creation. This is done by checking the box next to "Enable feature flag" when creating a new feature flag.
The feature flag name, which is used to reference the flag in your code, must be unique within an application. You can also use the key to filter feature flags that are loaded in your application, and you can add a prefix or a namespace to group your feature flags.
You can leave the label empty, as it's not required to create a feature flag. However, you can use labels to create different feature flags for the same key and filter flags loaded in your application based on the label.
On a similar theme: Next Js Feature Flags
Before diving into the world of Azure Feature Flags, ensure you have an active Azure account and subscription, as well as an existing Azure App Configuration store.
To navigate to the Visual Studio menu and select File > New > Project, you'll need to have Visual Studio installed on your machine.
You can export the connection string of your app configuration store by using the following code: `export ConnectionString='connection-string-of-your-app-configuration-store'`.
To configure the Azure extension, you'll need to navigate to your Azure DevOps project dashboard, click Project Settings, and then navigate to Pipelines, then Service Connections.
Here's a step-by-step guide to creating a service connection for LaunchDarkly:
- Navigate to your Azure DevOps project dashboard. Click Project Settings.
- Navigate to Pipelines, then Service Connections.
- Click Create service connection and select LaunchDarkly.
- Enter your LaunchDarkly API Access token.
- Enter a Service connection name.
- (Optional) Enter a Description.
- Click Save.
This will allow you to use the LaunchDarkly API in your Azure DevOps project.
Managing Variants
Managing Variants is a crucial step in harnessing the power of Azure Feature Flags. You can add variants by selecting Variant feature flag in the Feature manager.
To create a new variant, you need to provide a name and a value. The name must be unique within a feature flag, and you can choose from two variants by default, which are Off and On. You can also edit the value in a JSON editor for more complex configurations.
The default variant is the one that will be returned when no variant is assigned to an audience or the feature flag is disabled. You can choose the default variant from the dropdown list, and it will be displayed with the word Default next to it.
To allocate traffic across each variant, you need to distribute it so that it adds up to exactly 100%. You can also select the options Override by Groups and Override by Users to assign variants for select groups or users.
Here's a summary of the steps to allocate traffic:
- Distribute traffic across each variant, adding up to exactly 100%
- Optionally select Override by Groups and Override by Users to assign variants for select groups or users
- Optionally select Use custom seed and provide a nonempty string as a new seed value
- Select Review + create to see a summary of your new variant feature flag, and then select Create to finalize your operation
Troubleshooting and Optimization
Azure feature flags can be tricky to set up, but don't worry, it's not rocket science. You can troubleshoot common issues by checking the flag's status in the Azure portal.
If a flag is not working as expected, try refreshing the flag's configuration to see if it resolves the issue. This can be done by clicking on the flag's toggle button in the Azure portal.
A fresh viewpoint: Azure Developer Portal
Azure feature flags can also be optimized for better performance by using caching. Caching can reduce the load on the Azure platform and improve the overall user experience.
To optimize caching, you can use Azure's built-in caching mechanisms, such as Azure Cache for Redis. This can help reduce latency and improve the speed of your feature flags.
By following these troubleshooting and optimization tips, you can ensure that your Azure feature flags are working smoothly and efficiently.
Integration and Setup
To integrate Azure Feature Flags, you'll need an active Azure account and subscription, as well as an existing Azure App Configuration store.
You can start by navigating to the Visual Studio menu and selecting File > New > Project. Then, make sure to add the necessary NuGet packages, including Microsoft.Extensions.Configuration.AzureAppConfiguration and Microsoft.FeatureManagement.
To configure the App Configuration provider, create a new file named "Startup.cs" and inject Azure App Configuration services and feature manager through dependency injection. This involves adding code that looks like this: public override void ConfigureAppConfiguration(IFunctionsConfigurationBuilder builder) { builder.ConfigurationBuilder.AddAzureAppConfiguration(options => { options.Connect(Environment.GetEnvironmentVariable("ConnectionString")) .Select("_") .UseFeatureFlags(); }); }
Here are the essential tools and steps to get started:
- Active Azure account and subscription
- Existing Azure App Configuration store
- Navigating to the Visual Studio menu and selecting File > New > Project
- Adding necessary NuGet packages
- Configuring the App Configuration provider in the "Startup.cs" file
App Store Integration
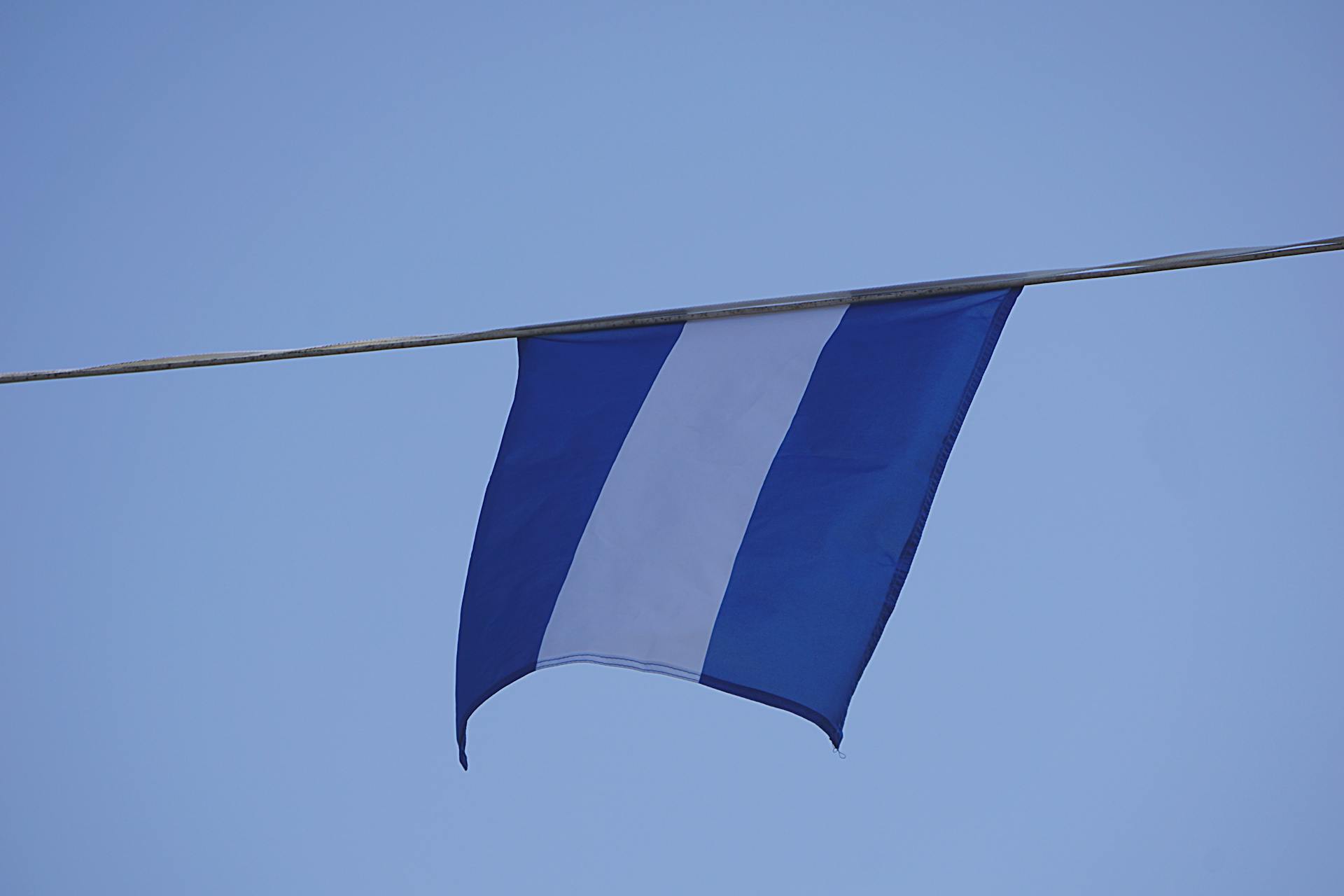
To integrate Azure App Configuration into your project, start by right-clicking your project and selecting "Manage NuGet Packages." Then, add the necessary NuGet packages, including Microsoft.Extensions.Configuration.AzureAppConfiguration and Microsoft.FeatureManagement.
You'll need to create a new file named "Startup.cs" to configure the App Configuration provider. This is where you'll inject Azure App Configuration services and feature manager through dependency injection.
To configure the App Configuration provider, use the code snippet: public override void ConfigureAppConfiguration(IFunctionsConfigurationBuilder builder) { builder.ConfigurationBuilder.AddAzureAppConfiguration(options => { options.Connect(Environment.GetEnvironmentVariable("ConnectionString")) .Select("_") .UseFeatureFlags(); }); }
You'll also need to add Azure App Configuration services and feature management through dependency injection using the code snippet: public override void Configure(IFunctionsHostBuilder builder) { builder.Services.AddAzureAppConfiguration(); builder.Services.AddFeatureManagement(); }
Explore further: Azure App Insights vs Azure Monitor
Adding to Spring Boot
In order to consume the feature toggle in your Spring Boot application, you'll need to add some specific settings to your bootstrap.properties file. This includes adding a "label-filter" that matches the profile of your Spring Boot application with the proper environment-related toggle to be loaded, as well as an "enabled" property to load the feature toggles as config.
To check the status of a toggle, you can use a FeatureManger instance. This allows you to determine whether a toggle is turned on or off.
Here are the specific settings you'll need to add to your bootstrap.properties file:
- label-filter
- enabled
Security and Access
Security and Access is a top priority when working with Azure Feature Flags. To ensure the security of your feature flags, you can create a custom role and add it to an API access token.
To create a custom role, click the gear icon to view Organization settings, then click Roles, and finally click Create role. This will open the "Create custom role" panel where you can give the custom role a human-readable name.
Granting the right permissions is crucial. You should grant write access to the environments and feature flags relevant to your Azure DevOps projects.
Here's a step-by-step guide to creating a custom role:
- Click the gear icon to view Organization settings.
- Click Roles.
- Click Create role. The "Create custom role" panel appears.
- Give the custom role a human-readable name.
- Grant write access to the environments and feature flags relevant to your Azure DevOps projects.
- Click Save role.
Strategies
When working with Azure Feature Flags, you have several strategies to consider.
Dynamic Configuration allows you to change feature toggle states without redeploying the application, providing more flexibility and control. You can use push based or pull based approaches to read toggle from cloud.
Static Configuration involves configuring feature toggles at the time of deployment or release. This is done by defining the state of a feature toggle (enabled or disabled) in a configuration file, environment variable, or deployment script.
To manage feature toggles across different environments, you can use labels. For example, you can create a feature flag named “demo-search” under Feature Manager and label it with a value like “prod” to represent the production environment.
Here are the main strategies to consider:
- Dynamic Configuration
- Static Configuration
Remember, you can find a complete example of how to implement these strategies in the article references.
Frequently Asked Questions
What are feature flags used for?
Feature flags enable you to turn features on or off without changing the code or deploying new software, allowing for flexible and controlled releases. This helps developers quickly test, roll out, and manage new features in a controlled environment.
Where are Azure feature flags stored?
Azure feature flags are stored in your configuration store, which can be accessed and managed through the Feature manager menu in Azure App Configuration.
Sources
- https://learn.microsoft.com/en-us/azure/azure-app-configuration/manage-feature-flags
- https://drkreddy.medium.com/feature-toggles-in-azure-building-better-software-faster-f157276161ce
- https://www.nected.ai/blog/azure-feature-flags-vs-nected
- https://docs.launchdarkly.com/integrations/azure-devops
- https://pypi.org/project/azure-appconfiguration-provider/
Featured Images: pexels.com