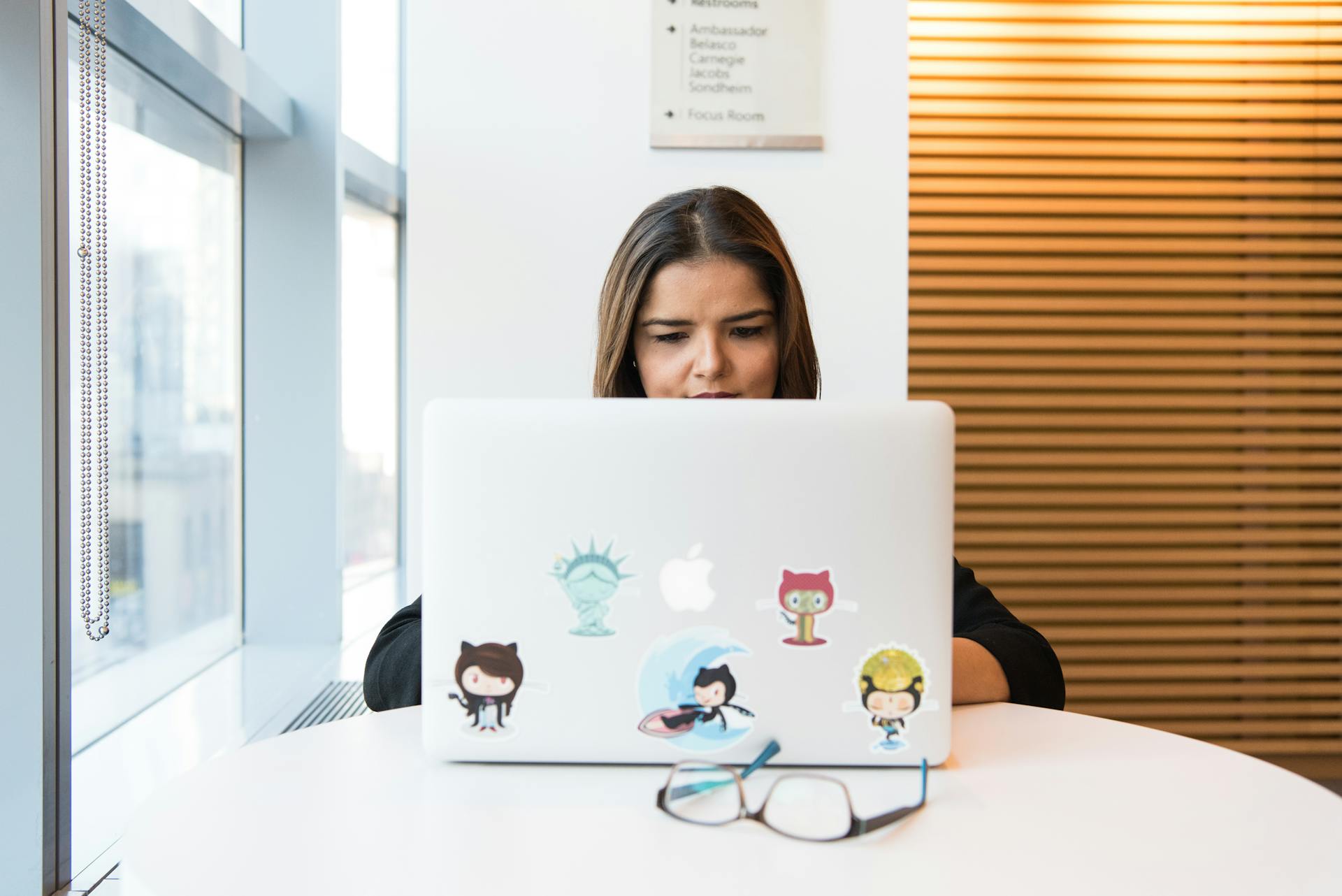
Next.js provides a built-in context API for sharing data between pages, making it easy to manage state and props.
The context API is a global object that can be accessed from any page or component, allowing you to share data between them.
Next.js also provides a built-in way to fetch data on the server-side, using the `getServerSideProps` method.
This method allows you to fetch data and pass it to the page as props, making it easy to manage data fetching and rendering.
By using the context API and `getServerSideProps` method together, you can create a robust and efficient data fetching system for your Next.js application.
Static Site Generation
Static Site Generation is a powerful feature in Next.js that allows you to pre-render pages at build time, making it an ideal choice for SEO and performance.
To use static site generation, you need to export an async function called getStaticProps along with your page component. This function will fetch the data at build time and return an object with props that will be passed into the component.
The getStaticProps function receives the context parameter, which contains some keys, including the params object. This object contains the route parameters for pages using dynamic routes.
You can also use getStaticPaths to define and return all the possible paths for dynamic routes. This function returns an object with paths on it, which is an array containing the params for each dynamic URL that needs to be statically generated.
Here's an example of how you can use getStaticPaths to fetch the data and generate paths for dynamic URLs:
In the getStaticPaths function, you can set the fallback key to true to generate the paths of some pages at build time and defer the rest to be generated at request time. If you set the fallback to false, a 404 page will be generated instead for pages whose paths weren't pre-generated at build time.
To generate static pages for dynamic routes, you need to use both getStaticPaths and getStaticProps. The getStaticProps function will fetch the data using the params object, which is passed from the getStaticPaths function.
For example, if you have a page that fetches a list of items and you want to generate a static page for each item based on its id, you can use getStaticPaths to generate the paths for each item and getStaticProps to fetch the data for each item.
By using static site generation, you can pre-render pages at build time and improve the performance of your Next.js application.
Server Side Rendering
Server Side Rendering is a powerful feature in Next.js that allows you to fetch data on the server and pass it to the client as props. This is achieved through the use of getServerSideProps, which is a function that runs on the server to fetch data and then passes it to the page as props.
getServerSideProps is great for fetching dynamic data that may not be best suited for getStaticProps, such as fetching from a database or an API route with data that changes often. It can also be used for secured data, as the code within the function only runs on the server.
To use getServerSideProps, you can create a folder in the pages folder of your Next.js app and add a file called index.js. In this file, you can write the code to fetch data from an API using getServerSideProps.
Here are the three primary ways to fetch data on the server with Next.js Pages Router:
- Server-side data fetching with getServerSideProps
- Static site generation (SSG) with getStaticProps
- Hybrid static site generation with incremental static regeneration (ISR) enabled in getStaticProps
getServerSideProps allows you to fetch data on each request from the client, making it great for dynamic data. You can use the context parameter to get useful information about the request, including the request path and cookies sent from the client.
To verify what code is sent to the client when using getServerSideProps, you can use the tool https://next-code-elimination.vercel.app/.
Incremental Static Regeneration
Incremental Static Regeneration is a game-changer for dynamic data. It allows you to update static data without blocking the current request, which is a huge improvement over traditional server-side generation.
With ISR, you can set an expiry time for static data, which Next.js will automatically refetch when it's reached. This means that if 60 seconds or more has passed after the last time getStaticProps was run, the code inside getStaticProps will be rerun, and the newly fetched data will be rendered for the next page visitor.
This approach is perfect for data sources that may change between builds, as it ensures that the most up-to-date information is always displayed. Next.js will refetch the data for the next request, but it won't block the current one, which is a win-win situation.
Client Side Rendering
Client Side Rendering in Next.js is a powerful tool that allows you to fetch data directly on the client-side, without the need for server-side rendering.
However, Next.js Pages Router offers three primary ways to fetch data on the server, which can also be relevant for client-side rendering. These methods include server-side data fetching with getServerSideProps, static site generation (SSG) with getStaticProps, and hybrid static site generation with incremental static regeneration (ISR) enabled in getStaticProps.
The three primary ways to fetch data on the server are:
- Server-side data fetching with getServerSideProps
- Static site generation (SSG) with getStaticProps
- Hybrid static site generation with incremental static regeneration (ISR) enabled in getStaticProps
While server-side rendering can be beneficial for performance, it's not always the best choice for every project. Client-side rendering can be a good option when you need to fetch data that changes frequently or is specific to the user's session.
Methods of
Next.js provides several methods for fetching data, making it easier to manage data between the client and server. The most common methods are useEffect and useState hook for client-side data fetching.
You can also use getServerSideProps for server-side data fetching, which is useful when you need to fetch data on every request. This method is particularly useful when you need to fetch data from an external API. For example, the Fake Store API can be used as a backend or database.
There are also getStaticProps and getStaticPath for static-site generation, which allows you to pre-render pages at build time. This can improve performance and reduce the number of requests to your server.
Here are the different methods of fetching data in Next.js:
- useEffect and useState hook for client-side data fetching.
- getServerSideProps for server-side data fetching
- getStaticProps for static-site generation
- getStaticPath for the static-site generation, which uses dynamic routes.
Features
In Next.js, there are three main ways to fetch data: client-side, server-side, and static-site generation. Client-side data fetching is done on the client, like the browser, after the UI has been sent to the user.
Client-side data fetching uses the useState and useEffect hooks from React to render data on the browser. This method reduces Search Engine optimization because the UI is sent to the user without the layout markup.
Server-side data fetching is done on the local machine before rendering it to the user. This method uses the getServerSideProps to fetch data from a database or an API as a backend.
Server-side data fetching works by fetching data every time it's added or updated, which can be slow on every browser refresh. The UI is created on the local machine due to frontend libraries and frameworks like React or Next.js.
Static-site generation is the fastest way to fetch data and send it to the user. This method pre-fetches data from the backend and sends it to the UI before sending it to the user.
Here are the main data fetching methods in Next.js:
- Client-side data fetching: Uses useState and useEffect hooks from React.
- Server-side data fetching: Uses getServerSideProps to fetch data from a database or API.
- Static-site generation: Uses getStaticProps and getStaticPaths to render static pages.
Methods of Data Fetching
You can use the useEffect and useState hook for client-side data fetching. This is done by fetching data from an external API, such as the Fake Store API used in the article.
There are three main methods of data fetching in Next.js: client-side data fetching, server-side data fetching, and static-site generation.
Client-side data fetching uses the useEffect and useState hook. Server-side data fetching uses the getServerSideProps function, which fetches data from the server. Static-site generation uses the getStaticProps function, which generates static pages.
Here are the four methods of data fetching in Next.js:
- useEffect and useState hook for client-side data fetching.
- getServerSideProps for server-side data fetching.
- getStaticProps for static-site generation.
- getStaticPath for the static-site generation, which uses dynamic routes.
To use server-side data fetching, you need to create a folder in the pages folder and a file called index.js. In this file, you use the getServerSideProps function to fetch data from an external API.
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://www.telerik.com/blogs/pre-rendering-data-fetching-strategies-next-js
- https://www.ssw.com.au/rules/fetch-data-nextjs/
- https://blog.openreplay.com/data-fetching-in-next-js/
Featured Images: pexels.com