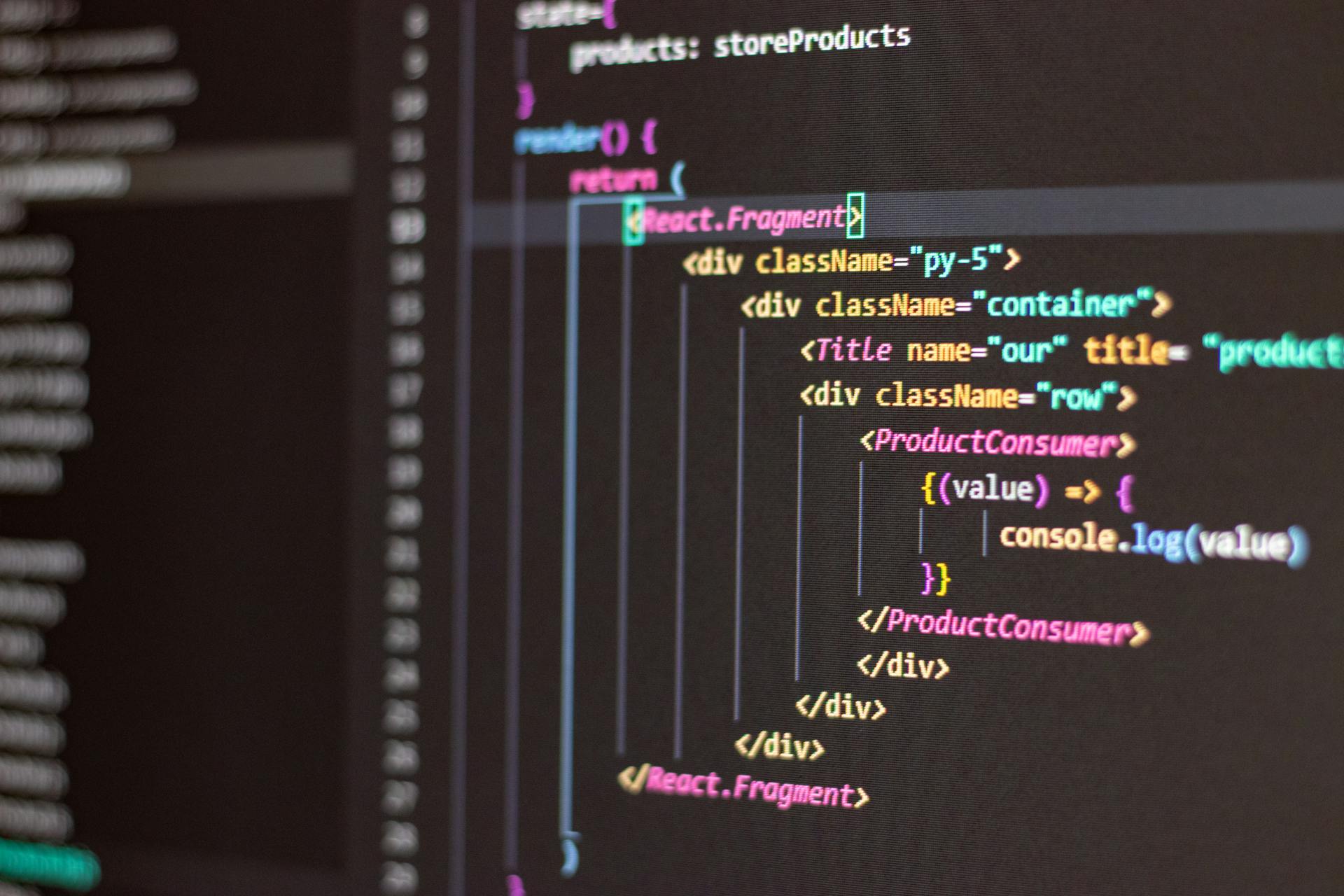
Speeding up a Next.js application's data fetching is crucial for a seamless user experience. By implementing a few proven techniques, you can significantly improve your app's performance.
Using a caching mechanism like Redis or Memcached can reduce the number of requests made to your database, resulting in faster page loads. This is particularly effective for frequently accessed data.
Implementing a data fetching strategy like "prefetching" or "preloading" can also help reduce the number of requests made to your database. By fetching data before it's needed, you can improve the user experience and reduce the load on your database.
Optimizing your API routes can also help speed up data fetching. By using techniques like API route caching and rate limiting, you can reduce the number of requests made to your API and improve performance.
Here's an interesting read: Next Js Performance Analyzer
Optimize Next.js Performance
Fetching data on the server can significantly improve performance, especially when dealing with complex data sets or large datasets. This approach reduces the load on the client-side, resulting in faster page loads and a better user experience.
Be mindful of potential performance issues introduced by some libraries, such as layout shifts, slow rendering, or increased memory usage. Regularly auditing the performance of your application helps identify bottlenecks and potential issues caused by third-party libraries.
Implementing performance monitoring is essential for maintaining and improving the performance of your NextJS application. Regular tracking of key performance indicators helps identify areas for optimization.
Next.js's built-in Image Component and Automatic Image Optimization can dramatically improve performance by optimizing your images. The `next/image` package allows you to include images in your Next.js application seamlessly, optimizing them in various ways.
Here are some techniques to improve performance:
- Use server-side rendering (SSR) and static site generation (SSG) to reduce loading speed and improve user experience.
- Audit the performance of your application regularly to identify bottlenecks and potential issues caused by third-party libraries.
- Optimize images using Next.js's built-in Image Component and Automatic Image Optimization.
These techniques can help you optimize your Next.js application's performance and provide a better user experience.
Efficient Data Fetching
Efficient data fetching is crucial for speeding up a Next.js application. By fetching data only when it's needed, you can reduce the amount of data being transferred and improve performance.
Fetching data only when it's needed can result in faster page loads due to reduced data transfer. This approach can also improve the overall performance of your application by avoiding unnecessary requests.
There are two primary patterns to consider when fetching data in Next.js: parallel and sequential data fetching. Understanding the differences between these patterns is crucial for optimizing your application's performance.
Parallel data fetching can improve performance by allowing multiple requests to be made simultaneously, while sequential data fetching can be more straightforward to implement but may lead to slower performance if not done correctly.
To fetch data efficiently, consider using client-side fetching with libraries like SWR and React Query. These libraries can help you fetch data in a way that's optimized for performance.
Here are some key benefits of efficient data fetching:
Preload for Improved Performance
Preloading data in your Next.js application can significantly improve performance by reducing the time it takes for pages to load. This technique involves fetching data in advance, providing a better user experience for your users.
Next.js offers three ways to implement preloading: getStaticProps, getServerSideProps, and useEffect. The choice of method depends on your specific use case.
To implement preloading effectively, consider the following best practices:
Fetching data on the server can significantly improve performance, especially when dealing with complex data sets or large datasets. By offloading data processing to the server, you can reduce the load on the client-side.
Code Splitting and Lazy Loading
Code Splitting and Lazy Loading are two powerful techniques to optimize the performance of your NextJS application. NextJS automatically handles code splitting at the page level, ensuring users download only the required JavaScript for each page.
This means that users only need to download the necessary code for the page they're visiting, resulting in faster load times. By minimizing the amount of JavaScript and resources loaded initially, you can provide a smoother and more responsive user experience.
Code splitting is the practice of breaking your application's JavaScript bundle into smaller chunks. This way, users only need to download the necessary code for the page they're visiting.
For more insights, see: Nextjs Spa
Here are the benefits of code splitting:
- Reduced initial load time
- Improved user experience
- Smaller JavaScript bundle size
Lazy loading is the process of deferring the loading of certain components or resources until they are needed. This reduces the initial load time of your application, as users only download the required assets when they become visible or interacted with.
You can implement lazy loading using dynamic imports in NextJS, which enables you to load components on demand. React.lazy and Suspense can also be used in combination with NextJS to further optimize component loading.
By implementing code splitting and lazy loading, you can lead to significant performance improvements in your NextJS application.
Related reading: Next Js Components
Image and Content Optimization
Optimizing images is a crucial aspect of improving the performance of your NextJS application. Proper image optimization can lead to faster load times, better responsiveness, and reduced bandwidth usage.
Next.js’s built-in Image Component and Automatic Image Optimization can dramatically improve performance by optimizing your images. The `next/image` package allows you to include images in your Next.js application seamlessly.
Next.js will automatically serve scaled images based on the device’s viewport and screen density, reducing the load time for users on smaller or lower-density screens.
For more insights, see: Next Js Performance Analyzer Library Npm
Reducing Third-Party Library Usage
Reducing Third-Party Library Usage is crucial for optimizing your NextJS application's performance. This is because some libraries can add unnecessary bloat to your bundle size, leading to longer load times.
Professional Next.js developers can critically evaluate if you really need a library or if built-in Next.js features or JavaScript/React APIs can achieve the same result.
Some libraries can provide valuable functionality, but they often come with a performance cost that can negatively impact your application’s load time and overall efficiency.
If the use of a third-party library is unavoidable, seasoned Next.js developers make sure to check if there’s a lighter version available or if they can import only the parts you need.
Readers also liked: Nextjs Performance
Monitoring and Best Practices
Regular performance monitoring is essential for maintaining and improving the performance of your NextJS application. By keeping track of key performance indicators, you can identify bottlenecks, inefficiencies, and areas for optimization.
Some libraries can introduce performance issues, such as layout shifts, slow rendering, or increased memory usage. Be mindful of these potential issues when choosing libraries to include in your application.
To optimize API performance and data fetching, consider techniques like offloading data processing to the server. This can reduce the load on the client-side, resulting in faster page loads and a better user experience.
Regularly auditing the performance of your application is crucial to identify bottlenecks and potential issues caused by third-party libraries. This can help you make informed decisions about which libraries to use and how to optimize their performance.
Here are some key performance indicators to track:
- Page load times
- Data processing times
- Memory usage
By monitoring these key performance indicators and being mindful of potential performance issues, you can ensure a smooth and responsive experience in your NextJS application.
Content Delivery Network
Using a Content Delivery Network (CDN) can greatly improve the performance of your Next.js application. By serving static material from a nearby CDN server, users experience faster load times and less latency.
A CDN can help you manage high traffic spikes without affecting your primary server, making it a great solution for scalability. With a CDN, your server will be less taxed when serving static material, which may result in lower bandwidth bills.
Here are the benefits of CDN integration:
- Faster Load Times: By serving material from the closest CDN server, users experience is delivered with less latency.
- Greater Scalability: High traffic spikes can be managed by CDNs without affecting your primary server.
- Reduced Bandwidth Costs: Your server will be less taxed when static material is offloaded to the CDN, which may result in lower bandwidth bills.
- Increased Availability: Because CDNs are dispersed geographically, your material is still available even in the event that one server goes down.
To integrate a CDN in your Next.js application, you'll need to select and configure a CDN provider, such as Cloudfare or Fastly. You'll be given a CDN URL to serve your assets from, which you'll need to specify in your next.config.js file.
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://nextjsstarter.com/blog/nextjs-data-fetching-10-api-best-practices/
- https://www.cronj.com/blog/top-tips-for-optimizing-the-performance-of-your-nextjs-application/
- https://clouddevs.com/next/performance-optimization/
- https://semaphoreci.com/blog/cache-optimization-nextjs
Featured Images: pexels.com