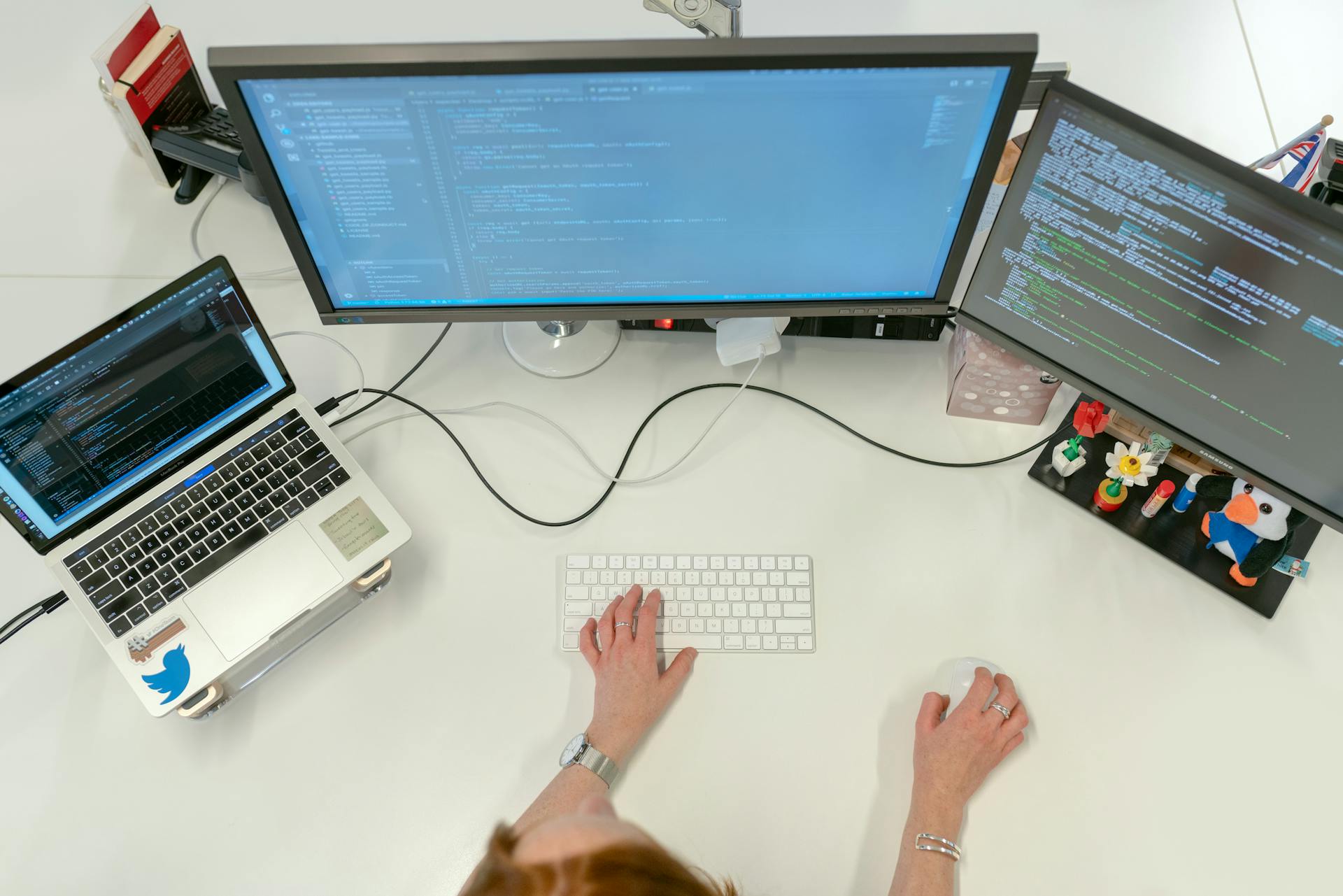
The Next.js performance analyzer library npm is a game-changer for developers looking to optimize their app's performance. By leveraging this library, developers can identify and fix performance bottlenecks, leading to a smoother user experience.
This library provides a set of tools that help analyze and optimize Next.js app performance, including automatic code splitting and lazy loading.
Curious to learn more? Check out: Next Js Performance Analyzer
Setting Up Next JS
Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites. It's a great choice for developers who want to create high-performance websites.
To set up Next.js, you need to create a new project using the `create-next-app` command. This command will scaffold a basic Next.js project with all the necessary dependencies.
Next.js projects are built using a custom configuration file called `next.config.js`. This file allows you to configure various aspects of your project, such as the development server and production build settings.
Next.js also comes with a built-in development server that provides hot reloading and live reloading features. This means you can see the changes you make to your code in real-time without having to manually restart the server.
To get started with Next.js, you need to install the `next` package using npm or yarn. This package provides the core functionality of Next.js and is required for building and running Next.js projects.
Next.js projects use a custom file structure that is optimized for static site generation. This means that your project directory will have a specific layout that includes folders for pages, components, and static assets.
Performance Optimization
To optimize your Next.js app's performance, you need to consider both client-side and server-side optimizations. Large images and fonts can significantly inflate your bundle size, but Next.js has built-in support for optimizing images and fonts to reduce bundle size.
Here are some key areas to focus on:
- Compress or resize images with large file sizes
- Remove duplicate npm packages
- Remove unused npm packages
- Use minification, tree shaking, and code splitting to optimize JavaScript files
Some popular npm packages can help optimize your Next.js app, including next-optimized-images, next-purgecss, and compression. These tools can significantly reduce load times and improve user experience.
Font Optimization
Font optimization is crucial for a smooth user experience. Large font files can significantly slow down your website.
Using custom fonts can increase your bundle size, so it's essential to minimize their impact. Next.js automatically optimizes Google Fonts, which can be a huge relief.
Loading fonts asynchronously or preloading them can improve performance. This will ensure your fonts are served in an optimized format, reducing their impact on performance.
Google Fonts are optimized by Next.js, which is a great feature. However, if you're using other fonts, you'll need to take extra steps to optimize them.
Check this out: Google Search Console Nextjs
Server-Side Optimizations
Server-side optimizations are crucial for performance optimization. A slow server can degrade performance regardless of bundle size, as mentioned earlier.
Optimizing server-side response time is essential to ensure fast delivery of JavaScript files. If your server is slow, it will negatively impact user experience.
To minimize server response time, consider optimizing your server configuration. This can include adjusting settings, upgrading hardware, or using cloud services.
A slow server can lead to increased latency, which can cause users to abandon your website. This can result in lost revenue and a negative impact on your online presence.
Consider reading: Next Js Build Time Slow
Optimizing server-side response time can be achieved through various means, including caching, content delivery networks (CDNs), and load balancing. These techniques can help reduce the load on your server and improve response times.
By implementing server-side optimizations, you can significantly improve the performance of your website. This can lead to increased user engagement, improved conversion rates, and a better overall user experience.
HTTP/2 and Compression
HTTP/2 and Compression can significantly reduce load times, especially for larger JavaScript bundles. This is because HTTP/2 minimizes the size of files sent from the server to the client.
By enabling Brotli compression, you can ensure that even large assets are delivered as efficiently as possible. This improves load times for your users.
Several npm packages can help you leverage HTTP/2 and compression in your Next.js app. Some popular choices include next-optimized-images and compression.
Analyzing Performance
Analyzing performance is a crucial step in optimizing your Next.js application. To run an analysis for your app bundles, you'll need to start your Next.js app in development mode with the ANALYZE environment variable set to true.
The @next/bundle-analyzer package provides a graphical representation of your JavaScript bundles. Each module is represented as a rectangle, where the size of the rectangle corresponds to the module's size in your bundle. Larger rectangles represent heavier modules, which take up more space and time to load.
You can use the command `npm run analyze` to view the analysis. This command will automatically create an `analyze` folder in your `.next` folder and open three files: `client.html`, `nodejs.html`, and `edge.html`. The `client.html` file represents the client side, and the `nodejs.html` file represents the server side.
The Next.js Bundle Analyzer provides detailed metrics, including load times and rendering speeds. To save time, you can create a quick command/script to run in your `package.json` file by adding the following script: `cross-env ANALYZE=true npm run analyze`.
The bundle analyzer helps identify potential bottlenecks and areas for improving an app's load speed and reducing its bundle size. To optimize app performance, consider the following tips:
- Spotting large dependencies: Inspect large modules to see which are third-party packages, then look for alternative packages that are smaller in size.
- Detecting duplicate code: If a library or module appears multiple times in the bundle, different versions of that library might be installed in your application. Cross-check and remove the version you are not using.
- Analyzing bundle size metrics: Consider optimizing a module if the stat size of that module is large. A large parsed size can also affect the memory usage and performance of the app. A large gzipped size can affect the loading times of your pages.
- Identifying unused code: Tree shaking can remove unused code, but sometimes, there may be cases where you have unshakable codes. Inspect your analysis report to confirm that there is no unused code in your bundle and manually exclude any found by modifying the webpack config in your `next.config.js` for example.
Here are some possible ways to address common issues:
By following these tips and using the bundle analyzer, you can optimize your Next.js application's performance and provide a better user experience.
Code Splitting and Size Reduction
Code splitting is a technique used to reduce the size of your Next.js bundle. It involves breaking up your JavaScript bundle into smaller pieces, which can be loaded on demand. This reduces the initial load time and improves performance. Next.js supports automatic code splitting, loading only the necessary JavaScript for the page being visited.
Dynamic imports can be used to further enhance code splitting. For example, you can load a component only when it's needed, like this: `import DynamicComponent from './DynamicComponent';`. This approach loads the component only when it's rendered, optimizing the loading of your application.
Measuring bundle size is crucial for understanding how much JavaScript your application is sending to users. Tools like webpack-bundle-analyzer can visualize the size of your bundles. To install and set up webpack-bundle-analyzer, run `npm install --save-dev webpack-bundle-analyzer` and update your `next.config.js` file.
Large images and fonts can significantly inflate your bundle size. Next.js has built-in support for optimizing images and fonts, which can be further leveraged to reduce bundle size.
For another approach, see: How to Install Next Js
Here are some common issues that can be identified using bundle analysis:
- Large dependencies: Inspect large modules to see which are third-party packages, then look for alternative packages that are smaller in size.
- Duplicate code: If a library or module appears multiple times in the bundle, different versions of that library might be installed in your application. Cross-check and remove the version you are not using.
- Analyzing bundle size metrics: Consider optimizing a module if the stat size of that module is large. A large parsed size can also affect the memory usage and performance of the app.
- Identifying unused code: Tree shaking can remove unused code, but sometimes, there may be cases where you have unshakable codes. Inspect your analysis report to confirm that there is no unused code in your bundle and manually exclude any found by modifying the webpack config in your `next.config.js` for example.
- Utilizing source maps: Source maps like source-map-explorer can help you map your minified code back to its original source. This is useful for debugging errors where you have to identify the files contributing to the minified code.
Some popular npm packages for optimization include:
- next-optimized-images: Automatically optimizes images for various formats and sizes.
- next-purgecss: Removes unused CSS from your application, reducing the size of stylesheets.
- compression: Enables Gzip or Brotli compression, reducing the size of files sent over the network.
Webpack and npm Packages
Webpack and npm Packages are essential tools for optimizing Next.js application performance. You can use npm packages like next-optimized-images to automatically optimize images for various formats and sizes, reducing the app's bundle size and improving load times.
Customizing Webpack configuration allows for further optimization of your Next.js application. You can modify the configuration to enable compression, minification, and other performance enhancements.
To customize the Webpack configuration, add the webpack property to your next.config.js file and pass your configurations on to it. This can be done by splitting the vendor libraries into a separate chunk for the client-side bundle.
Some popular npm packages for optimization include:
- next-optimized-images: Automatically optimizes images for various formats and sizes.
- next-purgecss: Removes unused CSS from your application, reducing the size of stylesheets.
- compression: Enables Gzip or Brotli compression, reducing the size of files sent over the network.
By installing and utilizing these packages, you can significantly reduce load times and improve user experience. Enabling minification reduces the size of your JavaScript files, leading to faster load times.
Sources
- https://www.syncfusion.com/blogs/post/optimize-next-js-app-bundle
- https://www.catchmetrics.io/blog/reducing-nextjs-bundle-size-with-nextjs-bundle-analyzer
- https://blog.logrocket.com/how-to-analyze-next-js-app-bundles/
- https://www.squash.io/how-to-use-a-nextjs-performance-analyzer-library/
- https://prateekshawebdesign.com/blog/top-performance-analyzer-libraries-for-nextjs-on-npm
Featured Images: pexels.com