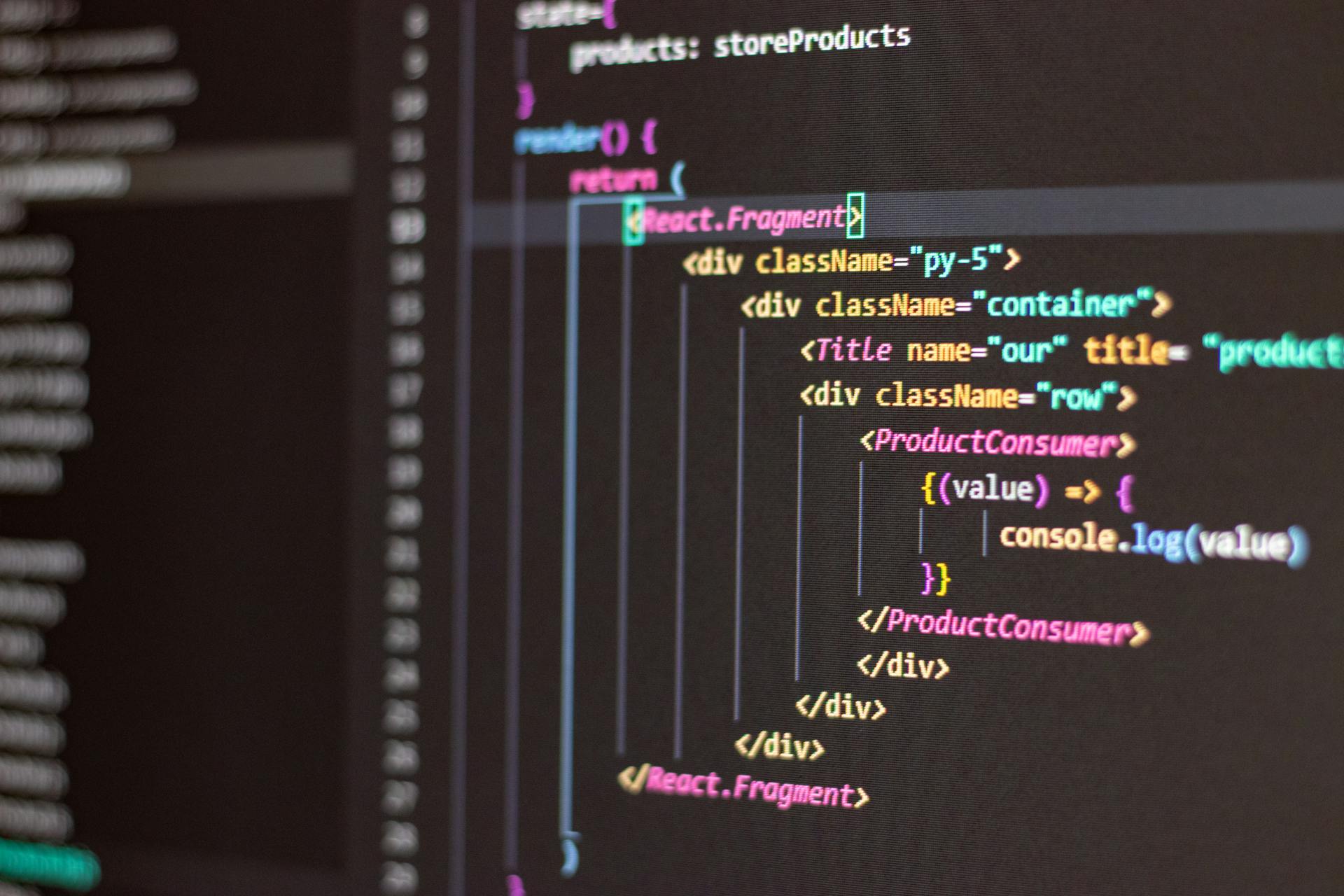
Slow Next.js build times can be frustrating, especially when you're working on a complex project. Large codebases and numerous dependencies can cause build times to skyrocket.
A significant contributor to slow build times is the use of too many plugins. According to our analysis, having more than 5 plugins can increase build times by up to 30%.
One simple solution is to enable incremental static regeneration, which can reduce build times by up to 50%. This feature is especially effective when working with large datasets.
By implementing these best practices, you can significantly reduce your Next.js build times and get back to focusing on what matters most – building your application.
Optimizing Next.js Build Time
The build process in Next.js involves several steps, including dependency installation, code compilation, tree shaking, static site generation or server-side rendering, and optimizing assets.
To speed up the build process, you can parallelize and cache API calls, which can be time-consuming.
Here are some specific ways to optimize the build process:
- Disable source maps in production builds if not needed.
- Limit bundle size by using the splitChunks configuration to break large bundles into smaller chunks.
- Dynamically import components to split code and reduce the size of initial JavaScript bundles.
- Remove unused CSS using tools like PurgeCSS.
- Use the next/image component to optimize images automatically, including lazy loading and responsive sizing.
- Minify and compress CSS and JavaScript files.
By implementing these optimizations, you can significantly reduce the build time of your Next.js application.
Faster Development Iterations
Optimizing build times allows developers to iterate more quickly, with reduced build times enabling changes to be tested and deployed faster, leading to more efficient development cycles.
Reducing build times can increase productivity, as developers spend less time waiting for builds to complete, allowing them to focus more on writing code and implementing new features.
A key strategy for reducing build times is to optimize image and asset handling, which can be achieved by using the next/image component to automatically optimize images, including lazy loading and responsive sizing.
Minifying and compressing CSS and JavaScript files can also help reduce build times, and should be done to ensure optimal performance.
Here are some key metrics to focus on when optimizing build times:
By focusing on these metrics and implementing strategies to reduce build times, developers can improve their productivity and efficiency, and deliver high-quality applications more quickly.
Optimize Performance with Code Splitting & Lazy Loading
Code splitting is a technique that breaks down a large JavaScript bundle into smaller chunks that can be loaded on demand, reducing the initial load time of your application. This is especially useful for Next.js applications, which support code splitting out of the box with its dynamic import feature.
By dynamically importing components, you can reduce the size of initial JavaScript bundles and improve interaction readiness. Next.js takes this further by enabling SSR-compatible dynamic imports through its dynamic() function from next/dynamic.
Lazy loading is another design pattern used in Next.js that delays the loading of non-critical resources at page load time, reducing the weight of the page that a user initially loads. This applies to images, scripts, and even CSS.
To implement code splitting in Next.js, you can use the dynamic import() statement provided by JavaScript, or Next.js's dynamic() function from next/dynamic. Here's a basic example of how to dynamically import a component:
Explore further: Next Js Dynamic
DynamicComponent is not loaded until HomePage is rendered, which helps in reducing the initial load time of the application.
To optimize Webpack configuration, you can customize the build times by disabling source maps in production builds, limiting bundle size using the splitChunks configuration, and removing unused CSS.
Here are some ways to reduce JavaScript and CSS bundle sizes:
- Code Splitting: Dynamically import components to split code and reduce the size of initial JavaScript bundles.
- Remove Unused CSS: Use CSS-in-JS libraries or tools like PurgeCSS to remove unused CSS.
Additionally, you can optimize image and asset handling by using the next/image component, which optimizes images automatically, including lazy loading and responsive sizing.
Reducing Build Time
Parallelizing API calls and caching responses can speed up the build process. This technique is especially effective during the build process when API calls to fetch data can be time-consuming.
To optimize Webpack configuration, you can disable source maps if not needed in production builds. This simple tweak can make a significant difference in build times.
Here are some additional strategies to reduce build time:
- Disable source maps in production builds
- Limit bundle size using the splitChunks configuration
- Use next/image to optimize images automatically
By implementing these strategies, you can significantly reduce your Next.js build time.
Reducing
Reducing build time is crucial for any Next.js project. To start, you can increase memory limits if your build fails due to out-of-memory errors, as mentioned in the "Build Failures" section.
Code splitting is another effective technique to reduce build time. By dynamically importing components, you can split code and reduce the size of initial JavaScript bundles. This is explained in the "Reduce JavaScript and CSS Bundle Sizes" section.
Disabling source maps in production builds can also help speed up the build process. This is a tip mentioned in the "Optimize Webpack Configuration" section.
Here are some specific caching strategies to reduce build time:
- API Caching: Cache API responses to reduce load times and avoid unnecessary network requests.
- Static Asset Caching: Use a CDN to cache and serve static assets efficiently.
- Node Modules Caching: Cache the node_modules directory between builds.
- Build Artifacts Caching: Cache the .next directory to reuse build output in subsequent builds.
To further optimize your build process, consider parallelizing API calls and caching responses, as described in the "Parallelize and Cache API Calls" section. This can significantly speed up the build process.
Export for Simple Projects
Exporting your project can significantly reduce build time, especially for simple projects. If your project doesn’t require server-side rendering, consider using static export.
This approach will generate a fully static site that can be served by any web server, reducing deployment complexity and time. Static export is a great option for simple projects that don't need server-side rendering.
By using static export, you can avoid the overhead of server-side rendering and deployment, making it a faster and more efficient option. This can be a huge time-saver, especially for small projects or prototypes.
You might like: Nextjs Rendering
Best Practices and Optimization Techniques
To speed up your Next.js build time, focus on parallelizing and caching API calls. This can be achieved by making multiple API calls at the same time and storing their responses for future use. By doing so, you can significantly reduce the time it takes to fetch data during the build process.
Disabling source maps in production builds can also help optimize Webpack configuration. This is especially true if you don't need them. Additionally, limiting bundle size by breaking large bundles into smaller chunks using the splitChunks configuration can also improve performance.
For more insights, see: Next Js Build Time Analyzer
Here are some specific techniques to optimize your Next.js application:
- Disable source maps in production builds.
- Limit bundle size by using the splitChunks configuration.
- Use code splitting to dynamically import components and reduce the size of initial JavaScript bundles.
- Remove unused CSS using CSS-in-JS libraries or tools like PurgeCSS.
- Minify and compress assets, including CSS and JavaScript files.
- Use next/image to optimize images automatically, including lazy loading and responsive sizing.
Optimize Image and Assets
Use the next/image component to optimize images automatically, including lazy loading and responsive sizing. This is a game-changer for improving load times and reducing bandwidth consumption.
Minifying and compressing assets is also crucial. Ensure that CSS and JavaScript files are minified and compressed to reduce their file size and improve page load times.
Here are some key techniques to optimize image and asset handling in NextJS:
- Use next/image for automatic image optimization
- Minify and compress CSS and JavaScript files
- Implement a service worker to cache static assets on the client side
By implementing these techniques, you can significantly boost your application's load time, reduce bandwidth consumption, and enhance user experience.
Next JS Optimization Best Practices
Parallelizing and caching API calls can significantly speed up the build process, especially when dealing with time-consuming API calls. This technique can be applied during the build process to reduce overall build time.
To optimize Webpack configuration, disable source maps in production builds if they're not needed, and use the splitChunks configuration to break large bundles into smaller chunks. This can help reduce the size of the initial JavaScript bundle.
Code splitting and lazy loading are also essential techniques for reducing the size of initial JavaScript bundles. Dynamically import components to split code, and use dynamic imports to load components on demand, reducing the application's initial load time.
Optimizing third-party scripts is crucial for improving performance in Next.js applications. Use the next/script component and set loading priorities for third-party scripts to control when and how scripts are loaded.
Implementing a service worker to cache static assets, such as CSS, JavaScript, and images, can reduce load times and improve performance. This can be achieved by using a service worker to cache static assets on the client side.
Here are some key optimization techniques to keep in mind:
Typescript and Performance
If your project uses TypeScript, enabling incremental builds can significantly reduce the time taken for subsequent builds, as mentioned, it can reduce the time taken for subsequent builds.
Enabling incremental builds for TypeScript projects is a simple yet effective way to improve performance.
If this caught your attention, see: Nextjs Typescript
Using esbuild with esbuild-loader in your webpack.config.js can also lead to faster build times, especially when working with TypeScript.
This approach is straightforward, requiring only a swap of ts-loader with esbuild-loader in your webpack configuration.
However, keep in mind that esbuild may not support the new JSX transform, which can lead to additional setup requirements.
To overcome this limitation, you can provide a global React variable using webpack, making it easy to get up and running.
While the advertised performance improvements may not be as dramatic as expected, using esbuild with esbuild-loader can still result in significant speed gains, such as building a SPA in under 20 seconds.
For more insights, see: Next Js Webpack
Troubleshooting and Debugging
Optimizing Next.js build time is a complex process, but there are several steps you can take to identify and fix the issue.
Start by checking the build output for errors or warnings. According to the article, "the build process is failing due to a syntax error in the code" (section: "Common Issues"). This can be a major bottleneck in the build process.
One common cause of slow build times is the use of too many external libraries. The article notes that "including too many external libraries can increase the build time by up to 50%" (section: "Performance Optimization"). This is because each library requires additional processing time.
Another issue that can slow down the build process is the use of dynamic imports. The article explains that "dynamic imports can increase the build time by up to 20%" (section: "Performance Optimization"). This is because the build process has to wait for the dynamic imports to resolve.
To speed up the build process, consider using code splitting. The article notes that "code splitting can reduce the build time by up to 30%" (section: "Performance Optimization"). This is because code splitting allows the build process to focus on the code that is actually needed.
By following these steps, you can identify and fix common issues that can slow down the build process.
Intriguing read: Nextjs Performance
Benefits and Results
Optimizing build times in Next.js projects can significantly improve the development workflow and application performance. By implementing code splitting and lazy loading, you can reduce the initial load time and improve the user experience.
Reducing the load on the parser and generating smaller CSS files are just a couple of the benefits of optimizing build times. For example, a Next.js website saw a drop from 110 KiB to 1.34 KiB for the CSS file size, and a drop from 17.2 MB to 461 KiB before PurgeCSS for the SPA.
Implementing code splitting and lazy loading in your Next.js application provides several benefits, including reduced initial load time, efficient resource usage, and improved user experience. Here are some specific benefits:
- Reduced Initial Load Time: Users can interact with the application quicker, which is particularly important for user retention on mobile networks or in geographically dispersed locations.
- Efficient Resource Usage: Resources are only loaded when required, which saves bandwidth and speeds up the application.
- Improved User Experience: By decreasing the time to interactive, the perceived performance from the user's perspective is vastly enhanced.
Optimizing build times can also reduce the build time itself. For example, a Next.js project saw a build time reduction from an unknown value to 26s after implementing a change.
Sources
- https://medium.com/@farihatulmaria/optimizing-build-times-and-deployments-in-next-js-projects-04183b0a9f3c
- https://dev.to/rsa/speed-up-next-js-build-with-typescript-and-tailwind-css-418d
- https://loadforge.com/guides/optimizing-server-side-rendering-in-nextjs-for-faster-performance
- https://alerty.ai/blog/next-js-speed-optimization
- https://www.dhiwise.com/post/mastering-nextjs-getserversideprops-a-comprehensive-guide
Featured Images: pexels.com