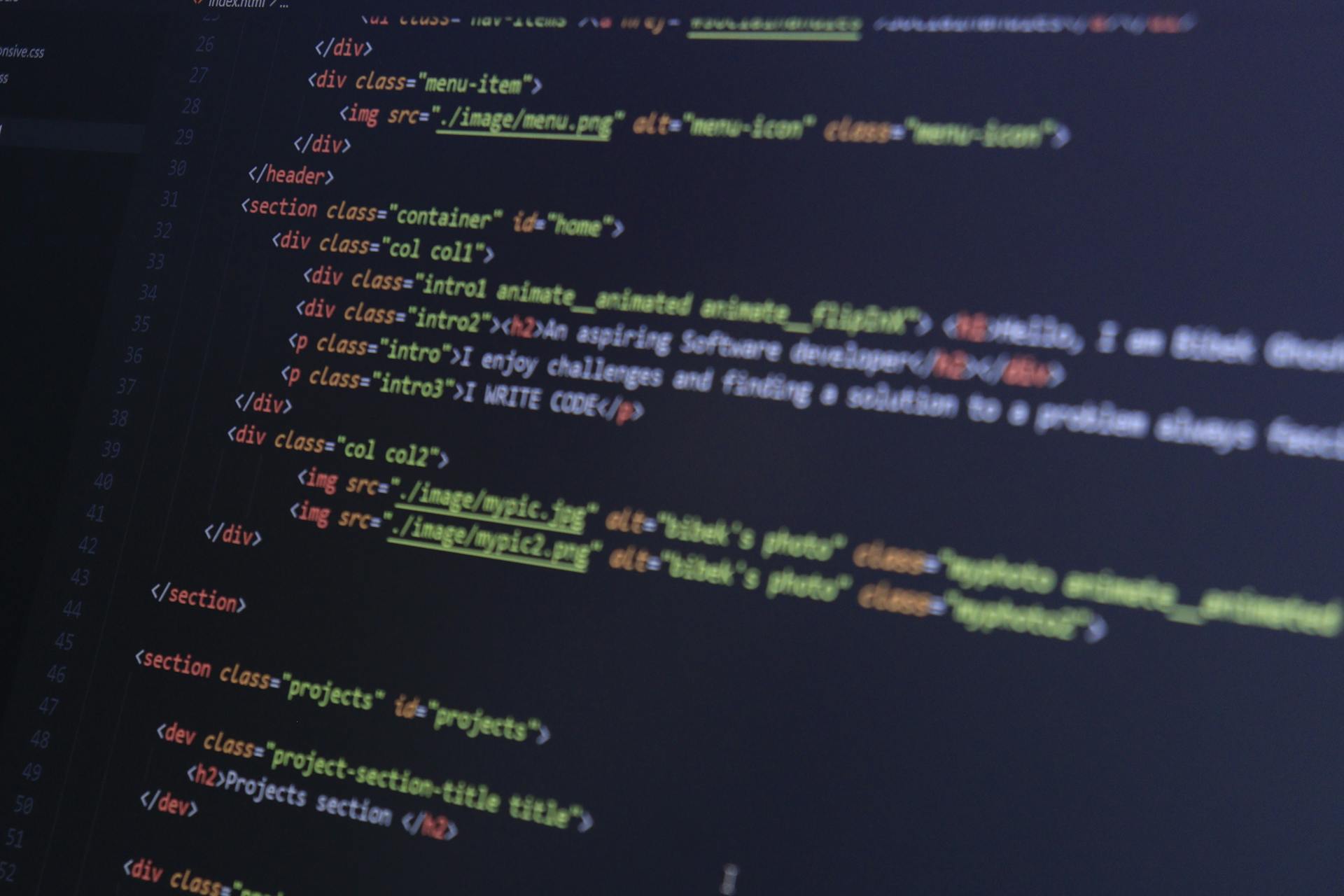
Nextjs offers two primary rendering strategies: Server-Side Rendering (SSR) and Static Site Generation (SSG). SSR renders pages on the server for each request, while SSG pre-renders pages at build time, resulting in faster page loads.
Server-Side Rendering (SSR) is ideal for applications with dynamic content, as it allows for real-time updates and improved SEO. This is because search engines can crawl and index the HTML content generated by the server.
Static Site Generation (SSG) is better suited for applications with static content, as it pre-renders pages at build time, reducing the load on the server and improving page load times. According to Nextjs documentation, SSG can reduce page load times by up to 90% compared to SSR.
Intriguing read: Nextjs Server Rendering Tailwind
Server-Side Rendering
Server-Side Rendering is an approach that fundamentally changes how web pages are delivered. It's an important concept in modern web development that can significantly enhance the performance and user experience of web applications.
Server-side rendering can reduce page load times, improve SEO and the user experience, and give an accessibility and performance boost. This is achieved by rendering web pages on the server rather than on the client-side.
To implement SSR using Next.js, you can follow a step-by-step guide that includes creating a new component named SimpleSSRComponent in the app/page.tsx file. This component can be used to test and understand the basics of SSR.
Server component trees allow you to organize your server components in a way that maximizes the efficiency of data fetching and rendering. By structuring your server components effectively, you can minimize the amount of data that needs to be transferred to the client for each request.
Three different server-rendering strategies are available in Next.js: static rendering, dynamic rendering, and streaming. These strategies can be used depending on the specific needs of your project.
To leverage SSR effectively, it's essential to structure your components appropriately. This includes organizing components into reusable pieces and ensuring that the components you intend to render on the server are React functional components.
Here are some key considerations for styling in a server-rendered environment:
- Consider using CSS-in-JS libraries like styled-components or Emotion to write CSS directly in your JavaScript or TypeScript components.
- For global styles, create app/global.css and include it in the layout.tsx.
- Scoped styles can be achieved by creating styles within the component file.
- Consider serving critical CSS inline within your HTML so initial styles load quickly.
By following these guidelines and strategies, you can effectively implement server-side rendering in your Next.js project and improve the performance and user experience of your web application.
Benefits of Server-Side Rendering
Server-side rendering (SSR) is a game-changer for web applications, and for good reason. It can significantly enhance the performance and user experience by reducing page load times.
SSR improves SEO by allowing search engine crawlers to easily access and index the content, which means your website's pages are more likely to be properly indexed and ranked in the search engine results pages (SERPs).
This can lead to a boost in organic traffic, making SSR a valuable tool for web developers and businesses aiming to increase their online visibility. By pre-rendering content, websites can load faster and provide a better user experience, especially for users on slower networks and older devices.
Server-side rendering is particularly beneficial for content-heavy websites, such as blogs, news sites, or e-commerce platforms, where pre-rendering content ensures faster page loads and better SEO.
Here are some optimal use cases for SSR implementation:
- Content-heavy websites
- Authentication and user-specific data
- Progressive enhancements
By leveraging SSR, you can give your users an immediate and functional experience, even before any JavaScript is executed, which reduces the frustration of waiting for content to load and improves the perception of your website's speed and reliability.
Implementing Server-Side Rendering
Server-side rendering (SSR) is an important concept in modern web development that fundamentally changes how web pages are delivered. It's an approach that can significantly enhance the performance and user experience of web applications by reducing page load times, improving SEO and the user experience, and giving an accessibility and performance boost.
To implement SSR, you can use Next.js, which provides a step-by-step guide to get you started. In your project folder, create a new component named SimpleSSRComponent in the app/page.tsx file, clearing any existing code in this file. This is where the magic happens, and you can start building your SSR-enabled application.
SSR can be used in various scenarios, including content-heavy websites, authentication and user-specific data, and progressive enhancements. Content-heavy websites, such as blogs or news sites, can greatly benefit from SSR, as pre-rendering content ensures faster page loads and better SEO.
Here are some optimal use cases for SSR implementation:
- Content-heavy websites: Websites with a significant amount of content, such as blogs, news sites, or e-commerce platforms, can greatly benefit from SSR.
- Authentication and user-specific data: SSR can be used for pages that require authentication or display user-specific data.
- Progressive enhancements: SSR can be used as part of a progressive enhancement strategy.
To leverage SSR effectively, you'll need to structure your components appropriately. Organize your components into reusable pieces, ensuring that the components you intend to render on the server are React functional components. Be mindful of client-side specific code, such as window or document references, which may not be available during server-side rendering. Conditionally include such code only when rendering on the client.
Optimizing Server-Side Rendering
Server-side rendering (SSR) is an important concept in modern web development that fundamentally changes how web pages are delivered, reducing page load times and improving SEO.
SSR can significantly enhance the performance and user experience of web applications by reducing page load times, improving SEO and the user experience, and giving an accessibility and performance boost.
To implement SSR using Next.js, you need to create a new component named SimpleSSRComponent in the app/page.tsx file.
Here are some strategies to consider for maintaining a fast and responsive SSR application:
- Performance budgets: Establish performance budgets to define acceptable limits for metrics like page load time, time to interact, and first contentful paint.
- Server load testing: Test your server's capacity to handle the load that SSR generates.
- Caching: Implement caching strategies at both the server and client levels.
- Content Delivery Networks (CDNs): Consider using CDNs to distribute your assets and content globally.
- Error handling and monitoring: Implement robust error handling and monitoring mechanisms.
SSR minimizes initial page load times because the server sends a fully pre-rendered HTML page to your browser, unlike in client-side rendering where the browser needs to fetch JavaScript files.
Routing and Navigation
Next.js has built-in routing that automatically maps files in your app directory to URLs, so you can create routes without manual configuration. This makes it easy to handle page transitions smoothly without a full page load, enhancing the perceived performance of your application.
You can use the app router for client-side navigations, which is a powerful tool for managing page transitions. The app router can handle page transitions smoothly without a full page load, greatly enhancing the perceived performance of your application.
To enable seamless navigation between pages, use the Link component from Next.js. This component allows you to create links to other pages in your application, making it easy to navigate between them.
Here's a quick rundown of how to use the Link component:
Dynamic routing simplifies handling various content with similar structures, such as an e-commerce website with hundreds of products. You can use dynamic segments if you have data that changes and want to create routes without knowing the specific segment names beforehand.
App Router Navigation
The app router is a powerful tool for managing client-side navigations, allowing for smooth page transitions without a full page load, which can greatly enhance the perceived performance of your application.
To enable seamless navigation between pages in your Next.js application, use the Link component, which automatically maps files in your app directory to URLs, so you can create routes without manual configuration.
The Link component is imported from Next.js and used to create a link to the desired page. For example, to link to the / page (home page), you can use the Link component like this.
Next.js has built-in routing that automatically maps files in your app directory to URLs, so you can create routes without manual configuration. This includes files like app/profile/page.tsx, which corresponds to the /profile route.
Here are the key benefits of using the Link component in your Next.js application:
- Seamless navigation between pages
- No manual route configuration required
Dynamic Routing
Dynamic routing is a powerful tool in Next.js that allows you to handle various content with similar structures. You can use dynamic segments if you have data that changes and want to create routes without knowing the specific segment names beforehand.
These segments can be populated when a request is made or prepared in advance during the build process, and is also called dynamic routing. You can simplify handling various content with similar structures by using dynamic routing.
Consider an e-commerce website where you sell different products. Creating a unique file for each product is impractical if they all share a common format, including a name, description, and price. Dynamic routing helps manage content efficiently, even if you have hundreds or thousands of different products.
Each product uses the same file structure, but displays unique product details based on the parameter provided in the URL. For example, you can create a dynamic route like app/products/[productId]/page.tsx to handle a range of products.
Here's a breakdown of how dynamic routing works:
With dynamic routing, you can create a single file structure that covers all products, making it easier to manage and maintain your content.
Error Handling and Data Fetching
Proper error handling is crucial for a good user experience, especially when rendering components on the client side, anticipating potential issues such as network errors or missing data.
You should provide appropriate fallbacks or error messages to handle these issues.
To fetch data during server-side rendering, Next.js internally uses the native fetch, which by default caches data at build time or request time, serving cached data from subsequent requests onwards.
To bypass the cache on every request, you can use the no-store filter in the fetch request.
For another approach, see: Next Js Build Time Slow
Implementing Error Handling
Implementing error handling is crucial for a good user experience, especially when rendering components on the client side.
You should anticipate potential issues such as network errors or missing data. This is crucial because network errors can occur at any time, and missing data can lead to a poor user experience.
Proper error handling provides appropriate fallbacks or error messages to handle these issues. A good error message should be clear and concise, providing the user with enough information to understand what went wrong and how to proceed.
Fallbacks can be used to display a default value or a placeholder when data is missing. This can be especially useful when displaying a list of items, where missing data can cause the list to be incomplete or inconsistent.
A unique perspective: Speed up a Next Js Application Fetching Data
React Data Fetching
React Data Fetching is a crucial aspect of building dynamic web applications. Proper error handling is crucial for a good user experience, especially when rendering components on the client side.
You should anticipate potential issues such as network errors or missing data and provide appropriate fallbacks or error messages. This is especially important when fetching data from external sources.
Next.js and React make it easy to fetch data from the internet in your web applications. To fetch data during server-side rendering, Next.js internally uses the native fetch.
By default, data from fetch is cached at build time or request time, and cached data is served from the next requests onwards. This can be beneficial for improving performance, but it also means that changes to the data won't be reflected immediately.
You can use the no-store filter in the fetch request to bypass the cache on every request. This is useful when you need to ensure that the latest data is always fetched.
Broaden your view: Nextjs Fetch
Next.js also allows you to use a time-based cache with a revalidate filter to update the cache with new data. This can be especially useful when working with data that changes frequently.
To fetch data from external sources, you can use the getStaticProps function in Next.js. This function is used to fetch data at build time and return it as props to the page component.
Recommended read: Nextjs Clear Cache
Frequently Asked Questions
Can Next.js do client-side rendering?
Yes, Next.js supports client-side rendering, which can be implemented using React's useEffect() hook in pages. This alternative approach allows for dynamic rendering on the client-side.
Sources
- https://www.dhiwise.com/post/nextjs-client-side-rendering-what-you-need-to-know
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
- https://www.telerik.com/blogs/pre-rendering-data-fetching-strategies-next-js
- https://nextjs.org/docs/app/building-your-application/rendering
- https://blog.appsignal.com/2023/12/13/server-side-rendering-with-nextjs-react-and-typescript.html
Featured Images: pexels.com