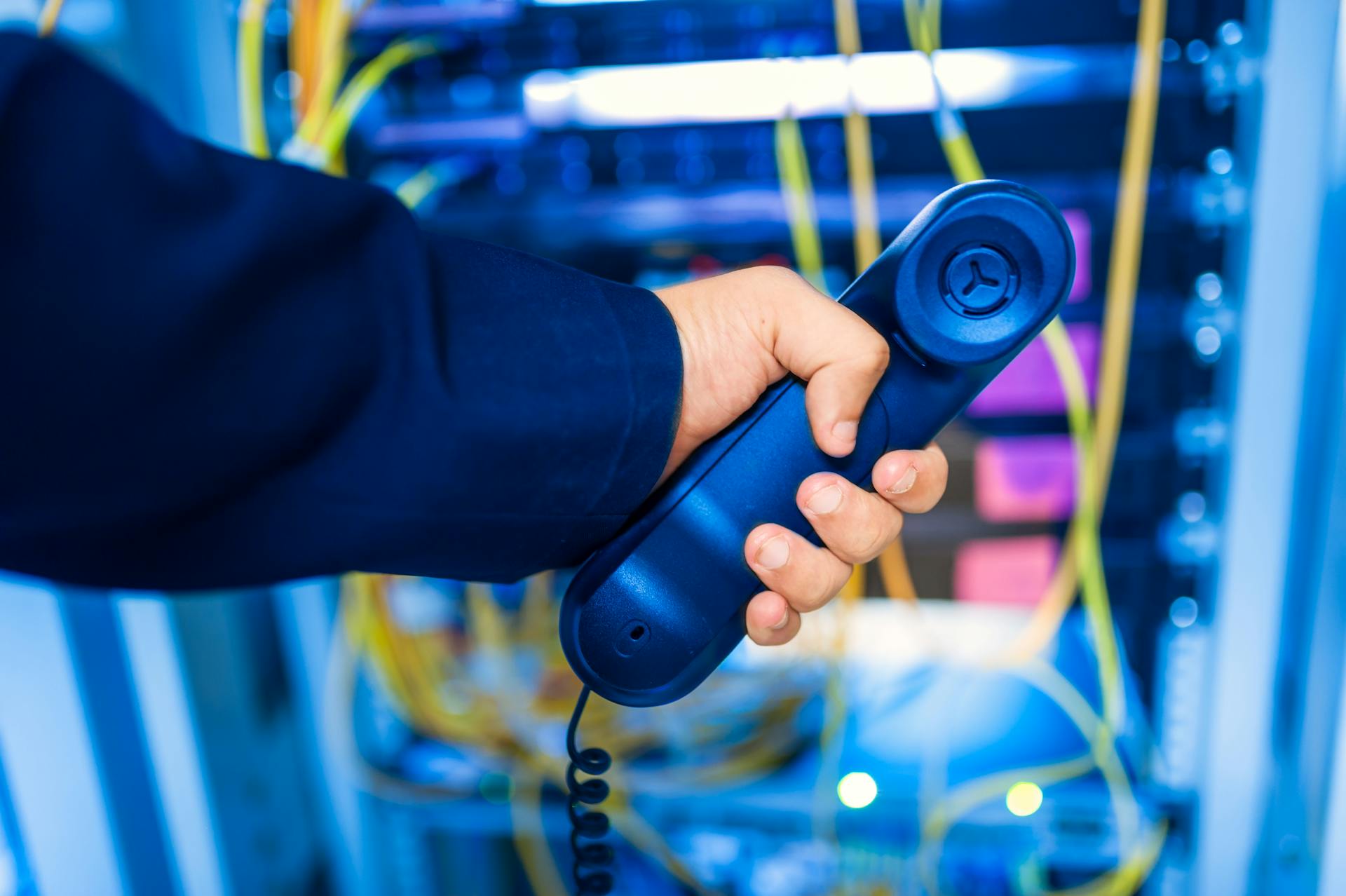
In Next.js Server-Only mode, the code structure is simplified and optimized for server-side rendering.
The `pages` directory remains the same, containing all your route components.
In a typical Next.js project, the `getStaticProps` method is used to pre-render pages at build time.
With Next.js Server-Only, this method is not needed, as pages are rendered on the server for each request.
This approach reduces the initial page load time and improves SEO.
The `getServerSideProps` method, on the other hand, is used to fetch data on the server for each request.
In Next.js Server-Only, this method is used to handle dynamic data fetching and server-side rendering.
By using `getServerSideProps`, you can ensure that your pages are always up-to-date and dynamic.
The `useEffect` hook is also used to fetch data on the client-side, but in Next.js Server-Only, it's recommended to use `getServerSideProps` instead.
This approach provides better performance and reduces the risk of data inconsistencies.
On a similar theme: Nextjs Server Rendering Tailwind
Isolating Server-Only Code
Isolating server-only code is crucial to prevent sensitive data exposure and security vulnerabilities. The challenge arises because JavaScript modules can be shared between server and client components, leading to the unintentional inclusion of server-side code in the client bundle.
Curious to learn more? Check out: Use Client Nextjs
Server-only code should be isolated by calling it within `getServerSideProps()`, which is the recommended way. This approach ensures that server-only code will neither be bundled nor invoked on the client side.
To isolate server-only code via module import alias, organize it in a separate folder, such as `src/SERVER_ONLY_MODULES`. Access server-only code using import aliases, like `@SERVER_ONLY_MODULES`.
When accessing server-only code, use a utility like `getMakeXhrCacheHelper()` to defensively check for the existence of the module. This is necessary because the `import()` statement would not resolve to the actual module on the client browser.
You can isolate server-only module imports for client bundle builds by overriding the Webpack config via `next.config.js`. This involves providing configuration to support the `@SERVER_ONLY_MODULES` import alias and resolving it to an empty module on the client browser.
Here's a summary of the two approaches to isolate server-only code:
Server Code Structure
Server code should be isolated to prevent leaks to the client. This can be achieved by using the server-only package, which throws an error if server-side code is imported into a client component.
Here's an interesting read: Next Js Debugging
To ensure server-only code is not bundled with client-side code, use getServerSideProps() to call server-only modules. This approach allows Next.js to perform tree-shaking, preventing server-only code from being invoked on the client side.
Server actions can be defined in separate files using the module-level use server directive, making it easier to organize server-side code. This approach ensures all functions in the file are treated as Server Actions, preventing accidental client-side execution.
See what others are reading: Next Js Client Side Rendering
Implementing Server Code
Implementing server code can be a challenge, especially when it comes to isolating sensitive information and business logic. This is where server-only code comes in, which is intended to be executed only on the server side.
Server-only code might include modules or functions that use server-specific libraries, access environment variables containing sensitive information, interact with databases or external APIs, or process confidential business logic.
The challenge arises because JavaScript modules can be shared between server and client components, leading to the unintentional inclusion of server-side code in the client bundle. This can expose sensitive data, increase the bundle size, and lead to potential security vulnerabilities.
To navigate this challenge, you can use the getServerSideProps() function to isolate server-only modules. This is the recommended way to ensure that server-only code is not bundled or invoked on the client side.
Here are the benefits of using getServerSideProps() to isolate server-only code:
- Server-only code is called only within getServerSideProps(),
- Next.js performs tree-shaking for getServerSideProps(), thus ensuring that server-only code will neither be bundled nor invoked on the client side.
Alternatively, you can use the server-only package to safeguard your application. This package will throw an error if someone tries to import server-side code into a client component, preventing the leak of sensitive information.
Note that this approach also works for getStaticProps(), and you can manually isolate server-only code if needed.
If this caught your attention, see: Nextjs Code Block
Defining Server Actions in Separate Files
To define Server Actions in separate files, you can use the module-level use server directive. This directive treats all functions in the file as Server Actions.
Using this approach, you can keep your Server Actions organized in separate files, making it easier to manage your code.
By defining Server Actions in separate files, you can avoid cluttering your main code with server-side logic. This helps maintain a clean and scalable code structure.
For example, you can create a file called "Actions" and define your Server Actions there. This is a good practice to follow when working with Server Components.
Explore further: Nextjs Server Actions File Upload
Server Components
Server Components are a game-changer for Next.js, allowing you to move server-side code to the edge of the network, improving performance and security.
To safeguard your application, you can install the server-only package, which will prevent server-side code from leaking to the client.
Server Components can't use event handlers, which adds complexity when dealing with event handlers in Next.js. This is where Server Actions come in, providing a workaround to make event handling possible.
To use Server Actions, you need to create a file in your Actions folder, like deleteTodo, and paste the code that defines the delete Server Action. This code takes in the id of the item to delete and deletes it from the database.
You can then wire up the Server Action in your Server Component by passing it to a form element, like a delete button, to trigger the action when clicked.
Server Components can be used to improve performance and security, but they require a different approach to event handling. With Server Actions, you can make event handling possible and create a seamless user experience.
You might enjoy: Next Js Route Handlers
Background and History
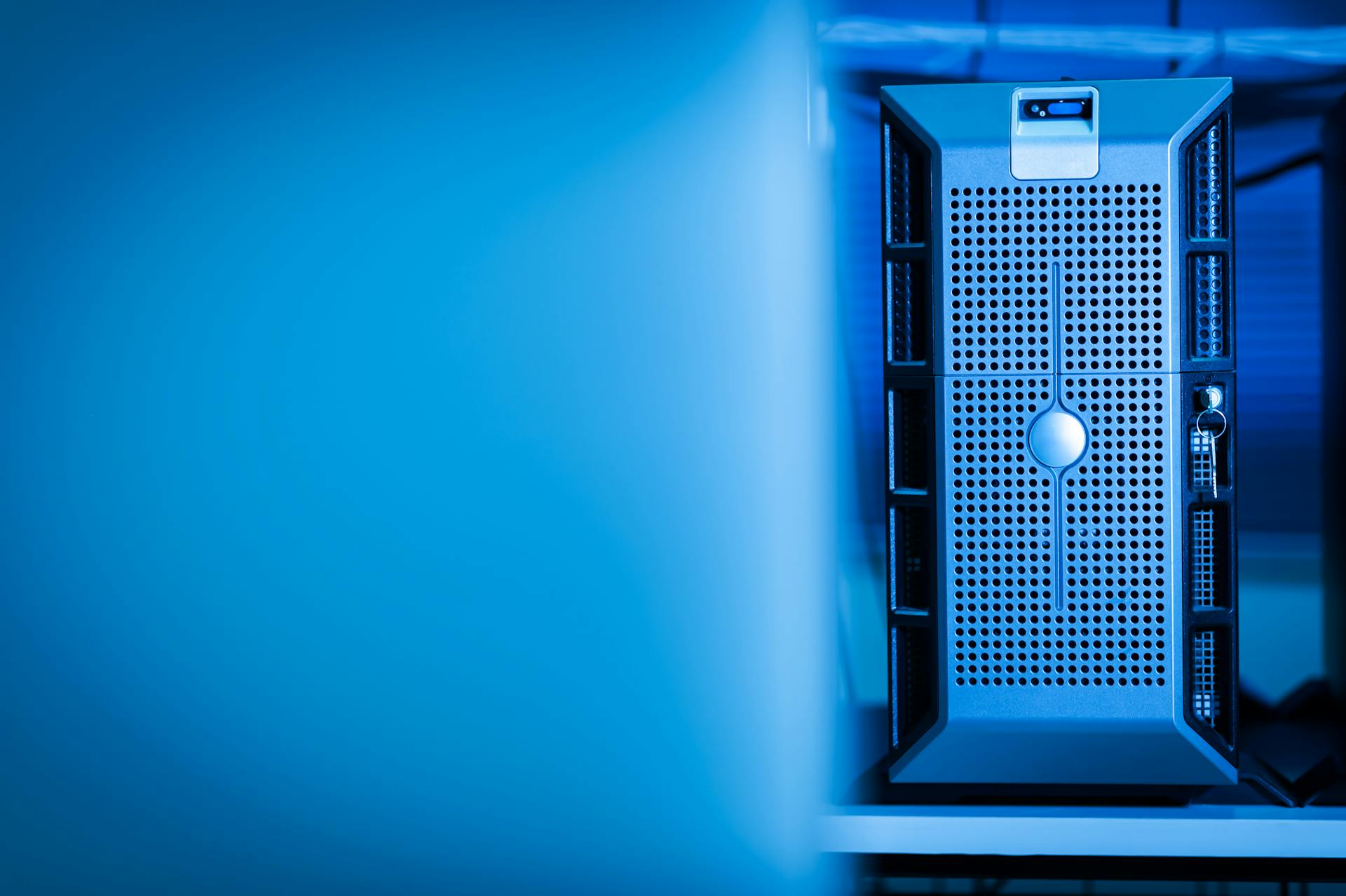
Next.js Server-Only was first introduced in Next.js 9.3, allowing developers to build server-rendered applications without client-side JavaScript.
The move to server-only was a significant shift from Next.js' traditional client-side rendering approach, which was introduced in Next.js 7.
Server-only mode enabled developers to take advantage of server-side rendering without the need for client-side JavaScript, making it an attractive option for developers looking to improve SEO and user experience.
This change was made to address the limitations of client-side rendering, such as slower page loads and increased load times.
With server-only mode, developers can now build applications that render on the server, providing a faster and more seamless user experience.
By leveraging server-side rendering, developers can improve the overall performance and scalability of their applications.
Discover more: Next Js Strict Mode
Sources
- https://www.builder.io/blog/server-only-next-app-router
- https://stackoverflow.com/questions/61611405/how-to-best-import-server-only-code-in-next-js
- https://nossbigg.github.io/2021/09/13/nextjs-isolate-server-only-modules.html
- https://www.codemancers.com/blog/2024-02-08-server-actions/
- https://blog.logrocket.com/diving-server-actions-next-js-14/
Featured Images: pexels.com