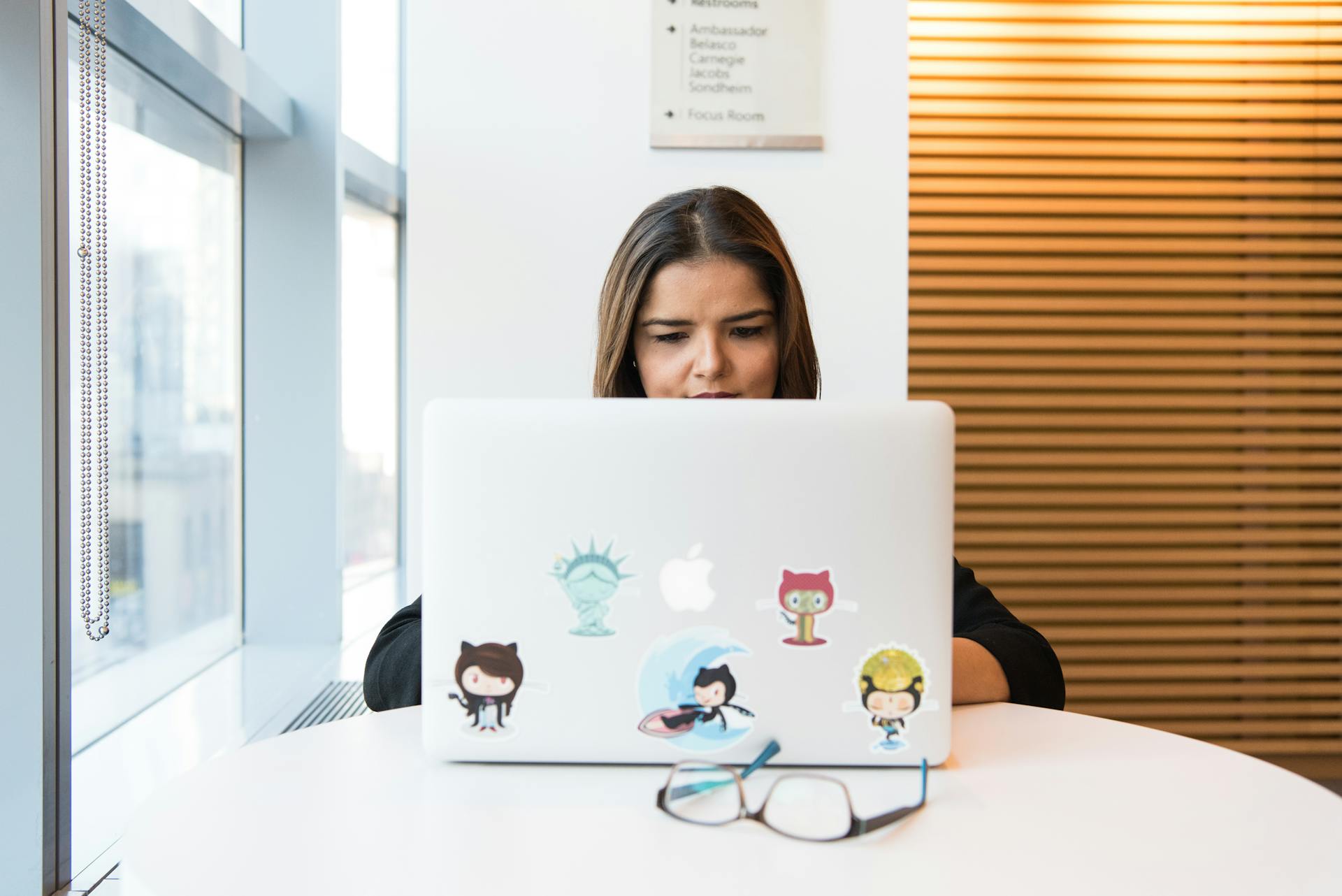
Route handlers are the backbone of Next.js routing, allowing you to define how your application responds to different URLs.
Next.js route handlers use a file-based approach, where each file in the pages directory represents a route. This makes it easy to manage and organize your routes.
By using a file-based approach, you can quickly create and modify routes without having to write complex code.
In Next.js, route handlers can also be defined using API routes, which allow you to handle API requests and return data in a JSON format.
Expand your knowledge: Next Js Using State Context
What Are Next.js Route Handlers?
Next.js route handlers are an essential part of building web applications with this popular framework. They allow developers to define and manage routes for their web applications using TypeScript/JavaScript files that contain handler functions.
You can define routes in Next.js by creating a file for each page, such as pages/users.js. The Remix routing system, on the other hand, is built on top of React Router and also uses handler functions to define routes.
Curious to learn more? Check out: Parallel Routes Next Js
Both Next.js and Remix support route-based code splitting, which means each route loads only the code necessary for that particular page. This practice significantly boosts site performance by reducing the amount of code that must be loaded on the initial execution of the application.
Route-based code splitting is a powerful feature that can improve the performance of your web application. It's especially useful for applications with many routes, as it reduces the amount of code that needs to be loaded.
You can also use nested routes in both Next.js and Remix, allowing pages and components to be created within folders in such a way that the components created with a nested folder structure within the directory will be treated as nested routes of a defined parent route.
Here are some key similarities between Next.js route handling and the Remix routing system:
- Defining and managing routes: Both frameworks allow developers to define and manage routes using handler functions
- Route-based code splitting: Both frameworks use route-based code splitting to improve site performance
- Nested route support: Both frameworks support nested routes, allowing for a folder structure within the directory
Getting Started with Next.js Route Handlers
To get started with Next.js route handlers, you'll first need to create a new Next.js application. This can be done with the following commands in your CLI:
```
npx create-next-app router-handler
cd router-handler
npm run dev
```
This will create a Next.js project folder called "router-handler" using Next.js v13.2. In this folder, you'll find the app directory, which contains the api directory and an example API route hello generated by default.
The api directory in the app folder is where you'll define your API routes. Each file in this directory corresponds to a specific route, as you'll see in the example of creating API routes in Next.js.
To create a basic API route, navigate to the pages/api directory, create a new file with a name that matches the desired API route, and define the route handler. The route handler function takes two parameters: req (the incoming request object) and res (the response object).
Here's an example of a basic route handler that sends a JSON response with a status code of 200 and a message:
```javascript
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, World!' });
}
```
By following these steps, you'll be well on your way to creating Next.js route handlers that meet your needs.
On a similar theme: Next Js Create App
Advanced Routing Techniques in Next.js
As you become more comfortable with the basics of routing in Next.js, you can start to explore some of the more advanced techniques that the framework offers. These techniques provide you with greater flexibility and control over how requests are handled, enabling you to build more complex and dynamic applications.
Next.js allows developers to define and manage routes for their web applications using TypeScript/JavaScript files that contain handler functions. This is similar to how Remix handles routing, as both frameworks use a similar approach.
Route-based code splitting is a key feature of Next.js, which means that each route loads only the code necessary for that particular page. This practice significantly boosts site performance because it reduces the amount of code that must be loaded on the initial execution of the application.
Both Next.js and Remix have support for nested routes, allowing pages and components to be created within folders in such a way that the components created with a nested folder structure within the directory will be treated as nested routes of a defined parent route.
You can create flexible API endpoints with Next.js by using dynamic API routes. This feature makes your API more versatile and user-friendly.
Readers also liked: Intercepting Routes Next Js Example
Security and Authorization in Next.js
Route protection is crucial for maintaining the security of sensitive information or functionality in your Next.js application.
To create secured routes, you need to use middleware functions that authenticate and authorize the requests made to the route before allowing access to it. This is typically done by examining the incoming request headers, cookies, query parameters, etc., and determining whether the user is authorized to access the requested resources.
A middleware function will return a 401 or 403 status if the user is not authorized, preventing unauthorized access to sensitive data or functionality.
To install the necessary package, you can enter the following command in the CLI: `npm install next-auth`. This will allow you to create secured API routes in the `api` directory.
The authentication option with its provider is defined in a file called `[...nextauth].js` within the `auth` folder. For example, using Google authentication providers, the option will be defined as follows:
Take a look at this: Next Js Cookies
```javascript
module.exports = {
// ...
providers: [
Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
// ...
};
```
Common authentication patterns used to secure routes in Next.js include:
- Session-based authentication: Sessions are created and saved in the browser for a specified amount of time. When a user logs in, a session is created, and if the browser is closed and opened again, the user doesn’t need to log in again.
- Token-based authentication: Users receive a unique token upon successful authentication, which is then sent to access routes that require authentication.
- OAuth and third-party provider authentication: This process allows users to log in using their credentials from third-party providers like Google, Facebook, or GitHub.
- Multi-factor authentication (MFA): An extra layer of security is added by requiring users to provide additional verification, such as a temporary code sent to their mobile device, in addition to their standard credentials.
Server-Side Logic and Middleware in Next.js
Server-side logic is a crucial aspect of Next.js development, and it's handled through API routes in the pages/api directory. These routes can be used to define functions for different HTTP methods like GET, POST, PUT, etc.
API routes are created by adding files to the pages/api directory, and they act as server components where you can define functions to handle incoming requests. For instance, to create an API route that handles user data, you might create a file named user.js in the pages/api directory.
Middleware in Next.js allows you to run code before a request is completed and can be used to modify the request or response, or to execute side effects. This is a powerful feature for tasks like authentication, redirects, and more.
Middleware functions can be used to abstract reusable code that runs before the handler is invoked. They are also essential for error handling, which is crucial in API routes.
On a similar theme: Next Js App vs Pages
Here are some key characteristics of middleware functions:
- Middleware functions can be used to abstract reusable code that runs before the handler is invoked.
- Error handling is crucial in API routes. Use try-catch blocks to catch and handle errors effectively.
Next.js also provides features to use server-side dynamic functions, such as cookies, headers, and redirects. Dynamic functions are executed on the server side when defined routes are accessed, making them suitable for handling tasks like data upload or requesting and accessing a database.
Static Route Handlers, on the other hand, allow developers to generate static content for specified routes, which is created at build time. This is particularly useful for frequently accessed routes containing content that won’t change often and can be pre-rendered ahead of time.
Additional reading: Next Js Client Side Rendering
Error Handling and Caching in Next.js
Error handling is a crucial aspect of building reliable Next.js API routes.
It ensures that your API endpoints can handle unexpected errors and provide clear error responses. This helps maintain a good user experience, which is essential for any application.
Caching API routes can improve performance by reducing the number of requests to your API. Next.js provides built-in support for caching API routes using the cache-control header.
Discover more: Next Js Error Boundary
Error Handling in Next.js
Error handling is a crucial aspect of building reliable Next.js API routes. It ensures that your API endpoints can handle unexpected errors, provide clear error responses, and maintain a good user experience.
By implementing error handling, you can prevent crashes and downtime, and instead return user-friendly error messages that help users recover from errors. This is especially important for APIs, where errors can be particularly frustrating for users.
Error handling also helps you debug and identify issues more efficiently. By catching and logging errors, you can track down problems and fix them before they become major issues.
For your interest: Nextjs Error Page
Caching in Next.js
Caching in Next.js can significantly improve the speed and efficiency of your API routes. By reducing the number of requests to your API, caching can help prevent bottlenecks and improve overall performance.
Next.js provides built-in support for caching API routes using the cache-control header. You can add the following lines to your now.json file to cache API routes for one hour.
Implementing caching strategies can make a huge difference in the performance of your Next.js API routes.
Check this out: Nextjs Performance
Comparison with Other Frameworks and Systems
Next.js stands out from other frameworks like React Router in its route handling capabilities. Next.js offers a flexible routing system that automatically splits code for each page, improving performance.
One of the key advantages of Next.js is its built-in API routes. This feature allows for convenient management of server-side logic within the project structure.
Next.js also supports both dynamic and static route generation, making it efficient for handling various content pages.
Let's take a look at some of the key differences between Next.js and other frameworks:
Overall, Next.js provides a more comprehensive and efficient route handling system compared to other frameworks.
Sources
- https://blog.logrocket.com/using-next-js-route-handlers/
- https://www.dhiwise.com/post/next-js-route-handlers-best-practices-for-improved-performance
- https://nextjs.org/docs/app/building-your-application/routing/route-handlers
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
Featured Images: pexels.com