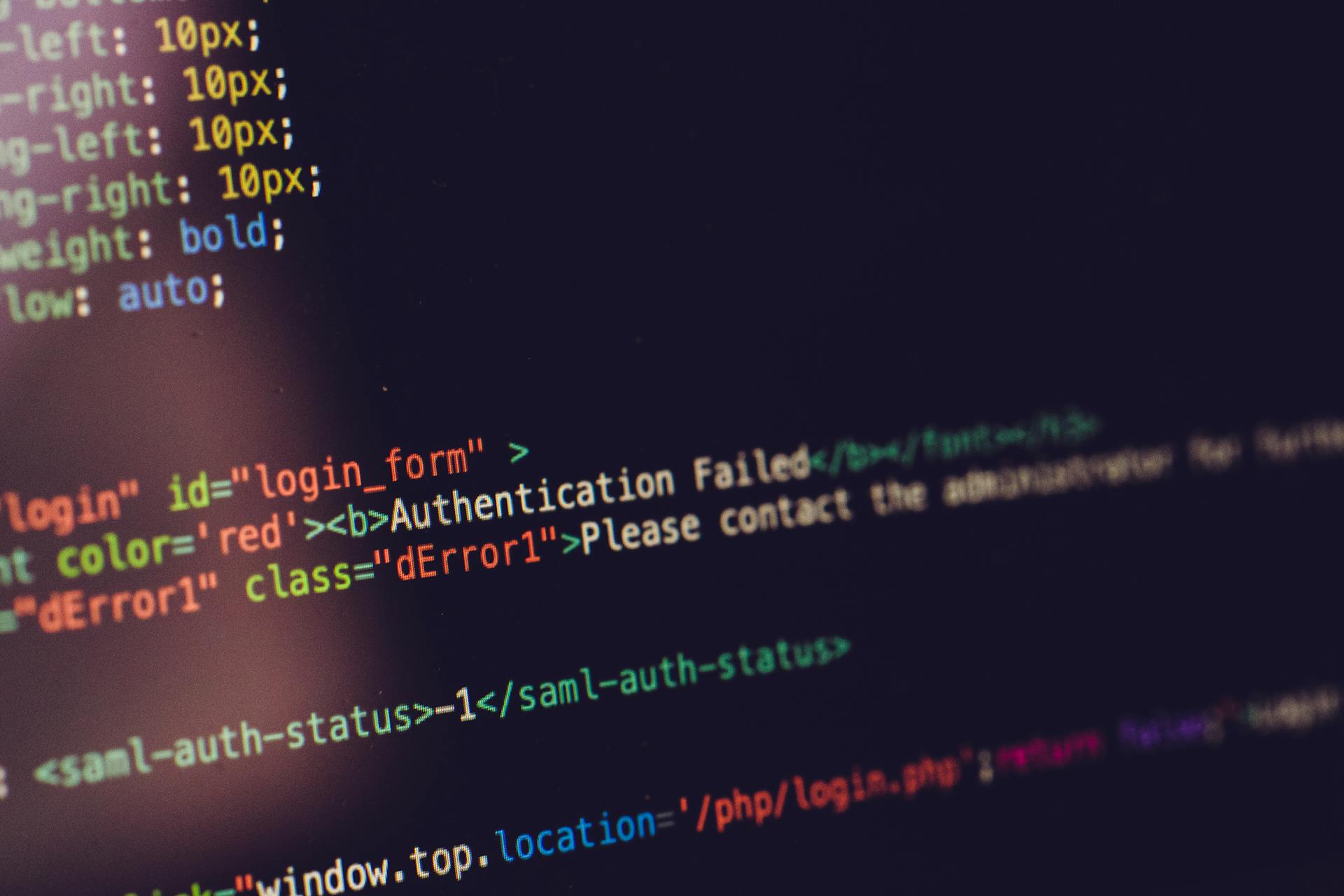
So, you're trying to decide between using a NextJS app and NextJS pages for your project? Well, let's break it down.
NextJS apps are great for complex applications with many features and integrations, as they allow for more flexibility and customization.
If you're building a simple website or blog, NextJS pages might be the way to go, as they provide a more straightforward and easy-to-use approach.
NextJS apps can handle multiple pages and routes with ease, making them perfect for larger applications that require more complexity.
Creating Your First Page
You'll need to create a new JavaScript file under the pages subdirectory to represent your first page.
Each file under the pages directory exports a React component that Next.js uses to render the page, and the default root route is index.js.
Go ahead and create this file under pages subdirectory, and without having to restart the app, Next.js detects the new index.js and the exported Index component and updates the view in the browser automatically.
You can think of the implied parent or shell component for the whole application as being implied through the pages subdirectory and hierarchy.
To achieve a uniform core interface for each page, you'd want to have a common layout that includes a header, a content container, and a navigation bar, just like you'd do with create-react-app using components.
Routing Basics
Routing is a critical aspect of web apps that enables users to move between various pages.
The Pages Directory and App Router are two crucial components that determine how users navigate through a Next.js application.
With Next.js, each page is represented by a JavaScript file under the pages subdirectory, and each file exports a React component that is used by Next.js to render the page.
To create a new page, simply create a new file under the pages directory, and Next.js will automatically detect it and update the view in the browser.
Here's a list of the basic routing steps:
- Create a new file under the pages directory to represent a new page.
- Export a React component from the new file that will be used to render the page.
- Next.js will automatically detect the new file and update the view in the browser.
The App Router is a powerful tool that enables developers to create dynamic and efficient single-page applications (SPAs) with a comprehensive set of features that simplify routing.
Creating a new page with the App Router involves nesting a page.js file within the appropriate folder to define your route.
Directory Function
The Pages Directory in Next.js automatically creates routes within the pages folder, allowing for efficient route management.
This approach maintains developer familiarity with how routing works, making it easier to transition between different routing mechanisms.
The key difference between the Pages Directory and the App Router lies in route generation, with the Pages Directory organizing routes within the pages folder, while the App Router organizes them within the app folder.
How the Directory Works
The Pages Directory is a crucial part of setting up routing in a Next application.
To set it up, you must first create a Next application by running a command on your local or code editor's terminal.
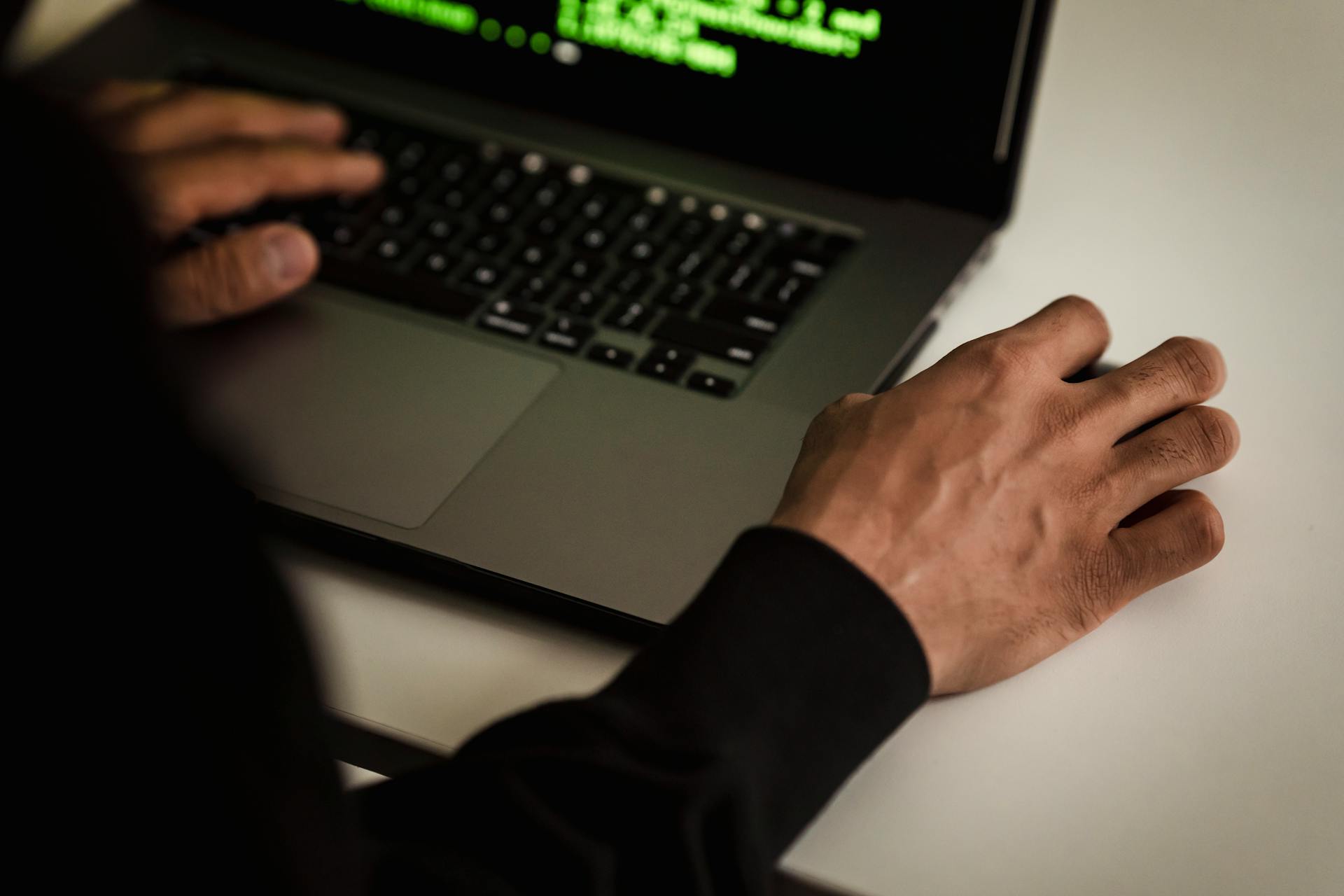
This command creates a list of configuration options, where you should select "No" for App Router during application setup.
By doing so, a pages folder is automatically created, which is where you can create routes.
To create a route, you need to create a folder within the pages folder and name it whatever you want the route to be, such as "about".
This folder will serve as the foundation for your route, allowing you to create the necessary files and configuration to bring it to life.
Directory Limitations
The Pages Directory, a great tool for structuring routes in Next.js, has some limitations to keep in mind.
Static exports are limited, relying on dynamic routes generated using getStaticProps and getStaticPaths. This means not all pages can be statically exported.
Edge Runtime configuration requires extra setup beyond what's possible in the Pages Directory.
Internationalization routing is supported, but you need to configure locales, default locales, and domain-specific locales in the next.config.js file, not the Pages Directory.
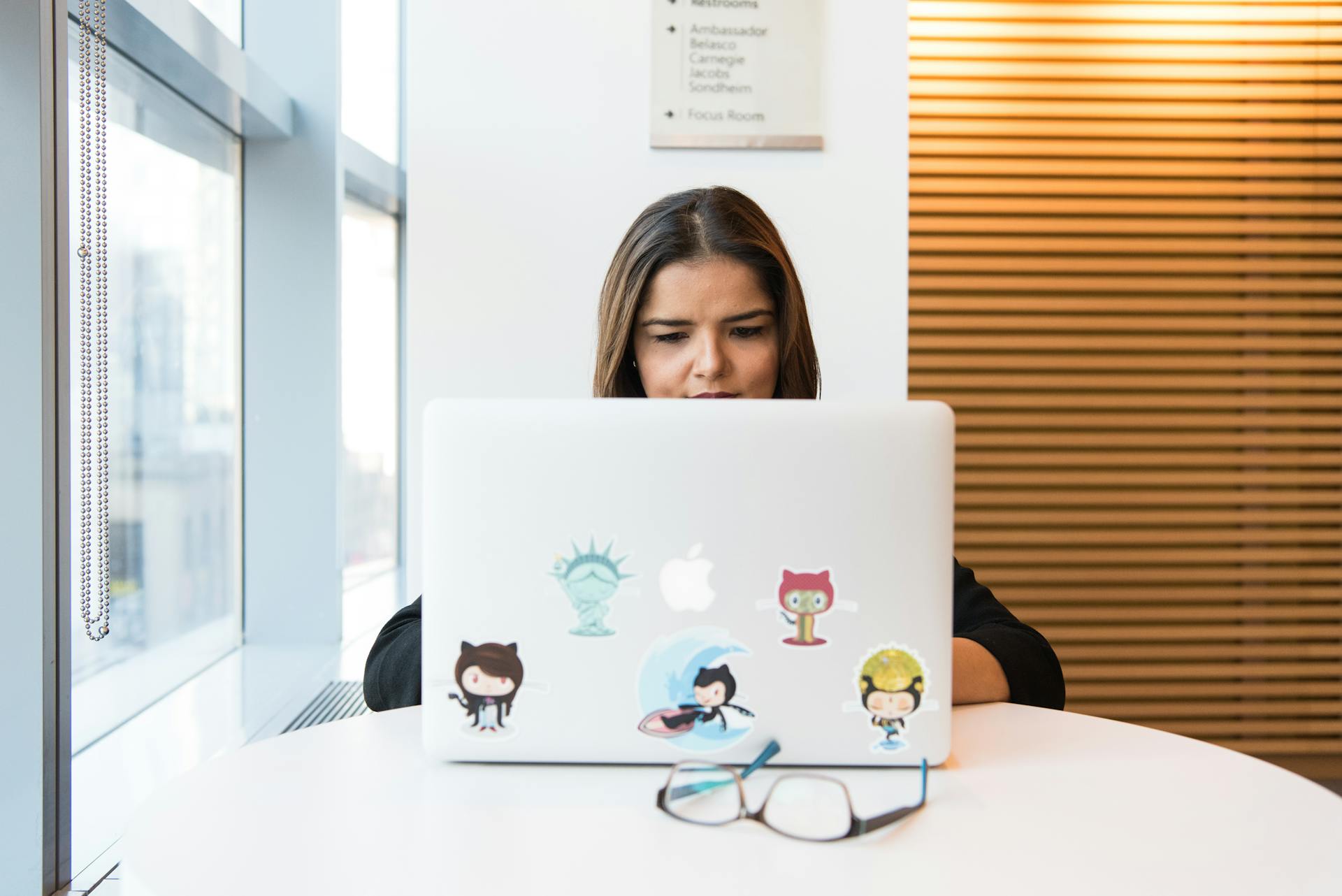
Serverless functions in the Pages Directory can handle basic API functionality, but more complex functions need extra configuration and alternative file placement.
Statically generated pages may not be optimized for visitors without additional CDN configuration or "vendoring", impacting performance and distribution.
Here are some key limitations to consider:
- Static exports rely on dynamic routes generated using getStaticProps and getStaticPaths.
- Edge Runtime configuration requires extra setup.
- Internationalization routing requires configuration in next.config.js.
- Serverless functions need extra configuration and alternative file placement.
- Statically generated pages may require additional CDN configuration or "vendoring" for optimal performance and distribution.
Directory vs Which
Choosing between the Pages Directory and the App Router requires understanding their unique strengths and considerations.
In the rapidly evolving world of web development, it's easy to get caught up in the hype surrounding new technologies and tools. However, striking a balance between excitement and caution is crucial.
The Pages Directory is a great option for projects with simple routing needs, as it provides a straightforward way to manage routes.
Both options have their own strengths and considerations, and understanding your needs and goals will help you determine which one to use in a given context.
The App Router, on the other hand, is better suited for complex projects with multiple features and integrations, offering more flexibility and customization options.
Ultimately, choosing between the Pages Directory and the App Router depends on your project's specific requirements and your team's expertise.
Features and Components
Next.js App Router is a powerful tool that enables developers to create dynamic and efficient single-page applications (SPAs). It simplifies routing and makes it easy to build complex applications.
Apart from routing, App Router boasts a range of other features, including comprehensive routing and the ability to create dynamic and efficient single-page applications.
Creating More
To create more pages with Next.js, you'll need to add new files under the pages directory. This is where the magic happens, and you can start building out your application's routing.
Each new page requires a corresponding file in the pages directory. For example, if you want to create a page for exploring products, you'll need to create an explore.js file.
As you add more pages, you can create routes for each view. For instance, you might want to create routes for /explore, /nearme, /mycart, and /profile.
Here are the routes you can create:
- /explore
- /nearme
- /mycart
- /profile
To see your new pages in action, simply visit the corresponding route in your browser, such as http://localhost:3000/explore.
Layout Component
A layout component is a versatile UI element that shapes a page's structure, including components like headers, footers, and sidebars.
It can even offer shared functions like navigation, making it a convenient choice for developers.
Layout components work with routing, enabling smooth transitions between app pages, and retain their state when routes change.
This helps ensure consistent and reusable layouts with minimal effort.
The layout component is designed to receive a children prop and wrap all page files in the same directory with it.
In fact, a layout component can be shared by multiple pages, as seen in the example where the layout.js component is shared by both the user and settings pages.
Template Components
Template components are like layouts, but they create a new instance for each child on navigation. This means recreating DOM elements, losing state, and resetting effects every time the route changes.
You can use them for things like tracking page views or interactive widgets. They're perfect for scenarios where you need to track user interactions or display dynamic content.
To create a template, export a default React component from a template.js file. This component should be designed to receive a children prop.
Server Components
Server components are a game-changer for Next.js projects, allowing you to render on the server and stream to the client, which accelerates page loading and enhances performance.
They work by loading different page contents in small chunks and independently, making your pages load faster.
One thing to keep in mind is that server components don't support client-side actions like click events and React hooks (useState, useRef).
To convert a server component into a client component, simply mark it with use client at the beginning of the file.
Server Components and Data
Server components bring a fresh data fetching pattern, allowing async components to fetch data within them.
This reduces reliance on APIs like getServerSideProps, making it easier to manage data fetching within your components.
To fetch data, simply mark a component as async and use the fetch function within, like this: Using the fetch function in a server component.
The App Router caches the fetched data on the server, eliminating the need to re-fetch that data on every request, unless a revalidation parameter is passed in in the fetch function.
Data Fetching
Server components bring a fresh data fetching pattern, allowing async components to fetch data within them.
This reduces reliance on APIs like getServerSideProps. Mark a component as async and use the fetch function within to fetch data. The App Router also caches the fetched data on the server, eliminating the need to re-fetch that data on every request.
A revalidation parameter can be passed in the fetch function to cause a re-fetch of new data. For example, using the fetch function with a revalidation parameter set to every 5 seconds causes a re-fetch of new data every 5 seconds.
Transitioning Between States
Transitioning between states can be a complex process, especially when dealing with server components and data. This is because server components can exist in multiple states, including the "Ready" state, where they are prepared to receive requests, and the "Busy" state, where they are actively processing requests.
In a distributed system, state transitions can occur due to changes in the system's configuration, such as the addition or removal of nodes. For example, when a new node is added to a distributed system, it will transition from the "Offline" state to the "Ready" state, where it can start receiving requests.
State transitions can also be triggered by external events, like network failures or node crashes. For instance, if a node crashes, it will transition from the "Ready" state to the "Offline" state, and other nodes in the system will need to adjust their state accordingly.
The type of state transition that occurs depends on the specific system configuration and the type of event that triggers the transition. For example, in a system with multiple nodes, a node failure might trigger a state transition to a "Recovery" state, where the system attempts to recover from the failure.
In a distributed system, state transitions can occur frequently, especially in systems with high node churn rates.
Route Management
Routing is a critical aspect of web apps that enables users to move between various pages. It guarantees that users can access different parts of an application, whether they are moving from a homepage to a product listing or navigating within single-page applications.
The Pages Directory and App Router are two crucial components that determine how users navigate through a Next.js application. The App Router is used to create routes, which involves creating folders within the app directory and nesting a page.js file within the appropriate folder.
Route Groups organize routes in the app directory without altering URL paths. Enclosing a folder name in parentheses creates a Route Group that keeps related routes together. This allows for logical grouping, nested layouts, and a clean URL structure.
Comparison and Migration
Migrating from Pages Router to App Router in Next.js can have several implications, including increased performance and improved flexibility. However, it also introduces a steeper learning curve due to the new set of concepts and APIs.
App Router can be more complex to set up and use than Pages Router, requiring a deeper understanding of React and Next.js. This can be a challenge for developers familiar with the Pages Router.
Here are the key differences between Pages Router and App Router to consider when making the switch:
To successfully migrate from Pages Router to App Router, follow the steps outlined in the step-by-step guide, starting with familiarizing yourself with the App Router concepts and APIs.
Migration Guide
Migrating from Pages Router to App Router can be a daunting task, but with the right approach, you can make the transition smoothly. The App Router introduces a new set of concepts and APIs that developers need to learn and understand.
To overcome the learning curve, it's essential to familiarize yourself with the App Router concepts and APIs. Read the official documentation, watch tutorials, and experiment with the App Router in a test project. This will help you grasp the new concepts and APIs.
The App Router requires a different approach to structuring and organizing the application's codebase. This can lead to confusion and errors if not done correctly. However, by following a step-by-step guide, you can ensure a successful migration.
Here are the steps to follow:
- Familiarize yourself with the App Router concepts and APIs.
- Gradually migrate your application's codebase to the App Router.
- Test your application thoroughly after each migration step.
- Update your application's deployment configuration to use the App Router.
Migrating to App Router can have several implications, including increased performance and improved flexibility. However, it can also increase complexity, especially for those who are new to App Router.
Comparing
Migration to the cloud can be a complex process, but comparing different cloud providers is a crucial step in making an informed decision.
Cloud providers like Amazon Web Services (AWS) and Microsoft Azure offer a wide range of services, including storage, computing, and analytics.
AWS has a strong focus on scalability and flexibility, making it a popular choice for businesses with fluctuating workloads.
Azure, on the other hand, offers a more comprehensive suite of services, including artificial intelligence and machine learning capabilities.
Ultimately, the choice between AWS and Azure depends on your specific needs and requirements.
Choosing the Right Tool
The choice between Next.js App and Pages Directory depends on your individual needs and objectives. Stability and user-friendliness are key concerns for the Pages Directory, making it a dependable option for simple routing tasks.
If you require more flexibility and customization, the App Router is a more suitable choice due to its advanced capabilities and programmability.
Here's a quick comparison of the key differences between the Pages Directory and the App Router:
Ultimately, staying knowledgeable about the capabilities and trade-offs of both options will help you make well-informed decisions for your project.
Selecting the Right Tool
Choosing the right tool for your project is crucial to its success. The choice between the Pages Directory and the App Router, or the Pages Router and the App Router, depends on your individual needs and objectives.
If stability and user-friendliness are your top priorities, the Pages Directory is a reliable option. It provides a solid foundation for simple routing tasks. You can also consider the Pages Router for simple applications with a few pages and a straightforward layout.
However, if you require more flexibility and customization, the App Router or App Router might be a better choice. They offer advanced capabilities and programmability, making them ideal for complex routing scenarios and true SSR for nested routes.
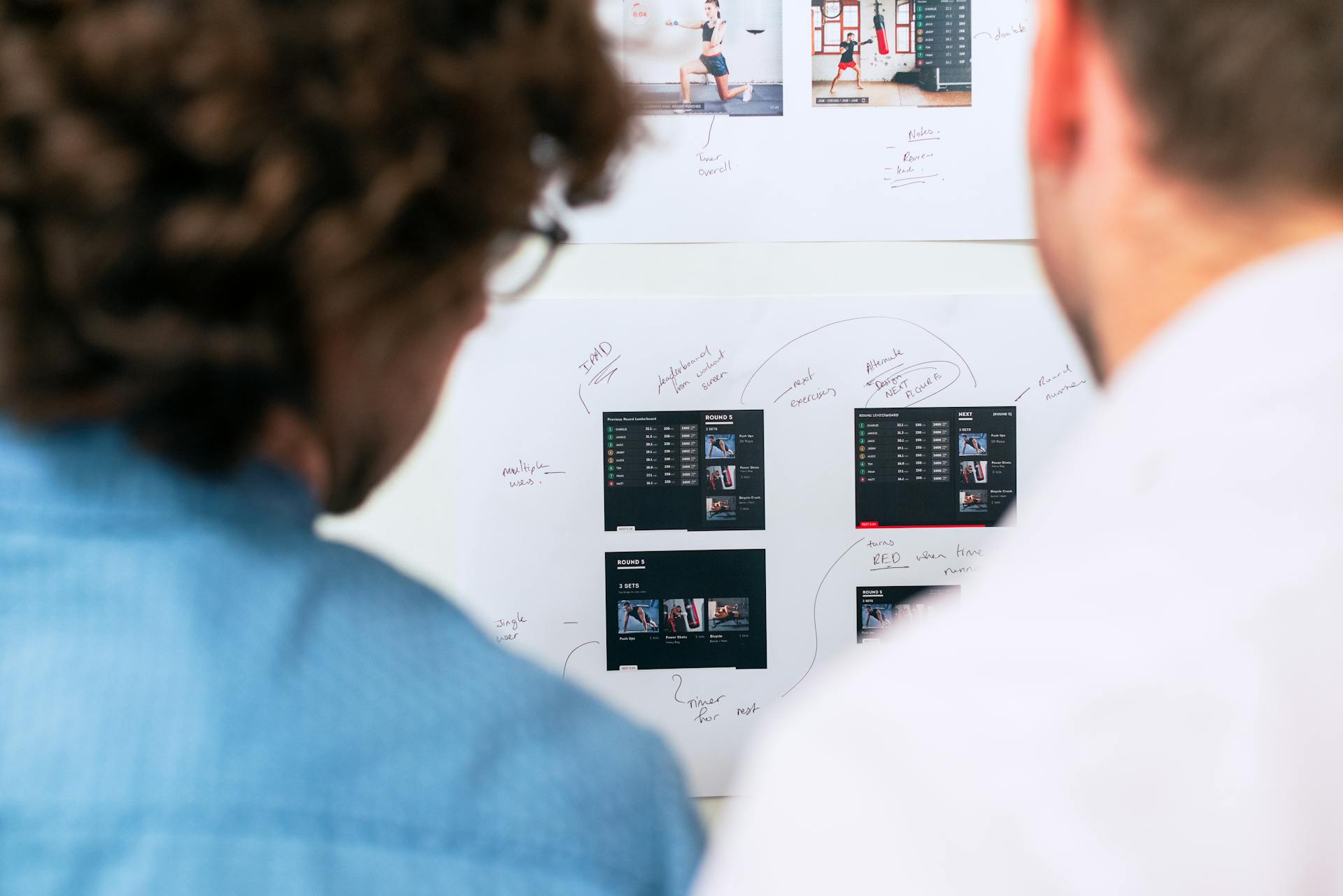
Here's a quick summary of the key differences between the Pages Directory and the App Router:
Ultimately, it's essential to stay knowledgeable about the capabilities and trade-offs of both the Pages Directory and the App Router to make well-informed decisions.
Adoption Pros and Cons
Adopting a new tool can be a game-changer, but it's essential to weigh the pros and cons before making a decision.
Next.js App Router is a great example of this, with several advantages that make it a compelling choice. It's a very efficient router that can improve the performance of your application.
However, there are some potential drawbacks to consider. Next.js App Router can be complex to set up and configure, especially for larger applications.
On the other hand, Next.js App Router is very easy to use and understand, even for beginners. This is a significant advantage, as it means you can get started quickly and start building your application without a lot of hassle.
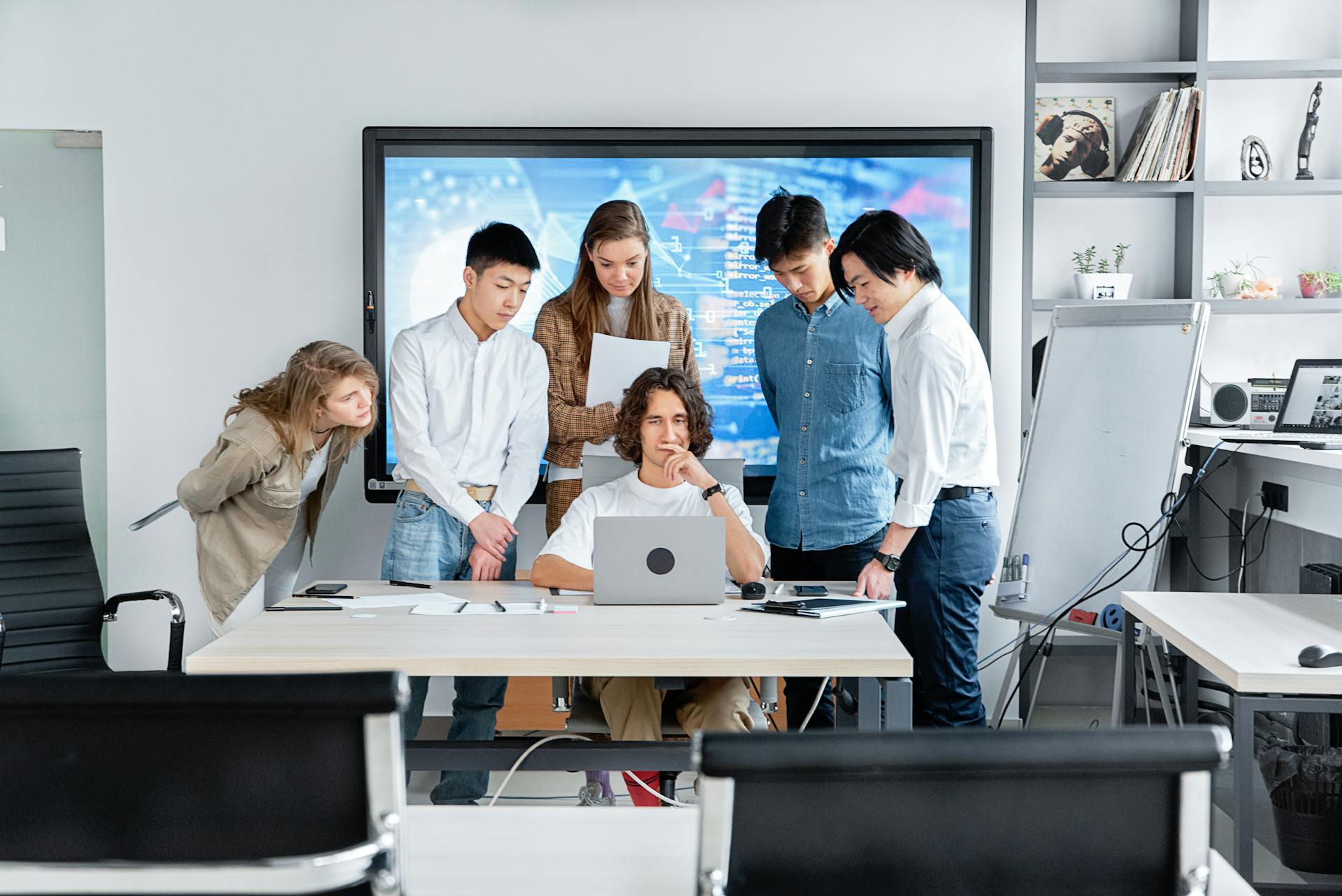
Here are some key pros and cons to consider:
- Improved performance: Next.js App Router can help improve the performance of your application.
- Easy to use: Next.js App Router is very easy to use and understand, even for beginners.
- Powerful features: Next.js App Router provides a comprehensive set of features that make it easy to build complex applications.
- Complexity: Next.js App Router can be complex to set up and configure, especially for larger applications.
- Lack of documentation: The documentation for Next.js App Router is not as extensive as it could be.
- Limited support for server-side rendering (SSR): Next.js App Router does not support SSR out of the box.
Overall, the decision to adopt Next.js App Router will depend on your specific needs and goals.
Frequently Asked Questions
Why use an app router instead of pages?
Use an App Router for complex routing scenarios where you need more control over your application's navigation. It's ideal for dynamic sites or apps with intricate routing needs.
Is Next.js good for apps?
Yes, Next.js is a great choice for building apps, offering built-in routing, data fetching, and optimization tools to boost performance and free up time for core functionality. With Next.js, you can focus on building a high-performance app without worrying about the underlying technical details.
What is the difference between Next.js routing and React routing?
Next.js routing uses file-based routing, whereas React Router employs a declarative style. This difference in approach affects how routes are configured and managed in each framework.
Sources
- https://www.freecodecamp.org/news/routing-in-nextjs/
- https://vercel.com/blog/common-mistakes-with-the-next-js-app-router-and-how-to-fix-them
- https://www.uxpin.com/studio/blog/nextjs-vs-react/
- https://auth0.com/blog/next-js-practical-introduction-for-react-developers-part-1/
- https://caisy.io/blog/nextjs-app-router-guide
Featured Images: pexels.com