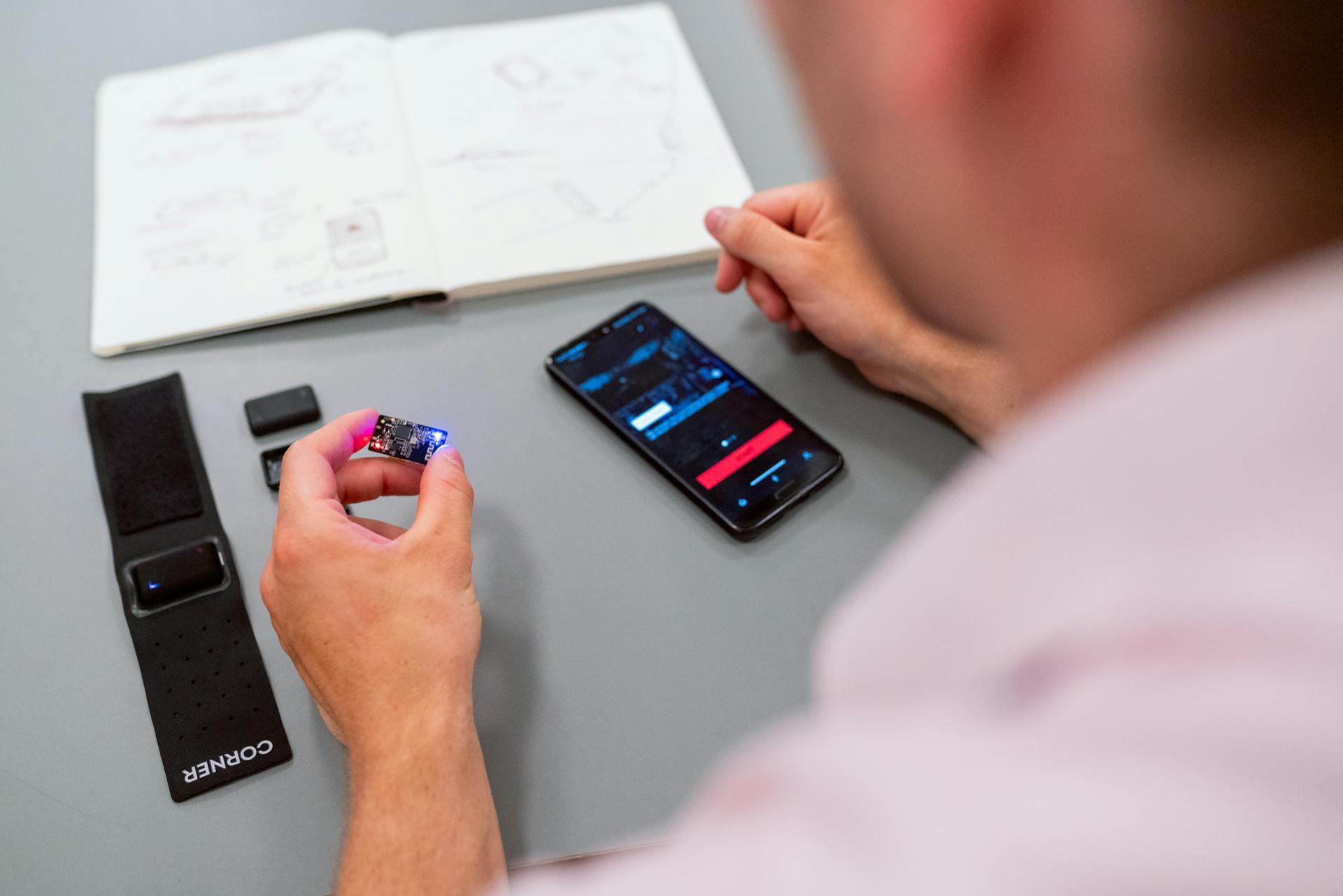
State management in Next.js 14 is now more streamlined than ever, thanks to the introduction of a new API route for fetching and updating data.
With the new `getServerSideProps` method, you can easily manage state by fetching data on the server and passing it down to the client.
This approach eliminates the need for complex state management libraries and makes your code more efficient.
In Next.js 14, you can also use the `useEffect` hook to fetch data on the client-side, allowing for more flexibility in your state management strategy.
By leveraging these new features, you can simplify your state management and focus on building a better user experience.
State Management Basics
State management in Next.js is a breeze, especially with the useState Hook. This Hook is a popular method for managing state in traditional React applications and works similarly in Next.js.
You can start by creating an application that allows users to increase their score by clicking a button. To do this, you'll need to include the following code in your index.js file: import the useState hook itself and set the initial state to be 0, then provide a setScore function to update the score later.
The useState Hook is a great way to manage state efficiently.
The Challenge
State management can be a challenge, especially in ecommerce websites where dynamism is crucial. Content changes relatively infrequently, but in ecommerce, it's essential to deliver information in real-time.
In the past, the common approach was directing browsers to fetch data from servers, which would then render to HTML on the client. This approach worked well with front-end frameworks like React.
However, with the introduction of new tools like React Server Components and Server Actions, developers need to think differently about how they build their React applications. This new paradigm requires a bit of a learning curve.
Ecommerce websites have to interact with a server that can deliver information in real-time, making it essential to optimize the storefront experience. To keep track of the user's Cart and optimize the storefront experience, client-side state and React Context were used in the past.
Statically generating and serving webpages via a CDN greatly benefits visitors, but ecommerce websites can't rely on this approach due to the need for real-time information.
Basic
In Next.js, state management is crucial for building robust and scalable applications. To begin with, you can use the useState Hook for managing state, just like in traditional React applications.
The useState Hook is a popular method for managing state in Next.js, and it works similarly to how it does in traditional React applications. To get started, you can create an application that allows users to increase their score by clicking a button.
To use the useState Hook, you need to import it and set the initial state to be 0. You also need to provide a setScore function so you can update the score later. For example, in the index.js file of the pages directory, you can include the following code:
Here's a breakdown of the useState Hook:
By using the useState Hook, you can easily manage state in your Next.js application.
It's worth noting that using the useState Hook can be sufficient for simple applications, but as your application grows, you may need to consider more advanced state management techniques, such as using Context providers or createSlice for Reducers and Actions.
Data Rehydration in SSR
Data rehydration is a crucial concept in server-side rendering, ensuring a seamless user experience by avoiding unnecessary data fetching and preserving the user's session.
In server-side rendering with Next.js and Redux Toolkit, data rehydration involves reconstructing the application state on the client-side. This process retrieves and deserializes the serialized state received from the server.
Rehydrating data helps maintain the user's session, which is especially important for applications that require users to log in or access sensitive information. By preserving the session, you can provide a more personalized and secure experience for your users.
Data rehydration also avoids unnecessary data fetching, which can improve application performance and reduce the load on your servers. This is particularly beneficial for applications with complex data structures or large datasets.
By incorporating data rehydration into your server-side rendering setup, you can create a more efficient and user-friendly application that meets the needs of your users.
Using Redux Toolkit in Components
The useState hook is great for managing state in functional components, but for more complex state management, we can use Redux Toolkit. This powerful tool provides a simplified way to create reducers and actions, making it easier to manage our application's state.
To get started with Redux Toolkit, we can use the createSlice function, which takes three parameters: the name of our slice, its initial state, and a reducers object containing all the reducers we want to perform on that slice. For example, we can create a profileSlice with an initial state of name: null, and a SET_NAME reducer that updates the name property of the state with the provided payload.
Using createSlice provides several benefits, including simplified reducer creation and easy state management. By using createSlice, we can easily create reducers and actions for our application's state, making it easier to manage and maintain our state logic.
Here's a summary of the benefits of using createSlice:
By using Redux Toolkit in our Next.js components, we can take advantage of these benefits and create a more maintainable and efficient state management system.
Requirements and Dependencies
To effectively manage state in your Next.js application, you'll need to meet some basic requirements.
First and foremost, you'll need Node.js installed on your system. This is a fundamental requirement for using Next.js.
Additionally, it's essential to have basic knowledge of Next.js and Redux. This will help you understand how to integrate Redux Toolkit with your application.
To get started, you'll need to install the required dependencies. Here are the specific requirements:
- Node.js installed on your system
- Basic knowledge of Next.js and Redux
With these prerequisites met, you'll be ready to integrate Redux Toolkit with your Next.js application.
Context API
The Context API is a powerful tool in Next.js that helps you manage state globally. It's recommended to use it when your app is complex and you need to access state across multiple levels.
You can create separate contexts for different states, such as authentication, user data, and theme. For example, you can create a theme context to manage the theme state of your app.
To create a context, you need to create a new file in a separate folder and include the Context object, ThemeProvider function, and initial value for the Context. The Context object is created with the createContext function.
You can then create a custom hook, useThemeContext, to access the theme state in individual pages or components. This hook allows you to access the theme state and update it when necessary.
To make the theme state globally accessible, you need to wrap the ThemeProvider around the entire app. This is done by importing the useThemeContext hook and accessing the theme state and setTheme function in the _app.js file.
The Context API also allows you to preserve state across routes. This means that when you switch between routes, the state is preserved and you can access it in both routes.
To avoid performance issues, it's a good practice to create multiple contexts for different pieces of state. This way, you can share state variables across components without causing unnecessary re-renders.
Data Fetching and Caching
Data Fetching and Caching is a crucial aspect of Next.js 14, and it's great that the built-in caching mechanism eliminates the need for keeping a global data state using Context or prop drilling.
Next.js has a caching mechanism built-in that automatically works with the native fetch API, caching every fetch request until revalidated. This means you can safely fetch the same data from every component that needs it without worrying about doing 20 duplicate requests.
The Medusa server already keeps track of the contents of the current shopping cart, and by retrieving this data, storing it in the Next cache, and then accessing that cached data from any component that might need it, you eliminate the need to manage the cart state in the client.
Revalidating the cart data whenever you modify it makes sure the cache always holds an updated version of the cart, accessible throughout the app. This leads to a responsive front-end performance when navigating through your app.
Fetching new data from the server is swift, and all subsequent requests load almost instantly from the cache. This is because Next.js's caching mechanism forces you to be meticulous about revalidation, which was not a concern previously.
Using SWR, a custom Hook library created by the Next.js team itself, simplifies many things, including syntax, scalability, and error handling.
Data Management
Data Management in Next.js 14 is a breeze, thanks to built-in caching and Server Actions. With Next.js's caching mechanism, every fetch request is automatically cached until revalidated, eliminating the need for keeping a global data state using Context or prop drilling.
You can safely fetch the same data from every component that needs it without worrying about doing 20 duplicate requests. This creates super fast interactions, but it also forces you to be meticulous about revalidation.
Server Actions are asynchronous functions executed on the server, which can manage form submissions and data mutations in Client Components. They trigger a POST request to the Next.js server, making them convenient to work with.
To create a Redux Toolkit slice, you need to use the createSlice function from @reduxjs/toolkit. This function takes three parameters: the name of your slice, its initial state, and a reducers object containing all the reducers you want to perform on that slice.
Using createSlice provides several benefits, including simplified reducer creation and easy state management. Here are the benefits of using createSlice:
Fetching and Caching
Fetching and caching in Next.js is a game-changer for responsive front-end performance. It eliminates the need for keeping a global data state using Context or prop drilling, making it a more efficient approach.
With Next.js's caching mechanism, every fetch request is automatically cached until revalidated. This means you can safely fetch the same data from every component that needs it without worrying about doing duplicate requests.
Re-fetching the same data multiple times in a component tree may seem counterintuitive at first, but it's a necessary pattern to ensure the cache is always up-to-date. You must remember to revalidate the relevant parts of the cache every time you mutate data on the server.
Fetching new data from the server is swift, and all subsequent requests load almost instantly from the cache. This leads to a responsive front-end performance when navigating through your app.
Here's a breakdown of the benefits of Next.js's caching mechanism:
Management
Server components can't directly use client-side hooks like useState() and useEffect(), but you can use libraries like Zustand for state management.
Server components dominate in the /app router, which means they run on the server during the initial request, giving you direct access to the server process and the ability to connect to a database.
You can still create traditional client-side components that interact with the browser environment by including the 'use client' statement at the top of the component file.
Server components can use server-side storage mechanisms like cookies or sessions for stateful data that must be persisted across user sessions.
Cookies can be accessed in a server component, and you can leverage Server Actions to set or delete cookies.
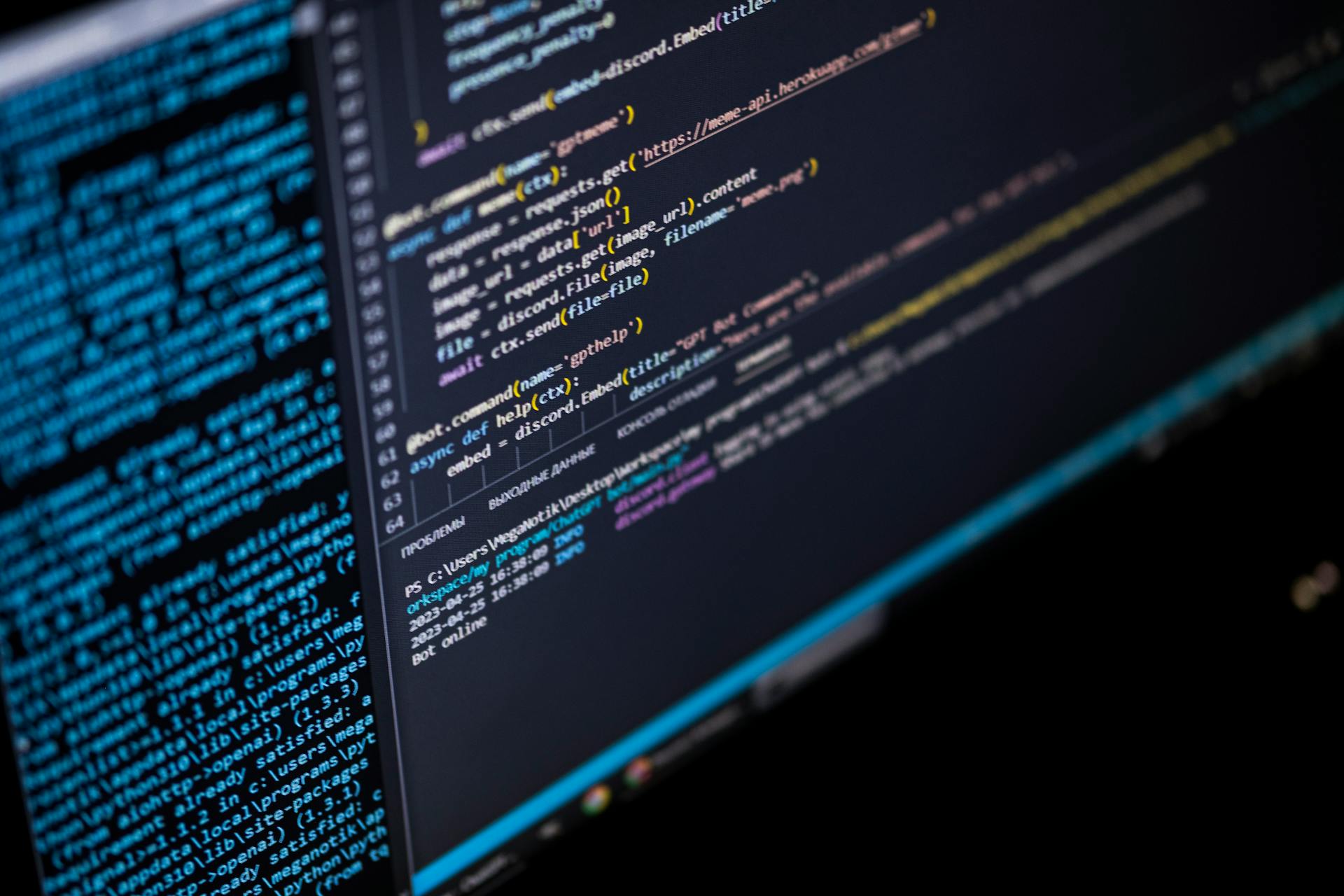
Data rehydration is a crucial concept in server-side rendering (SSR) with Next.js and Redux Toolkit, which involves reconstructing the application state on the client-side by retrieving and deserializing the serialized state received from the server.
Redux Toolkit provides an efficient way to manage state in Next.js applications, allowing you to use the useState hook to manage state in your components.
Server-Client Communication
Server-Client Communication is crucial for maintaining a seamless user experience in Next.js 14. You can achieve this by syncing the state between the server and client-side using a library like next-redux-wrapper.
To sync the state, you need to pre-fetch all queries via initiate actions on the server-side. This is done by using await Promise.all(dispatch(api.util.getRunningQueriesThunk())). This ensures that the user's session is preserved when navigating between pages or reloading the application.
By rehydrating the application state, you can avoid unnecessary data fetching, which improves performance and reduces latency. To configure rehydration, use the extractRehydrationInfo option in your createApi call on the client-side.
Here are the reasons why data rehydration matters:
Server-Client Communication
Server-Client Communication is a crucial aspect of building scalable and efficient applications. To maintain state between the server and client, you need to sync the state between the Next.js server and client-side when using Redux Toolkit.
One way to achieve this is by using a library like next-redux-wrapper, which provides a way to rehydrate the Redux store on the client-side. This library helps ensure that the user's session is preserved when navigating between pages or reloading the application.
Here are the reasons why data rehydration matters:
To implement data rehydration, you can use the initiate actions and wait for each query to finish on the server-side. On the client-side, configure rehydration using the extractRehydrationInfo option in your createApi call.
Moving to URL
Moving to URL is a strategy that involves manipulating the URL to reflect and manage the application's state. This approach provides a global state when using Server Side Rendering.
One reason to move state to the URL is to enable static pre-rendering. This is particularly useful for multi-region or multi-language stores that want to rank well in all regions.
Moving state to the URL also makes the country code globally available. This is a key benefit, especially when working with Server Components.
A good example of this is the checkout flow, where the state is relocated to a URL search parameter to keep track of the completed checkout steps. This allows the checkout UI to be rendered accordingly.
Here are some reasons to consider moving state to the URL:
- Enables static pre-rendering.
- Makes the country code globally available.
- Allows search engines to index localized pages, providing a massive SEO benefit.
Debugging
Debugging is a critical part of building a robust and maintainable Next.js application.
Debugging Redux state is a crucial part of building a robust and maintainable Next.js application. Redux DevTools is a powerful tool that provides a comprehensive overview of your application's state, allowing you to monitor and debug your Redux store effectively.
Having a clear view of your application's state can save you hours of troubleshooting time. With Redux DevTools, you can inspect the state of your application at any given time, making it easier to identify and fix issues.
Redux DevTools is a game-changer for debugging Redux state. It's a must-have tool for any Next.js developer working with Redux.
Environment Setup
To set up the environment for state management in Next.js 14, start by navigating into the project directory.
Create a new folder for your Redux-related code, such as "redux" or "store", to keep your files organized. This will help you keep your project tidy and make it easier to find the code you need.
In the next step, you'll configure the Redux store for your Next.js 14 project. This is a crucial step, as it will allow you to manage your application's state effectively.
Frequently Asked Questions
How to manage global state in NextJS 14?
To manage global state in NextJS 14, consider using state management libraries like Redux with Redux Toolkit, Zustand, or Jotai, which provide a more structured approach than global variables. These libraries help you keep your state organized and maintainable, ensuring a smooth user experience.
Sources
- https://blog.logrocket.com/guide-state-management-next-js/
- https://www.smashingmagazine.com/2021/08/state-management-nextjs/
- https://medusajs.com/blog/client-server-transition-learnings-nextjs-14-server-components/
- https://www.thirdrocktechkno.com/blog/the-way-of-managing-the-state-for-next-js
- https://nextjsstarter.com/blog/redux-toolkit-state-management-in-nextjs-14/
Featured Images: pexels.com