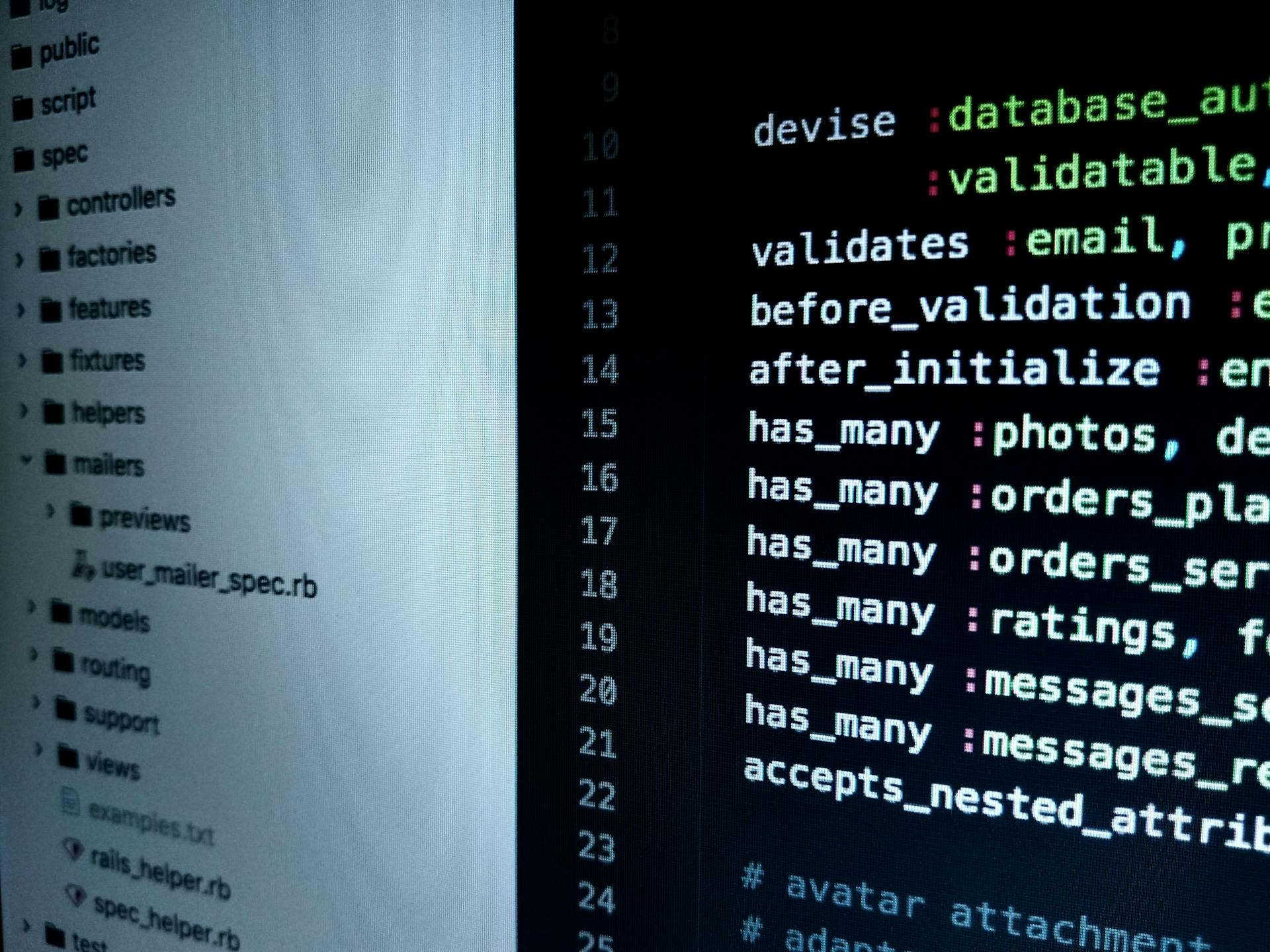
Implementing Redux with Next.js can seem daunting, but don't worry, it's actually quite straightforward. With Next.js, you can easily integrate Redux into your application by using the built-in support for Redux.
First, let's start with setting up Redux in your Next.js project. This involves installing the necessary packages, including `@reduxjs/toolkit` and `react-redux`. In the article, we covered how to install these packages and set up the Redux store.
To get started with Redux in Next.js, you'll need to create a store instance using the `configureStore` function from `@reduxjs/toolkit`. This function takes in the reducer and any other options you might need. For example, you can pass in an `initialState` object to set the initial state of your store.
Getting Started
To get started with Redux in Next.js, you'll need to install Redux and Redux Toolkit. This will enable you to build a simple application that tracks user login status using Redux Toolkit and Firebase as the backend.
You'll also need to add Redux Toolkit to your project using the `--save` option, which adds the package dependency to your `package.json` file. This is a crucial step in setting up Redux in your Next.js application.
Next, you'll need to install `next-redux-wrapper` since you're working with Redux and the Next.js framework. This library helps you wrap your application with the Redux store.
Here's a brief summary of the packages you'll need to install:
- Redux: for state management
- Redux Toolkit: for simplifying Redux setup and usage
- next-redux-wrapper: for integrating Redux with Next.js
By following these steps, you'll be well on your way to setting up Redux in your Next.js application.
Setup
To set up Redux in a Next.js application, start by creating a file called store.ts in the /redux folder. This file will configure the store.
Export the store so it can be used in the Redux provider throughout the application.
You'll also want to export RootState, which will allow you to inject the Redux state variables into your components easily.
Export AppDispatch to use actions defined above directly into your components using props.
Lastly, export AppThunk, which represents a thunk action that can be dispatched in your application. Thunk actions are asynchronous actions that can contain side effects, often used with the Redux-thunk middleware.
Defining Redux Components
To create a Redux Provider, you'll need to create a file called ReduxProvider.tsx and write the code that uses the Provider component from react-redux to pass the Redux store as a prop.
The Provider component is a crucial part of integrating Redux with React applications, and it's especially useful in Next.js projects where maintainability and reusability are key.
To incorporate Redux into a component, you'll need to create the component itself, such as a login page, and import the necessary functions, including the Provider component.
In the src/app/login/ directory, create a file called page.tsx and import the functions you need to successfully incorporate Redux.
To test Redux functionality in a component, you'll need to create a folder named components and a file named Auth.js, where you can add code to handle user authentication using the useDispatch hook.
The useSelector hook is also essential for extracting data from the Redux store, such as isLoggedIn and displayName properties from the auth slice.
Finally, don't forget to import your Auth.js file into the page.tsx file, which is the main component in your application.
You might enjoy: Next Js Free Code Camp
Utilizing Redux in Next.js
To incorporate Redux into your Next.js application, you'll want to create a component that enables users to input their username and password for logging in. In the src/app/login/ directory, create a file called page.tsx and start by importing the functions you need.
In a typical Next.js application with Redux, it's crucial to organize the Redux-related files in a way that complements the file-system-based routing mechanism of Next.js. A common structure involves a store directory at the project's root, with subdirectories for slices, actions, and reducers.
To create a custom provider in Redux, create a file named ReduxProvider.js in your Redux folder and add the following code. This custom provider wraps your entire application to make the Redux store accessible to all components.
In a Next.js application with Redux, it's essential to decide thoughtfully what state belongs to Redux and what belongs to component state. This helps prevent unnecessary complications and data syncing issues.
To set up a Redux store for server-side rendering in Next.js, you'll need to create a function that configures the store anew for each incoming server request. This ensures per-request store creation and appropriate hydration on the client side.
To handle data rehydration in Redux, you'll need to incorporate a base reducer that manages the HYDRATE action by merging the server's state with the existing client state. This involves determining the specifics of state merging on a per-slice or per-reducer basis.
In a Next.js application with Redux, it's crucial to consider caching strategies, especially in server-rendered applications. Incorrectly invalidating the cache or over-reliance on client-side fetching can lead to performance issues.
To optimize the app's performance, you should synthesize the store initial state and preloaded state wisely. This involves only including necessary initial data and using server-side calculated defaults to avoid over-fetching data and decreasing memory overhead.
Consider reading: Apolloclient Nextjs
Managing State and Data
To get data from Redux stores, you'll use the `useSelector` hook and the `getItemsInCart` method. This method fetches the store value for `itemsInCart`.
You can update the store value of `itemsInCart` by using the `useDispatch` hook and the `setItemsInCart` method. This method increments the value by one at the click of a button.
Getting and Updating Values
To get the store values, you need to export another method in the cartSlice file, just like you did with setItemsInCart and setTotalAmount. This method will get the store values, such as getItemsInCart and getTotalAmount.
To get data from Redux stores, you'll use useSelector and getItemsInCart. Import those first, and then use them to fetch the store value for itemsInCart.
To update the store value of itemsInCart, you'll need to import useDispatch and setItemsInCart. This will allow you to increment the value by one when a button is clicked.
Initially, the application's UI will show the default or initial state value. At the click of a button, the value will increment by one, thanks to the power of Redux Toolkit.
Curious to learn more? Check out: Why Use Next Js
Data Available on Pages
Data is readily available on pages, making global state management with Redux unnecessary if you're using Next.js's data fetching mechanisms in Server Components.
You can easily access the data your components need through these mechanisms, which can be a more efficient approach than managing it globally.
Sometimes, the data you need is already available on the page, eliminating the need for Redux altogether.
This can save you time and effort, especially if you're working with large amounts of data.
Best Practices and Optimization
To avoid performance issues as your Next.js application grows, managing state slices is crucial. Breaking down slices into smaller segments and using selectors to extract information can greatly improve responsiveness and maintainability.
RTK's integration with Immer guarantees state immutability, while batched actions from React-Redux can minimize re-renders. This is particularly important when dealing with complex state transitions.
Use RTK Query's automatic cache management to minimize re-rendering and enhance performance. This innovation negates the need for managing loading states explicitly.
You might like: Nextjs Performance
Antipatterns and Best Practices in Next.js
Antipatterns in Next.js Redux applications often stem from a misunderstanding of Redux's principles or a misalignment with Next.js's architecture. One such pitfall is overfetching data from the server or the redux store, which can lead to bloated redux state trees and unnecessary network requests.
This happens when developers fetch more information than needed, just because it's available. I've seen this happen in projects where the team was trying to be too comprehensive with their data fetching, without considering the performance implications.
State duplication is another antipattern that can lead to unnecessary complications and data syncing issues. It occurs when developers mirror backend data structures inside the Redux store without considering how the data will be used within the application.
Using Redux middleware incorrectly can also lead to problems. Developers might misuse middleware like redux-thunk or redux-saga for simple actions that could be handled within the component state.
In server-rendered Next.js applications, it's essential to consider caching strategies to avoid performance issues. Incorrectly invalidating the cache or over-reliance on client-side fetching when data could be refreshed on the server can lead to a poor user experience.
By being mindful of these antipatterns, you can create a more streamlined and efficient application architecture.
Scaling Next.js: Middleware and Optimization
To scale Next.js, middleware is a crucial component that helps streamline state management. Redux Toolkit (RTK) is a powerful middleware that simplifies store setup, actions, reducers, and sagas/thunks, reducing boilerplate code.
Middleware like RTK and RTK Query can handle asynchronous events or side-effectful actions, making complex state transitions more manageable. RTK Query, in particular, optimizes data fetching and caching operations, minimizing re-rendering and enhancing performance.
The Redux DevTools extension is a must-have for debugging, offering a real-time overview of state changes and actions. To maximize its benefits, ensure that the devTools flag is conditionally enabled based on the environment.
As applications grow, managing state slices becomes increasingly important. Breaking down slices into smaller segments and using selectors to extract information can greatly improve responsiveness and maintainability. RTK's integration with Immer guarantees state immutability, while batched actions from React-Redux can minimize re-renders.
Using middleware incorrectly can lead to problems, such as misusing middleware like redux-thunk or redux-saga for simple actions that could be handled within the component state.
Additional reading: Query in Nextjs
Advanced Concepts
Redux with Next.js allows for a scalable and maintainable state management system.
By using the `useSelector` hook, you can easily access and manipulate state from your components, as shown in the "Setting Up Redux" section.
Redux's connect function can be used to connect your components to the Redux store, making it easier to manage global state.
The example in the "Connecting Components to the Redux Store" section demonstrates how to use connect to connect a component to the store.
Using the `useDispatch` hook, you can dispatch actions to update the state, as seen in the "Dispatching Actions" section.
Redux's middleware feature allows you to add functionality to the Redux store, as shown in the "Using Middleware" section.
Next.js's built-in support for Redux makes it easy to integrate Redux into your Next.js project, as seen in the "Setting Up Redux" section.
Frequently Asked Questions
Can you use React and Next.js together?
Yes, you can use React and Next.js together to build dynamic web applications. This powerful combination enables fast and interactive web development.
What is the difference between Next.js context and Redux?
Next.js Context and Redux differ in their approach to state management, with Context handling state changes at the component level and Redux managing state in a centralized store
Does Next.js use Redux?
Next.js can use Redux, but it's not a requirement. Redux is a suitable option for large-scale or complex Next.js projects, especially when server-side rendering is involved.
Is Next.js replacing React?
No, Next.js is not replacing React, but rather enhancing its capabilities for certain types of projects with features like server-side rendering and routing. Next.js and React can be used together to create powerful and efficient web applications.
What is Redux used for?
Redux is a state management tool that helps you manage global state across your application, making it easier to understand and predict how your application will behave. It simplifies complex state updates, making your code more maintainable and efficient.
Sources
- https://medium.com/@himanshu.sharma.for.work/setting-up-redux-in-next-js-with-typescript-65eda6decdbf
- https://jscrambler.com/blog/working-with-redux-in-next-js
- https://www.telerik.com/blogs/how-manage-state-nextjs-redux-toolkit
- https://borstch.com/blog/development/setting-up-redux-with-nextjs
- https://victoriacheng15.vercel.app/posts/nextjs-state-management-with-redux-toolkit-part-1/
Featured Images: pexels.com