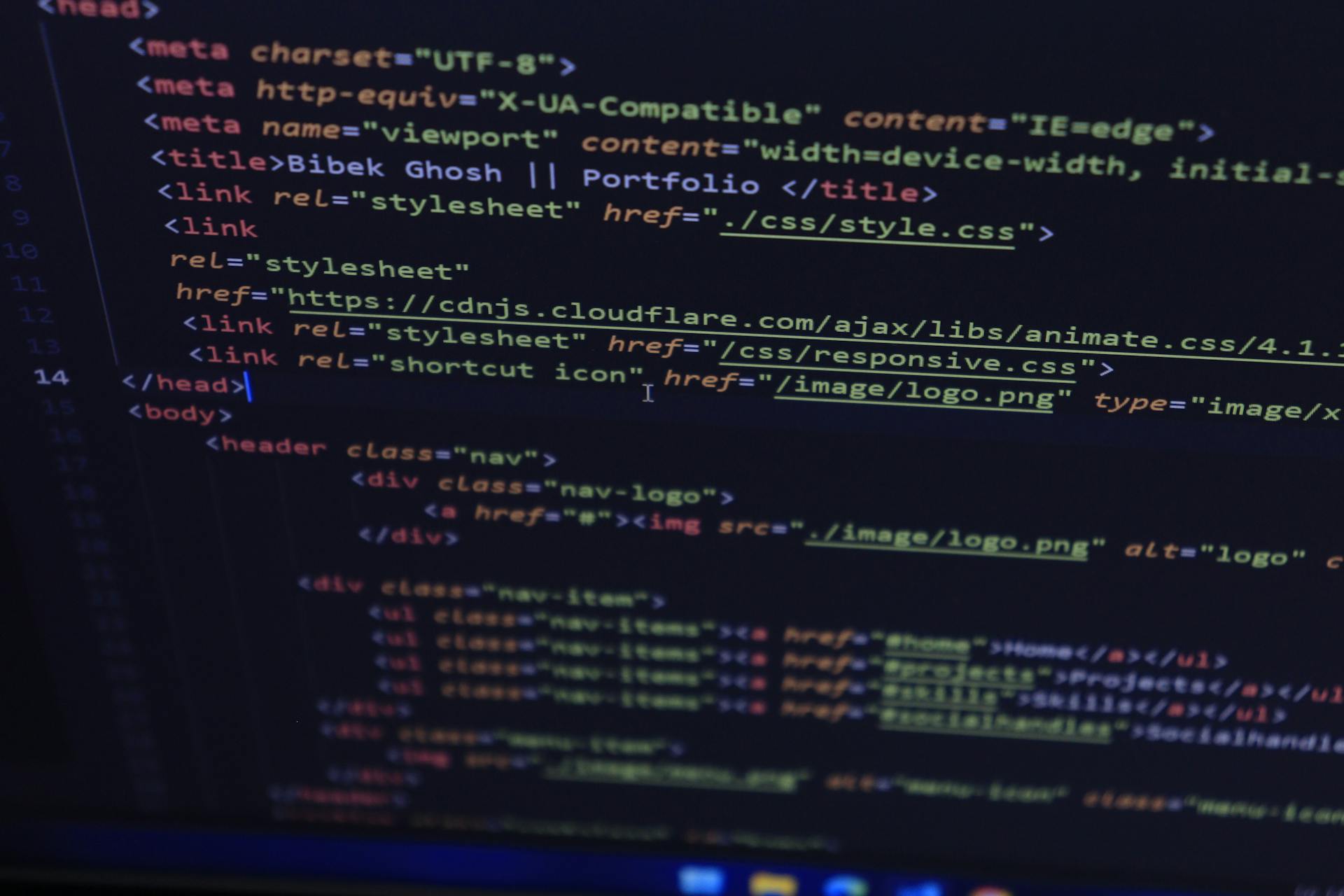
Query in Next.js allows you to fetch data from an API or database and render it on your pages.
Next.js provides a built-in API route feature, which enables you to create API routes using the same file system structure as your pages.
API routes are a great way to handle data fetching and server-side rendering in Next.js.
You can create API routes by adding a new file in the pages/api directory.
API routes support both GET and POST requests, allowing you to fetch data or send data to the server.
Next.js also provides a fetch API that you can use to fetch data from an API or database.
The fetch API is a built-in function in Next.js that allows you to make HTTP requests to an API or database.
You can use the fetch API to fetch data from an API or database and render it on your pages.
Next.js provides a powerful feature called getServerSideProps, which allows you to fetch data on the server and render it on the client.
getServerSideProps is a function that returns an object with a props property, which contains the fetched data.
You can use getServerSideProps to fetch data from an API or database and render it on your pages.
API routes and getServerSideProps are two powerful features in Next.js that allow you to handle data fetching and server-side rendering.
Querying Data
React Query simplifies fetching data on the server side in Next.js applications. This synergy allows developers to pre-fetch data on the server and seamlessly pass it as props to React components.
Using getStaticProps, data can be fetched at build time, enabling content to load instantly upon request. This method is incredibly useful for static sites requiring periodic updates from a server.
React Query enhances data fetching by managing server-state data caching, updating, and synchronizing, leading to improved performance and user experience.
Fetching Data with React
React Query is a powerful library that simplifies data fetching, caching, and synchronization in React applications, including those built with Next.js.
By using React Query, developers can handle asynchronous data fetching and updates through a straightforward API, focusing more on building features than on the intricacies of data management.
React Query automatically caches and updates fetched data based on unique keys for each query, significantly enhancing application performance by reducing the need for repetitive server requests.
Next.js is a highly sought-after framework for React developers aiming for optimized performance and SEO-friendly web applications, and it shines in its ability to facilitate server-side rendering (SSR) and static site generation (SSG).
By pre-rendering pages on the server, Next.js ensures that contents are immediately available to search engines and improves the load time for end-users.
To fetch data on the server with Next.js and React Query, developers can use getServerSideProps and getStaticProps, which allow them to pre-fetch data on the server and seamlessly pass it as props to React components.
React Query enhances this process by managing server-state data caching, updating, and synchronizing, leading to improved performance and user experience.
By caching server-side data fetched during rendering, React Query minimizes the need to refetch data on the client-side, further enhancing the application's efficiency.
To set up React Query in a Next.js project, developers need to install the React Query library and create a React Query Client, which serves as the backbone for managing queries and the cache of the application.
The QueryClient can be created by instantiating a new QueryClient object, and it's essential to wrap the application's root component with the QueryClientProvider to make the QueryClient accessible in any component of the Next.js application.
By following these steps, developers can smoothly integrate React Query into their Next.js projects, elevating their application's data management and fetching capabilities to new heights.
React Query's useQuery hook allows developers to optimize data fetching strategies according to the specific needs of each page within an application.
This hook can be used in conjunction with getStaticProps and getServerSideProps to fetch data at build time or request time, further optimizing the user experience.
Implementing React Query in conjunction with Next.js's data fetching methods requires careful consideration of data staleness and cache invalidation.
React Query's tools for query invalidation and refetching become particularly handy in such scenarios, allowing developers to refetch data on the client side when deemed stale.
The clear distinction between static generation and server-side rendering, coupled with effective state management and caching, lays the foundation for high-performance applications that handle server-state data gracefully.
Manipulating
Manipulating data is a crucial step in querying data, and it's essential to understand the different types of manipulation.
Filtering data is a common form of manipulation, where you can narrow down your results by specifying conditions. For example, you can filter data to show only rows where a specific column meets a certain criteria.
Sorting data is another manipulation technique, where you can arrange your data in ascending or descending order based on one or more columns. This is useful when you need to analyze data in a specific sequence.
Limiting data is a form of manipulation that allows you to restrict the number of rows returned in your query results. This is useful when working with large datasets and you only need a subset of the data.
Caching and Performance
When your app is deployed, Prismic requests will be cached and tagged with "prismic", allowing for clearing Prismic queries from the fetch() caching using On-demand Revalidation.
This approach enables better performance by reducing the number of requests made to Prismic.
You can configure the Prismic client's cache and next parameters using the fetchOptions parameter.
The recommended fetchOptions value for the best performance is not specified in the text, but it's mentioned that you can override the cache and next parameters on individual queries as needed.
While developing your app locally, Prismic requests will be cached for 5 seconds.
This caching behavior can be useful for rapid development and testing, but it's essential to consider the performance implications when switching to production.
Navigating
Navigating with query parameters in Next.js is a breeze, thanks to the useRouter hook and Next.js router methods. You can programmatically navigate between pages and manipulate query parameters using router.push and router.replace.
These methods allow you to update the URL and navigate to a new page without a full page refresh, providing a smoother user experience. You can use them to update the current URL with new query parameters, like in the example where the handleSearch function uses router.push to update the search term.
To preserve query parameters during navigation, you have several strategies at your disposal. You can pass query parameters manually when using router.push or router.replace, store them in local storage or session storage, or use a global state management library like Redux or Zustand.
Here are some ways to preserve query parameters during navigation:
Server-Side Rendering and Static Generation
Server-Side Rendering and Static Generation are two powerful features in Next.js that allow you to fetch data based on query parameters.
You can use the getServerSideProps function to fetch data on the server for each request, and it receives the context object, which includes the query object.
To access query parameters in getServerSideProps, you can extract the query object from the context and log the query parameters accordingly.
For static generation, Next.js provides getStaticProps and getStaticPaths, which are used to fetch data at build time and specify dynamic routes that should be pre-rendered.
You can define paths that include query parameters in getStaticPaths, and then access these parameters through the params object provided by the context in getStaticProps.
Prisma can be used inside getStaticProps to send queries to your database for static site generation.
Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
You can query your database with Prisma in getStaticProps to generate a static page with dynamic data, giving you full rendering flexibility.
Display your data using client-side rendering, server-side rendering, and static site generation to suit your needs.
React and Next.js Integration
React Query simplifies data fetching, caching, and synchronization without boilerplate code, making it a powerful library for React applications, including those built with Next.js.
By integrating React Query with Next.js, developers can leverage the strengths of both libraries, providing a seamless architecture for fetching, caching, and serving data in React applications.
This combination enhances the scalability and maintainability of applications, streamlines the development process, and abstracts common data fetching and state management patterns.
With React Query, developers can implement advanced data synchronization techniques, such as background data fetching and automatic refetching on window focus, which are crucial for maintaining the freshness of server-side data in client-side applications.
By following the steps outlined in the documentation, developers can smoothly integrate React Query into their Next.js projects, elevating their application's data management and fetching capabilities to new heights.
The integration of React Query with Next.js promotes a pattern that optimizes data fetching efficiency without sacrificing the rich user experience of dynamic web applications.
Prisma and Next Integration
Prisma is the perfect companion for Next.js if you need to work with a database in your app. It blurs the lines between client and server, supporting pre-rendering pages at build time or request time.
You can access your database with Prisma at build time using getStaticProps, at request time using getServerSideProps, or by separating the backend out into a standalone server. Prisma Accelerate can speed up your database queries in Serverless or Edge environments, ensuring a scalable connection pool and caching results at the Edge.
Next.js will pass the props to your React components if you use Prisma inside of getStaticProps, enabling static rendering of your page with dynamic data. This is especially useful for static pages like blogs and marketing sites.
Depending on your needs, you can query your database with Prisma in Next.js API routes, in getServerSideProps, or in getStaticProps for full rendering flexibility and top performance.
Setting Up React
To set up React Query in a Next.js project, start by installing the React Query library using npm or yarn. Run npm install react-query or yarn add react-query in the terminal to get started.
The first step is crucial, as it equips your Next.js application with the necessary React Query package. This package is the backbone of your data management and fetching operations.
Next, create a React Query Client by instantiating a new QueryClient. This is typically done inside the custom _app.tsx file to ensure global availability.
A new QueryClient object is created, ready to be used throughout the application. This object is the foundation for managing queries and the cache of your application.
To make the QueryClient accessible in any component of your Next.js application, wrap your application's root component with the QueryClientProvider. This is done by passing the created QueryClient as a prop.
Embed the component hierarchies within the QueryClientProvider in your custom _app.tsx file. This ensures that React Query’s features are ubiquitously available.
Installing the ReactQueryDevtools is a recommendable next step during the development phase. This provides an insightful interface for tracking, managing, and debugging your queries efficiently.
Ensure each instance of QueryClient is unique to each server-side render by initializing the QueryClient inside a useState hook or using a useRef hook. This practice helps maintain isolation of data between different users and requests.
Frequently Asked Questions
How to get query params in Next.js 12?
To get query parameters in Next.js 12, use the `useRouter` hook from `next/router` and access the `query` object within your functional component. This allows for easy extraction of URL query parameters.
Sources
- https://brockherion.dev/blog/posts/setting-up-and-using-react-query-in-nextjs/
- https://prismic.io/docs/fetch-data-nextjs
- https://www.dhiwise.com/post/navigating-nextjs-query-params-a-comprehensive-guide
- https://borstch.com/blog/development/integrating-react-query-library-with-nextjs-for-server-side-rendering-and-data-fetching
- https://www.prisma.io/nextjs
Featured Images: pexels.com