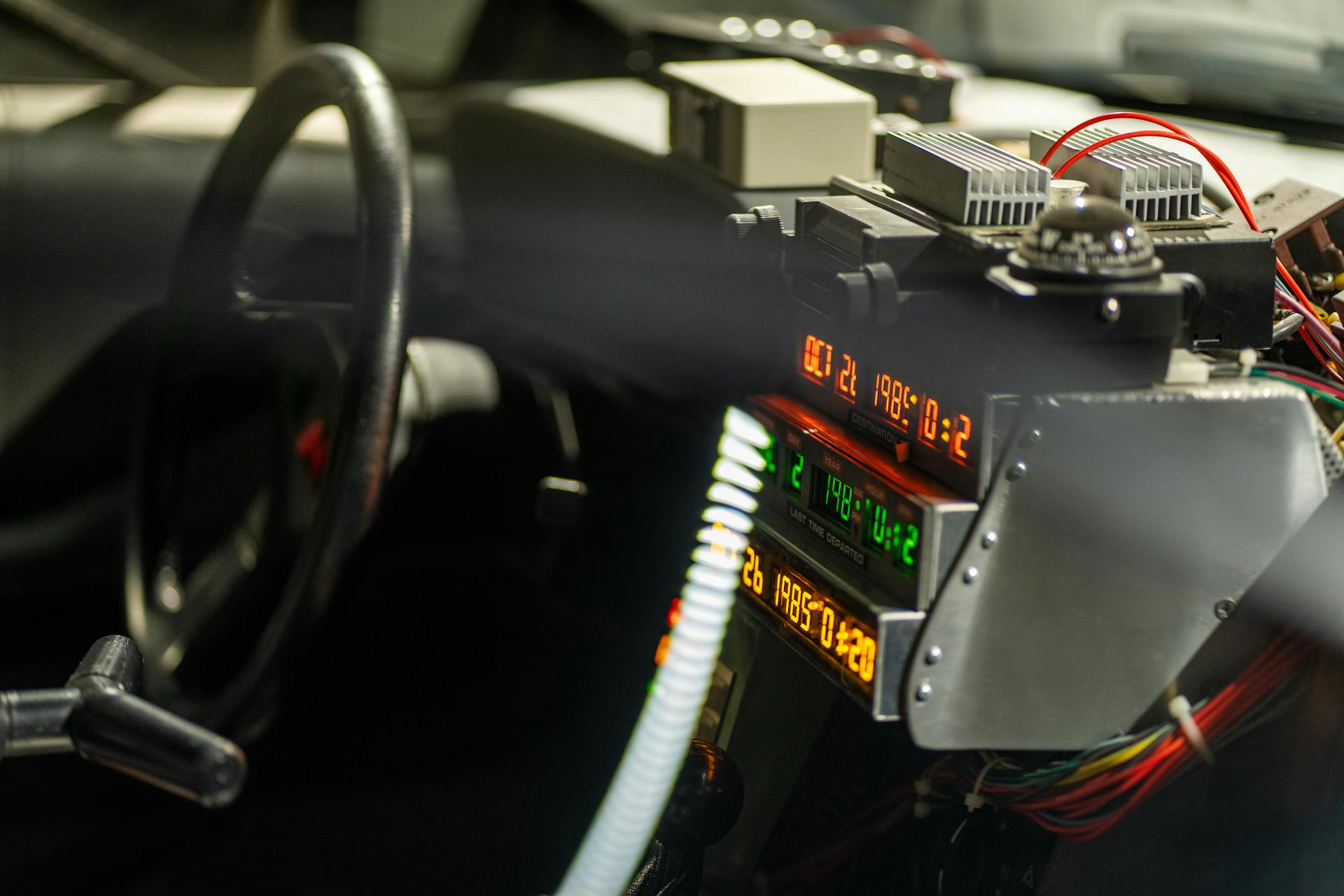
Let's dive into setting up a Next.js Prisma Docker project. To start, you'll need to create a new project folder and navigate to it in your terminal.
Next, initialize a new Next.js project using the command `npx create-next-app my-app`. This will create a basic Next.js project structure.
To set up Prisma, create a new file called `schema.prisma` in the root of your project. In this file, define your database schema using Prisma's schema language.
Now, you're ready to run your project with Docker.
Take a look at this: Next Js Cookies
Development Setup
Efficiency in development is a major advantage of using Docker Compose, which eliminates tedious configuration processes and allows developers to encapsulate all dependencies into a single, reproducible environment.
With Docker Compose orchestrating the containers, developers can focus on writing code without worrying about environment-specific issues or manually configuring databases.
To set up your local development environment, run the following command to create a new Next.js project.
Prisma is a powerful tool for database management, providing an ORM (Object Relational Mapper) for efficient interaction with your database.
Related reading: Mern Stack Development Company
To integrate Prisma with your Next.js project, install Prisma and the Prisma Client using npm or yarn.
Here are the steps to initialize Prisma:
- Run the command `npx prisma init` to create a new Prisma directory and a `schema.prisma` file.
- Specify the `DATABASE_URL` in your env file to connect Prisma to your database.
In your env file, you'll need to replace the placeholders with your actual database credentials.
With Prisma set up, you can configure it to use your database as its datasource db in the `schema.prisma` file.
Broaden your view: Nextjs Server Actions File Upload
Prisma
Prisma is a powerful tool that simplifies database management by providing an ORM (Object Relational Mapper) for efficient interaction with your database. It allows developers to work with objects in their programming language rather than dealing directly with database tables and SQL queries.
Prisma provides a higher-level, object-oriented abstraction over the relational database, which simplifies code and makes it more intuitive for developers. This abstraction can lead to faster development cycles since developers can focus more on business logic and less on low-level database operations.
Prisma can significantly reduce the amount of boilerplate code needed for database interactions, reducing the risk of SQL injection attacks by using parameterized statements. It can also automate the creation and modification of database schemas based on changes to the application's data model.
If this caught your attention, see: Nextjs Neon Sql
To install Prisma, you need to add it as a dependency to your project and initialize it with the `npx prisma init` command. This command creates a new Prisma directory with a prisma schema file and a new .env file for database configuration.
Here are the benefits of using Prisma:
- Higher-level, object-oriented abstraction over the relational database
- Significant reduction in boilerplate code for database interactions
- Automated creation and modification of database schemas
- Reduced risk of SQL injection attacks
Prisma supports databases like PostgreSQL, MySQL, SQLite, and others, and you can specify the DATABASE_URL for connecting Prisma to your database in the .env file.
Recommended read: Prisma Nextjs
API and Data
To set up API endpoints in Next.js, create a new folder called api inside the app directory. This will be the path to your endpoint, for example, api/users. Inside the users folder, create a route.ts file where you configure the different route handlers.
To handle different requests in this endpoint like POST and GET, you need to export a function with the name of the individual http verb. For example, for adding data, you add code to your route.ts file to extract the request body and make a short validation because the email is required.
You might like: Nextjs Folder Structure
You can use a tool like Postman to test your API endpoints. For example, when trying to add data via Postman, you should get a response like this: {"id": 1, "name": "John", "email": "[email protected]"}.
Here are the HTTP verbs you can use to interact with your API endpoints:
- GET: Retrieve data
- POST: Create new data
- PUT: Update existing data
- DELETE: Delete data
Make sure to install Prisma and the Prisma Client to interact with your database from within your app. Initialize Prisma using the npx prisma init command to create a new Prisma directory and a prisma schema file (schema.prisma).
API Setup
To set up an API, you need to create a new folder called api inside the app directory. This is where your API endpoints will live.
For this tutorial, we'll be using the app router, so you'll need to create a new folder called users inside the api directory. This will be the path to our endpoint api/users.
Inside the users folder, you'll need to create a route.ts file where you configure the different route handlers.
A different take: Next Js App
Adding Data
Adding data to our database is a straightforward process. We can handle different requests like POST and GET by exporting a function with the name of the individual http verb.
To add data, we extract the request body from our request. We then make a short validation because the email is required.
The request body is then added to create a new row inside our User table using the Prisma client created earlier in the lib folder. The User model imported from the lib folder is also used for this purpose.
This approach is powerful because it ensures we have one single source of truth for our User model whenever we make changes to it.
Readers also liked: What Is .next Folder in Next Js
Local Development
Setting up a local development environment with Next.js, Prisma, and Docker is a game-changer for developers. You can encapsulate all dependencies, including the Next.js application and Postgres database, into a single reproducible environment, eliminating tedious configuration processes.
Recommended read: Nextjs Build Fails Environment Variables
This setup allows you to create a consistent environment across development, testing, and deployment, reducing the risk of unexpected issues cropping up in production. With Docker Compose orchestrating the containers, you can have confidence that your application behaves predictably in different environments.
To start all the services, simply use the command: `docker-compose up -d`. This command builds and starts each container according to the configuration in the `docker-compose.yml` file. You should see logs from both the database and the Next.js application in the terminal.
A unique perspective: Next Js Spa
Efficiency in Development
Local development is a breeze with the right setup. With Docker Compose orchestrating the containers, tedious configuration processes are a thing of the past.
Developers can encapsulate all dependencies into a single, reproducible environment, eliminating hours wasted on troubleshooting environment-specific issues. This setup is a game-changer.
You can add a new entry inside the scripts section of your package.json file, which makes it easy to create a reproducible environment. Now you're ready to focus on what matters most – building your application.
With this setup, you can create models of your data and feed your database with some data, all without the hassle of manual configuration. Your development process just got a whole lot more efficient.
Take a look at this: Nextjs Development Company
Local Development Environment
You can create a local development environment with Docker Compose, which eliminates tedious configuration processes and allows developers to encapsulate all dependencies into a single, reproducible environment.
This setup is particularly useful for Next.js applications, as it enables developers to troubleshoot environment-specific issues more efficiently.
To create a local development environment, run the command to create a new Next.js project, and then add a new entry inside the scripts section of your package.json file.
You're now ready to create models of your data and feed your database with some data.
Here's a step-by-step guide to setting up a local development environment:
1. Create an env file in the root directory of your Next.js project.
2. Add the DATABASE_URL for Prisma to connect to your database in the env file.
3. Use environment variables in Prisma by referring to the DATABASE_URL variable in your prisma schema file.
By following these steps, you can ensure consistency across environments and mitigate the risk of unexpected issues cropping up in production.
Here's a list of the benefits of a well-structured local setup:
- Eliminates tedious configuration processes
- Encapsulates all dependencies into a single, reproducible environment
- Enables developers to troubleshoot environment-specific issues more efficiently
- Ensures consistency across environments
- Mitigates the risk of unexpected issues cropping up in production
Project Setup
To set up your project for Next.js, Prisma, and Docker, you'll want to start by creating a local development environment. Run the command to create a new Next.js project.
You'll also need to configure Next.js to work with Docker. This involves adding "output: 'standalone'" to your next.config.js file, which will create a standalone folder with only the necessary files for a production deployment. This helps reduce image size.
To configure Prisma, you'll need to change the provider from sqlite to postgresql in your schema.prisma file, and add binaryTargets to the generator block. This will allow you to use the Prisma CLI inside the Docker container. You can also use a multi-stage Dockerfile to optimize the build and separate dependencies for the development and production environments.
Here are the key components to include in your Docker Compose file:
- db service: runs PostgreSQL as the database with environment variables for database setup and uses a named volume to persist data
- app service: builds the Dockerfile for Next.js with the DATABASE_URL pointing to the db service for database access
- networks: both services are assigned to the app-network to ensure communication
- dependencies: the db service starts before the Next.js application
Consistency Across Environments
Consistency Across Environments is crucial for a seamless development process. Developers can have confidence that the application behaves predictably in different environments, mitigating the risk of unexpected issues cropping up in production.
A well-structured local setup is key to achieving consistency. This means having a consistent environment for development, testing, and eventually deployment. With a consistent setup, developers can avoid the frustration of debugging issues that only appear in certain environments.
To ensure consistency, developers can use tools like Docker to create a standalone folder that copies only the necessary files for a production deployment. This includes select files in node_modules.
Here's a breakdown of the benefits of consistency across environments:
By following best practices for consistency, developers can ensure that their application behaves predictably in different environments, making it easier to debug and deploy.
Ease of Collaboration
Having a consistent development setup is crucial for seamless collaboration among team members. In a collaborative development environment, the ability to share a consistent development setup becomes a game-changer. This allows team members to effortlessly clone the project repository and bring up the entire environment with a single command.
This level of ease is a significant time-saver, as developers no longer need to spend precious time troubleshooting setup issues or dealing with discrepancies between individual machines.
Worth a look: Nextjs Team
Configure to Work
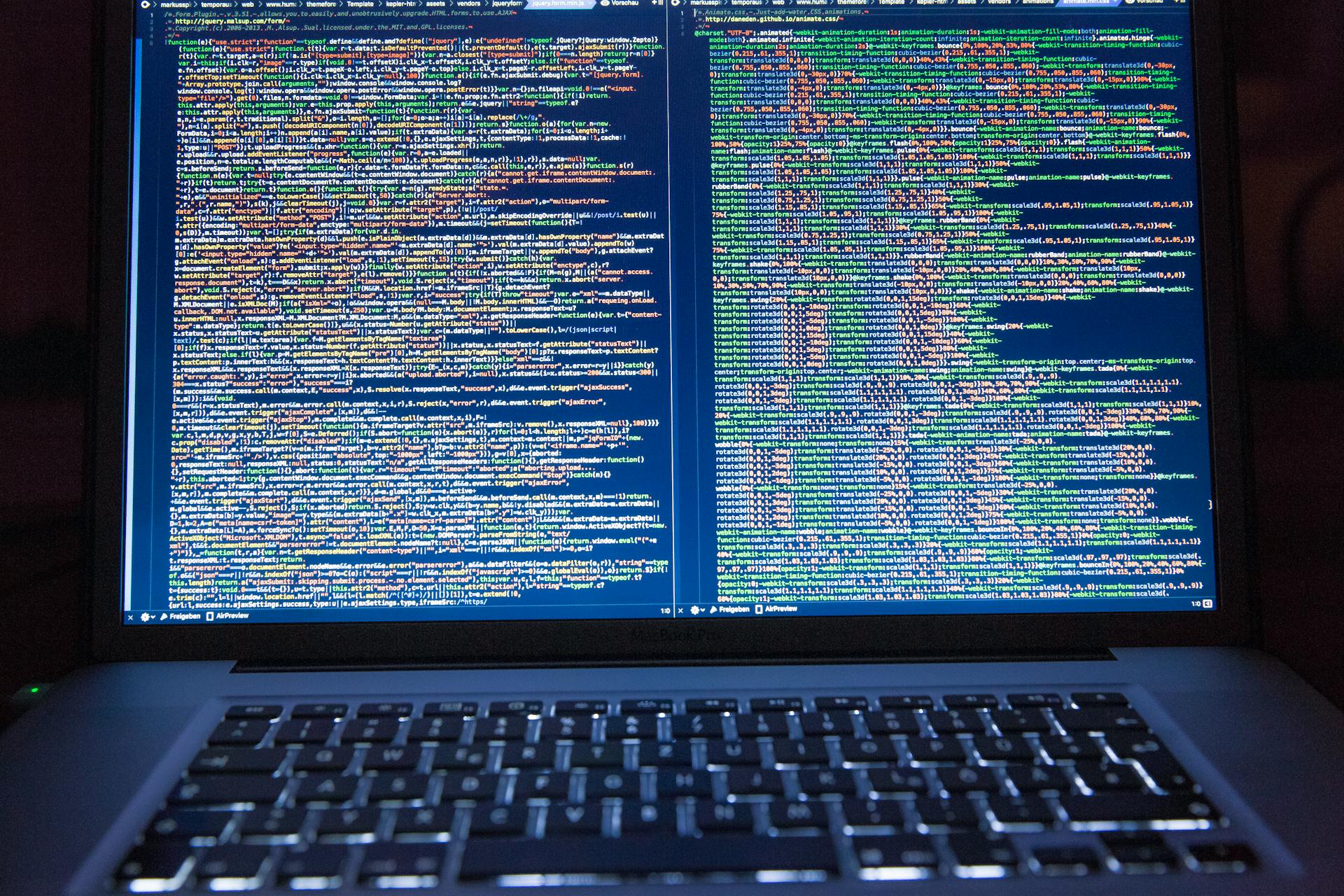
To configure your project to work seamlessly with Prisma, you'll want to install Prisma and the Prisma Client. This is done by running the command `npx prisma init`, which will create a new Prisma directory and a `schema.prisma` file.
In your `env` file, specify the `DATABASE_URL` for connecting Prisma to your database. This connection string should be replaced with your actual database details, such as username, password, and database name.
To ensure consistency across environments, create a well-structured local setup. This will make the transition from development to testing and deployment a seamless process. Developers can have confidence that the application behaves predictably in different environments, mitigating the risk of unexpected issues cropping up in production.
To configure Next.js to work with Docker, add `output: "standalone"` to the `next.config.js` file. This will reduce image size.
Here are the specific steps to configure Prisma to work with Docker:
1. Change the provider from SQLite to PostgreSQL.
For more insights, see: File Upload Next Js Supabase
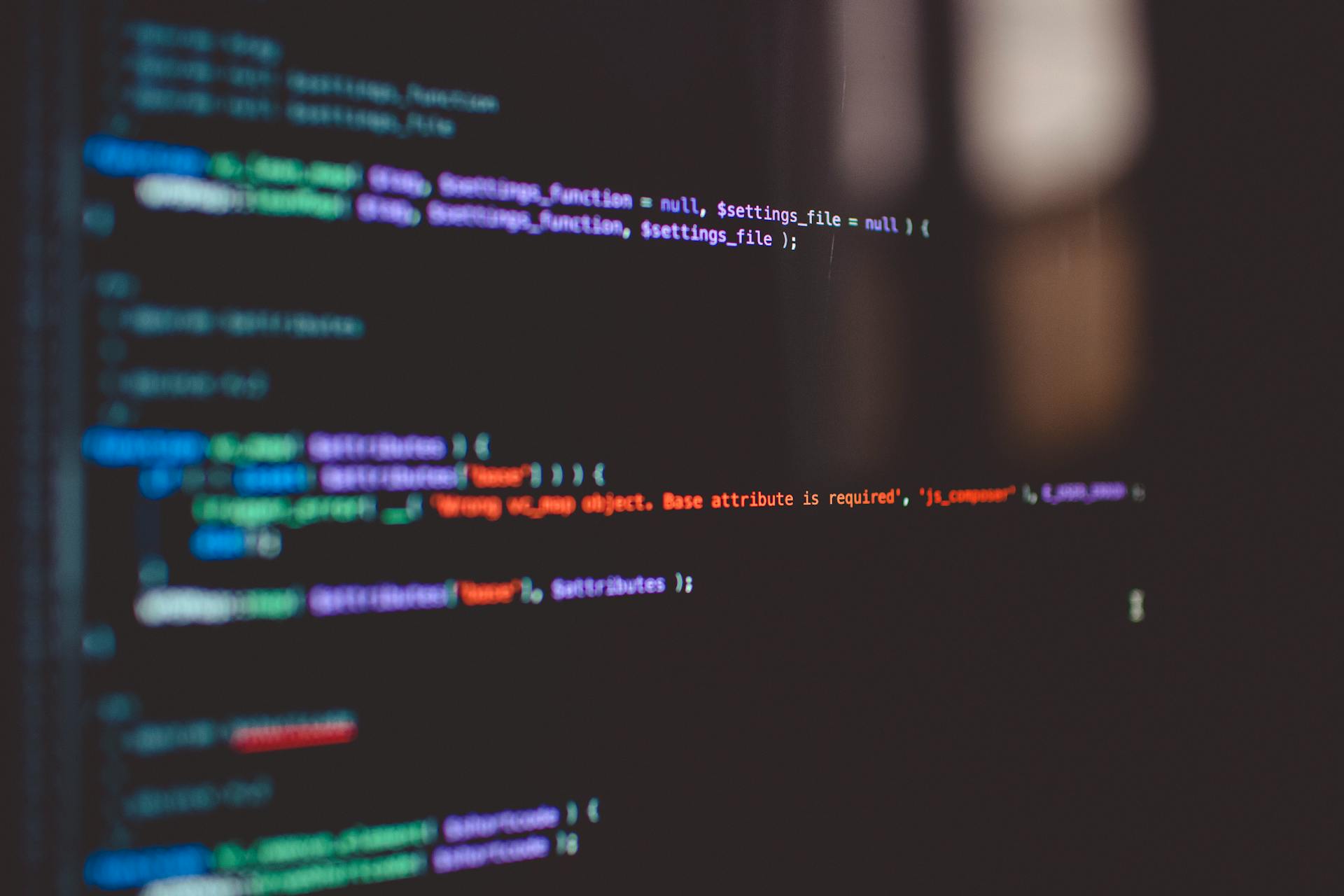
2. Add `binaryTargets` to the generator block, which allows you to use the Prisma CLI inside the Docker container. This requires specifying your OS and architecture, such as "linux-musl-arm64-openssl-3.0.x" for an M1 Mac.
To create a Dockerfile for your Next.js and Prisma application, use a multi-stage Dockerfile to optimize the build and separate dependencies for the development and production environments. This will reduce the image size by splitting the build process into distinct stages.
Here's an example Dockerfile for a Next.js and Prisma application:
- Install dependencies: The first stage (builder) installs all the required dependencies and builds the Next.js application.
- Copy only necessary files: The second stage (production image) copies only essential files (like the .next build folder and node_modules) to keep the final Docker image slim and optimized.
- Expose and run the app: Exposes port 3000 for the app to run and starts the application.
Remember to add environment variables, such as `DATABASE_URL`, to your `env` file and ensure they point to the database service in your Docker Compose setup.
To deploy your Dockerized application, make sure to generate the Prisma Client by running `npx prisma generate` as part of the Dockerfile or manually within the container after running `docker-compose up`. If you make changes to the Prisma schema file, you'll need to rerun migrations using `npx prisma migrate dev`.
For your interest: Running Json Nextjs
Sources
- https://jean-marc.io/blog/setup-next.js-with-postgres-prisma-docker
- https://blog.alexefimenko.com/posts/nextjs-postgres-s3-locally
- https://www.dhiwise.com/post/how-to-build-app-with-nextjs-prisma-and-docker
- https://anuragkanoria11.hashnode.dev/dockerize-nextjs-with-prisma-app
- https://yaraoncode.me/post/building-next-js-app-with-next-auth-and-prisma-on-top-postgres
Featured Images: pexels.com