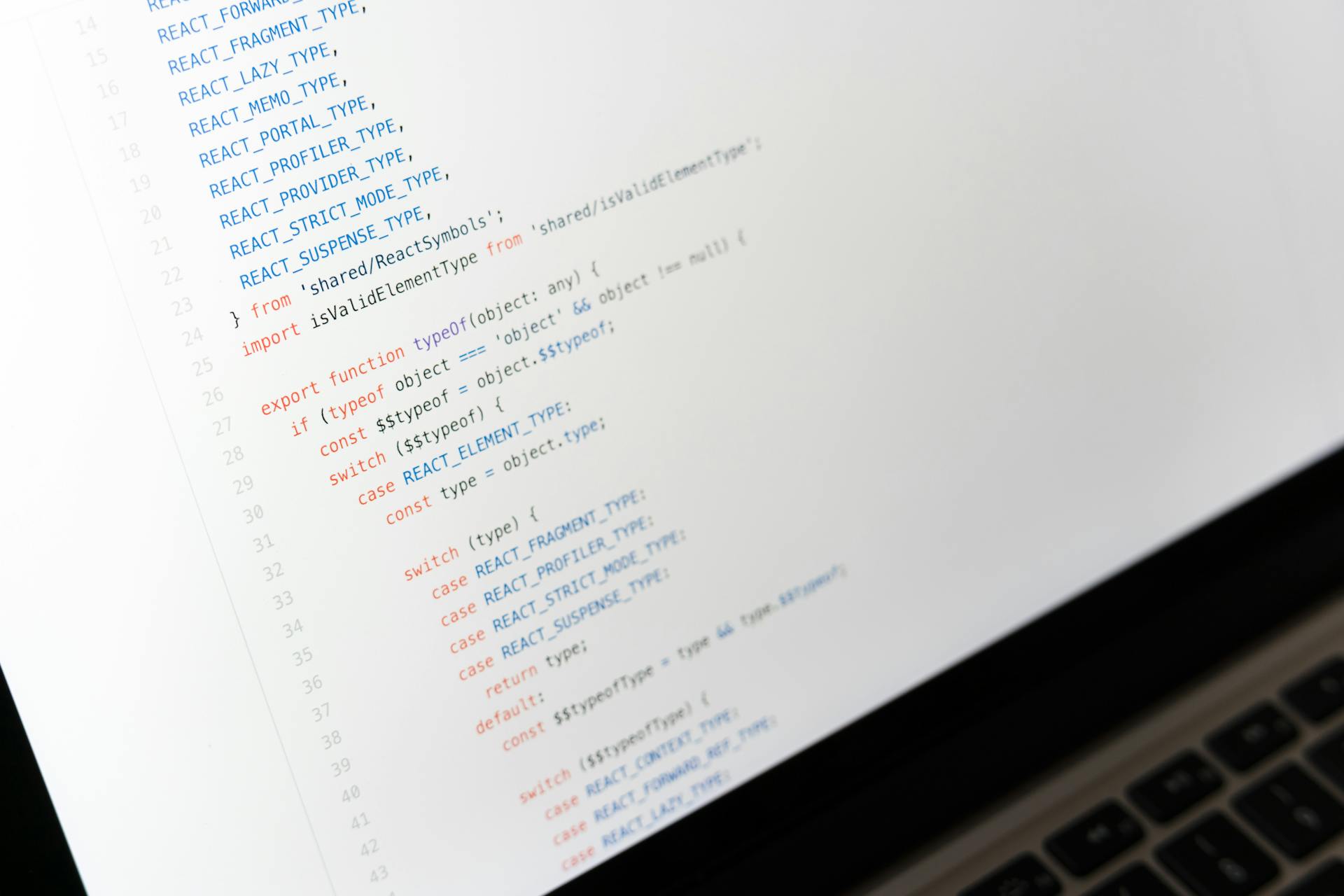
Json is a lightweight data interchange format that is easy to read and write. It's a great choice for building data-driven applications.
First, you'll need to create a new Next.js project using the `npx create-next-app` command. This will set up a basic project structure for you to work with.
Json files are typically used to store data in a human-readable format. They can be easily parsed and generated using libraries like `jsonify`.
Expand your knowledge: Next Js Using State Context
File Structure and Setup
To get started with running JSON Next.js, you'll first need to understand the file structure. The main files and directories to use are in the following list:
- pages/
- frontaid.content.json
- frontaid.model.json
- package.json
The pages directory contains definitions for the web app structure, the index page, and the dynamic pages. You can update the JSON content file, such as changing the project title or adding new dynamic pages, and Next.js will adapt accordingly.
Suggestion: Next Js Pages
File Structure
Let's take a closer look at the file structure for our web app. We have a main directory called pages that contains the definitions for our web app structure and the index page.
Take a look at this: Next Js Project Structure
The pages directory also includes dynamic pages, which are essential for creating a engaging user experience. The frontaid.content.json file is where we'll find the actual content that enriches our pages.
This file is crucial for adding depth and context to our web app. We also have a frontaid.model.json file, which includes the data model used with FrontAid CMS. If you don't plan on using FrontAid, you can safely ignore this file.
Finally, we have a package.json file that lists our dependencies and includes some useful scripts. Here's a rundown of our main files and directories:
- pages/
- frontaid.content.json
- frontaid.model.json
- package.json
Next.js API Routes
Next.js supports API Routes, enabling you to build your API directly within your Next.js application. This feature allows you to create server-side endpoints that can handle requests and return JSON responses without the need for a separate backend server.
API Routes are a powerful tool for building robust APIs that integrate seamlessly with your frontend application. They enable you to define server-side endpoints that can be used to handle requests and return data in a JSON format.
Explore further: Next Js Single Page Application
To create an API Route in Next.js, you can simply create a new file in the pages/api directory. This is where Next.js will look for API Route files by default, making it easy to get started with API development.
API Routes can be used to handle a wide range of requests, from simple GET requests to more complex POST and PUT requests. By using API Routes, you can keep your API logic separate from your frontend code, making it easier to manage and maintain your application.
Consider reading: Cookies Nextjs
Dynamic Pages and Routing
To create dynamic pages in a JSON Next.js project, you need to define a Catch all Route. This is done by creating a file named [...slug].jsx, which imports content from a JSON file and uses it to format each page.
The [...slug].jsx file defines two important functions: getStaticPaths and getStaticProps. The getStaticPaths function returns a list of all dynamic routes that the catch all component should render. This list is an object with a paths property, which contains an array of route objects, each with a params property.
A unique perspective: File Upload Next Js Supabase
Each route object contains an array of path components separated by slashes. For example, the route object for the path /some/nested/route would look like this: {params: ['some', 'nested', 'route']}. This is how Next.js knows which routes to prerender.
The getStaticProps function returns the component props given the context. In the example, it uses the params property to create an actual path string and searches for a matching page in the pages array. Once it finds the matching page, it returns it as a props object.
If you encounter an error like "Error occurred prerendering page "/[…slug]" during the build, it's likely due to a bug in the version of Next.js used in the example. You can check the [...slug].jsx file on GitHub for a possible solution.
Next.js automatically creates all necessary files for dynamic routes and static pages. However, a navigation that lists all pages is still missing.
Intriguing read: Next Js Props
Logging and Debugging
Automatically patched globals with next-logger can format your logs as JSON and add metadata like hostname and timestamp.
Using next-logger with Next.js allows you to continue using console.log without code changes.
In Next.js, you can enable next-logger in next.config.js for a convenient logging solution.
next-logger uses Next.js Instrumentation hooks to replace the built-in logger with their custom one.
This implementation works well for running your own Next.js server or using serverless functions with the Node runtime.
Recommended read: Using State in Next Js
Add a Backend
Next.js makes it easy to create a backend API with its built-in API route handlers. This means you can focus on building your application without worrying about setting up a separate backend server.
To get started, you'll define routes using the file-system-based router, where the folder structure directly defines the routes. This approach is straightforward and intuitive.
You'll need to create a simple API that returns information about dinosaurs. This can be done by creating a folder structure that defines the routes, such as /api, /api/dinosaurs, and /api/dinosaur/[dinosaur].
The first route, /api, will return the string "Welcome to the dinosaur API". This sets the stage for the rest of your API.
Discover more: Is Next Js Backend
Next, you'll set up /api/dinosaurs to return all the dinosaurs. This will be a crucial part of your API, allowing users to access all the dinosaur data.
Finally, you'll create /api/dinosaur/[dinosaur] to return a specific dinosaur based on the name in the URL. This will enable users to access individual dinosaur data.
By following these steps, you'll have a basic backend API up and running with Next.js.
See what others are reading: Nextjs App Route Get Ssr Data
Sources
- https://blog.arcjet.com/structured-logging-in-json-for-next-js/
- https://frontaid.io/blog/using-nextjs-with-json-file/
- https://www.restack.io/p/nested-json-structure-examples-answer-return-json-next-js
- https://docs.deno.com/runtime/tutorials/how_to_with_npm/next/
- https://developer.mozilla.org/en-US/blog/static-site-generation-with-nextjs/
Featured Images: pexels.com