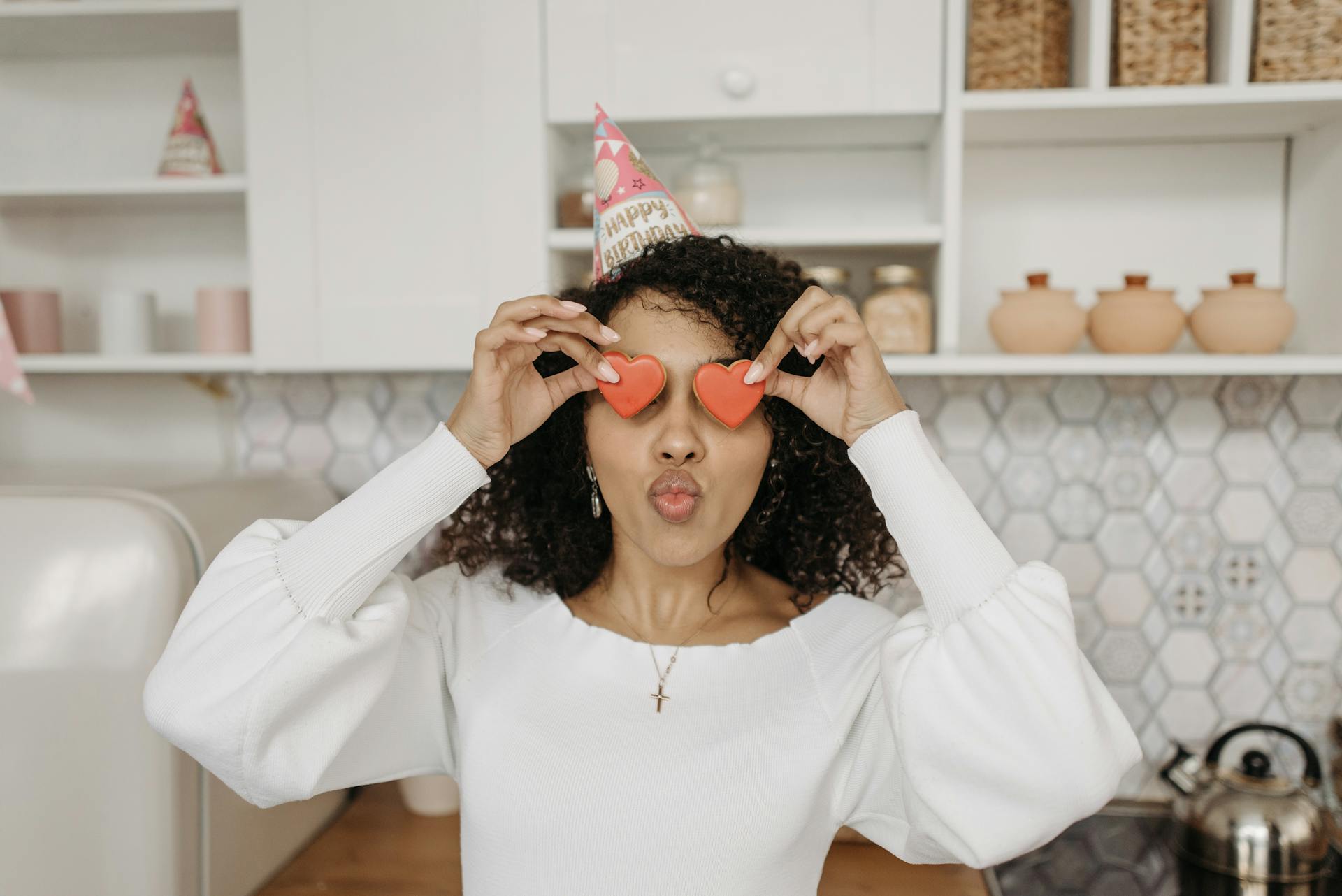
When using Next JS, it's essential to follow best practices for props to ensure your web application scales well.
Use a single source of truth for props, such as a centralized API or a library like Redux, to avoid prop duplication and inconsistencies.
A well-structured props system can make or break the performance and maintainability of your application.
In Next JS, props are passed from the parent component to the child component through the `props` object, but it's crucial to use destructuring to avoid unnecessary prop assignments.
Use default prop values to ensure that your components render correctly even when props are missing or incorrect.
Destructuring props in the Next JS `getStaticProps` method can be used to pre-render pages with dynamic props, but it requires careful handling of prop dependencies.
See what others are reading: Next Js Single Page Application
Understanding Props
Props are a fundamental concept in Next.js, allowing you to pass data from a parent component to its child component. This is done through the use of props, which can be effortlessly transferred between components.
If this caught your attention, see: Next Js Loading Initial Props
In Next.js, data can be transferred from a parent component to its child component through props, just like in React. This approach is straightforward and widely used.
Props can be used to transfer any type of data, including strings, numbers, and objects. For example, data can be transferred from a parent component to its child component through props, as shown in the example.
The data received through props can be used to render content inside a component. In the example, the ChildComponent receives the data through props and renders it inside a paragraph tag.
Recommended read: Nextjs App Route Get Ssr Data
Props in Next.js
Props in Next.js are a crucial part of data fetching and rendering strategies. They allow you to pass data from the server to the client-side component, enabling dynamic rendering and improved performance.
getServerSideProps returns an object with the props key containing the fetched data, which is automatically passed as props to the page component. This object can also include the revalidate option to specify how often the data should be revalidated and regenerated.
See what others are reading: Next Js Client Side Rendering
With getServerSideProps, you know that data is fetched only during the initial server-side request, making it easier to understand when and where data is retrieved. This approach is ideal for pages with dynamic routes, where the content depends on the route parameters.
Here are some key benefits of using props in Next.js:
- Improved performance by reducing unnecessary data fetching
- Better predictability of server-side data fetching
- Enhanced security by fetching authenticated data securely on the server side
The getServerSideProps lifecycle executes on each request, making it suitable for pages that require dynamic data or data that changes frequently. This lifecycle takes a context object as a parameter, which contains page data such as params, res, req, query, etc.
In contrast, getStaticProps returns an object with the props key containing the fetched data, which is used to pre-render the page into a static HTML file. This approach is ideal for pages with content that doesn’t change frequently.
Here's a summary of the differences between getServerSideProps and getStaticProps:
Optimizing Props
getServerSideProps runs on every request, which means it can slow down your site if you're doing a lot of data fetching or computations.
Returning the required props object with the props property can help reduce the number of repetitive calls. This is because Next.js will memoize the request and reuse the result for subsequent calls, avoiding multiple requests to the server.
Smarter caching can also improve performance by caching the results of your request based on the content that was requested. This means that if a user requests a specific page, Next.js will only cache the data for that page.
This approach is different from traditional caching, which simply stores the entire site in a cache and serves it to all users. As a result, smarter caching can save a lot of time by not having to load the entire site from scratch.
Incremental updates are another benefit of smarter caching, allowing only the affected parts of the site to be updated in the cache after making a small change to your code.
If this caught your attention, see: Next Js Typescript Example Props
Data Transfer
Data Transfer is a fundamental concept in Next.js, and understanding how it works is crucial for building efficient and scalable applications.
In Next.js, data transfer occurs between pages through the use of props, which are short for properties. Props are used to pass data from a parent component to its child components.
The `getStaticProps` method is a key component of data transfer in Next.js, allowing developers to pre-render pages at build time. This method is particularly useful for static sites and blogs.
By using `getStaticProps`, developers can fetch data from external APIs or databases and pass it to their pages as props. This approach enables fast page loads and improved SEO.
In Next.js, props can also be passed between pages using the `Link` component. This component allows developers to create links between pages while preserving the context of the current page.
The `Link` component uses the `as` prop to specify the URL of the linked page, while the `href` prop is used to specify the URL of the linked page in the browser's address bar.
Suggestion: Next Js Using State Context
Converting Props
You can convert your existing data fetching logic from getInitialProps to getServerSideProps or getStaticProps in Next.js.
Migrating from getInitialProps to getServerSideProps involves three steps: removing getInitialProps, replacing it with getServerSideProps, and passing props to the component.
First, remove getInitialProps from your page components. This is the first step in both migrating to getServerSideProps and getStaticProps.
To replace getInitialProps with getServerSideProps, you need to change the method in your page components. The structure of getServerSideProps is different from getInitialProps, and it returns an object with the props key containing the fetched data.
With getServerSideProps, the fetched data will be automatically passed as props to the page component. You can access this data using the props parameter of your page component.
Here's a brief comparison of the two methods:
Data revalidation is an optional feature with getStaticProps that allows you to specify how often the data should be revalidated and regenerated. This is useful when you want to update the data periodically without redeploying your application.
Additional reading: Next Js Fetch Data save in Context and Next Route
Sources
- https://www.geeksforgeeks.org/next-js-getinitialprops/
- https://blog.logrocket.com/data-fetching-next-js-getserversideprops-getstaticprops/
- https://medium.com/@tyliang/nextjs-efficient-data-transfer-leveraging-react-props-and-context-in-next-js-b85bd87a77a2
- https://www.storyblok.com/tp/add-a-headless-cms-to-next-js-in-5-minutes
- https://www.shecodes.io/athena/41267-how-to-use-props-between-pages-in-next-js
Featured Images: pexels.com