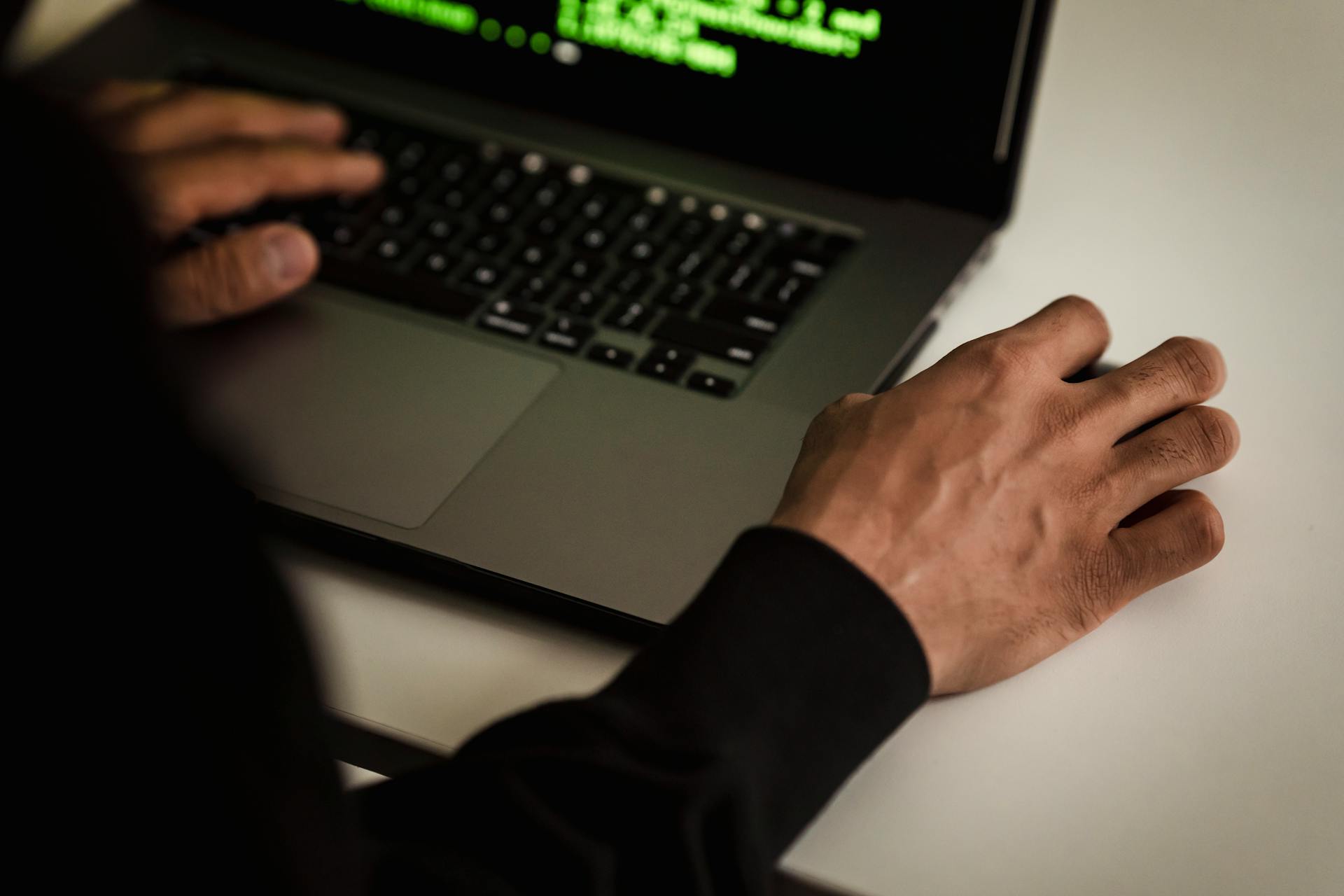
Props in Next.js with TypeScript can be a bit tricky to understand at first, but don't worry, we'll break it down in a way that's easy to grasp.
One of the key things to know is that in Next.js with TypeScript, props are type-checked at runtime, which means you can catch errors early on and avoid runtime crashes.
To make the most of this feature, you can use the `type` attribute in your JSX elements to specify the expected type of the prop. This is especially useful for complex components where you need to ensure that props are passed in the correct format.
For example, if you have a component that expects a string prop, you can define it like this: `type Props = { name: string; }`.
Expand your knowledge: Next Js Props
TypeScript Basics
TypeScript is a superset of JavaScript that adds optional static typing and other features to improve the development experience.
It's compiled to JavaScript, allowing you to write modern JavaScript code with optional static type checking.
The TypeScript compiler, tsc, is used to compile TypeScript code into JavaScript.
TypeScript code is written in .ts files, which are then compiled to .js files.
Optional static typing means you can add type annotations to your code, which can help catch errors at compile-time.
This allows you to write more robust and maintainable code.
Passing Props
You can pass props to a child component in two steps. First, pass some props to the child component, such as an object and a number.
Passing props to a child component is useful for giving it specific information it needs to render correctly. For example, let's pass two props, person and size, to the Avatar component.
To pass props, you don't need to "know" what's being rendered inside of the component. The Card component, for instance, can wrap any nested content, including JSX, and receive it as a prop called children.
You can think of a component with a children prop as having a "hole" that can be "filled in" by its parent components with arbitrary JSX. This flexible pattern is often used for visual wrappers like panels and grids.
See what others are reading: Nextjs Pathname Server Component
If you need to add more icon folders, you can chain ternary operations to accept multiple params or conditions. This way, the component can handle different icon folders and still render correctly.
In some cases, you may want to forward all of your props to a child component using the JSX spread syntax. This can make your code more concise, but use it with restraint, as it can indicate that you should split your components and pass children as JSX.
Requiring more props can be done by using the ternary operator to add a new prop that takes a new icon path as data. This way, you can add new functionality without breaking existing code.
Discover more: Next Js Component Library
Optional Props
Optional props can be a real pain to deal with, especially when they're not optional by default. You can make a prop optional by using the concept of optional chaining in JavaScript.
This means you can modify a prop to be optional without having to pass a specific value every time. For example, if you have a component that uses an icon, you can make the dashboard prop optional by using optional chaining.
If you have a component that looks like this, it can be a real mess when the project grows and you have more icons in their respective modules. But with optional chaining, you can modify the prop to be optional and avoid this issue.
A default value for a prop can be specified using destructuring, by putting = and the default value right after the parameter. For instance, if you have a component that uses an avatar, you can specify a default size of 100 by passing size={100}.
Forwarding Props
Forwarding props can be a convenient way to pass all props from a parent component to its child, especially when the child doesn't use any of its own props. This is known as the spread syntax.
Use spread syntax with restraint, as it can indicate that your components are too complex and need to be split. You can think of it as a "hole" that can be filled in by its parent components with arbitrary JSX.
The spread syntax can be useful for components that forward all of their props to their children, like the Profile component which forwards all its props to the Avatar component. This can make your code more concise and easier to read.
If this caught your attention, see: Useeffect Nextjs
Forwarding with JSX Spread
Forwarding all props to a child component can make sense if you don't use any of your props directly.
Repetitive code can be more legible, but using the spread syntax can be more concise.
Use spread syntax with restraint, as it can indicate that you should split your components and pass children as JSX.
The Clock component receives two props from its parent component: color and time.
Take a look at this: How to Use Reducer Api in Next Js 14
Scaling This Component
Scaling this component is definitely possible, especially when you consider chaining ternary operations to accept multiple params or conditions.
This approach allows the component to handle multiple icon folders and props, making it more versatile and adaptable.
You can pass specific props, such as settings, to access icons from particular folders.
The default path for the component's interface is always "/icons/${name}.svg", which means all other props are optional.
Recommended read: Next Js Loading Initial Props
Advanced Topics
Static site generation is a powerful feature in Next.js, and you can use Prisma to query your database at build time with the getStaticProps function.
Prisma can be used inside getStaticProps to send queries to your database, allowing you to render static pages with dynamic data.
Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
Next.js and Prisma are a great combo for React apps that need a database, offering full rendering flexibility and top performance.
A different take: React and Next Js
Featured Images: pexels.com