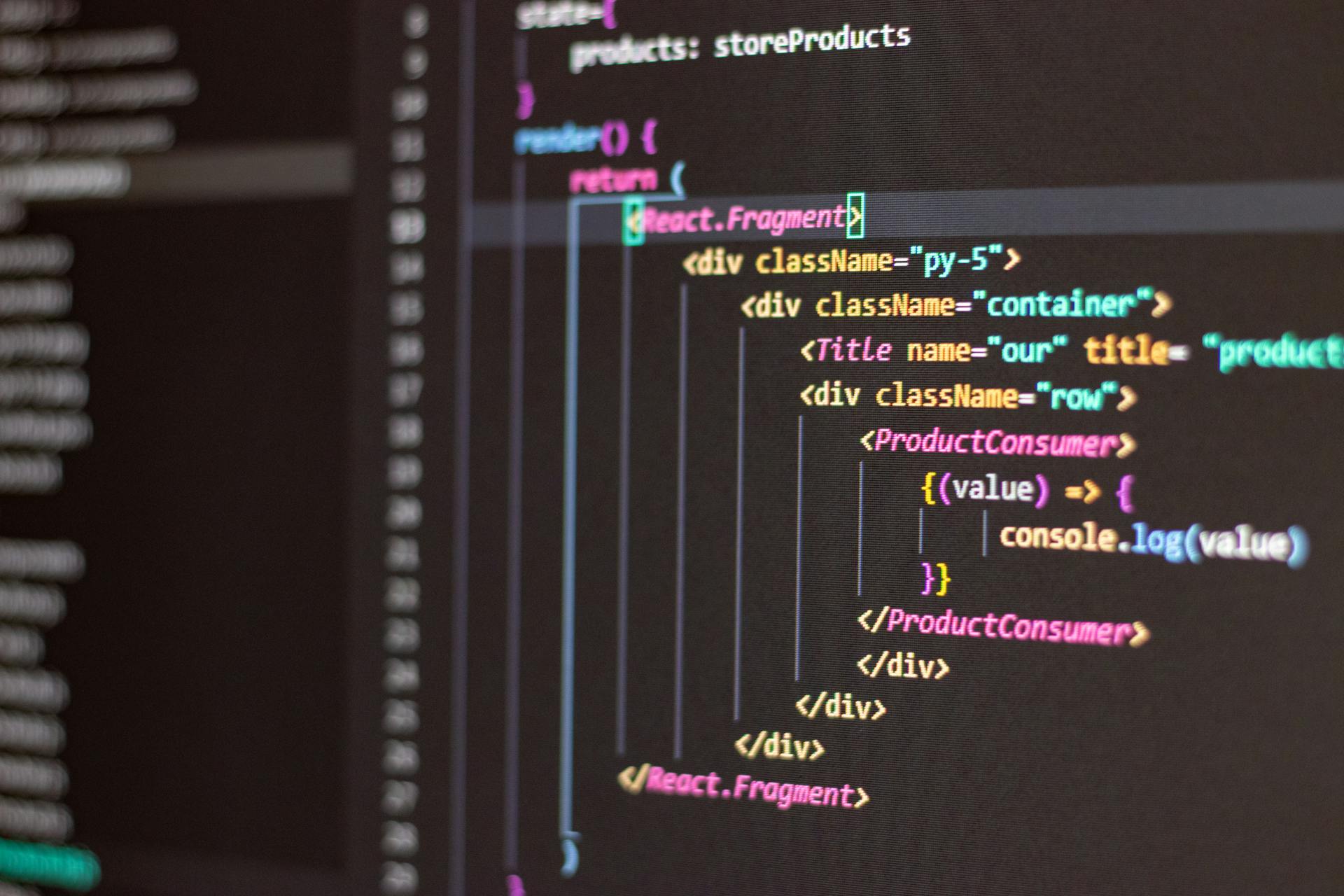
Using a Next Js component library can significantly speed up your web development process.
Next Js is built on top of React, allowing for seamless integration with existing React projects.
This means you can leverage the vast ecosystem of React components and libraries, making development even more efficient.
A Next Js component library can help you create reusable UI components, reducing code duplication and making maintenance easier.
For example, you can use a library like Tailwind UI to create customizable UI components that fit your project's style.
Check this out: Next Js Movie Streaming Ui
Popular Next.js Component Libraries
If you're looking for popular Next.js component libraries, you've got a few great options to choose from. One of them is Shuffle, which offers 38+ Tailwind templates and a component library to help you get started.
Ripple UI is another framework worth checking out, built on top of Tailwind CSS and featuring reusable components.
You might like: Nextjs Pathname Server Component
Shuffle Library
Shuffle Library is a great choice for Next.js developers. It offers a large collection of 38+ Tailwind templates and a component library to speed up your development process.
Recommended read: Icons in Next Js
Shuffle Library is known for its simplicity and ease of use. It's a great resource for anyone looking to get started with Next.js quickly.
One of the standout features of Shuffle Library is its extensive template collection. This can be a huge time-saver for developers who need to build multiple projects with similar layouts.
You might enjoy: Next Js Performance Analyzer Library Npm
Ripple UI
Ripple UI is a custom framework built on top of Tailwind CSS, offering a collection of reusable components.
With Ripple UI, developers can access a library of pre-designed components to enhance their Next.js projects.
Ripple UI's framework is designed to be highly customizable, allowing developers to tailor the components to their specific needs.
Ripple UI is a great option for developers looking for a flexible and modular approach to building their Next.js applications.
Ripple UI's components are built with Tailwind CSS, a popular utility-first CSS framework known for its simplicity and ease of use.
Ripple UI's reusable components can be easily integrated into any Next.js project, saving developers time and effort.
Ripple UI's framework is ideal for developers who want to create custom and unique user interfaces for their Next.js applications.
Ripple UI's components are designed to be highly responsive, ensuring a smooth user experience across various devices and screen sizes.
Consider reading: Next Js Components Folder
API and Integration
Next.js allows for the creation of API routes alongside components, enabling seamless integration of frontend and backend logic within a single project.
This reduces the need for separate backend services for simple APIs, streamlining development. By integrating API routes, you can build more efficient applications.
Next.js also provides specialized functions for data fetching within components, aligning with its rendering strategies (SSR and SSG).
Readers also liked: Styled-components Next Js
API Routes Integration
API Routes Integration is a powerful feature that allows you to create API routes alongside your components, enabling seamless integration of frontend and backend logic within a single project.
This reduces the need for separate backend services for simple APIs, streamlining development and making it easier to manage your project's complexity.
By integrating API routes with your components, you can create a more cohesive and efficient development workflow.
You might enjoy: Next Js Component
Data Attributes
Data Attributes are a powerful way to add functionality to your UI components.
You can use data attributes to set up a modal component by adding the data-modal-target attribute to the modal element and the data-modal-{toggle|show|hide} attribute to the trigger element.
To toggle, show, or hide the component, you simply need to click on the trigger element.
A unique perspective: Next Js Modal
ESM and CJS
Flowbite's API supports both CJS and ESM for JavaScript, which can be helpful if you need to expand the default capabilities of the data attributes interface.
This means you can choose the format that works best for your project. Flowbite's API is flexible and adaptable.
You can import and create a new Modal component inside JavaScript, as shown in an example.
Creating and Using Components
Creating a Next.js component library requires a solid understanding of how to create and use components. To start, you'll want to organize your components logically, grouping related components together in directories like components, layouts, and pages.
This structure promotes maintainability and reusability. For example, you can create a Button component in the components directory and then use it in multiple pages.
Here are some common use cases for Next.js components:
- Navigation Bars: Creating reusable headers and navigation menus that appear across multiple pages.
- Forms: Building forms for user input, handling validation, and submission.
- Cards and Lists: Displaying data in structured formats like cards, tables, or lists.
- Modals and Dialogs: Creating pop-up components for user interactions without navigating away from the current page.
- Dynamic Content: Rendering content based on user interactions or fetched data, enhancing interactivity.
Types of
Creating a robust and maintainable application requires a well-structured approach to component development. Next.js offers a flexible and intuitive way to organize components into different types, each serving a specific purpose.
Page Components are located in the pages directory, where each file automatically becomes a route in the application, simplifying the routing process.
Layout Components define the common layout structure shared across multiple pages, promoting consistency and reducing redundancy.
UI Components are reusable components like buttons, forms, modals, and card elements that can be used across different parts of the application to maintain a consistent look and feel.
Dynamic Components are used to load data dynamically or change based on user interactions, enhancing the interactivity and responsiveness of the application.
Here's a summary of the different types of components in Next.js:
- Page Components: Located in the pages directory, each file becomes a route in the application.
- Layout Components: Define the common layout structure shared across multiple pages.
- UI Components: Reusable components like buttons, forms, modals, and card elements.
- Dynamic Components: Load data dynamically or change based on user interactions.
Structure of a
A Next.js component is essentially a standard React component with some added Next.js features. It's like a supercharged React component.
To start building a Next.js component, you'll need to import any necessary libraries, other components, or styles. This is typically done in the import statements section of your component.
A typical Next.js component follows a specific structure, which includes defining the component itself, and then exporting it so it can be used elsewhere in your application. This is where the magic happens!
See what others are reading: Next Js Component Rendering Analyzer
Here are the essential parts of a Next.js component:
- Import Statements: Bring in necessary libraries, other components, and styles.
- Component Definition: Define the component using either a functional or class-based approach.
- Export Statement: Export the component to make it available for use in other parts of the application.
This structure is key to creating reusable components that can be easily dropped into different parts of your application.
Creating and Using
Creating and Using Components is a fundamental part of building robust and maintainable applications, especially with Next.js.
To create a Next.js component, start by creating a file in the components directory, such as Button.js, which encapsulates both functionality and styling.
A typical Next.js component follows the structure of a standard React component, augmented with Next.js-specific features. This includes import statements, component definition, and export statements.
You can use Next.js components to create reusable headers and navigation menus that appear across multiple pages, like navigation bars.
Some common use cases for Next.js components include building forms for user input, handling validation, and submission, as well as displaying data in structured formats like cards, tables, or lists.
To use a Next.js component in a page, create or update a page to include the component, promoting reusability and maintainability.
Related reading: Nextjs 404
Here are some common use cases for Next.js components:
Components can be easily reused across different pages or sections of the application, showcasing the modularity that Next.js Components provide.
Styling and Optimization
Next.js offers built-in image optimization, allowing components to leverage optimized images that are automatically resized, formatted, and served efficiently. This feature improves page load times and enhances visual quality without additional configuration.
Next.js supports various styling options, including CSS Modules, styled-jsx, and integration with CSS-in-JS libraries like styled-components. This flexibility enables developers to choose the styling approach that best fits their workflow and project requirements.
You can use styled-jsx for scoped styling directly within the component, making it easy to manage styles. Just remember to import the library and use it within your components.
The Image component in Next.js automatically optimizes images, reducing load times and improving visual quality without additional effort. This is a game-changer for performance-critical applications.
Recommended read: How to Use Reducer Api in Next Js 14
Libraries like styled-components or emotion can be integrated for more advanced styling needs, offering dynamic styling capabilities. This is particularly useful when working on complex projects that require fine-grained control over styles.
Optimizing Next.js Components is crucial for delivering a smooth user experience, and there are several techniques to achieve this, such as using the Image component or leveraging styled-jsx. By applying these techniques, you can ensure your components are efficient and performant.
Optimization Techniques
Image optimization is a must in Next.js, and it's surprisingly easy to set up. Next.js offers built-in image optimization, allowing components to leverage optimized images that are automatically resized, formatted, and served efficiently.
To get the most out of Next.js, make sure to utilize static generation where possible. This means using getStaticProps for pages that don’t require frequent updates, resulting in faster load times and better performance.
Minimizing JavaScript payloads is also crucial for a smooth user experience. Keep JavaScript bundles lean by avoiding unnecessary libraries and optimizing imports, and don't forget to take advantage of tree-shaking to remove unused code and further reduce bundle sizes.
Discover more: Nextjs Set Background Image
Image Optimization
Next.js offers built-in image optimization, allowing components to leverage optimized images that are automatically resized, formatted, and served efficiently.
This feature improves page load times and enhances visual quality without additional configuration. Next.js's Image component automatically optimizes images, reducing load times and improving visual quality without additional effort.
The Image component optimizes image loading and performance automatically, handling image resizing, format selection, and lazy loading. This means you can focus on building your application without worrying about image optimization.
Take a look at this: Next Js 14 Redirect to Another Page Loading Indicator
Optimizing
Optimizing is a crucial step in delivering a smooth user experience. Next.js offers built-in image optimization, allowing components to leverage optimized images that are automatically resized, formatted, and served efficiently.
Image optimization is a game-changer for page load times and visual quality. Next.js's Image component automatically optimizes images, reducing load times and improving visual quality without additional effort.
To further optimize your Next.js components, consider utilizing static generation where possible. Static Site Generation builds pages at build time, resulting in faster load times and better performance.
Readers also liked: Nextjs 14 Image
Static generation can be particularly effective for pages that don't require frequent updates. Use getStaticProps for these types of pages.
Minimizing JavaScript payloads is another key optimization technique. Keep JavaScript bundles lean by avoiding unnecessary libraries and optimizing imports.
Tree-shaking helps remove unused code, further reducing bundle sizes. This can make a significant difference in the performance of your application.
Broaden your view: Nextjs Pages
Ecosystem and Migration
Next.js boasts a rich ecosystem of tools and libraries that enhance component development, including Vercel, Tailwind CSS, Styled-Components, Storybook, and NextAuth.js.
These tools can significantly streamline your development process, providing robust solutions for common challenges.
To leverage these tools effectively, you can integrate them into your Next.js project using the Create Next App tool, which provides a ready-to-use project structure.
Here's a brief overview of some of the key tools in the Next.js ecosystem:
The Ecosystem
Next.js has a rich ecosystem of tools and libraries that can greatly enhance your component development experience. This includes Vercel, the platform behind Next.js, which offers optimized deployments and powerful serverless functions.
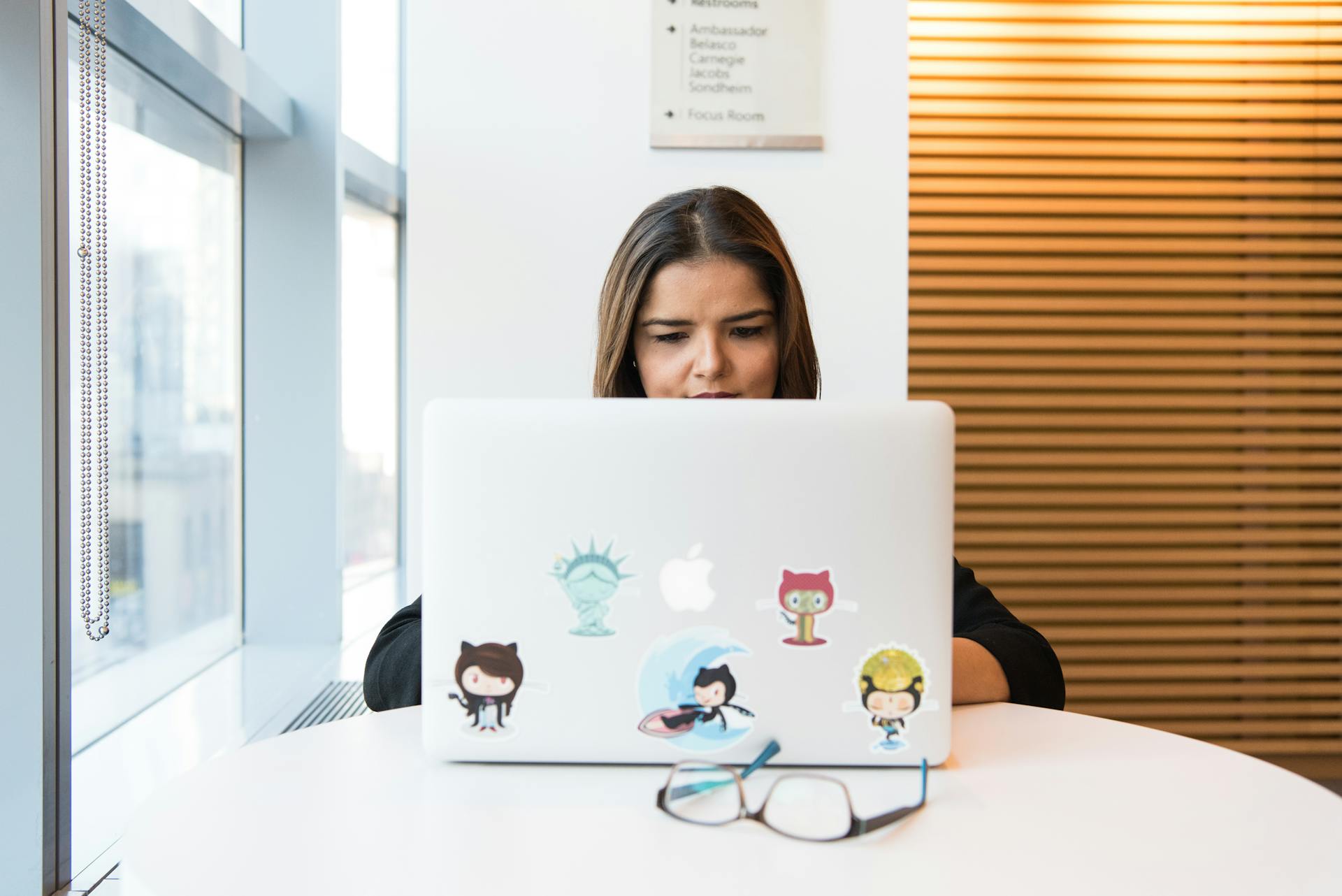
Vercel is the platform behind Next.js, offering optimized deployments and powerful serverless functions. Tailwind CSS is a utility-first CSS framework that pairs well with Next.js for rapid styling.
Tailwind CSS is a utility-first CSS framework that pairs well with Next.js for rapid styling. Styled-Components is a popular CSS-in-JS library that allows for dynamic and scoped styling within components.
Styled-Components is a popular CSS-in-JS library that allows for dynamic and scoped styling within components. Storybook is a tool for developing and testing UI components in isolation, facilitating better design and collaboration.
Storybook is a tool for developing and testing UI components in isolation, facilitating better design and collaboration. NextAuth.js is an authentication library that integrates seamlessly with Next.js Components to manage user authentication and authorization.
NextAuth.js is an authentication library that integrates seamlessly with Next.js Components to manage user authentication and authorization. By leveraging these tools, you can significantly streamline your development process and provide robust solutions for common challenges.
Here are some key tools to consider:
- Vercel: Optimized deployments and serverless functions
- Tailwind CSS: Utility-first CSS framework for rapid styling
- Styled-Components: Dynamic and scoped styling within components
- Storybook: Developing and testing UI components in isolation
- NextAuth.js: Seamless integration for user authentication and authorization
Migrating Existing
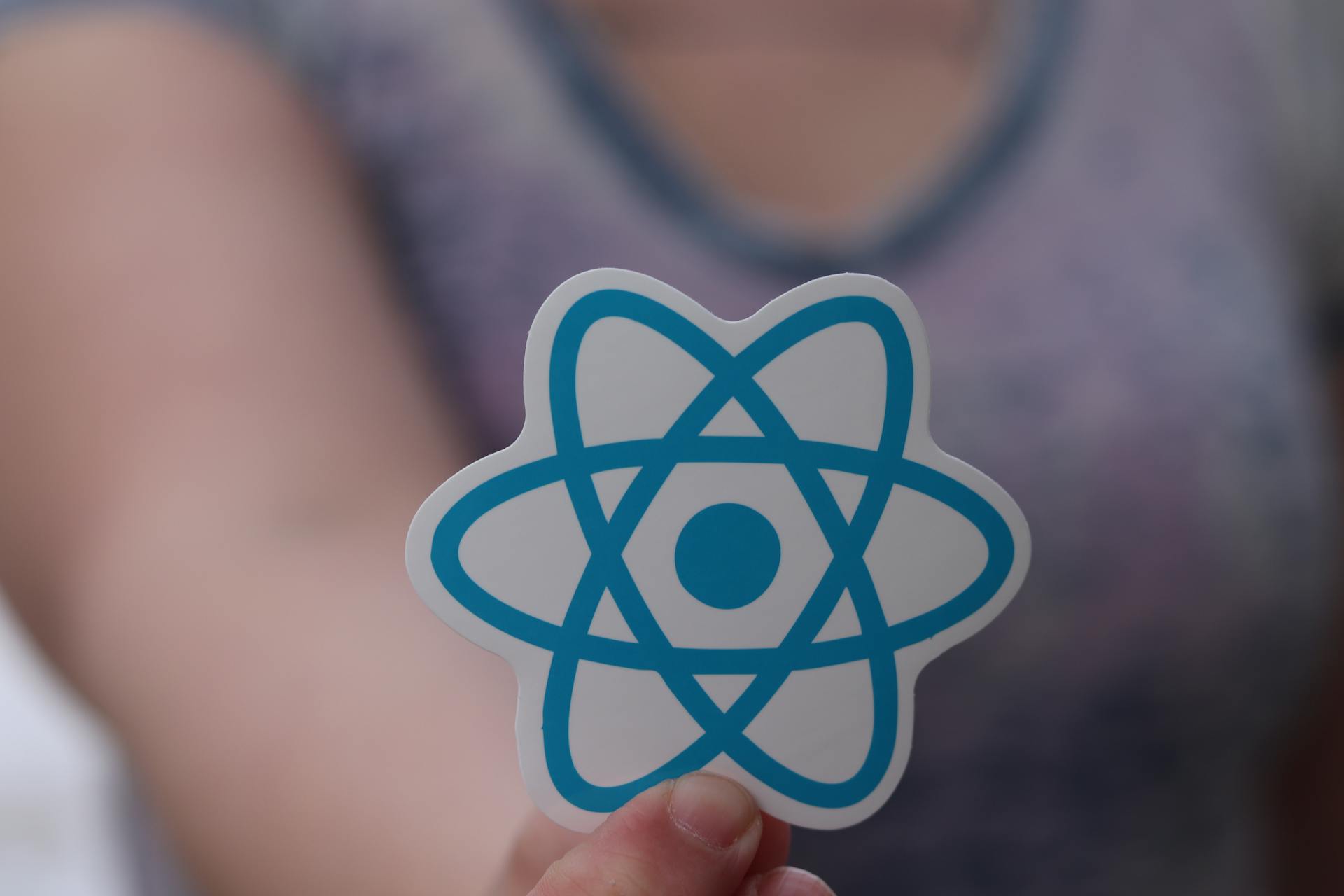
Migrating from another framework or vanilla React to Next.js involves several steps to ensure a smooth transition.
You'll need to analyze your existing components to understand how they'll fit into the Next.js framework. This involves examining their structure, functionality, and dependencies.
Set up a new Next.js project using the Create Next App tool, which provides a ready-to-use project structure.
Recreate your existing component structure by moving them into the components directory of your Next.js project. Adjust imports and exports as needed to align with Next.js conventions.
Here are the key steps to follow:
- Analyze Existing Components
- Set Up the Next.js Project
- Recreate Component Structure
- Integrate Routing
- Adjust Data Fetching Methods
- Optimize Styling
- Test Thoroughly
A methodical approach to migration helps preserve functionality while leveraging Next.js’s powerful features.
Featured Images: pexels.com