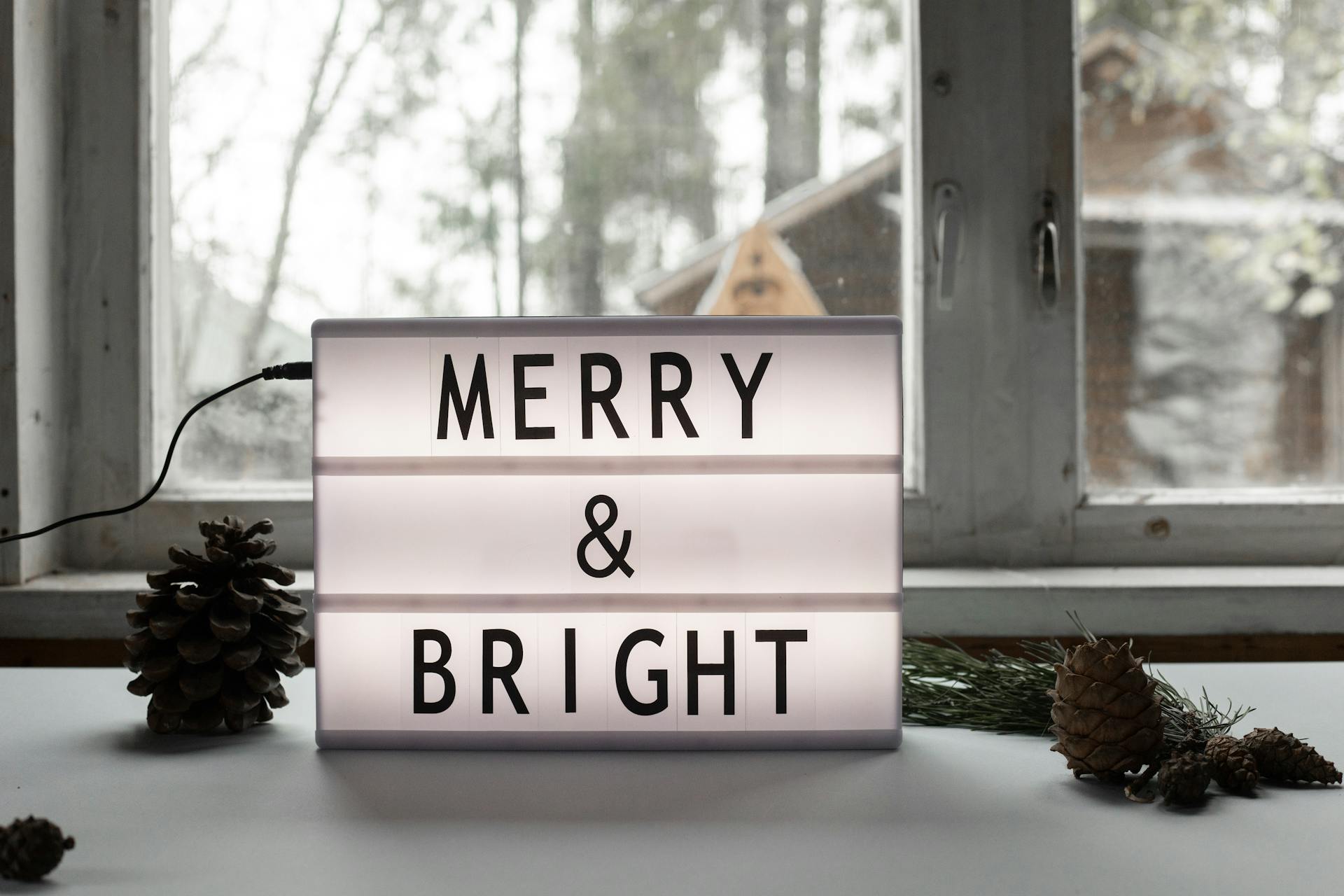
You can create a modal in Next Js using the built-in `useState` hook to manage the modal's state. This is a great way to add a modal to your Next Js app.
To customize the modal, you can use CSS to style it and add your own content. For example, you can add a title, a description, and a button to close the modal.
In Next Js, you can also use the `useEffect` hook to handle the modal's lifecycle, such as when the modal is opened or closed. This can be useful for adding animations or other effects to the modal.
For another approach, see: Nextjs Context
Creating a Reusable Modal
A reusable modal is a game-changer for any web application. It contains the RenderInner component that we want to display inside the modal and its required props.
To make a modal reusable, you'll want to pass in the necessary properties as props to it. This includes the source attribute of the thumbnail image, the dimensions of the thumbnail image, the alternative text for the thumbnail image, the source attribute of the video, and the dimensions of the video.
Explore further: Next Js Props
Here's a list of the properties you'll want to pass to your reusable modal:
- Source attribute (src) of the thumbnail image
- Dimensions of the thumbnail image
- Alternative text (alt) for the thumbnail image
- Source attribute (src) of the video
- Dimensions of the video
By passing these properties as props, you'll be able to reuse your modal component across your application.
Modal Component
The Modal Component is a crucial part of any Next.js application.
To open a modal, you can use the `open` method, which creates a ref for the modal and updates the state of the wrapper component to show the modal. You can even open multiple modals on top of each other.
The `close` method is used to close the modal, and the `updateProps` method allows you to update the props within the modal from any component where the modal is used.
To register a modal in your Next.js application, you need to do it in the root file, specifically in `_app.tsx`. This is where you assign the ref to the modal wrapper component and control the opening and closing of the modal.
Here are the key points to keep in mind when registering a modal:
- Assign the ref to the modal wrapper component in `_app.tsx`.
- Use the `open` and `close` methods to control the modal's visibility.
- Use the `updateProps` method to update the props within the modal.
Modal Behavior
To control the behavior of your modal in Next.js, you can use the toggle function on the Modal object to toggle the modal element's visibility. This function is part of the Modal object's methods.
You can also use the show and hide functions to explicitly show or hide the modal element. The isHidden and isVisible functions can be used to check if the modal is hidden or visible. For example, you can use the isHidden function to check if the modal is hidden before toggling it.
Here are the Modal object's methods:
Defining the Initial State with useState
To start, you need to define the initial state of the modal. This is done by using the useState hook from React and setting the initial state to false. By doing this, the modal will be closed by default.
The useState hook is a built-in React hook that allows you to add state to functional components. In this case, we're using it to define the initial state of the modal.
Here's an example of how to use useState to define the initial state of the modal:
```jsx
const [modalOpen, setModalOpen] = useState(false);
```
This code creates a state variable called modalOpen and sets its initial value to false. The setModalOpen function is used to update the state later on.
Here's an interesting read: Nextjs Usestate
Playing Automatically When
In Next.js, the modal content gets unmounted when the modal is closed, so we don't need to worry about pausing the video when the modal is closed.
To start a video automatically when a modal opens, you need to reference the video and tell it to start playing. We can't use the usual useEffect React hook because the video is inside a component that gets unmounted when the modal closes, and the ref would return null.
The afterEnter callback in the Transition component is the way to go. This callback runs after the modal has finished opening, so you can access the video element through the ref and tell it to play.
You can also pass the videoRef to the Dialog's initialFocus property to ensure the video element is focused when the modal is opened.
For another approach, see: Next Js Video
Object Parameters
To initialize a Modal object, you need to set the main modal element as a JavaScript object. This is achieved by passing the target element as a required parameter.
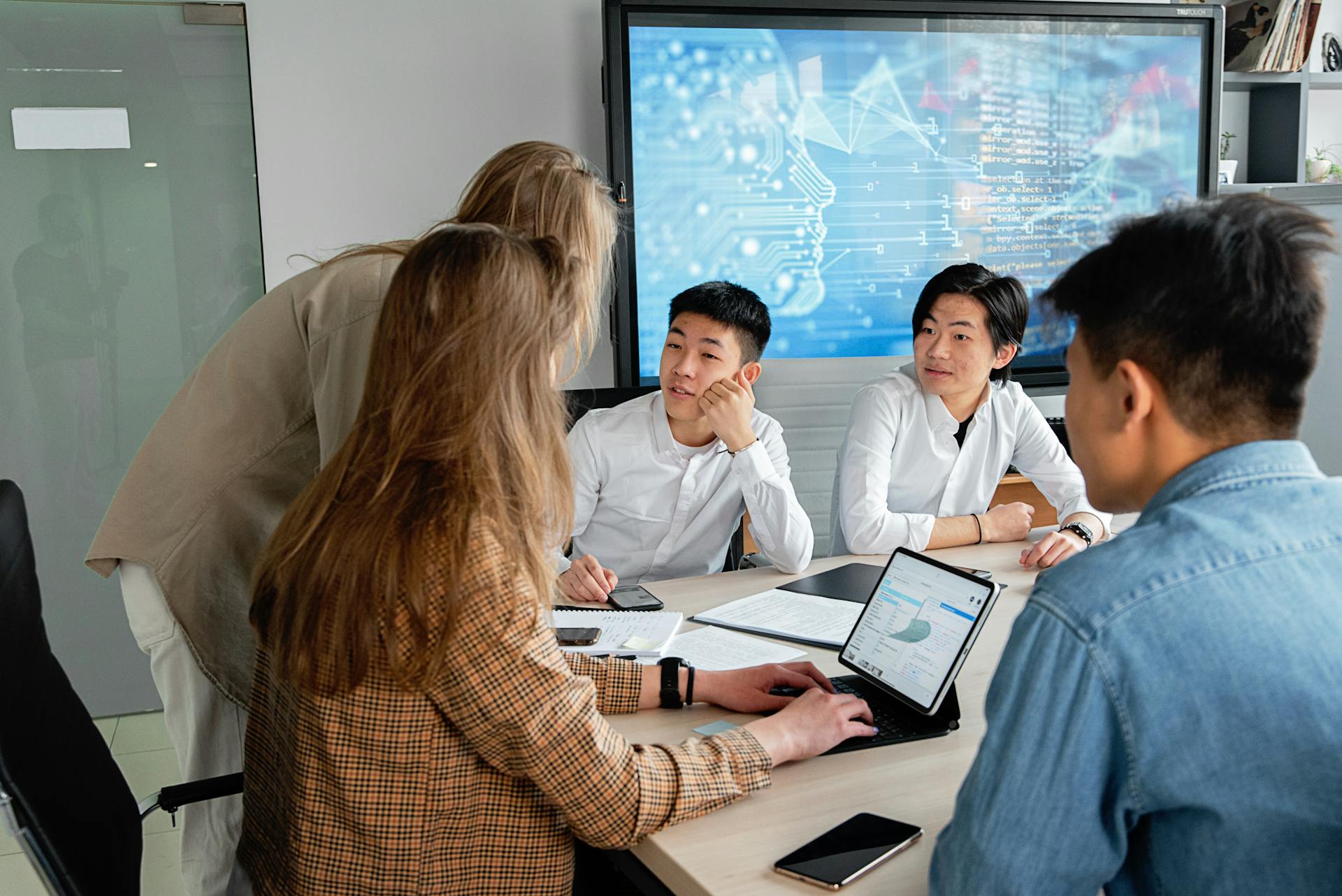
The target element is the main modal element that will be used as a JavaScript object. It's a required parameter, so make sure to include it when initializing the Modal object.
You can also pass an optional options object to set the default state of the modal, placement, and animations. This options object allows you to customize the modal's behavior.
Here's a breakdown of the parameters you can pass to initialize a Modal object:
By understanding these parameters, you can effectively initialize a Modal object and customize its behavior to suit your needs.
Modal Positioning Bottom Right
In Next.js modals, positioning the modal at the bottom right of the screen is a popular choice for a reason. It's a great way to keep the modal out of the way of other content while still being easily accessible.
To position a modal at the bottom right, you can use the `bottom` and `right` properties in the modal's style. For example, `style={{ bottom: 0, right: 0 }}` will place the modal at the very bottom right corner of the screen.
The `zIndex` property can also be used to ensure the modal appears on top of other elements. In the example, `zIndex={1000}` is used to give the modal a high enough index to appear above other content.
By positioning the modal at the bottom right, you can create a clean and unobtrusive user experience. This is especially important for modals that need to be accessed frequently, as it minimizes the amount of screen real estate they occupy.
Modal Customization
You can customize the position of the modal element by using the 'placement' option, which accepts a string value such as 'top-left' or 'bottom-right'.
The 'placement' option allows you to choose from a variety of positions, including 'top', 'center', and 'right', combined with 'left', 'center', or 'right'.
To prevent closing the modal when clicking outside, you can use the 'backdrop' option and choose between 'static' or 'dynamic'.
Here are the available options for the 'backdrop' option:
You can also customize the backdrop element by using the 'backdropClasses' option, which accepts a string of Tailwind CSS classes.
Options
When customizing your modal, you have several options to choose from. The placement option allows you to set the position of the modal element relative to the document body by choosing one of the values from {top|center|right}-{left|center|right}, such as top-left or bottom-right.
You can choose between static or dynamic backdrop options to prevent closing the modal when clicking outside. The backdropClasses option lets you set a string of Tailwind CSS classes for the backdrop element, like 'bg-blue-500 dark:bg-blue-400'.
Setting the closable option to false disables closing the modal on hitting ESC or clicking on the backdrop. You can also set callback functions for various events, such as the onHide, onShow, and onToggle events.
Here are the options in a table format:
Making the Video Component Reusable
Making a video component reusable is crucial for customization. It allows you to use the same component in different parts of your application.
To ensure the modal video component is reusable, you should pass the following properties as props to it: the source attribute (src) of the thumbnail image, the dimensions of the thumbnail image, the alternative text (alt) for the thumbnail image, the source attribute (src) of the video, and the dimensions of the video.
Here's a list of the required props:
- The source attribute (src) of the thumbnail image
- The dimensions of the thumbnail image
- The alternative text (alt) for the thumbnail image
- The source attribute (src) of the video
- The dimensions of the video
To ensure these props are properly structured, you can define an object with a TypeScript interface.
Frequently Asked Questions
What is a modal in NextJS?
A NextJS modal is a lightweight popup window consisting of a header, body, and footer, built with HTML, CSS, and JavaScript. It's a versatile tool for creating interactive and engaging user experiences in your NextJS application.
How to open a modal on button click in NextJS?
To open a modal on button click in NextJS, you'll need to pass a function to handle the button click event and trigger the modal's appearance. The number of buttons and their functionality will determine the complexity of the implementation.
Where is the modal placement in next UI?
The default modal placement in Next UI is auto, which centers the modal on larger screens and places it at the bottom on mobile. You can customize the placement using the placement prop.
Sources
- https://articles.wesionary.team/creating-and-opening-modals-in-global-scope-using-nextjs-746cebfe1c96
- https://utahedu.devcamp.com/dissecting-react-js/guide/triggering-react-modal-open-link-click
- https://cruip.com/how-to-build-a-modal-video-component-with-tailwind-css-and-next-js/
- https://flowbite.com/docs/components/modal/
- https://stackoverflow.com/questions/76268977/how-to-create-a-portal-modal-in-next-13-4
Featured Images: pexels.com