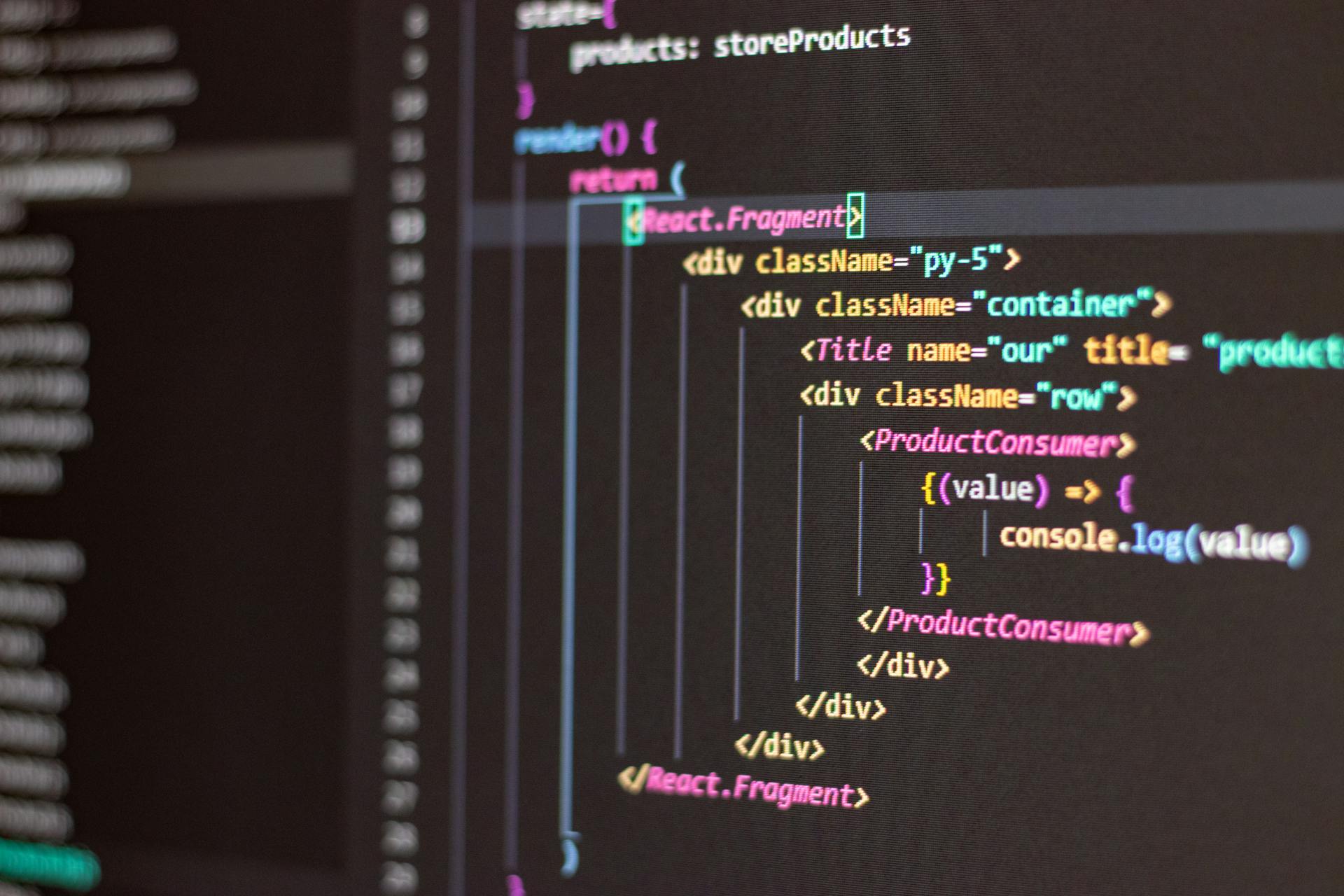
In Next.js, useState is a hook that allows you to add state to functional components.
It's a simple yet powerful tool that helps manage application state.
With useState, you can easily update state variables and trigger re-renders of your components.
This is especially useful when building dynamic user interfaces.
You can use useState to store and update data in your components, like a user's name or a list of items.
For example, you might use useState to store a user's input in a form and then update the component's state with the new input.
By using useState, you can keep your code organized and easy to maintain.
You can also use useState with other hooks, like useEffect, to create more complex state management systems.
Consider reading: Can I Store Json in Nextjs
How It Works
In Next.js, the useState hook is used to manage state in functional components. This hook allows you to add state to a component, and it's a crucial part of building interactive user interfaces.
Each time the user interacts with the component, such as clicking a button, the state is updated. This is a fundamental concept in building dynamic user interfaces.
The state is stored in a JavaScript object, making it easy to access and update. This object is then passed down from the parent element to the child elements, ensuring that all components are aware of the current state.
Regardless of how the application manages its data, the state must always be passed down from the parent element to the child elements. This is a critical aspect of building scalable and maintainable applications.
useState Hook Basics
The useState Hook is a popular method for managing state in Next.js, and it works similarly to traditional React applications.
You can use the useState Hook to keep track of the state of functional components, and it allows you to hold a single value of any data type.
To get started with the useState Hook, you need to call it in the function component, and it will return two values: the current state and a function that updates the state.
For more insights, see: Next Js Function Handlers to Filter Data
The initial state is set to zero (0) by default, but you can set it to any value you want.
The useState Hook takes an initial value as an argument, and it's used to initialize the state.
The state function updater is a special function that updates the state, and it's returned along with the current state.
You can use the state function updater to increase the initial value of the state by 1, as shown in the example where the addCount function is used to increase the initial value of the state by 1.
Recommended read: Can Nextjs Be Used on Traditional Web Application
State Management Techniques
As you start building your Next.js app, you'll need to manage state efficiently. Basic state management in Next.js involves using Hooks, techniques, and tools to keep your code organized.
Prop drilling is a technique used in more advanced applications where you have multiple components. It involves passing state from the parent level to the children, which can be multiple levels deep.
For another approach, see: Next Js Multiple Middleware
To illustrate this, let's consider a basic example with two levels of components. In this case, you'll need to drill through the component to pass values to the children components Message and Button.
SWR, a custom Hook library created by the Next.js team, offers an alternative method for data fetching. It simplifies things like caching, revalidation, and error handling, making it a great choice for scalability.
The useState hook is a widely used method for managing state in functional components. It returns two values: the current state and a function to update the state, which can be used to increase the initial value of the state by 1.
Consider reading: Next Js Components Folder
Basic Management
Basic Management is a fundamental aspect of Next.js state management. In Next.js, you can use the useState hook to manage state in your functional components.
The useState hook is a widely used tool that allows you to keep track of the state of your components. It takes any data type and holds a single value.
A different take: Next Js Component
To initialize the state, you call the useState function in your component, which returns two values: the current state and a function that updates the state. The initial state is set to zero (0) by default.
You can use this function to update the state by passing a new value, as seen in the example where the addCount function increases the initial value of the state by 1.
Prop Drilling Technique
Prop drilling is a technique used in Next.js to manage state in complex applications.
In large applications, you'll divide code into different components to make it easier to scale and maintain.
This technique is called prop drilling because you're passing state down from the parent level to the children.
As soon as you have multiple components, you'll need to drill through them to pass state to the children that need it.
Prop drilling can be multiple levels deep, but for this tutorial, we'll create a basic example just two levels deep.
The Switch component itself doesn't need active and toggle values, but we have to pass those values to the children components Message and Button.
Managing State with Hooks
Managing State with Hooks is a fundamental aspect of building dynamic and interactive applications with Next.js.
You can use the useState Hook to manage state in Next.js, which is a popular method for managing state in traditional React applications. It works similarly in Next.js.
The useState Hook returns an array with exactly two items: the current state of this state variable, initially set to the initial state you provided, and the set function that lets you change it to any other value in response to interaction.
To update what's on the screen, call the set function with some next state: React will store the next state, render your component again with the new values, and update the UI.
Here are the steps to use the useState Hook:
1. Call useState at the top level of your component to declare one or more state variables.
2. The useState Hook returns an array with exactly two items: the current state of this state variable, initially set to the initial state you provided, and the set function that lets you change it to any other value in response to interaction.
Expand your knowledge: Next Js Call Api on the Server Example
3. To update what's on the screen, call the set function with some next state: React will store the next state, render your component again with the new values, and update the UI.
The convention is to name state variables like [something, setSomething] using array destructuring.
You can use state to store and increment the number of times a user has clicked the "Like" button. In fact, the React hook used to manage state is called: useState()
You can also add the initial value of your likes state to 0. Then, you can check the initial state is working by using the state variable inside your component.
For another approach, see: Update Nextjs Version
Sources
- https://blog.logrocket.com/guide-state-management-next-js/
- https://www.thirdrocktechkno.com/blog/the-way-of-managing-the-state-for-next-js
- https://peterkellner.net/2023/05/07/Migrating-from-useState-to-useReducer-Building-a-Counter-in-a-NextJS-App/
- https://react.dev/reference/react/useState
- https://nextjs.org/learn/react-foundations/updating-state
Featured Images: pexels.com