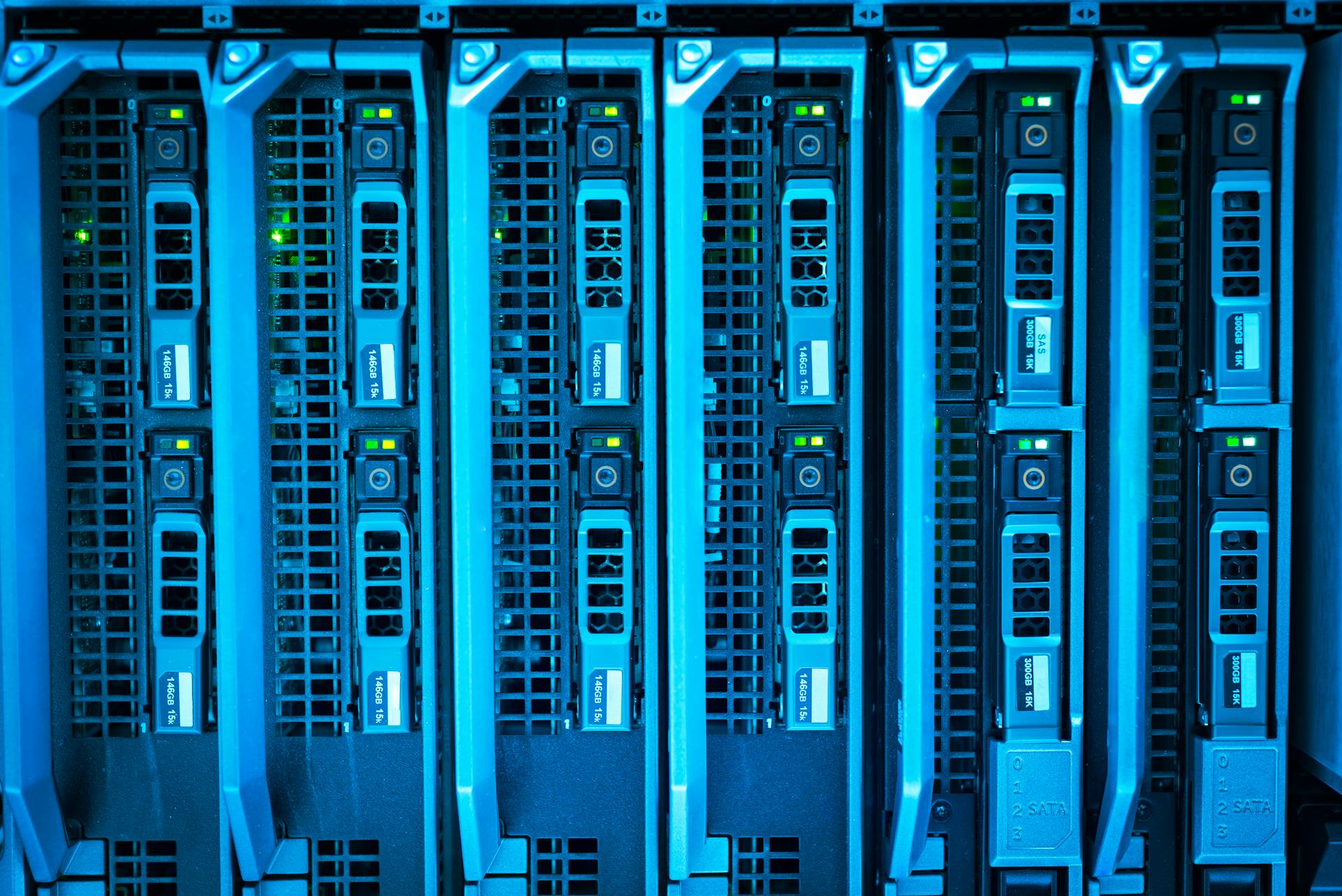
Nextjs allows you to use multiple middleware functions to handle requests and responses.
You can use the `next.config.js` file to configure multiple middlewares.
To add multiple middlewares, you need to import them in the `next.config.js` file and then use the `middleware` option.
Nextjs provides built-in support for popular middleware libraries like `helmet` and `compression`.
Understanding NextJS
Next.js is a powerful framework that lets you build server-side rendered (SSR) and statically generated websites and applications. It's known for its flexibility and scalability.
Next.js middleware acts as a gatekeeper for your application, intercepting incoming requests and allowing you to modify them, return a response, or pass the request along to the next middleware in the chain. This flexibility is a game-changer for developers who want to implement authentication checks, rate limiting, and more within the Next.js server environment.
Configuring multiple middlewares in Next.js requires a bit more setup compared to Express or Koa. However, with a few clever configurations, you can effectively manage multiple middleware functions, each tailored to specific parts of your application.
Next.js traditionally limits you to a single middleware file, but you can leverage the userAgent helper from next/server for user agent parsing. This allows you to parse user agent information and make informed decisions about how to handle requests.
Error Handling
Error Handling is a crucial aspect of working with multiple middlewares in Next.js. Middleware functions can no longer send response bodies directly.
To handle errors and edge cases, you should use the NextResponse object to rewrite or redirect to specific pages that display the appropriate message. This ensures a smooth user experience, even when things don't go as planned.
Handling an authorization error, for instance, involves redirecting to a custom error page. By doing so, you can display a suitable message to the user.
Real-World Applications
Configuring NextJS multiple middlewares opens up a plethora of possibilities for enhancing your Next.js projects.
Middleware in the context of Next.js executes during the server-side rendering phase, dealing with incoming requests before they reach your React components. This functionality enables developers to customize the handling of each request on the fly.
Multiple middlewares can significantly impact your Next.js projects by allowing you to handle requests in a more customized way. This can lead to faster and more secure web applications.
Next.js middleware refers to functions that execute during the server-side rendering phase, and it's the key to faster and more secure web applications.
Authentication and Authorization
Authentication and Authorization is a crucial aspect of any web application, and Next.js makes it easy to manage with multiple middlewares.
One of the most common use cases for middleware is to manage authentication and authorization.
By setting up a dedicated authentication middleware, you can verify user credentials and manage session tokens.
Authentication middleware can be used to check for a valid authorization token in the request headers.
This ensures that only authenticated users can access protected routes, like the login page.
A simplified example of how you might set up an authentication middleware is to redirect unauthenticated users to the login page.
This approach helps to manage session tokens and verify user credentials before a request reaches your API routes or specific pages.
API Routes and Static Files
Multiple middlewares can be incredibly valuable for managing API routes and serving static files.
Middleware can preprocess requests, perform validations, or log request data for analytics when it comes to API routes.
For static files, middleware can help with setting custom cache headers or redirecting requests based on the requested file type or path.
This middleware checks if the request is for a path that starts with /static and sets long-term cache headers on the response, optimizing the delivery of static assets.
By using multiple middlewares, you can streamline your development process and improve the performance of your Next.js application.
Custom cache headers can be set for static assets using middleware, which can significantly reduce the load on your server and improve page load times.
Conclusion
Configuring NextJS multiple middlewares can significantly enhance the functionality, security, and performance of your applications.
By following the principle of keeping each middleware focused on a single responsibility, developers can implement complex logic such as authentication and rate limiting with ease.
Carefully managing the order of execution is crucial to ensure that each request is processed as intended.
Remember, the key to effectively using multiple middlewares is to keep each one focused on a single task, making it easier to implement complex logic and improve your application's architecture.
Multiple Middlewares
Multiple middlewares are a powerful feature in Next.js, allowing you to create complex logic for handling requests. You can write multiple middlewares to achieve different goals, such as checking the database's readiness.
One thing to be aware of is that the order of middlewares matters. If a middleware tries to access a property that's only available after another middleware, it will fail. For example, the `withCheckDb` middleware needs to be inside `withDatabase` because it tries to access `req.db`, which is only available after `withDatabase`.
You can use the `next` object's request and response properties to define routing and path matching for each middleware, giving you fine-grained control over which middleware runs on different paths. This is especially useful for managing API routes and serving static files.
Routing and Path Matching
Routing and Path Matching is a crucial aspect of configuring multiple middlewares in Next.js. You can use the next object's request and response properties to define routing and path matching for each middleware.
The URLPattern API is a powerful tool for matching specific paths. With it, you can manipulate the request and response objects based on the URL path.
To tailor the behavior of your application precisely, you can use the URLPattern API to match specific paths. This approach gives you fine-grained control over which middleware runs on different paths.
In Next.js, the middleware function allows you to manipulate the request and response objects based on the URL path. This is particularly useful for defining routing and path matching for each middleware.
You can use the URLPattern API to match specific paths, allowing you to tailor the behavior of your application precisely. This approach is especially useful when dealing with multiple middlewares in Next.js.
Possible Solutions
If you're unsure of which approach to use for multiple middlewares, I recommend considering Next.js's built-in functionality. You can use the next object's request and response properties to define routing and path matching for each middleware, giving you fine-grained control over which middleware runs on different paths.
Next.js middleware operates at an essential layer within the framework's architecture, configured to run after the HTTP server has parsed the incoming request but before it reaches the React application logic. This allows middleware to act on the request, modifying headers, query parameters, the request path, and other aspects of the request or to decide on the control flow.
One possible solution is to use the URLPattern API to match specific paths, allowing you to tailor the behavior of your application precisely. For example, you can use the URLPattern API to match specific paths and apply middleware accordingly.
Alternatively, you can use next-connect, which allows you to use Express middleware syntax like you used to with Express.js. With next-connect, you can replicate the use/withDatabase and use/withCheckDb functions, taking into account the differences in syntax and behavior.
Here's a comparison of the two approaches:
Project Setup
To set up your Next.js project for multiple middlewares, start by creating a new file named middleware.js at the root of your project or inside the pages directory for page-specific middleware. This is where you'll define your middleware functions.
Ensure you're working in a Next.js environment, and if you've just set up your project, you can create the middleware.js file immediately. This basic setup allows you to begin using middleware in your Next.js applications.
Install Additional Dependencies
To effectively incorporate middleware into your Next.js application, you'll need to install additional dependencies depending on your requirements.
You'll likely need to install libraries to handle cookies or sessions within your middleware, especially in scenarios involving user authentication and session management.
Next.js supports API routes and middleware out of the box, but installing additional libraries is necessary for certain use cases.
These packages are not mandatory for all middleware use cases, but they're common in scenarios involving user authentication and session management.
You can install packages like those used for handling cookies or sessions to enhance your middleware functionality.
Setting Up
To set up your project for Next.js middleware, start by creating a new file named middleware.js at the root of your project or inside the pages directory for page-specific middleware. This is where you'll define your middleware functions.
You'll also need to ensure you're working in a Next.js environment, which means you've set up your project correctly from the start. This includes installing necessary dependencies and configuring a basic structure.
Create a middleware folder in your project's root folder to keep your middleware files organized and scalable. Inside this folder, create a config.ts file that will serve as the heart of your middleware configuration.
In this config.ts file, you can define and export multiple middleware functions, allowing you to maintain a clean separation of concerns and make your middleware easier to test and debug.
To begin using middleware in your Next.js applications, set up your project correctly from the start by following a step-by-step guide on initial setup, installing necessary dependencies, and configuring a basic structure.
Sources
- https://nextjs.org/docs/pages/building-your-application/routing/middleware
- https://www.dhiwise.com/post/how-to-configure-nextjs-multiple-middlewares
- https://hoangvvo.com/blog/nextjs-middleware
- https://loadforge.com/guides/nextjs-middleware-key-to-faster-web-applications
- https://clerk.com/docs/references/nextjs/clerk-middleware
Featured Images: pexels.com