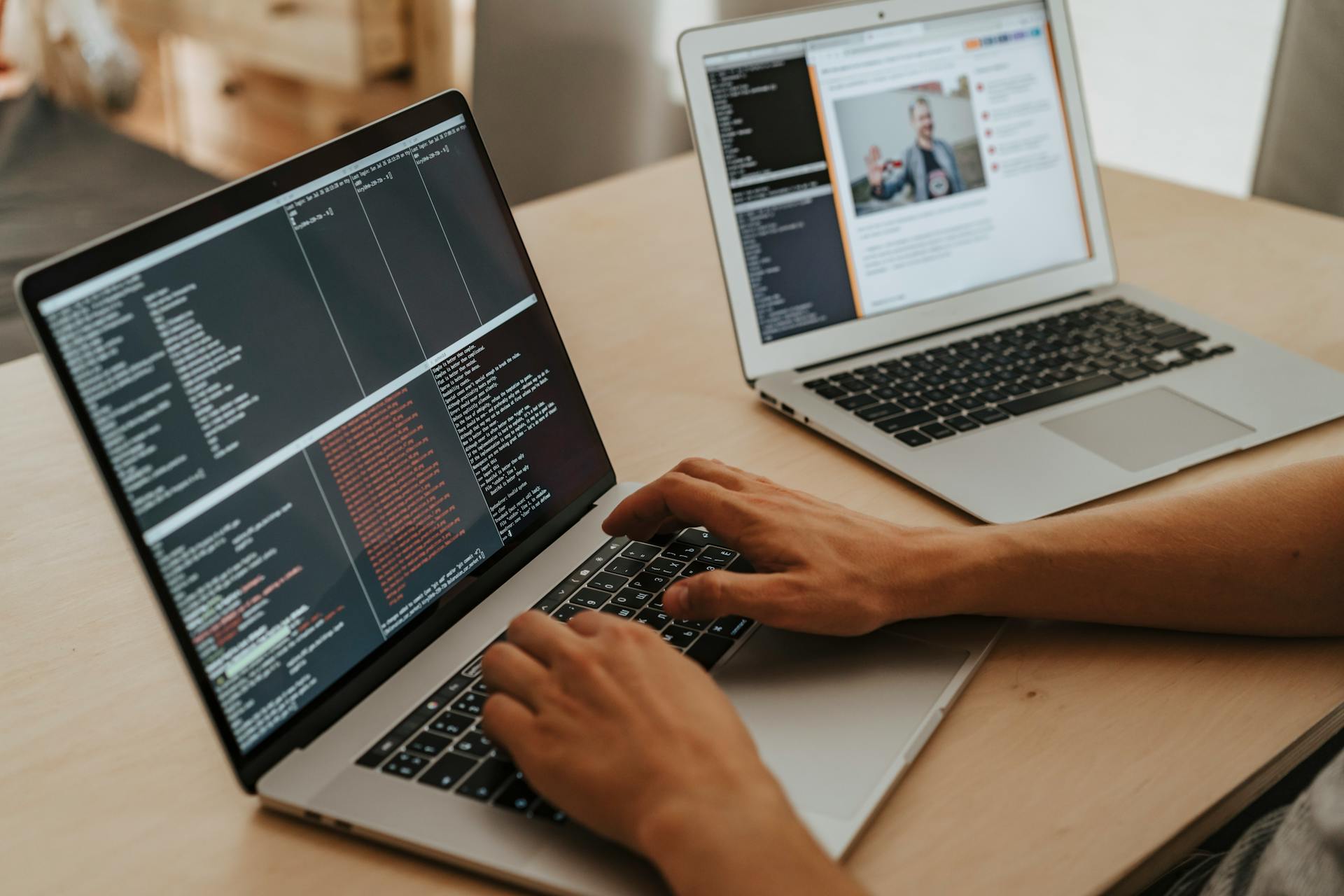
The Nextjs Middleware Matcher is a powerful tool that allows you to unlock advanced request control in your Nextjs applications.
With the Middleware Matcher, you can now match incoming requests against a set of rules, enabling you to execute specific middleware functions based on the request's properties.
This means you can create more tailored and efficient middleware functions that cater to specific use cases.
By leveraging the Middleware Matcher, you can create a more customized and optimized experience for your users.
URL Pattern Matching
URL Pattern Matching is a powerful feature in Next.js middleware that allows you to match URLs or parts of URLs against a template. It's based on the syntax from the path-to-regexp library, similar to routing systems in popular frameworks like Express or Ruby on Rails.
You can implement URL pattern matching in Next.js middleware to enhance modularity, improve code organization, increase flexibility, and potentially improve performance. By executing only relevant code for each route, you can make your Next.js application more efficient.
You might like: Next.js
To specify which routes your middleware should apply to, you can use a matcher. A matcher can be a string or a regular expression that matches the route pattern. For example, you can use `/about` to match the `/about` route, or `/blog/:slug` to match any route that starts with `/blog/` followed by a slug.
Here are some examples of matchers:
- /about: Matches the /about route.
- /blog/:slug: Matches any route that starts with /blog/ followed by a slug.
- /api/*: Matches any route that starts with /api/.
- /([a-zA-Z0-9-_]+): Matches any route that consists of alphanumeric characters, hyphens, and underscores.
You can also specify multiple matchers by using an array of strings or regular expressions. Middleware will be invoked for any route that matches any of the specified matchers. To do this, simply declare the matchers in an array format. For example, you can declare a matcher that always applies to the `/about` and `/dashboard` paths by using the following code:
```
const matcher = ['/about', '/dashboard'];
```
This matcher can be used to specify which routes your middleware should apply to, allowing you to keep your middleware clean and easy to understand.
Implementation Details
The nextjs middleware matcher is a powerful tool for managing routes in your Next.js application. It leverages the web standard URL Pattern API to define different patterns and their corresponding handlers.
The matcher function returns an array of pattern objects, each containing a URL pattern and its corresponding handler function. This allows for easy management of different Next.js routes.
To use the matcher, you need to define the routes where the middleware will apply. You can use the wildcard syntax to match a group of routes. For example, /api/users/:path* will match all routes starting with /api/users.
Here are some key points to keep in mind when working with the matcher:
- The matcher function is executed before the route's logic is reached.
- The project can have only one middleware file at the same level as the "app" directory.
- If your Next.js project has the "src" folder, the middleware file location is "src/middleware.ts"; otherwise, it is "./middleware.ts".
- Inside the next.js middleware file, you must define two things: the routes where the middleware will apply and an action to execute before continuing the request execution.
The matcher supports the wildcard syntax, so you can match a group of routes. The syntax /api/users/:path* says: Apply the middleware on all the routes starting with /api/users. It will match the routes like /api/users/123, /api/users/profile, etc...
Here are some examples of how to use the matcher:
The NextResponse object is central to what you can do within your middleware. You can return a specific response, modify the response, or rewrite the request path using this object.
Example Use Cases
Next.js middleware matcher is a powerful tool that allows you to handle common scenarios in a centralized way.
You can use Next.js middleware matcher to perform specific checks and redirects based on user status and requested paths, as seen in example handlers for common Next.js scenarios.
With Next.js middleware, you can do authorization checks in one place, which works for all routes, eliminating the need to add checks on each route individually.
Using a library like Next Auth can be cumbersome, as you must add authorization checks on each route.
Next.js middleware matcher can handle cases where you need to generate tokens and pass the plain user role to the request header "Authorization".
For another approach, see: How to Use Google Fonts in Nextjs
Next.js Middleware
You can use Next.js middleware to rewrite URLs, as seen in the example where `/dashboard` is rewritten to `/dashboard/1`. This is useful when you have a dashboard with all empty data except for the first item.
Next.js middleware can be used to change the URL of a page without affecting its content. In the example, the URL `/dashboard` is changed to `/dashboard/1` without exposing the resource number to the user.
The middleware function uses NextResponse to rewrite the URL. This is a powerful feature that allows you to manipulate the URL of a page without affecting its content.
In the example, the `/dashboard` page is rendered as if it's a page with the URL `/dashboard/1`, but the actual URL is still `/dashboard`. This is achieved by using a middleware function to rewrite the URL.
The app/dashboard/[slug]/page.tsx file is used to render the dashboard page, and the middleware function is used to rewrite the URL to `/dashboard/1`. This is a great way to handle cases where the dashboard has all empty data except for the first item.
Consider reading: Next Js 14 Redirect to Another Page Loading Indicator
Sources
- https://makerkit.dev/blog/tutorials/nextjs-middleware-url-patterns
- https://velog.io/@jay/Next.js-13-master-course-middleware
- https://supabase.com/docs/guides/auth/server-side/nextjs
- https://codeparrot.ai/blogs/nextjs-middleware-simple-guide-to-control-requests
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
Featured Images: pexels.com