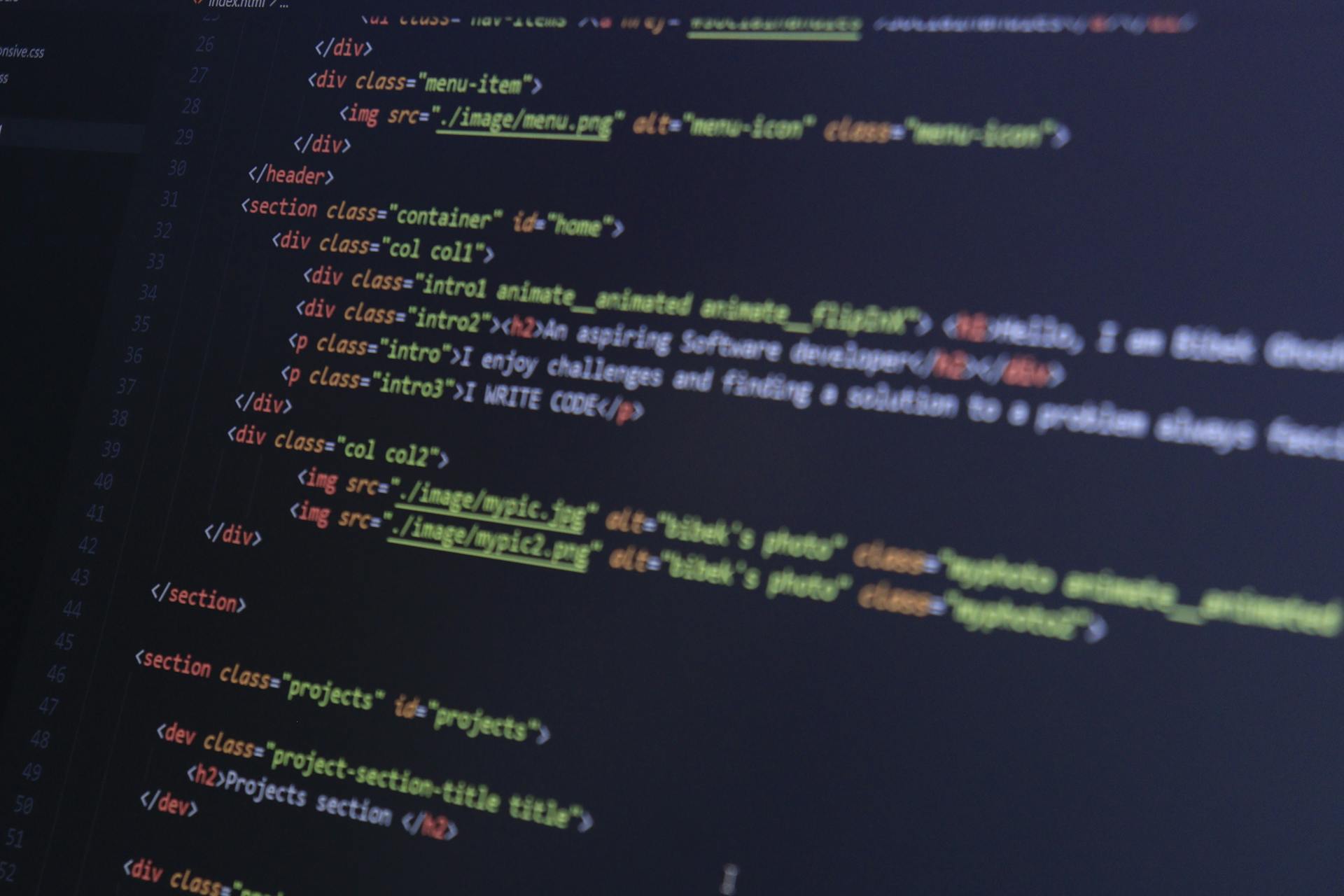
Adding a custom font to Next JS can greatly enhance the user experience of your website. This is because custom fonts can improve readability and make your content stand out.
To get started, you'll need to install the next-font-loader package. This package allows you to easily integrate custom fonts into your Next JS project.
Next, you'll need to create a fonts directory in the root of your project. This is where you'll store your custom font files.
Optimization Benefits
Adding a custom font to your Next.js app can be a game-changer for your website's look and feel. With next/font, you get automatic font optimization, which eliminates the need to manually set up font delivery.
Automatic font optimization is key to a seamless user experience. By default, Next.js will optimize web fonts, including custom fonts, eliminating the need for manual setup.
No external requests mean improved privacy and performance. next/font completely removes external network requests, which is a big win for your website's speed and security.
Built-in automatic self-hosting is another perk. CSS and font files are downloaded at build time and automatically self-hosted with the rest of your static assets.
Automatic matching fallback is a clever feature. next/font provides a custom fallback that closely matches your font and calculates things like the size-adjust property.
Here are some of the key benefits of using next/font for font optimization:
- Automatic font optimization: No manual setup required.
- No external requests: Improved privacy and performance.
- Built-in automatic self-hosting: Self-hosted with static assets.
- Automatic matching fallback: Custom fallback with size-adjust property.
- Preloading strategy: Critical fonts load quicker.
- Automatic font subsetting: Google fonts are automatically subset.
Automatic font subsetting is also a plus. With next/font, Google fonts are automatically subset, reducing the size of font files and ensuring quicker font loading times.
Custom Font Configuration
Custom Font Configuration is a crucial step in adding custom fonts to your Next.js app. You can use next/font to load custom fonts from Google Fonts or locally.
To load fonts from Google Fonts, you can use the next/font/google module, which allows you to import fonts from Google Fonts and load them into your app. For example, you can import the Open Sans and Roboto Mono fonts from next/font/google and load them with the variable option to define the CSS variable name.
You can also load fonts locally by using the next/font/local module. This module allows you to load fonts from a local file and make them available to your app. To load a local font, you need to import the font loader from next/font/local and define a new instance where you specify the src of the local font files.
Once you have loaded your fonts, you can use them in your app by referencing the CSS variables in your HTML document. For example, you can use the --openSans variable to reference the Open Sans font in your HTML document.
To use the loaded fonts with Tailwind CSS, you need to add an entry to your Tailwind config to create a new font family that uses the loaded fonts. You can then use the font family in your Tailwind config to create utility classes that apply the loaded fonts to your app.
Here are the steps to add custom fonts to your Next.js app using next/font and Tailwind CSS:
- Import the font loader from next/font/google or next/font/local
- Load the fonts with the variable option to define the CSS variable name
- Attach the CSS variables to your HTML document
- Add an entry to your Tailwind config to create a new font family that uses the loaded fonts
- Use the font family in your Tailwind config to create utility classes that apply the loaded fonts to your app
By following these steps, you can easily add custom fonts to your Next.js app using next/font and Tailwind CSS.
Adding Custom Font to Next.js
Adding custom fonts to your Next.js site can be a bit tricky, but don't worry, I've got you covered. You can use the next/font/google package to load fonts from Google Fonts, which is a great option if you're already using Google Fonts.
To add a custom font to your Next.js site, you can use the next/font/local package, which allows you to load fonts from a local file. This is a good option if you have a custom font that you want to use on your site.
One way to add a custom font to your Next.js site is to use the next/font/local package and configure it to load the font from a local file. For example, you can add the following code to your pages/_app.js file to load a custom font:
You can also use the @font-face CSS property to import fonts from a local file. This is similar to the previous example, but instead of using the next/font/local package, you use the @font-face property to import the font.
To use a custom font in your Next.js site, you need to import the font loader from next/font/local and specify the source of the font. For example, you can add the following code to your pages/_app.js file to load a custom font:
If you want to import multiple files for a single font, you need to use an Array in your src. For example:
Once you have imported your font, you can use it globally in your _app.js file or locally within any page. To use the font locally, you need to import the Head component where you need your font:
Here's a summary of the steps to add a custom font to your Next.js site:
Remember to replace the "my-font" placeholder with the actual name of your font file.
Loading and Initializing
To load custom fonts from Google Fonts, you'll need to import them in your _app.jsx/tsx file using next/font/google. This will allow you to generate a definition for a CSS --var for each font.
Make sure to use the variable configuration prop to pass the generated variables into the wrapper class, so that they're available to any child of that element in the DOM.
You can also load custom fonts from a local file using next/font/local, which allows you to map font weights to different files as needed.
To generate CSS variables for each font, you'll need to add the resulting CSS classes to the root of your Next.js app, making these variables available to any child of the App component.
This process is the same regardless of whether you're using Google Fonts or loading font files from your local repository.
Using Custom Font in Next.js
Using custom fonts in Next.js is a breeze with the right tools. You can use the next/font/google package to load fonts from Google Fonts, which is a great option if you're already using Google Fonts.
The process is slightly different for loading font files that are stored locally in your repo, but the basic steps are the same. You can import the font as a function from next/fonts/google and then use it globally in your _app.js file.
To use custom fonts in Next.js with next/font/local, you need to import the function and specify the source of your font. You can put the font files anywhere you want, just make sure to change the src and font name accordingly.
Using @font-face in your CSS files is another option, where you download the font files into your project and then use @import. This method is similar to using a CDN, but it's a good idea to use a local font file for performance reasons.
The next/font package provides an easy way to load fonts in your Next.js app, while maintaining performance and minimizing Cumulative Layout Shift (CLS). It uses the Font Loading API to asynchronously load local font files stored in your repo, or from popular font providers.
Here are the three steps to use next/font to apply a Google font globally:
- Import the font
- Configure the font object
- Attach the font to the JSX code
You can do all three of these things in your layout.js file.
Custom Font in Next.js Pages
To add a custom font to your Next.js pages, you can use the next/font/local package, which allows you to load fonts from a local file. This method is similar to using a CDN, but you download the font files into your project and then use @import.
You can download the font from Google Fonts and unpack it within your project, such as in the /assets/fontName folder. To use the font, you need to import it in your CSS file using the @font-face CSS property. For example, if you have a font file named "my-font.woff2" in your /assets/fontName folder, you can use the following code in your CSS file:
@font-face {
font-family: 'My Font';
src: url('/assets/fontName/my-font.woff2');
}
Alternatively, you can use the next/font/local function to import the font in your JavaScript file. This function allows you to load fonts from a local file and returns an object that you can use to apply the font style.
Here's an example of how you can use the next/font/local function to import a font:
```html
import { Font } from 'next/font/local';
const myFont = Font({
src: '/assets/fontName/my-font.woff2',
fontDisplay: 'swap',
});
// Use the font in your CSS file
.my-font {
font-family: 'My Font', sans-serif;
font-size: 16px;
}
```
Head Component with CDN
The Head Component with CDN is a great way to add Google fonts to your Next.js pages. You can use the Next.js head component to add the font links from Google Fonts.
To do this, you need to import the Head component in Next.js. Then, you can paste the copied links from Google Fonts within the Head component. This will add the font links to the HTML head of your page.
Here are the steps to follow:
1. Import the Head component in Next.js.
2. Paste the copied links from Google Fonts within the Head component.
3. Make sure to change the component name to your desired name.
4. Note that the third link's href can be different, depending on your font choice.
By following these steps, you can easily add Google fonts to your Next.js pages using the Head component with CDN.
Use in Next
In Next.js, you can use custom fonts in various ways. You can use the next/font package to load fonts from Google Fonts or local files, or you can use the Tailwind CSS framework to style your fonts.
To use next/font with the Pages Router, you can follow a step-by-step tutorial that walks you through several approaches to working with the next/font package using the Pages Router. This tutorial is available on the official Next.js documentation.
You can also use next/font to apply a font to a specific component. To do this, you need to add the font as a variable, as shown in the Tailwind CSS section, and then use the font instance to apply the font style.
Another way to add custom fonts in Next.js is by using the next/font/local package. This package allows you to use custom fonts or any other fonts that are not available on Google Fonts. You can import the font as a function in your file, specify the source of your font, and then use it globally in your _app.js file or locally within any page.
You can also download files locally and then use @font-face in your CSS files. This method is similar to the previous example, but instead of using a CDN, you download the font files into your project and then use @import.
Here are some examples of how to use next/font with Tailwind CSS:
You can use these methods to add custom fonts to your Next.js app using next/font and Tailwind CSS. This will improve user experience without sacrificing performance.
Frequently Asked Questions
How to use multiple fonts in Next JS 14?
To use multiple fonts in Next JS 14, import fonts from a font library and configure them with subsets, CSS variables, and weights for seamless global styling. This allows you to easily switch between fonts in your application's global styles.
Sources
- https://prismic.io/blog/nextjs-fonts
- https://mikebifulco.com/posts/custom-fonts-with-next-font-and-tailwind
- https://shipsaas.com/blog/how-to-add-font-in-nextjs
- https://medium.com/frontendweb/how-to-add-font-in-next-js-7a7fba80d528
- https://blog.logrocket.com/next-js-font-optimization-custom-google-fonts/
Featured Images: pexels.com