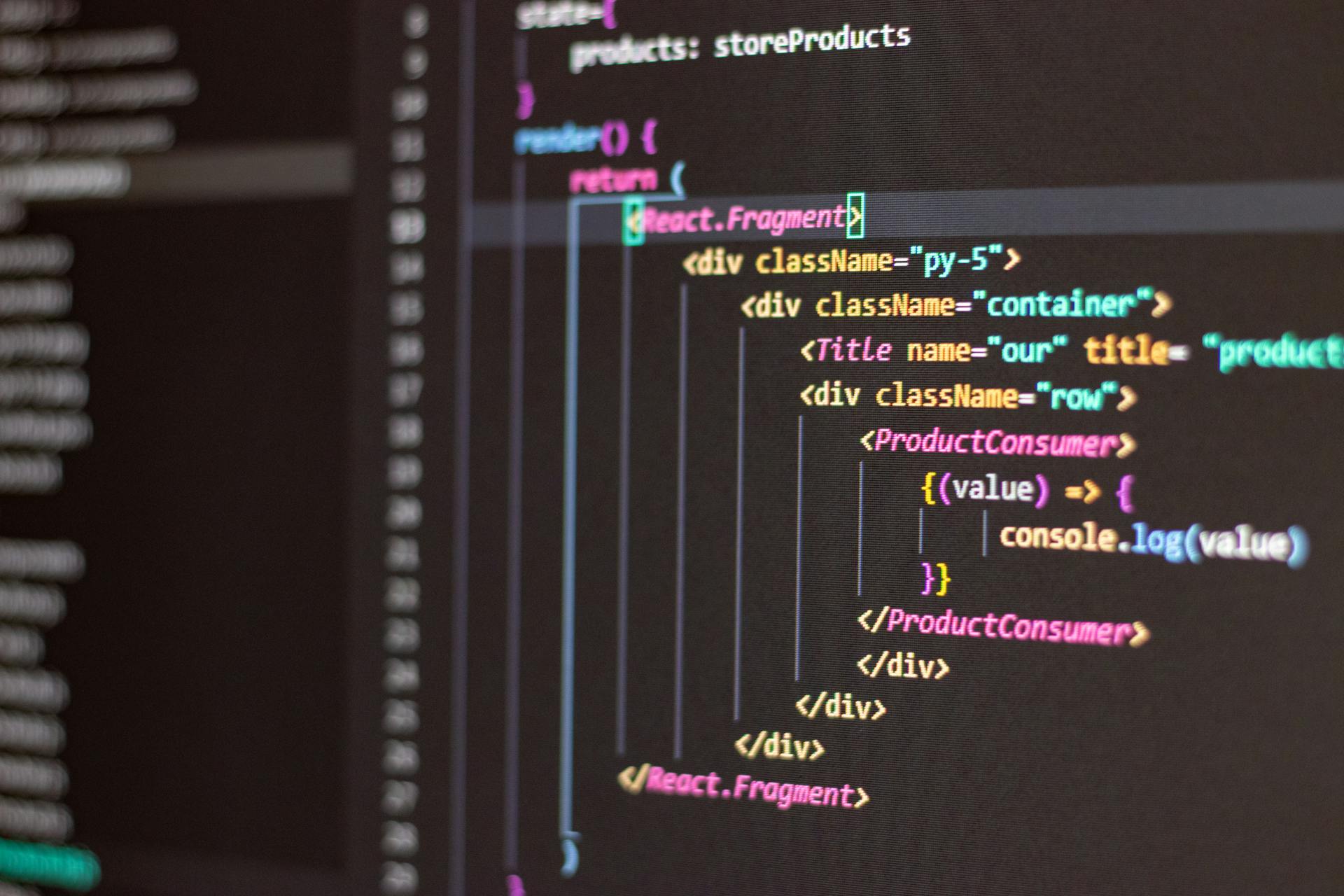
Next.js provides a hook called `useEffect` that allows you to run some side-effecting code when a component mounts or updates.
`useEffect` is a powerful tool in Next.js, but it can also be a performance bottleneck if not used carefully.
To use `useEffect` effectively, you need to understand how it works and how to optimize it for performance.
`useEffect` runs after every render, including the initial render, which can lead to unnecessary re-renders and slow down your application.
If this caught your attention, see: Deploy Nextjs on Render
Next.js Use Effect Basics
In Next.js, useEffect is a powerful Hook that helps manage side effects in functional components. It's a game-changer for handling updates, unlike class components that require special code for handling updates in the componentDidUpdate method.
When using useEffect, you don't need to add special code to handle updates because it cleans up the previous effects before applying the next effects. This ensures consistency and prevents bugs that are common in class components.
If this caught your attention, see: Next Js Component
For example, consider a FriendStatus component that displays whether a friend is online or not. If the friend prop changes while the component is on the screen, a class component would continue displaying the online status of a different friend, causing a bug. But a Next.js component using Hooks doesn't suffer from this bug, thanks to useEffect's default behavior.
Intriguing read: Nextjs Ui Library
No Dependencies
In Next.js, you might not see the option to have no dependencies in your useEffect hook, but it's a valid use case.
This can happen when your useEffect hook is synchronized with external state, or when you simply want it to run once.
You can use no dependencies when you have a component that fetches data from an API, like our imaginary social media example.
In this case, we take in the user as a prop and fetch the friends list for our user in the component.
Every time the user changes and the component re-renders, we re-fetch the list of users.
This can be a problem if the effect function is doing something expensive or time-consuming, like a complex API request.
In such cases, it's essential to include a dependency array to ensure the effect function is not executed unnecessarily.
It's also worth noting that it's easier to just place your logic inside the body of your component, which will run every render.
Intriguing read: Next Js Function Handlers to Filter Data
Effects Without Cleanup
Sometimes we want to run some additional code after React has updated the DOM. Network requests are common examples of effects that don’t require a cleanup.
We can run them and immediately forget about them, making them easy to manage.
You might like: Run Nextjs on Bluehost
Effects Run Explanation
Effects in Next.js run on every update because it ensures consistency by default and prevents bugs that are common in class components due to missing update logic.
The effect cleanup phase happens after every re-render because it helps us create components with fewer bugs. This design is especially helpful when dealing with components that use external data sources.
Related reading: Next Js Components Folder
If a component's props change while it's on the screen, the effect cleanup phase ensures that the previous effects are cleaned up before applying the next effects. This prevents bugs like displaying the online status of a different friend.
In a class component, we would need to add componentDidUpdate to handle this case, but forgetting to do so is a common source of bugs in React applications.
The version of the FriendStatus component that uses Hooks doesn't suffer from this bug because useEffect handles updates by default. It cleans up the previous effects before applying the next effects.
This behavior ensures consistency and prevents bugs that are common in class components. To illustrate this, consider the sequence of subscribe and unsubscribe calls that the FriendStatus component could produce over time.
Consider reading: Nextjs Pathname Server Component
Using a PostHog Hook
Using a PostHog hook can be a good idea when you want to initialize PostHog when the app is loaded. This is because it lets you specify side effects that are caused by rendering itself, rather than by a particular event.
You can technically use a window object check to initialize PostHog, but this can cause a hydration and/or mismatch error. This is because it happens outside the React lifecycle, meaning it happens earlier and can lead to issues like Warning: Prop dangerouslySetInnerHTML did not match.
The React docs recommend using a useEffect hook for this purpose, making it a better choice for initializing PostHog.
For another approach, see: React Next Js
Why Does the Pageview Component Need a Hook?
The pageview component needs a useEffect because not using one might lead to duplicate page views being tracked if the component re-renders for reasons other than navigation.
This can happen when the component is re-rendered due to a change in props or state, but not because of a navigation event. If you don't use a useEffect, you might end up tracking the same page view multiple times.
Here are some reasons why using a useEffect is a better approach:
- Not using a useEffect hook, but this might lead to duplicate page views being tracked if the component re-renders for reasons other than navigation.
- Using window.navigation to track pageviews, but this approach is more complex and is not supported in all browsers.
In fact, using a useEffect is a simpler and more reliable way to track page views, making it a better choice for most use cases.
Basics of Next.js
Next.js is a powerful framework built on top of React that simplifies and improves the building of modern front-end apps.
It combines the best features of server-side rendering (SSR) and client-side rendering (CSR), offering developers flexibility and performance optimization.
Use Effect with Cleanup
Effects with cleanup are essential in Next.js to prevent side effects and memory leaks. You can use the cleanup function to clean up any resources or effects created by the hook.
The cleanup function is executed when the component is unmounted, before the new effect is applied. This means you can use it to cancel any ongoing network requests, unsubscribe from event listeners, or perform any other necessary cleanup.
To use the cleanup function, simply return a function from the effect callback. This function will be called when the component unmounts, allowing you to clean up any resources that were created during the effect.
Expand your knowledge: Next Js Loading
Empty Array
Using an empty array as the second argument to useEffect can be a useful pattern, especially when fetching data that doesn't depend on any parameters.
This approach only runs the effect on the first render, which is perfect for data that doesn't change over time. In the case of data fetching, you can use this pattern to start the data fetching when your component first renders.
For example, if you're fetching data that doesn't depend on any state or events, you can simply pass an empty array as the second argument to useEffect.
Using an empty array as the second argument is closer to the familiar componentDidMount and componentWillUnmount mental model, but it's not always the best solution to avoid re-running effects too often.
You can also use an empty array when you want to run an effect and clean it up only once, on mount and unmount. This is useful when you have an effect that doesn't depend on any values from props or state.
Additional reading: Nextjs Usecontext
Effects with Cleanup
Effects can cause side effects and memory leaks if not used properly, but the cleanup function can help prevent this.
The cleanup function is a way to clean up any resources or effects created by the hook, and it's executed when the component is unmounted.
You can use the cleanup function to cancel ongoing network requests, unsubscribe from event listeners, or perform any other necessary cleanup.
To use the cleanup function, simply return a function from the effect callback, which will be called when the component unmounts.
This allows you to keep the logic for adding and removing subscriptions close to each other, just like in the example with the ChatAPI module.
React will run the cleanup function when the component unmounts, but it will also clean up effects from the previous render before running the effects next time.
This helps avoid bugs and ensures consistency by default, preventing issues that are common in class components due to missing update logic.
You can return a named function, an arrow function, or call it something different from the effect, just like in the example where we called it "cleanup" to clarify its purpose.
By using the cleanup function, you can create components with fewer bugs and improve overall performance.
Readers also liked: How to Use Context Api in Next Js 14
Performance Optimization
In some cases, optimizing performance by skipping effects is crucial. You can solve this by writing an extra comparison with prevProps or prevState inside componentDidUpdate.
Optimizing performance by skipping effects is a common requirement. It's built into the useEffect Hook API, allowing you to skip applying an effect if certain values haven’t changed between re-renders.
Passing an array as an optional second argument to useEffect is the key to this optimization. This array should include all values from the component scope that change over time and are used by the effect.
If the array includes all necessary values, React will skip the effect when the values haven't changed. For example, if you pass [count] as the second argument, React will skip the effect if the count is still 5 after re-rendering.
However, if one of the values in the array has changed, React will re-apply the effect. This means that if you pass [count] and the count is updated to 6, React will re-run the effect because 5 !== 6.
This optimization also works for effects that have a cleanup phase. But be sure to include all necessary values in the array to avoid referencing stale values from previous renders.
Passing an empty array ([]) as a second argument tells React that your effect doesn’t depend on any values from props or state. This means the effect will only run once, on mount and unmount.
Using Swr to Fetch Data Efficiently
Next.js offers several methods for data fetching, but using SWR can make it more efficient. SWR is a great tool for managing data fetching in Next.js projects.
With SWR, you can ensure that the UI stays fast and responsive while new data is fetched. This is especially useful when dealing with dynamic updates without a full page reload.
One of the key benefits of using SWR is that it can help reduce the number of unnecessary API calls. By caching data and only refetching it when necessary, you can improve the performance of your application.
For your interest: Using State in Next Js
SWR can be used in conjunction with the useEffect hook from React to execute code after the component has rendered. This allows you to fetch data from an API endpoint using the fetch API or any other data fetching library.
By using SWR with Next.js, you can create a seamless and efficient data fetching experience for your users.
Here's an interesting read: Fetch Next Js
Overview
Next.js is a robust React framework that optimizes server-side rendering (SSR) and client-side rendering (CSR) capabilities. Its comprehensive toolkit for data fetching and server actions has made it the go-to choice for developers.
Next.js is built on top of React, which simplifies and improves the building of modern front-end apps. It combines the best features of SSR and CSR, offering developers flexibility and performance optimization.
Delivering flawless user experience and structured data retrieval is crucial in web development, and Next.js has become the top choice for achieving this goal. Next.js is designed to handle specific actions and data operations, making it an ideal choice for developers.
A unique perspective: Next Js Server Side Api Call
Server actions are an alpha feature in Next.js, enabling developers to build custom server endpoints that handle specific actions and data operations. This feature is a game-changer for developers who want to bridge the gap between the client and server seamlessly.
Here are the key topics we'll cover in this tutorial:
- Basics of Next.js
- Data Fetching in Next.js
- Server actions in Next.js
Frequently Asked Questions
How to use useEffect in SSR?
Use the `useEffect` hook with an empty dependency array to perform server-side data fetching, ensuring data is fetched before rendering the component
Does Next.js use useEffect?
Yes, Next.js supports the use of useEffect, but with specific considerations for server and client components. Learn more about the nuances of using useEffect in Next.js applications.
Sources
- https://upmostly.com/next-js/a-developers-guide-to-using-useeffect-in-next-js
- https://legacy.reactjs.org/docs/hooks-effect.html
- https://posthog.com/docs/libraries/next-js
- https://refine.dev/blog/next-js-server-actions-and-data-fetching/
- https://nextjs.org/docs/app/building-your-application/data-fetching/server-actions-and-mutations
Featured Images: pexels.com