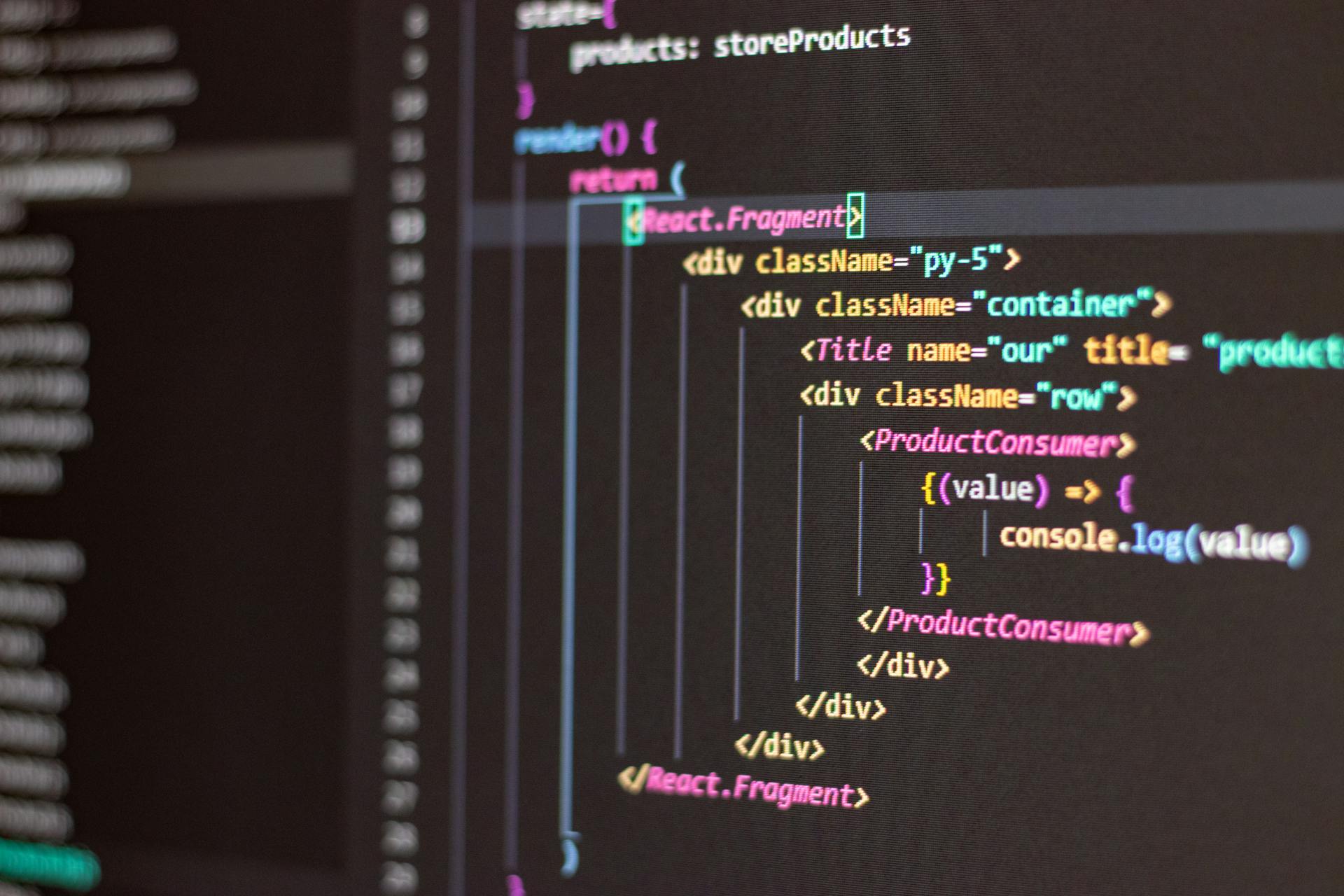
To use Context API in Next JS 14 for efficient state management, you'll need to create a context file. This file will serve as a centralized hub for your app's state.
Context API in Next JS 14 allows you to manage global state in a predictable and easy-to-debug way. By using the createContext function, you can create a context that can be used throughout your app.
For instance, in the example from the article section, a context file is created to manage the app's theme. The context file is then wrapped around the app's components using the Provider component.
Broaden your view: Next Js Using State Context
API Setup Guide
To set up the Context API in Next.js 14, you need to create a new context in a file where you define its shape and initial value.
First, you'll create a provider component that wraps your application and provides the context to all components.
Next.js 14 makes it easy to wrap your application with the AuthProvider you just created, typically done in your _app.js file.
To consume the context in your functional components, you can use the useContext hook provided by React.
Creating a new context is a straightforward process that sets the stage for the rest of the setup.
Broaden your view: Next Js Fetch Data save in Context and Next Route
Creating and Consuming Context API
Creating and Consuming Context API is a crucial step in using Context API in Next.js 14. You can create a context by calling the createContext method, as seen in Example 2. This method returns a Context object that you can use to provide the context to your application.
The context object has a Provider property that you need to use to provide the context to your application. This is typically done in a new file where you can define the shape of the context and its initial value.
You can provide the context to your application by wrapping your main component with the Context.Provider component, as shown in Example 1. This allows all components inside the Context to access the context values.
To consume the context, you can use the useContext hook, as seen in Example 3. This hook looks for the nearest Context.Provider and gives you its value. You can use this hook in any functional component to access the context values.
Explore further: Next Js Loader
Here's a step-by-step guide to consuming the context:
1. Import the useContext hook.
2. Use the hook to get the context value.
Here's an example of how to consume the context:
```html
import { useContext } from 'react';
import { PostsContext } from './context.js';
function PostsList() {
const posts = useContext(PostsContext);
return (
{posts.map((post) => (
{post.title}
))}
);
}
```
Passing Data with Context API
Context API is a powerful tool for sharing data between React components. It's one of the state management tools bundled with the React.js library itself.
To share data between components, you can use the useContext Hooks to set a value. This is done by importing all modules and using the useContext Hooks to get a value.
When passing data deeply into the tree, useContext returns the context value for the context you passed. React searches the component tree and finds the closest context provider above for that particular context.
To pass context to a Button, wrap it or one of its parent components into the corresponding context provider. This way, the Button will receive the correct value.
Recommended read: Nextjs save Data Pulled from Api Using State Context
Here's a summary of the steps to pass context:
- Create a new context
- Create a provider component
- Wrap your application with the provider
- Consume the context in your functional components using the useContext hook
Note that to update context, combine it with state. Declare a state variable in the parent component, and pass the current state down as the context value to the provider.
Broaden your view: Using State in Next Js
Optimizing Context API Usage
Passing objects and functions via context can lead to unnecessary re-renders, especially when the underlying data hasn't changed. You can use the useMemo and useCallback hooks to optimize this.
When re-rendering, React will re-render all components deep in the tree that call useContext, even if the underlying data hasn't changed. This can be problematic in larger apps.
To help React take advantage of this fact, you can wrap the login function with useCallback and wrap the object creation into useMemo. This is a performance optimization that can make a big difference.
By using these hooks, you can ensure that components only re-render when the underlying data has changed, rather than on every re-render. This can improve the performance of your application and make it more efficient.
Here's a summary of the optimization techniques:
By applying these optimization techniques, you can make the most out of the Context API in Next.js 14 and create a more efficient and scalable application.
Managing Context API Behavior
You might have a provider without a value in the tree, which can cause your context to be undefined. This can happen if you forget to specify the value prop or use a different prop name by mistake.
React will warn you in the console if this is the case. To fix it, simply call the prop value to ensure it's being passed correctly.
Here are some common pitfalls to watch out for:
- Forgetting to specify the value prop in your provider
- Mistakingly using a different prop name
By being aware of these potential issues, you can ensure your context API behaves as expected and your app remains stable.
Native React Management
Native React Management is all about using React's built-in features to manage state and behavior in your application. The Context API is React's own solution for managing global state, allowing you to share values between components without prop drilling.
To use the Context API, you need to create a new context, define its shape and initial value, and then create a provider component that wraps your application and provides the context to all components. This is typically done in your _app.js file.
Suggestion: Next Js and React
The Context API is particularly useful for global state that needs to be accessed in many parts of your Next.js app. Here are some scenarios where global state is preferable:
- User authentication status that needs to be accessed across various parts of the app.
- Theme settings or UI preferences that affect the entire application.
- Data that is fetched and should be cached and shared, like a user's profile information.
To consume the context in your functional components, you can use the useContext hook provided by React. This hook allows you to access the context value from anywhere in your component tree.
In some cases, you might encounter issues with your component not seeing the value from your provider. This can happen if you're rendering the provider in the same component as where you're calling useContext(), or if you've forgotten to wrap your component with the provider. Be sure to check your component hierarchy using React DevTools to ensure everything is set up correctly.
For more insights, see: Next Js Usecontext
Overriding a Theme
You can override the context for a part of the tree by wrapping that part in a provider with a different value. This allows you to change the theme for specific components without affecting the rest of the application.
The Context API makes it easy to override a theme by creating a new provider with a different value. For example, in Example 3: Overriding a theme, the button inside the Footer receives a different context value ("light") than the buttons outside ("dark").
You can nest and override providers as many times as you need, giving you fine-grained control over the context values used by different parts of your application.
To override a theme, you'll need to create a new provider component that wraps the specific components you want to override. This provider should have a different value for the context you want to change.
Here's an example of how you might override a theme using the Context API:
In this example, the parent provider sets the theme to "dark", but the child provider overrides this value to "light". The grandchild provider then reverts back to the parent provider's value of "dark".
See what others are reading: Nextjs Dark Mode
Troubleshooting Context API Issues
So you're having trouble getting your component to see the value from the provider? First, check if you're rendering the provider in the same component as where you're calling useContext(). If so, move the provider above and outside the component calling useContext().
If that's not the issue, make sure you're wrapping your component with the provider, and that the hierarchy is correct using React DevTools.
There's also a chance you're running into a build issue with your tooling, which can cause the provider and consumer to see different objects. This can happen if you use symlinks, so try verifying this by assigning the provider and consumer to globals like window.SomeContext1 and window.SomeContext2, and then checking if they're the same in the console.
Here are the common causes of this issue in a nutshell:
- Rendering the provider in the same component as the consumer
- Forgetting to wrap the component with the provider
- Build issue with symlinks
Best Practices for Context API
To create a new context, you need to define its shape and initial value in a new file. This is the first step in setting up the Context API in Next.js.
Create a provider component that will wrap your application and provide the context to all components within your app. This is crucial for making the context available to your components.
Wrap your application with the provider component in your _app.js file. This is typically done after creating the provider component.
To consume the context in your functional components, use the useContext hook provided by React. This hook allows you to access the context from any component within your app.
By following these best practices, you can effectively use the Context API in Next.js to manage state and props across your application.
A different take: Next Js Useeffect
Sources
- https://episyche.com/blog/how-to-use-context-api-in-a-nextjs-app
- https://egghead.io/lessons/next-js-make-user-state-globally-accessible-in-next-js-with-react-context-and-providers
- https://react.dev/reference/react/useContext
- https://wanago.io/2020/09/28/react-context-api-hooks-typescript/
- https://www.dhiwise.com/post/nextjs-global-state-creating-and-managing-state
Featured Images: pexels.com