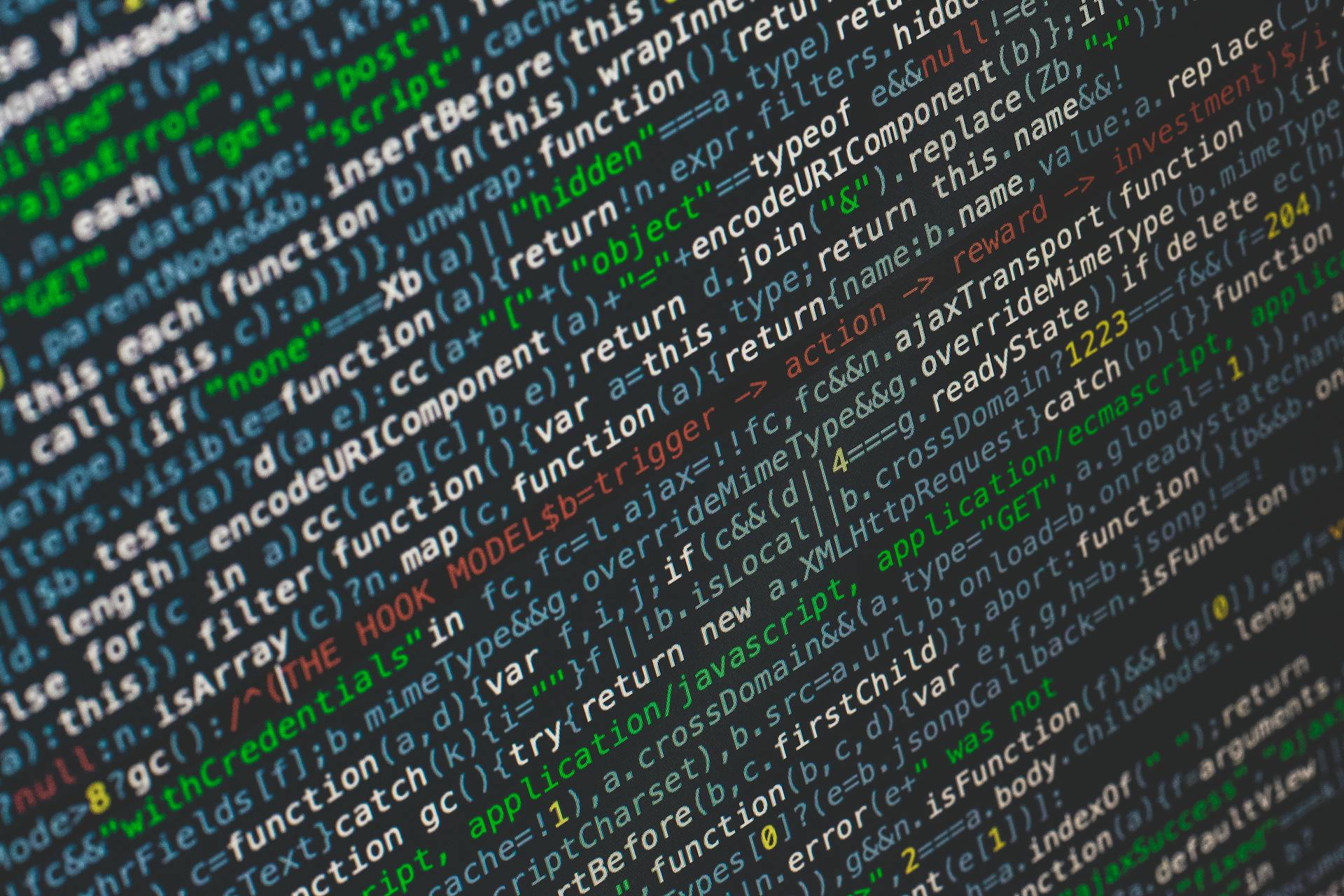
In Next.js, you can save data pulled from an API using state context for dynamic content. This approach allows you to create dynamic pages that update in real-time.
To achieve this, you'll need to create a context object that will hold the state of your data. This context object will be used to update the component tree, making it dynamic.
By using state context, you can easily manage data changes and re-render the component tree accordingly. This is particularly useful when dealing with data that changes frequently, such as user authentication or real-time updates.
In Next.js, you can use the `useState` hook to create a state context. This hook allows you to create a state variable and an update function that can be used to update the state.
Consider reading: Next Js Fetch Data save in Context and Next Route
Setting Up Next.js
To get started with Next.js, you'll need to install it using npm or yarn.
Create a new project by running `npx create-next-app my-app` in your terminal. This will set up a basic Next.js project for you.
In the project directory, you'll find a file called `pages/index.js` which is the entry point for your application.
Additional reading: Save Api Data on Local Storage Next Js
Creating a Next.js Project
To create a Next.js project, you'll need to run the command `npx create-next-app my-app` in your terminal.
This command will scaffold a new Next.js project with a basic directory structure.
Next.js projects have a `pages` directory where you'll create individual pages for your application.
The `pages` directory is where you'll define routes for your application.
Each page in the `pages` directory is a separate JavaScript file that exports a React component.
The `index.js` file in the `pages` directory is the entry point for your application.
The `next.config.js` file is where you'll configure your Next.js application.
In the `next.config.js` file, you can customize settings such as the output directory and the development server.
Discover more: Nextjs App Route Get Ssr Data
Configuring API Routes
To configure API routes in Next.js, you need to create a new file in the pages/api directory.
API routes are used to handle server-side logic, such as interacting with databases or external APIs.
You can create a new API route by creating a new file in the pages/api directory, for example, pages/api/hello.js.
A fresh viewpoint: How to save as an Html File
In this file, you can export a function that responds to GET requests, like this: export default async function handler(req, res) { res.status(200).json({ message: 'Hello World' }); }.
This will create a new API route at the root of your application that responds with a JSON object.
You can also use Next.js built-in API route handlers, such as createNextApiHandler, to simplify your code.
For example, you can use createNextApiHandler to create a new API route that responds to GET requests, like this: const handler = createNextApiHandler(); export default handler;.
This will create a new API route that responds with a JSON object.
You can customize the API route by passing options to createNextApiHandler, such as setting the default status code or adding middleware.
Saving Data from API
Saving data from an API is crucial when building a Next.js application that interacts with external data sources.
To save data from an API, you can use the useState hook in Next.js to store the data in the component's state.
When working with API data, it's essential to handle errors and exceptions that may occur during data fetching.
Next.js provides a built-in error handling mechanism using the try-catch block to catch and handle errors when fetching data from an API.
Using State Context
State context is a powerful tool for managing API requests and responses. It helps keep track of the current state of the application, making it easier to handle complex requests.
By using state context, you can avoid making multiple requests to the API when only one is needed. For example, in the "Managing API Requests" section, we learned that making multiple requests can lead to unnecessary data duplication.
State context also helps prevent errors caused by incomplete or outdated data. In the "Handling API Errors" section, we saw how using state context can help catch and handle errors more efficiently.
In the "Optimizing API Performance" section, we discovered that state context can be used to cache frequently requested data, reducing the number of requests made to the API.
Consider reading: Data Engineering Using Databricks on Aws and Azure
Handling API Responses
APIs often return responses in JSON format, which can be parsed using libraries like json.loads().
When dealing with API responses, it's essential to check for errors and exceptions, as seen in the example of the "Error Handling" section.
APIs can also return responses with multiple data types, such as JSON and XML, which requires parsing accordingly.
API responses can be paginated, requiring the use of pagination parameters like page and limit, as demonstrated in the "Paginating API Responses" section.
APIs may return responses with nested objects, which can be accessed using dot notation, as shown in the "Parsing Nested Objects" section.
API responses can be cached to improve performance, but this requires careful consideration of cache expiration times and invalidation strategies.
Example Implementation
Here's the example implementation:
To manage the loading state, we need to create a new React state variable, isRefreshing, and set it to true when we make the request.
We also need to track theData prop, which is a placeholder for whatever server data looks like, to determine when the server returns fresh data.
By putting the effect hook in place, we can terminate the loading state when the server returns fresh data, and protect against "incidental" renders.
Linking to the Example
To link to the example, you can use the anchor tag.
In the previous section, we created a simple example using HTML.
The anchor tag is used to create a hyperlink, and it has two main attributes: href and text.
In our example, we used the href attribute to specify the URL of the linked page, and the text attribute to specify the text that will be displayed.
If this caught your attention, see: Data Text Html
Code Snippets and Demos
We've got the code snippets and demos to make this example implementation come alive.
To create a loading state, we need a new React state variable, isRefreshing, which gets set to true when we make the request and false when the component re-renders with the new data.
We can put the isRefreshing state in an effect hook to track theData prop and terminate the loading state when the server returns fresh data. This protects us against "incidental" renders.
Here's what this looks like: we set isRefreshing to true when making the request, and it gets set back to false when the component re-renders with the new data.
Sources
- https://supabase.com/docs/guides/getting-started/tutorials/with-nextjs
- https://clerk.com/blog/complete-guide-session-management-nextjs
- https://www.joshwcomeau.com/nextjs/refreshing-server-side-props/
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://egghead.io/lessons/next-js-make-user-state-globally-accessible-in-next-js-with-react-context-and-providers
Featured Images: pexels.com