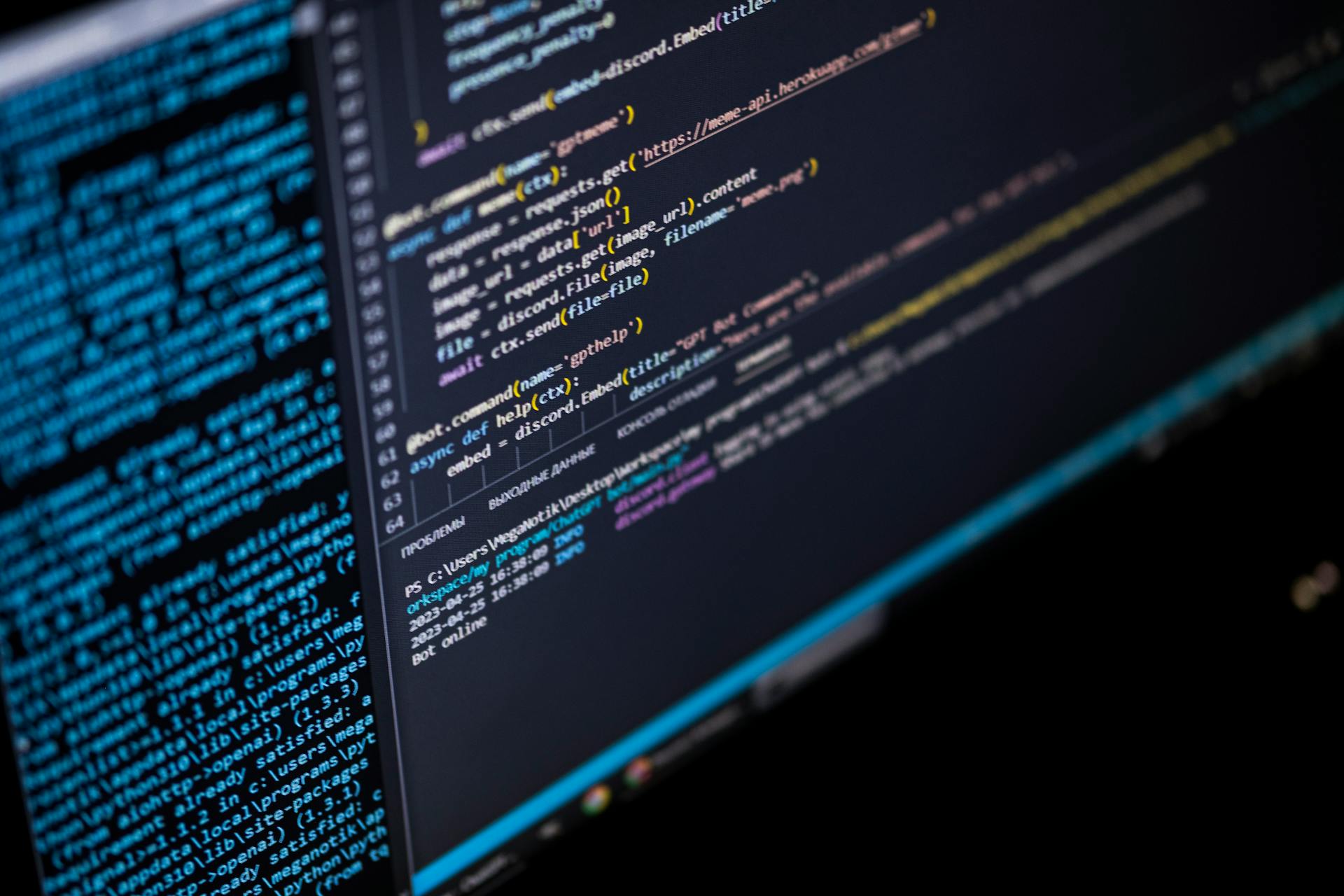
In Next.js, making API calls to another API can be a powerful way to build complex applications. By layering your project, you can optimize performance and keep your code organized.
To achieve optimal performance, consider using the `useEffect` hook to fetch data only when it's needed. This can be especially useful when working with APIs that return large amounts of data.
A well-structured project layering approach can help you avoid performance issues caused by nested API calls. By breaking down your code into smaller, modular components, you can easily manage dependencies and optimize your application's performance.
In Next.js, using the `getServerSideProps` method can help you fetch data on the server-side, reducing the load on your API and improving performance. This method is especially useful when working with APIs that return static data.
See what others are reading: Why Are Apis Important
Easy Data Caching
Next.js comes with a special implementation of the fetch() function that allows for easy data caching.
This implementation automatically caches the data returned by fetch() requests in the Data Cache on the server, reducing network usage and improving page generation time.
Suggestion: Next Js Fetch
The cache behavior depends on the optional cache option, which can be set to either force-cache or no-store.
You can also use the next.revalidate option to set the cache lifetime of a resource, specifying the number of seconds it should be in the cache.
For example, setting next.revalidate to 0 will prevent the resource from being cached.
The fetch() function in Next.js also supports all the options of the Fetch API, allowing you to customize the behavior of the API call as needed.
Here are the possible values for the cache option:
- force-cache: Before making the request, Next.js looks for a matching response in its cache. If there is a match and it is fresh, it returns that data. Otherwise, the server performs the request and updates the cache with the updated response. This is the default value.
- no-store: Next.js ignores the cache, fetching the resource on every request and not updating the cache with the retrieved content.
By implementing the API layer with the Promise-based fetch() HTTP client, you can gain access to all these powerful caching options.
Route Handlers
In a Next.js App Router application, top-level page components usually consist of several server components that retrieve data to be rendered in the HTML by making requests to API endpoints.
The API layer is the part of your Next.js Application that contains the programming logic for retrieving data via some interface on the server.
To make an API call in a Next.js server component, you can use the API layer as in the example below: ProductListComponent is a server component, and ProductAPI.getAll() will generate a server-to-server HTTP request.
Server components like ProductListComponent typically retrieve data from the API layer, which in turn makes requests to API endpoints.
The API layer is encapsulated in the api folder, which consists of several files with a specific naming convention. This convention includes the external service qualifier, which facilitates the organization of all APIs that reference the same external service or backend in the same file.
Recommended read: Next Js Call Api on the Server Example
Implementing API Calls
You can implement an API layer in Next.js with fetch to retrieve data when generating pages on the server.
To dynamically retrieve data on the frontend, integrate your Next.js application with a React API layer.
Calling an API in Next.js is straightforward, you can use the fetch API or other libraries like axios to make HTTP requests directly from your Next.js frontend code.
You can set up an API route in Next.js by creating a file inside the pages/api directory, which will be treated as an API endpoint.
Visiting /api/hello will return a JSON response with a message.
To call an external API from the frontend, use the fetch API like this.
Remember to handle the loading and error states appropriately when making API calls to ensure a smooth user experience.
Testing and Responses
To test Next.js API calls, you can use tools like Apidog, which allows you to send HTTP GET requests and see the response.
Select "GET" as the method of the request and click on the “Send” button to send the request to the API.
Apidog shows you the URL, parameters, headers, and body of the request, and the status, headers, and body of the response.
A fresh viewpoint: Nextjs Headers
Testing HTTP GET Requests
Testing HTTP GET requests is a crucial step in ensuring your API is functioning correctly.
To start, select "GET" as the method of the request.
You can then send the request to the API by clicking on the “Send” button.
Apidog will show you the URL, parameters, headers, and body of the request, and the status, headers, and body of the response.
This allows you to compare the response time, size, and format of the request and response with different web APIs.
You can also see the response time, size, and format of the request and response.
This helps you identify any issues with your API's performance and make necessary adjustments.
Check this out: Next Js Send Email
Handling Responses
Handling Responses is crucial in API routes in Next.js, as it involves sending back data to the client after processing the request.
You can handle responses in a simple way by sending back a JSON object containing user data, like in the example where a GET request is made to /api/user.
Always remember to send back a response to avoid leaving the client hanging, which includes sending back appropriate status codes and messages.
Worth a look: Nextjs save Data Pulled from Api Using State Context
To handle errors, you can use try...catch blocks to catch any errors that occur during the request processing and send an appropriate response, like a 500 Internal Server Error status, along with a JSON object describing the error.
If a different method is used, the server will return a 405 Method Not Allowed status, so be sure to check the method being used.
Remember to log the error so you can diagnose the issue later, and always send back a response to the client.
API Call Basics
API calls are a fundamental part of building complex software systems, and Next.js makes it easy to make API calls to both internal and external APIs.
You can use the fetch API or libraries like axios to make HTTP requests directly from your Next.js frontend code. This is a straightforward process, and you can even use the built-in API routes feature to create server-side API endpoints.
See what others are reading: Nextjs React Query
APIs, or Application Programming Interfaces, are a set of protocols and tools that allow different software applications to communicate with each other. They enable developers to use functionalities of one application within another by sending requests and receiving responses.
There are different types of APIs, such as REST, SOAP, and GraphQL, each with its own set of rules and use cases. APIs define the methods and data formats for requesting and sending information between systems.
To ensure a smooth user experience, remember to handle the loading and error states appropriately when making API calls.
See what others are reading: Next Js Express
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://nextjs.org/docs/app/building-your-application/routing/route-handlers
- https://nextjs.org/docs/pages/building-your-application/routing/api-routes
- https://semaphoreci.com/blog/next-js-site-api-layer
- https://apidog.com/blog/next-js-call-api/
Featured Images: pexels.com