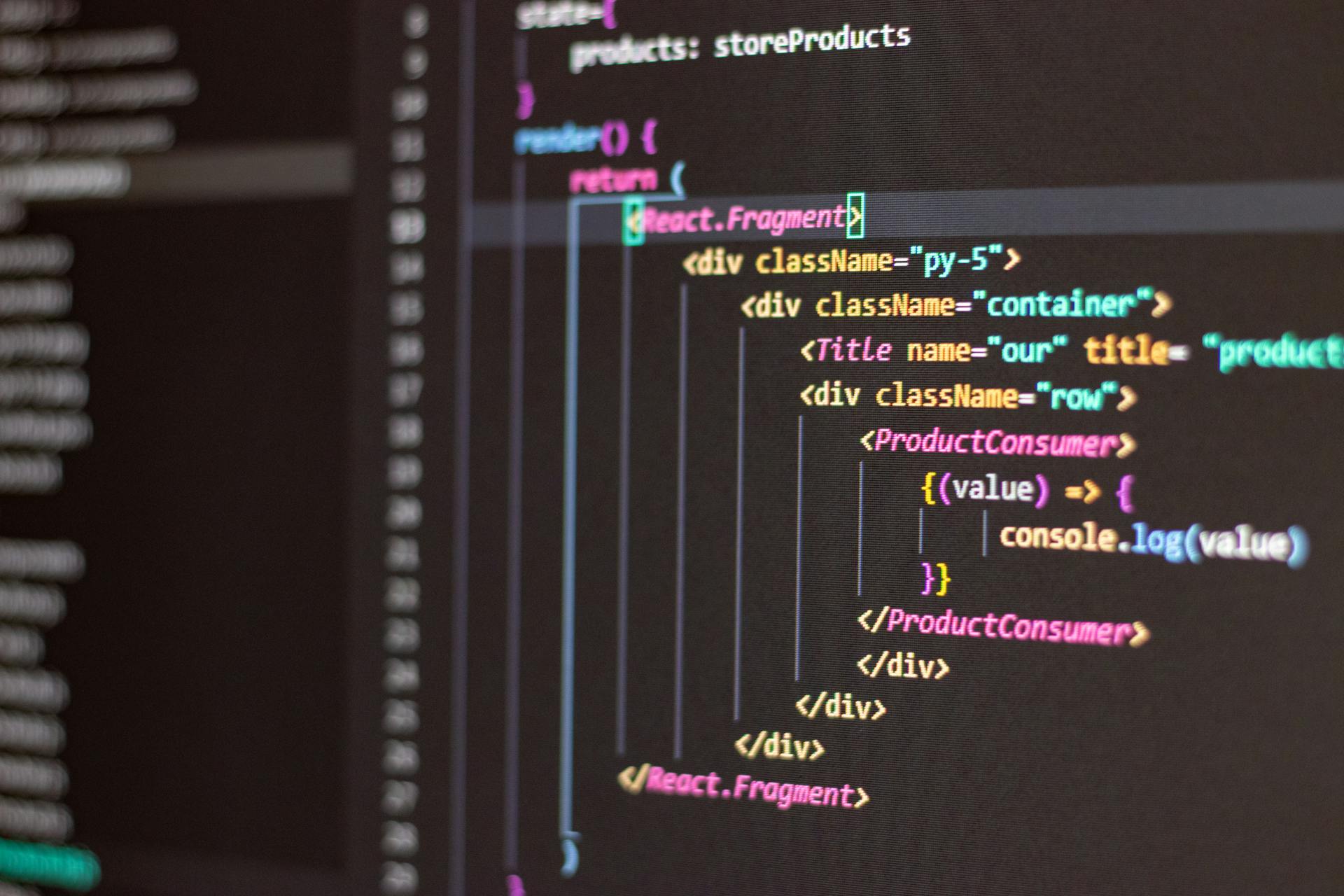
To send email with Next.js and Nodemailer, you'll need to install the Nodemailer package using npm or yarn.
You can do this by running the command npm install nodemailer in your terminal. This will install the necessary dependencies for sending emails with Nodemailer.
Nodemailer is a popular email transport agent for Node.js, and it's widely used in production environments. It supports various email services like Gmail, Outlook, and more.
To set up Nodemailer in your Next.js project, you'll need to create a new file for your email configuration. This file will contain the necessary settings for your email service provider.
Explore further: Install Next Js in React
Project Setup
To set up your Next.js project, you'll need to follow a few steps. First, installation will prompt you with a series of questions.
You'll then see a folder created with your project name and the required dependencies installed. Next, you'll need to install packages for sending emails using React Email and Resend Email API.
For more insights, see: Azure Send Email
Here are the packages you'll be using:
- resend: This service will be used to send emails.
- react-email: This package allows you to create email templates using React Js.
- @react-email/render: This package converts React Js based templates to an HTML string.
A .env.local file will need to be created in the root of your project. In this file, paste the Resend API key you created earlier.
Creating a Form
To create a simple Next.js form, you'll want to create a file named actions.ts in the src folder or the root of your project.
This file will contain the code that handles the form submission. You'll need to update the API endpoint to match where you created your file and update the body values to correspond to your own contact form.
Within app/page.tsx, you'll need to copy some code to get started. This code will communicate with the API endpoint and send an email via nodemailer.
To send an email, you'll need to create a folder called components/ at the same level as the app/ directory. Inside this folder, create a file named ContactForm.tsx.
This file will contain a basic form that takes in the user's email and message. When the user submits the form, the code inside route.ts will be executed, sending an email to the entered email.
Consider reading: Nextjs Api Call Another Api
Here's a brief overview of the steps to create the Contact Form:
- Create a file named ContactForm.tsx inside the components/ directory.
- Copy the code for ContactForm.tsx, which includes a basic form that takes in the user's email and message.
- When the user submits the form, the code inside route.ts will be executed, sending an email to the entered email.
NextJS Server Action
NextJS Server Action is a game-changer for handling form submissions and email sending.
It's an asynchronous function that runs on the server, making it easier to handle sensitive logic like communicating with the database. This means you don't have to create API routes for such tasks.
The sendEmail function is a server action that receives the previous state and the formData, giving you access to the form values. It uses resend to send the email to the provided email address.
You need to use the verified email address from your Resend Email API account in the from field to ensure successful email delivery. This is crucial for email sending to work correctly.
In some cases, the react property might not work, and you might receive an error while sending emails. If that happens, you can convert the React component into an HTML string to resolve the issue.
To use NextJS Server Action with a form, you need to use the useFormState hook, which accepts the action and the initial state. This hook returns the new state and the action function that you can attach to your form.
Broaden your view: Nextjs save Data Pulled from Api Using State Context
API Route Creation
API routes are a crucial part of sending emails in Next.js, and we're going to create one using the App Router and Next.js 13.
To create a custom request handler, we'll use the app directory and create a new file called `route.jsx` in the `src/app/api/contact` directory. This is where the magic happens.
In this file, we'll import `NextResponse` and `nodemailer`, and retrieve the form data from the request. We can handle the `FormData` object directly without needing to change it to JSON.
Here's a summary of the steps to create the API route:
- Create a new file called `route.jsx` in the `src/app/api/contact` directory
- Import `NextResponse` and `nodemailer`
- Retrieve the form data from the request
- Handle the `FormData` object directly
With these steps, we'll have a functional API route that can handle form submissions and send emails using Nodemailer.
Integrate with Router
The App Router is a new paradigm for building applications using React Server Components.
You can integrate Resend with the App Router and the latest versions of Next.js.
Front End
To start building the contact form, we need to add the shell of the form within src/app/contact/page.jsx. You can access your app via http://localhost:3000/ after running the code.
Next, we'll create the event handler for when you submit the form, which is a crucial step in making the form functional.
You might like: Next Js Formdata
Event Handling
Event handling is a crucial part of sending emails in Next.js.
You'll need to prevent the browser from reloading the page after submitting the form, which is done using event.preventDefault().
The event handler sends a POST request to the /api/email endpoint with the data contained in the formData object.
It's essential to wrap the code that sends the email in a try-except block to catch any errors that may occur.
To send the email, you'll need to create a nodemailer object.
Marking your onSubmit function as async is necessary because you'll be using the await statement to send the email.
The await statement is used to wait for the email to be sent before proceeding with the next line of code.
The API endpoint and body values should be updated based on your specific contact form.
Check this out: Nextjs Forms
Sources
- https://resend.com/nextjs
- https://medium.com/@jamesowen.dev/how-to-send-email-forms-using-next-js-13-with-nodemailer-1bc9e5dd5e80
- https://ekomenyong.com/insights/nextjs-resend-contact-form
- https://mydevpa.ge/blog/how-to-send-emails-using-next-14-resend-and-react-email
- https://blog.stackademic.com/automate-200-emails-daily-nodemailer-next-js-13-integration-c7773ab63d5d
Featured Images: pexels.com