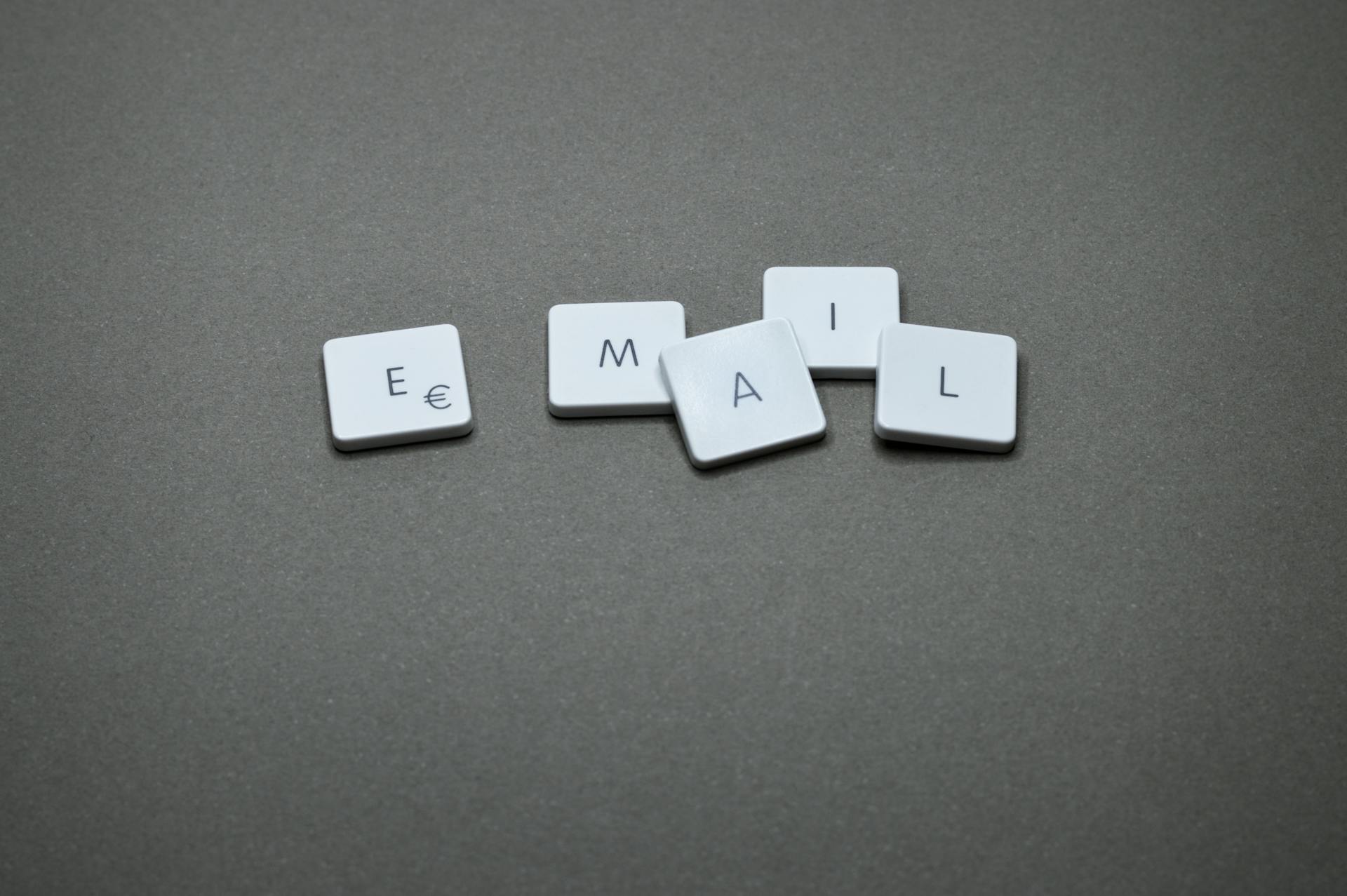
Sending emails through Azure is a straightforward process that can be easily integrated into your applications.
You can use the Azure SendGrid service to send emails, which provides a simple API for sending emails.
To get started, you'll need to create a SendGrid account and obtain an API key, which is required for sending emails.
With Azure SendGrid, you can send emails using various formats, including plain text and HTML.
Worth a look: Php Contact Form Send Email Codepen
Prerequisites
To get started with sending emails using Azure, you'll need to meet a few prerequisites.
You'll need an Azure account with an active subscription, which you can create for free.
You'll also need the latest version of the .NET Core client library for your operating system.
To send emails, you'll require an Azure Email Communication Services Resource that's been created and is ready to go, along with a provisioned domain.
Additionally, you'll need an active Communication Services resource connected with your Email Domain.
For another approach, see: Azure Domains
You can create these resources by following the links provided in the article.
Here's a quick rundown of what you'll need:
- Azure account with an active subscription
- Latest version of .NET Core client library for your operating system
- Azure Email Communication Services Resource with a provisioned domain
- Active Communication Services resource connected with Email Domain
Note that completing this quick start will incur a small cost of a few USD cents or less in your Azure account.
Sending Email
To send an email using Azure, you'll need to create an Email Communication Service resource and connect it to your Azure Communication Service resource. This will allow you to use the Email API to send emails.
You can send emails using the Email API, which is a part of the Azure Communication Services. This API allows you to send emails to multiple recipients and track the delivery status of your emails. The Email API also supports custom email headers, attachments, and reply-to addresses.
To send an email, you'll need to construct your email message with the sender's information, recipient's information, and the email content. You can use the EmailMessage object to define the email message, which includes properties such as attachments, content, headers, recipients, reply-to addresses, and sender address.
You might like: Azure Email
You can use the SendAsync method to send the email, which takes the EmailMessage object as a parameter. This method returns a response object that contains the message ID, which you can use to track the delivery status of your email.
Here are the steps to send an email using Azure:
1. Create an Email Communication Service resource and connect it to your Azure Communication Service resource.
2. Construct your email message using the EmailMessage object.
3. Call the SendAsync method to send the email.
4. Track the delivery status of your email using the message ID.
Here are the optional parameters you can use when sending an email:
- `--html`: Use HTML email body instead of text.
- `--importance`: Set the importance type for the email (high, normal, or low).
- `--to`: Set the list of email recipients.
- `--cc`: Set carbon copy email addresses.
- `--bcc`: Set blind carbon copy email addresses.
- `--reply-to`: Set Reply-To email address.
- `--disable-tracking`: Disable user engagement tracking for this request.
- `--attachments`: Set the list of email attachments.
- `--attachment-types`: Set the list of email attachment types.
Here's an example of how to send an email using Azure:
```csharp
EmailMessage emailMessage = new EmailMessage();
emailMessage.SenderAddress = "your-email-address";
emailMessage.Recipients = new[] { "recipient-email-address" };
emailMessage.Content = "Hello, this is a test email.";
emailMessage.Attachments = new[] { new EmailAttachment("attachment.txt") };
Response response = await emailService.SendAsync(emailMessage);
string messageId = response.MessageId;
```
Check this out: Next Js Send Email
Authentication and Security
Authentication and Security is a crucial aspect of Azure Send Email. You can authenticate an email client in a few different ways: Connection String, Azure Active Directory, or AzureKeyCredential.
There are three environment variables that Azure Identity SDK reads at runtime to authenticate an application: COMMUNICATION_SERVICES_CONNECTION_STRING, AZURE_TENANT_ID, and AZURE_CLIENT_ID.
To authenticate using Azure Active Directory, you'll need to create an Azure Active Directory Registered Application and set the environment variables. This will allow your application to use DefaultAzureCredential to authenticate the email client.
Client Authentication
Client authentication is a crucial step in setting up an email client with Azure Communication Services. There are a few different options available, each with its own unique approach.
One option is to use a connection string, which can be retrieved from an environment variable named COMMUNICATION_SERVICES_CONNECTION_STRING. This variable is used to authenticate the email client and connect to the Azure Communication Services resource.
Another option is to use Azure Active Directory, which requires installing the Azure.Identity library package. This package allows you to authenticate using DefaultAzureCredential, which reads values from three environment variables at runtime.
Additional reading: What Are Linked Services in Azure
AzureKeyCredential is also a viable option, which can be used to authenticate an email client. The key and endpoint can be found on the "Keys" pane under "Settings" in your Communication Services Resource.
Here are the different authentication options summarized:
- Connection String: retrieved from COMMUNICATION_SERVICES_CONNECTION_STRING environment variable
- Azure Active Directory: uses DefaultAzureCredential and three environment variables
- AzureKeyCredential: key and endpoint found on "Keys" pane under "Settings" in Communication Services Resource
Each of these options has its own unique benefits and trade-offs, and the choice ultimately depends on your specific use case and requirements.
SMTP Restrictions
If you're using Azure for your computing needs, be aware that there are some SMTP restrictions in place. Since November 15, 2017, Microsoft has banned outbound SMTP communication from Azure computing resources in trial subscriptions.
This restriction applies to unauthenticated email delivery via SMTP port 25 through direct DNS MX lookups. The goal is to protect Azure data center IP addresses from reputation abuse.
If you're an enterprise user, don't worry - this restriction doesn't affect you. However, if you're a trial subscriber, you can still send emails from Azure, but you'll need to use an SMTP relay service.
Discover more: Windows Azure Trial
Pay-As-You-Go subscribers can unblock the restriction by sending a support request to Microsoft, which will be approved if everything checks out.
Here are some alternatives to consider:
You can also use Azure Logic Apps to send emails with Mailtrap via SMTP as a response to specific events. For example, if someone adds a new tweet, the Logic Apps will send an email automatically.
You might like: Azure Functions vs Logic Apps
Throttling
Throttling can occur when sending emails, causing your application to hang.
This is often handled through logging or implementing a custom policy.
If you're experiencing throttling, you can request to increase your sending volume by submitting a support request.
You can do this once your application is ready to go live and you need to send a volume of messages exceeding the rate limits.
The initial sandbox setup is designed to help developers start building their application, but it may have limitations on sending volume.
Delivery and Status
To get the status of an email delivery, you can poll for the status of the operation by setting a loop on the operation status object returned from the EmailClient's begin_send method.
The EmailSendOperation object contains an EmailSendStatus object that contains the current status of the Email Send operation, as well as an error object with failure details if the current status is in a failed state.
You can refresh the email operation status by calling the UpdateStatusAsync method.
The EmailSendStatus object can have one of the following statuses: Canceled, Failed, NotStarted, Running, or Succeeded.
Here's a list of the possible delivery states you can get from the EmailDeliveryReportReceived event:
- Delivered.
- Failed.
- Quarantined.
Additionally, you can subscribe to Email Operational logs that provide information related to delivery metrics for messages sent from the Email service.
The EmailSendResult object contains the status of a long-running operation, including an error object with failure details if the status is a non-success terminal state.
The EmailSendResult object has the following properties:
By referencing the EmailSendResult object, you can get the actual email delivery status, which includes the delivery state, such as Delivered, Failed, or Quarantined.
Optional Features
Optional Features can really make a big difference in how you send emails through Azure. You can choose to send an HTML email body instead of plain text.
To set the importance type for your email, you can use the --importance parameter and select from high, normal, or low. Normal is the default setting.
You can also specify a list of email recipients with the --to parameter. And, you can use a list of recipients with --cc and --bcc, similar to --to, with at least one recipient required.
Attachments can be added to your email with the --attachments parameter. You can also specify the types of attachments with the --attachment-types parameter, listing the types in the same order as the attachments.
By default, user engagement tracking is enabled, but you can disable it with the --disable-tracking parameter. The Reply-To email address can be set with the --reply-to parameter.
Third-Party Services
Using a third-party service can be a great way to send emails from Azure. Azure Communication Service Email is still under public review and can't be used in production until it becomes generally available.
There are three main reasons why you might need a third-party SMTP service: Azure email has limitations when it comes to sending volume, and users can send only a certain number of emails per day. Microsoft also doesn't allow outbound SMTP communication from Azure, which can affect free Azure subscribers.
To send emails from Azure, you can use an authenticated SMTP relay service, also known as a Smart Host, with TLS support. This provides an intermediary SMTP server between the mail servers of the sender and recipient, reducing the likelihood that an email provider will reject your email.
Suggestion: Azure Smtp Service
Grid Integration
Integrating SendGrid into your Azure environment is a straightforward process. You'll first see a Deployment Succeeded pop-up, and your account will be listed in SendGrid Accounts.
To get started, you'll need to enter your brand-new account and click the Manage button to initiate the email verification process. SendGrid will send you a confirmation email to your address.
You might enjoy: Sendgrid in Azure
You can opt for SendGrid SMTP API, which requires generating an API Key. To do this, click Manage=> Settings=> API Keys.
Once you've clicked Create API Key, provide a name for the key and select a permission, then click Create & View once ready.
Your API Key will be displayed, so be sure to copy and save it – you won't be able to see it again.
Here's a quick rundown of the steps:
- Deployment Succeeded pop-up displays your account in SendGrid Accounts.
- Enter your account, click Manage, and initiate email verification.
- Generate an API Key by clicking Manage=> Settings=> API Keys.
- Create & View your API Key, and copy it before it's gone.
Benefits of Third-Party Services
Using third-party services can be a game-changer for your email sending needs. They offer a more reliable and secure way to send emails, especially when compared to Azure Communication Service Email, which is still in public review and not suitable for production yet.
Azure Communication Service Email has limitations on sending volume, and users can only send a certain number of emails per day. This is a major drawback for businesses that need to send high volumes of emails.
Microsoft also restricts outbound SMTP communication from Azure to protect Azure data center IP addresses from reputation abuse. This restriction affects users with free Azure subscriptions, but pay-as-you-go subscribers can unblock it with Microsoft's approval.
The solution to these limitations is to use an authenticated SMTP relay service, also known as a Smart Host, with TLS support. This provides an intermediary SMTP server between the mail servers of the sender and recipient.
Using a third-party SMTP service like Mailtrap Email Sending can help maintain your sender reputation and reduce the likelihood of email rejection. It's a more secure and reliable option than relying on Azure Communication Service Email.
Here are some key benefits of using a third-party SMTP service:
Overall, using a third-party SMTP service like Mailtrap Email Sending is a better option than relying on Azure Communication Service Email, especially for businesses that need to send high volumes of emails.
Via SendGrid
Via SendGrid, you can send emails from Azure with ease. SendGrid is a third-party SMTP service that can help you overcome the limitations of Azure Communication Service Email.
Azure Communication Service Email is still under public review and can't be used in production until it becomes generally available. This means you can't rely on it for sending emails in your app.
If you're a free Azure subscriber, you'll face another limitation: Microsoft doesn't allow outbound SMTP communication from Azure to protect Azure data center IP addresses from reputation abuse. This restriction affects unauthenticated email delivery via SMTP port 25 through direct DNS MX lookups.
To send emails from Azure, you can use a third-party SMTP service like SendGrid, which provides an authenticated SMTP relay service with TLS support. This service reduces the likelihood of your email being rejected by the recipient's email provider.
Here are the steps to send emails from Azure via SendGrid:
1. Create an account on SendGrid and get your API key.
2. Use your SendGrid SMTP server credentials to send emails via PowerShell or Azure Logic Apps.
3. You can also use Azure Automation runbooks to send emails with SendGrid.
By using SendGrid, you can overcome the limitations of Azure Communication Service Email and send emails from Azure with confidence.
Gmail
Gmail integration can be a bit tricky, but don't worry, I've got you covered.
You have two options to send emails from Azure with Gmail: creating an SMTP action or a connector.
To create an SMTP action, you need to insert the credentials for your Gmail's SMTP server. This is similar to what we did with Mailtrap Email API.
Creating a connector is another option, where you can use 'When a HTTP request is received' as a trigger and choose Gmail and 'Send Email' as an action.
When you choose this option, you'll be redirected to a window where you need to sign into your Google account.
Once you're signed in, you can choose the To addresses and compile an email by selecting parameters from the dropdown menu.
How to Test
Testing is a crucial part of sending emails from Azure, and you'll want to make sure your emails are bug-free before sending them to recipients.
To test emails in Azure, you can use Mailtrap Email Testing, which is an Email Sandbox for inspecting and debugging emails in staging and dev environments. This tool allows you to validate HTML/CSS, test Bcc email headers, view emails in raw and text formats, and analyze them for spam and blacklists.
You can integrate Mailtrap Email Testing with your Azure app via an API or SMTP, or send emails directly to your inbox. To start, sign in to your Mailtrap account and navigate to the 'Email Testing' tab.
To integrate Mailtrap with your Azure app, select your preferred inbox, open the 'SMTP Settings' tab, and choose the language you're using with your Azure app from the dropdown menu. For example, if you're using C#, you can use the following sample code:
See what others are reading: Azure Web App Container
```
For SMTP integration, press 'Show Credentials' in the 'SMTP Settings' tab. Copy credentials and use them to send emails from your email client.
```
Alternatively, you can send emails directly to Mailtrap's inbox by navigating to the 'Email Address' tab and copying the email address you'll see there. This feature is available for Business, Premium, and Enterprise plans.
Here are the steps to test your email sending in Azure:
- Connect to the SMTP relay server
- Send an email from it without authentication
- If the email is sent successfully, your SMTP server is an open relay and needs to be fixed
Note that you can't use Mailtrap for sending test emails from Azure for now, but you can use the Mail Server Testing Tool to test the SendGrid SMTP relay.
Sources
- https://learn.microsoft.com/en-us/azure/communication-services/quickstarts/email/send-email
- https://faun.dev/c/stories/nataliiapolomkina/the-most-advanced-guide-on-sending-emails-from-azure/
- https://learn.microsoft.com/en-us/rest/api/communication/dataplane/email/send
- https://practical365.com/ecs-email-communication-services/
- https://mailtrap.io/blog/azure-send-email/
Featured Images: pexels.com